In C++, a boolean operator is a function that allows you to define how two objects of a class interact with the logical operators (such as `&&`, `||`, and `!`), often by overloading the `operator bool`.
Here's a simple code snippet demonstrating how to overload the boolean conversion operator:
#include <iostream>
class MyBoolean {
public:
MyBoolean(int value) : value(value) {}
// Overload the boolean conversion operator
operator bool() const {
return value != 0; // Non-zero value is true
}
private:
int value;
};
int main() {
MyBoolean obj(5);
if (obj) {
std::cout << "The object is true!" << std::endl;
} else {
std::cout << "The object is false!" << std::endl;
}
return 0;
}
Understanding Boolean Data Type
The boolean data type in C++ consists of two values: `true` and `false`. These values are integral to making decisions in programming, as they represent the outcome of conditions and logical expressions.
In programming, booleans play a critical role in controlling the flow of execution. For instance, they are often used in `if` statements and loops to determine whether or not a block of code should execute. An example of a boolean expression is:
bool isEven = (number % 2 == 0); // true if number is even
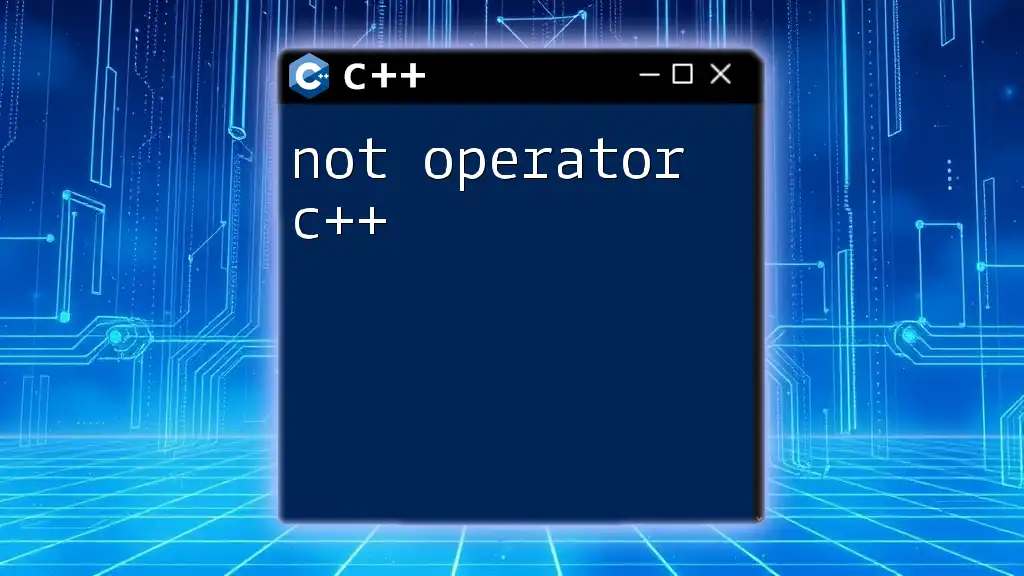
What are Operators in C++?
Operators are symbols that perform operations on variables and values. They are categorized into various types, including arithmetic, relational, logical, and more.
Boolean Operators in C++
Boolean operators specifically deal with true/false values and include AND, OR, and NOT. These operators can combine multiple conditions and produce a single boolean result.
