Vector operations in C++ allow you to easily manipulate and manage dynamic arrays with built-in methods for adding, removing, and accessing elements.
Here’s a simple example that demonstrates basic vector operations, such as creating a vector, adding elements, and accessing them:
#include <iostream>
#include <vector>
int main() {
std::vector<int> v; // Create a vector of integers
v.push_back(10); // Add element 10
v.push_back(20); // Add element 20
std::cout << v[0] << ", " << v[1]; // Access and print elements
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array that can grow or shrink in size. Unlike traditional arrays, vectors provide flexibility and ease of use. They can hold elements of the same data type and offer features such as automatic resizing, making them an essential part of the Standard Template Library (STL).
Comparison with Arrays: Vectors outperform arrays in several ways:
- Dynamic sizing: Vectors can adjust their size at runtime, while arrays have a fixed size once declared.
- Convenience: Vectors come with a variety of built-in functions, reducing the need for manual size management.
- Safety: Vectors provide methods like `.at()` that perform bounds checking, which can prevent runtime errors.
Declaring Vectors
To declare a vector in C++, the syntax is simple. You start by including the `<vector>` header and then use the `std::vector` template.
Example Code Snippet:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector; // Declaration of a vector of integers
return 0;
}
Explanation: This code snippet declares an empty vector named `myVector` that is designed to hold integers. Understanding how to initialize vectors is fundamental for performing vector operations in C++.
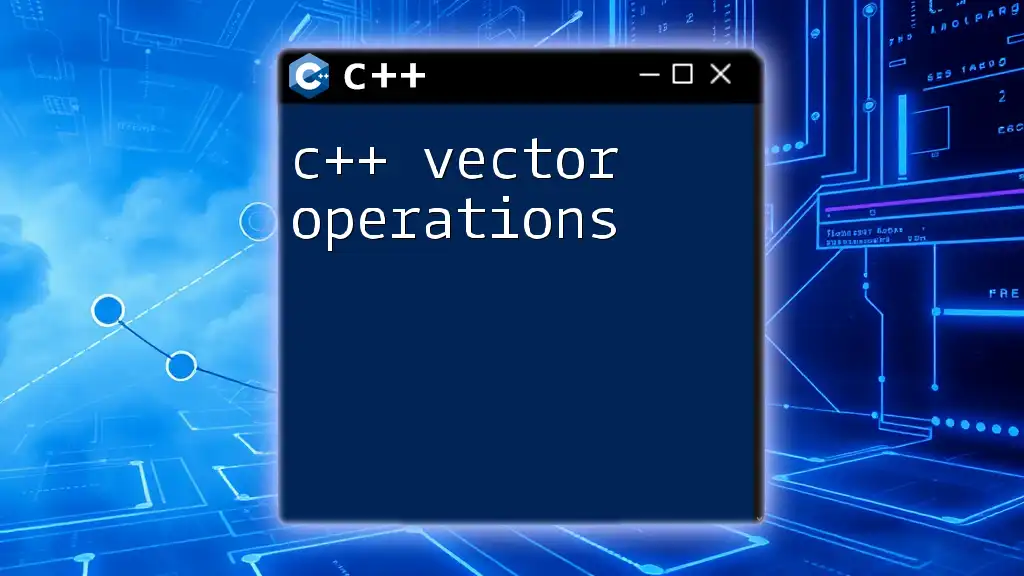
Basic Vector Operations
Adding Elements
Adding elements to a vector is straightforward. You can use the push_back() method or the emplace_back() method.
Example Code Snippet:
myVector.push_back(10); // Adds 10 at the end of the vector
myVector.emplace_back(20); // Constructs and adds 20 in place
Explanation:
- push_back() adds an element to the end of the vector. It is convenient for adding values, but it involves a copy operation.
- emplace_back() constructs a new element in place, making it more efficient when dealing with complex data types.
Accessing Elements
You can access elements in a vector using the operator[] or the at() method.
Example Code Snippet:
std::cout << myVector[0]; // Access using operator[]
std::cout << myVector.at(1); // Safe access with at()
Explanation:
- The operator[] method provides quick access but does not perform any boundary checks, which can lead to potential runtime errors.
- The at() method, on the other hand, provides safe access by throwing an `std::out_of_range` exception if the index is invalid.
Removing Elements
Removing elements can be done using pop_back(), erase(), and clear().