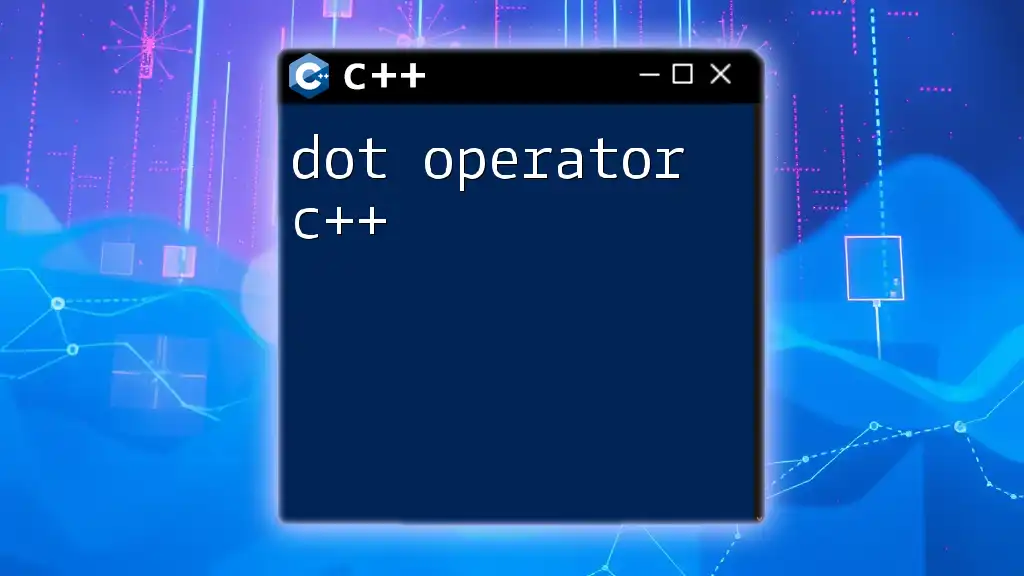
Understanding the bool Operator in C++
What is the `bool` Operator?
The `bool` operator in C++ is utilized to define boolean variables. It essentially allows you to specify condition checks straightforwardly. Unlike other data types, booleans can only hold `true` or `false`.
A typical declaration of a boolean variable looks like this:
bool isAvailable = true;
You can assign values based on conditions or directly use boolean literals.
Syntax of the bool Operator
The syntax for declaring boolean variables involves using the `bool` keyword followed by the variable name and an assignment of either `true` or `false`. For example:
bool isRaining = false;
This straightforward declaration makes it easy to handle conditional logic in your code.
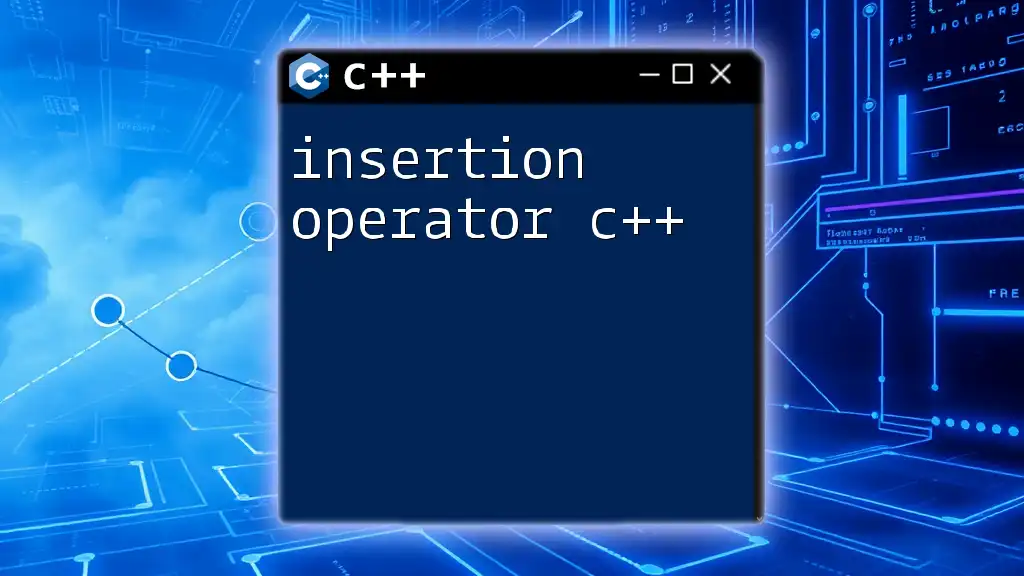
Boolean Expressions in C++
Creating Boolean Expressions
A boolean expression is an expression that evaluates to either `true` or `false`. They are created using relational and logical operators. Commonly used relational operators include `==`, `!=`, `>`, and `<`.
Here’s an example of a boolean expression:
bool isGreater = (5 > 3); // Evaluates to true
bool isEqual = (10 == 10); // Evaluates to true
Evaluating Boolean Expressions
C++ evaluates boolean expressions based on operator precedence. Moreover, C++ utilizes short-circuit evaluation in logical operations. For instance, in the expression `A && B`, if A is `false`, C++ will not evaluate B because the overall result cannot be `true`.