Example Code Snippet:
myVector.pop_back(); // Removes last element
myVector.erase(myVector.begin() + 1); // Removes element at index 1
myVector.clear(); // Clears all elements
Explanation: Each of these methods serves a specific purpose:
- pop_back() eliminates the last element in the vector.
- erase() removes a specific element based on its position.
- clear() removes all elements from the vector, effectively resetting it.
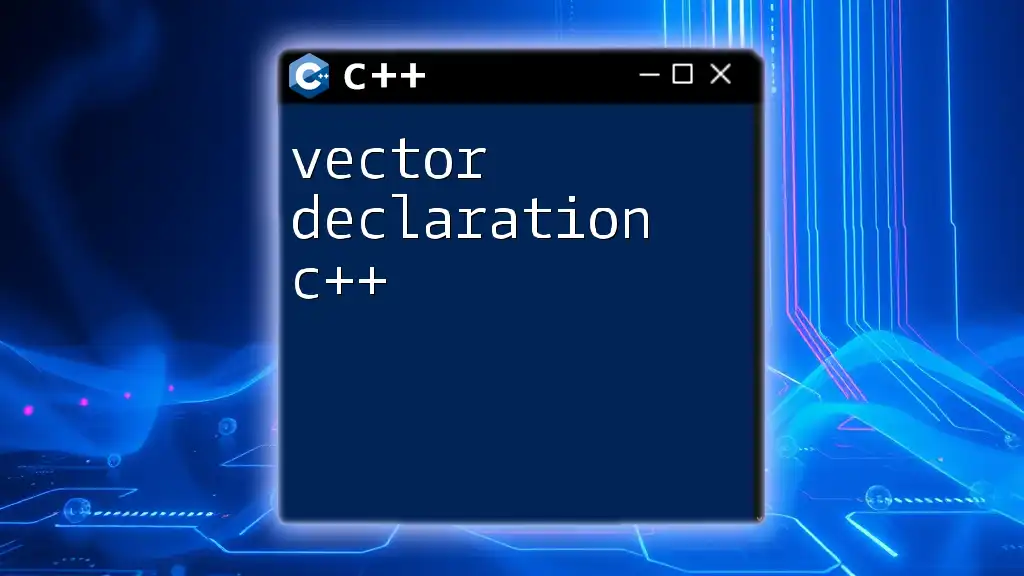
Advanced Vector Operations
Iterating through Vectors
Iterating through a vector can be efficiently done using traditional for loops or more modern range-based for loops.
Example Code Snippet:
for (int num : myVector) {
std::cout << num << " "; // Range-based for loop
}
Explanation: Range-based for loops make it easier to iterate through all the elements in a vector without the need for an index variable, improving readability and reducing the chances of errors.
Sorting and Searching
You may want to sort or find elements within a vector. C++ STL provides functions like sort() and find() for these operations.
Example Code Snippet:
#include <algorithm> // Required for sort and find
#include <vector>
#include <iostream>
std::vector<int> myVector = {5, 2, 3, 1, 4};
std::sort(myVector.begin(), myVector.end());
auto it = std::find(myVector.begin(), myVector.end(), 3);
Explanation:
- The sort() function sorts the elements between the two specified iterators.
- The find() function searches for a specific value, returning an iterator to the element if found.
Resizing and Capacity
Understanding how to manage the size and capacity of a vector is critical for performance optimization. You can use the resize() and reserve() methods.
Example Code Snippet:
myVector.resize(5); // Resizes vector to have 5 elements
myVector.reserve(10); // Reserves space for at least 10 elements
Explanation:
- resize() changes the size of the vector, and if the new size is larger, new default-initialized elements are added.
- reserve() allocates memory for future elements without changing the current size, which is useful for performance optimization in scenarios where the size of the vector is known in advance.
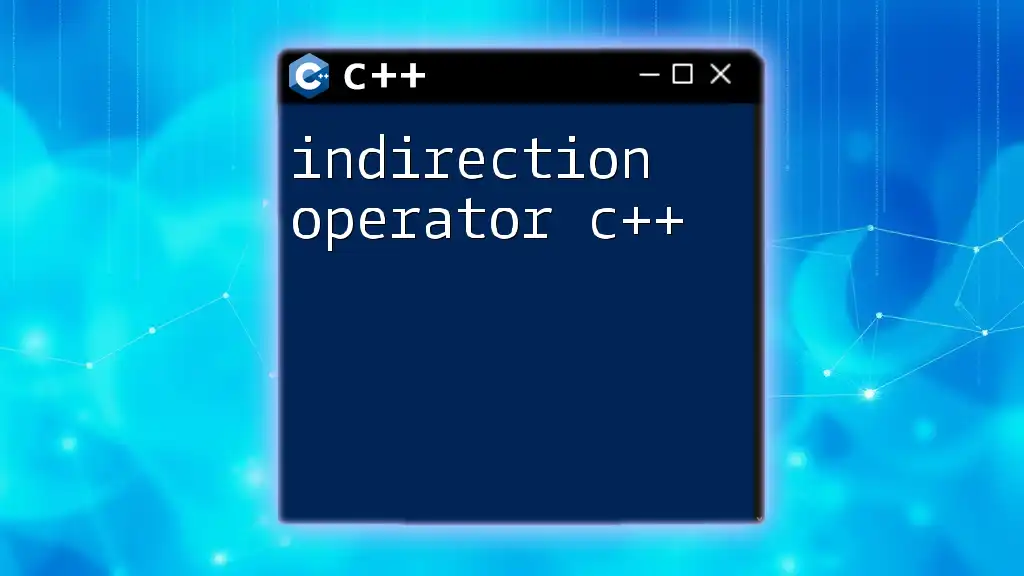
Specialized Vector Operations
Multi-Dimensional Vectors
C++ allows you to create multi-dimensional vectors by nesting vectors. This is particularly useful for applications like matrices.
Example Code Snippet:
std::vector<std::vector<int>> matrix(3, std::vector<int>(3)); // 3x3 matrix
Explanation: In this example, we create a 3x3 matrix where each element is a vector. This approach allows for easy manipulation and traversal of multi-dimensional data.
Using Vectors with Algorithms
One of the powerful features of vectors is their compatibility with algorithms defined in the STL.
Example Code Snippet:
#include <vector>
#include <algorithm> // For transform
#include <iostream>
std::vector<int> result;
std::transform(myVector.begin(), myVector.end(), std::back_inserter(result), [](int x) { return x * 2; });
Explanation: The std::transform function applies a transformation to each element in the vector, showcasing how vectors can be manipulated efficiently using functional programming techniques in C++.
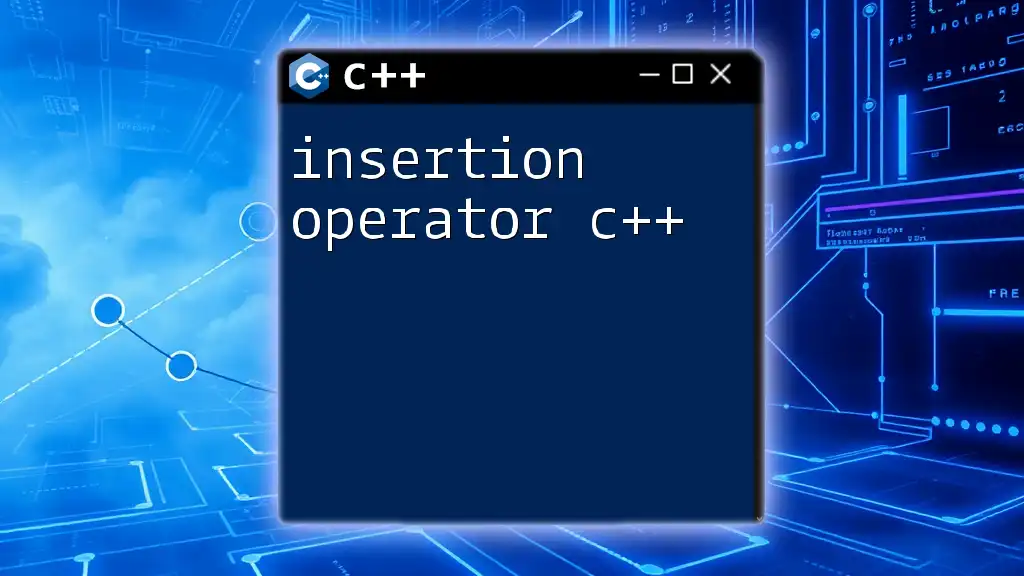
Best Practices and Common Pitfalls
Performance Considerations
While vectors are versatile, understanding when to use them versus other containers (such as lists or deques) is crucial for optimizing performance. For instance, when frequent insertions and deletions are necessary, a list may be more efficient.
Common Mistakes
- Forgetting to include the `<vector>` header: Always remember to include this header before using vectors.
- Improper index usage: Using an index that exceeds the vector size can lead to undefined behavior if you use operator[].