This can be demonstrated with the following code:
bool condition1 = (5 > 3); // true
bool condition2 = (5 / 0 == 0); // This will not be executed due to short-circuit
bool result = (condition1 && condition2); // result will be false
Short-circuit evaluation is particularly beneficial in preventing runtime errors, such as division by zero.
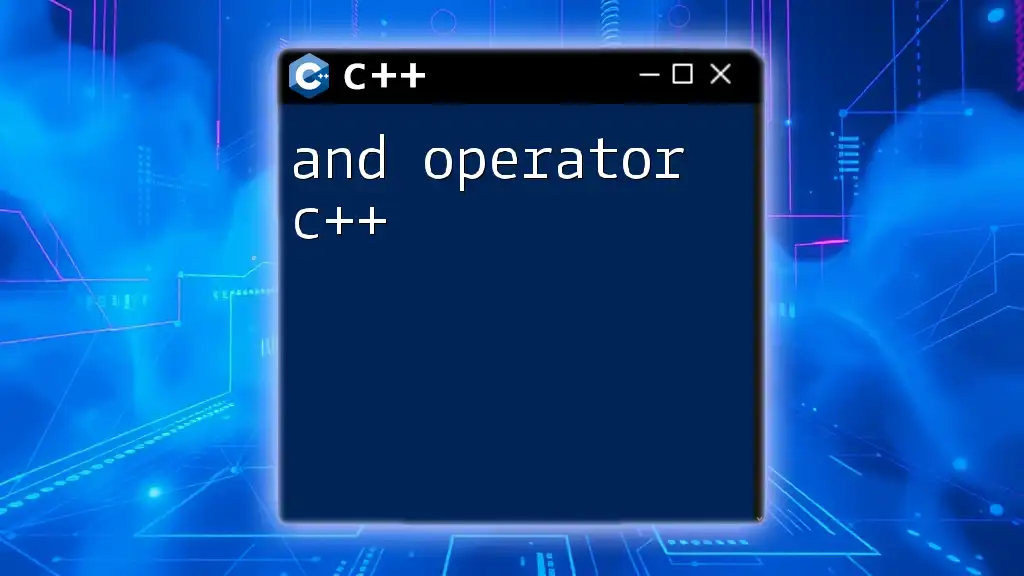
Operator Overloading for bool in C++
What is Operator Overloading?
Operator overloading allows you to redefine the behavior of operators for user-defined types. It is powerful for classes, especially when you want to customize how boolean operations behave for class instances.
Overloading the Logical Operators
You can overload logical operators like `&&` (AND) and `||` (OR) for custom classes. Below is a simple example illustrating this:
class BoolOperator {
public:
bool value;
BoolOperator(bool val) : value(val) {}
BoolOperator operator&&(const BoolOperator& other) {
return BoolOperator(this->value && other.value);
}
BoolOperator operator||(const BoolOperator& other) {
return BoolOperator(this->value || other.value);
}
};
In this example, you can create instances of `BoolOperator` and use logical operators seamlessly with your customized logic.