- Mixed types in vectors: Vectors can only store elements of the same data type, which is a fundamental rule of type safety in C++.
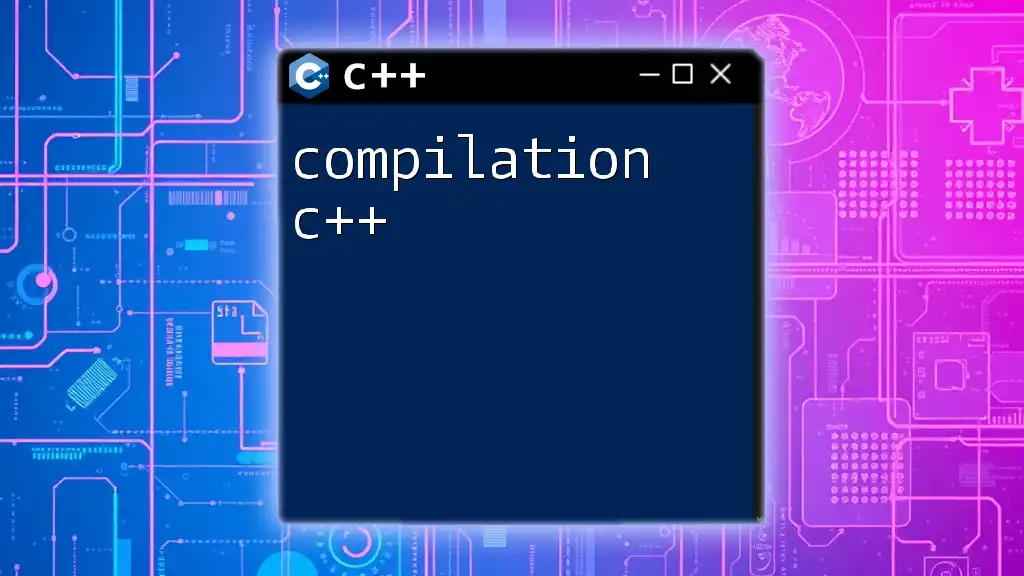
Conclusion
Mastering vector operations in C++ is foundational for leveraging the power of the language and the STL. By understanding the functionalities offered by vectors, you can write more efficient and safer code. Explore various vector operations, experiment with different examples, and elevate your programming skills.
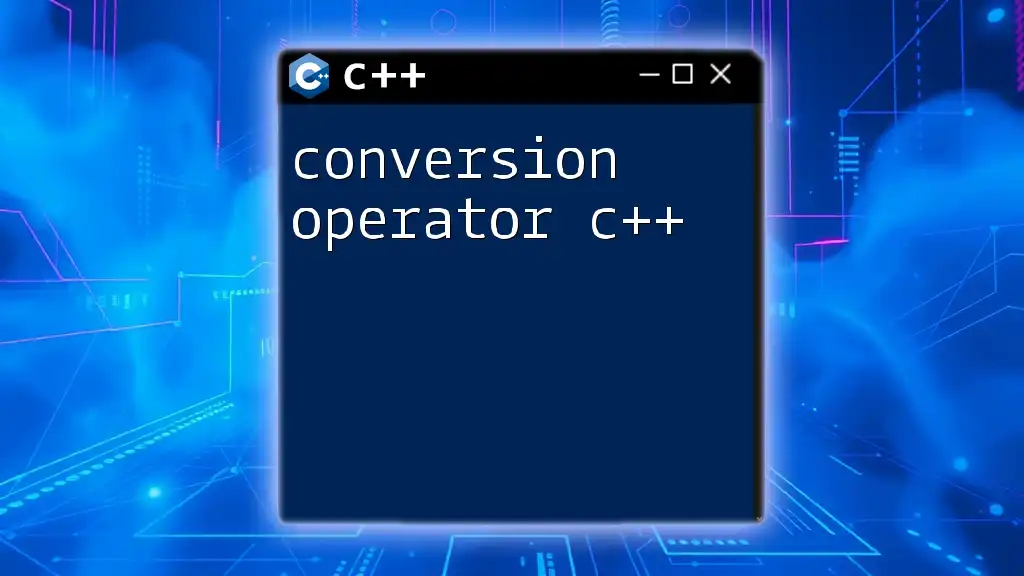
Additional Resources
To deepen your understanding of vector operations in C++, consider exploring documentation on C++ STL, reputable coding challenge platforms, and insightful books that cover advanced C++ topics. Engaging with the community can also enhance your learning journey.