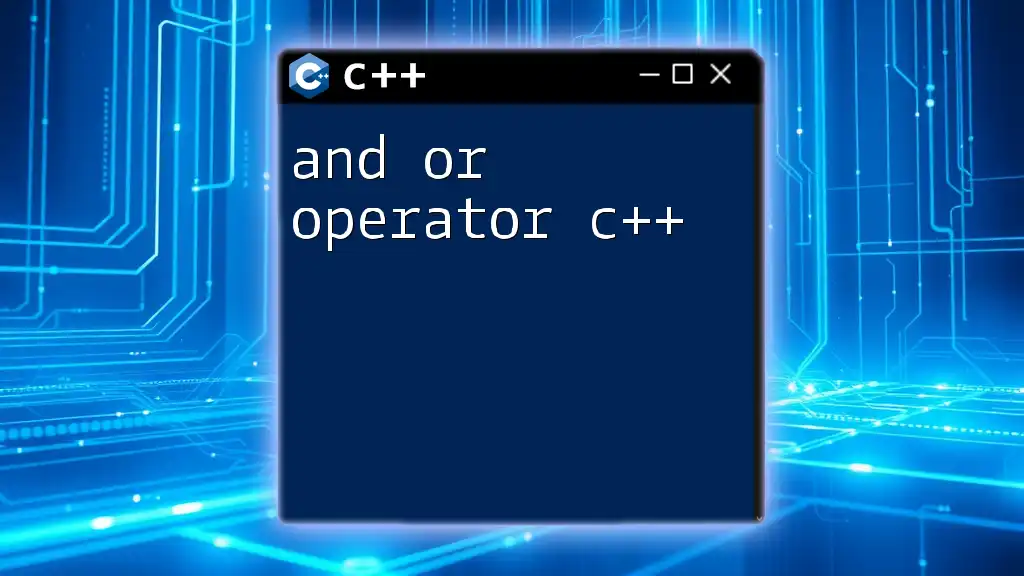
Best Practices for Using bool Operators
Tips for Effective Boolean Logic
Using boolean logic requires clarity and simplicity. Avoid writing complex conditions that can confuse both the compiler and future developers. Clear and concise boolean expressions improve code maintainability.
For example, avoid deep nesting of conditions:
// Less clear
if ((a > b) && (c < d) && (e == f)) { ... }
// More clear
bool isCondition1 = (a > b);
bool isCondition2 = (c < d);
bool isCondition3 = (e == f);
if (isCondition1 && isCondition2 && isCondition3) { ... }
Error Handling in Boolean Operations
When working with boolean operations, errors may arise due to misplaced parentheses or logical errors. Always ensure that conditions make logical sense and are achieved without unnecessary complications. Take the time to test boolean expressions thoroughly to avoid edge cases that might lead to incorrect program behavior.
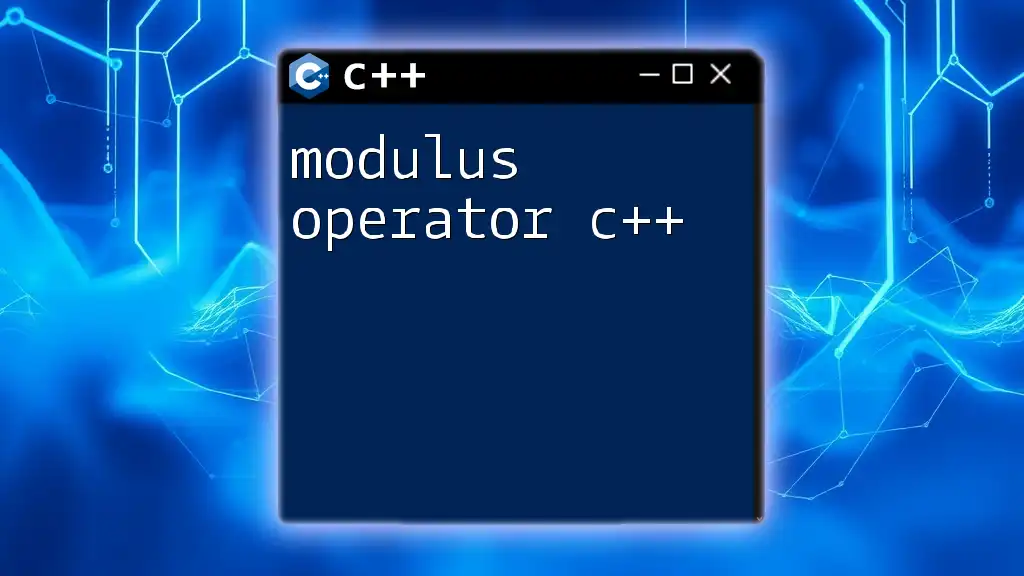
Conclusion
In summary, understanding the bool operator in C++ is crucial for anyone looking to master conditional logic in programming. It allows for clear decision-making processes and efficient control flow within your programs. Armed with these techniques, you can confidently use boolean logic to create robust and effective C++ applications.
Further Resources
For thorough learning, resources such as recommended programming books and online courses are extremely helpful. Referencing the official C++ documentation can also provide valuable insights into the intricate workings of the boolean operators in the language. Happy coding!