The modulus operator in C++ (`%`) returns the remainder of a division between two integers.
Here's a code snippet demonstrating its usage:
#include <iostream>
int main() {
int a = 10;
int b = 3;
int result = a % b; // result will be 1
std::cout << "The modulus of " << a << " and " << b << " is " << result << std::endl;
return 0;
}
What is the Modulus Operator?
The modulus operator in C++ is represented by the percent sign `%`. Its primary purpose is to calculate the remainder of a division operation. For example, when you divide 10 by 3, the quotient is 3 and the remainder is 1. Thus,
10 % 3 // results in 1
This operation is crucial in various programming tasks, particularly in dealing with integers and implementing algorithms that require cyclic calculations.
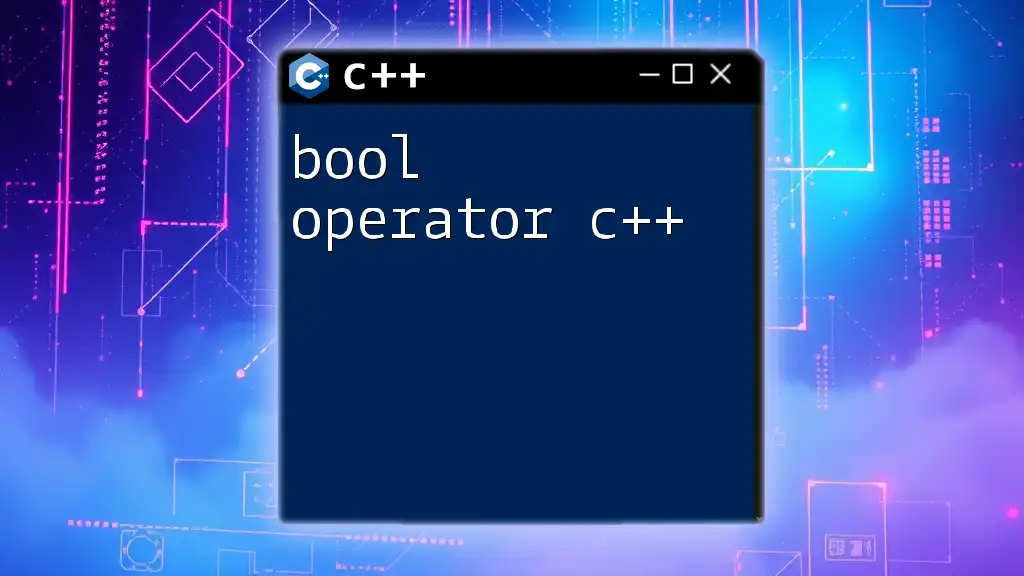
Why Learn the C++ Modulus Operator?
Understanding the modulus operator is vital for several reasons:
- Common Use Cases: The modulus operator is widely used to determine if a number is even or odd, restrict values within a certain range (like circular arrays), and in hashing algorithms.
- Importance in Algorithms and Mathematics: Many mathematical concepts and algorithms revolve around the ability to compute remainders efficiently. Having a firm grasp on the modulus operator will enhance your overall programming skills.
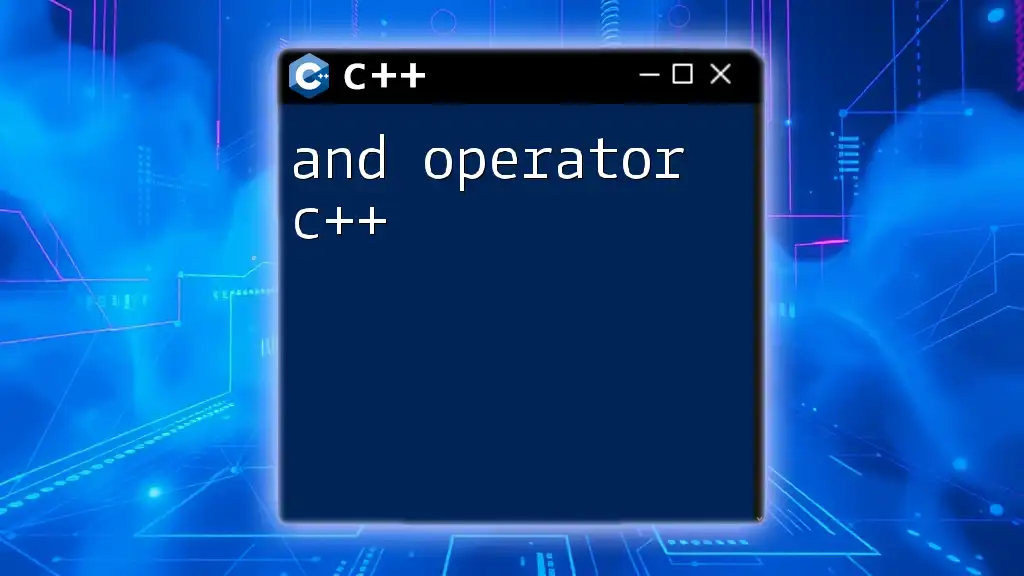
What is Modulus in C++?
In the context of C++, modulus refers to operations involving the `%` operator for numbers. It is essential to recognize that the behavior of the modulus operator can differ from other programming languages, particularly regarding negative operands.
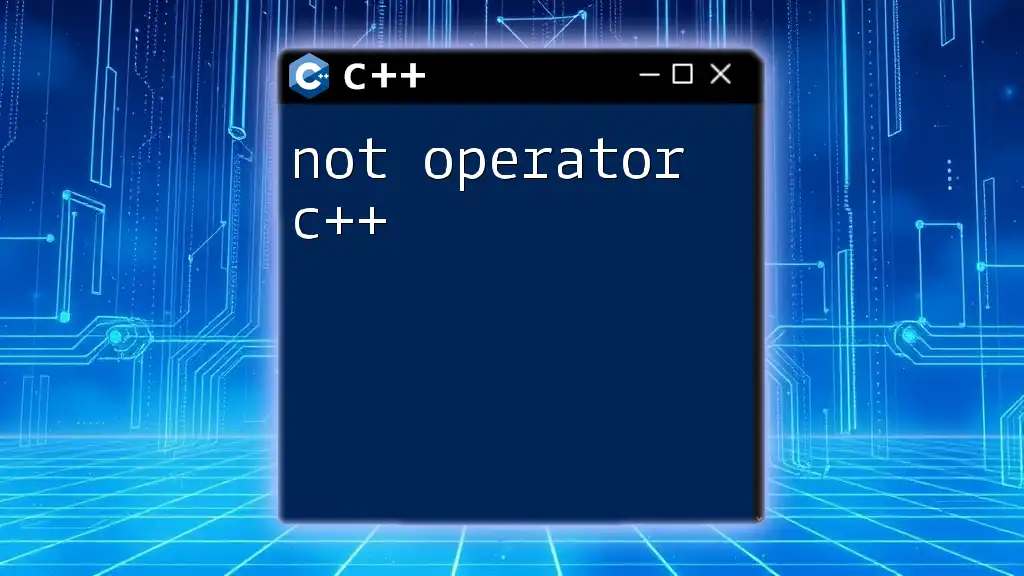
Modulo Operator in C++
The syntax for the modulo operator in C++ is straightforward:
result = a % b;
Here, `a` and `b` are two integer values. If `b` is not zero, the expression will yield the remainder of the division of `a` by `b`. Understanding this syntax is crucial for utilizing the modulus operator effectively.
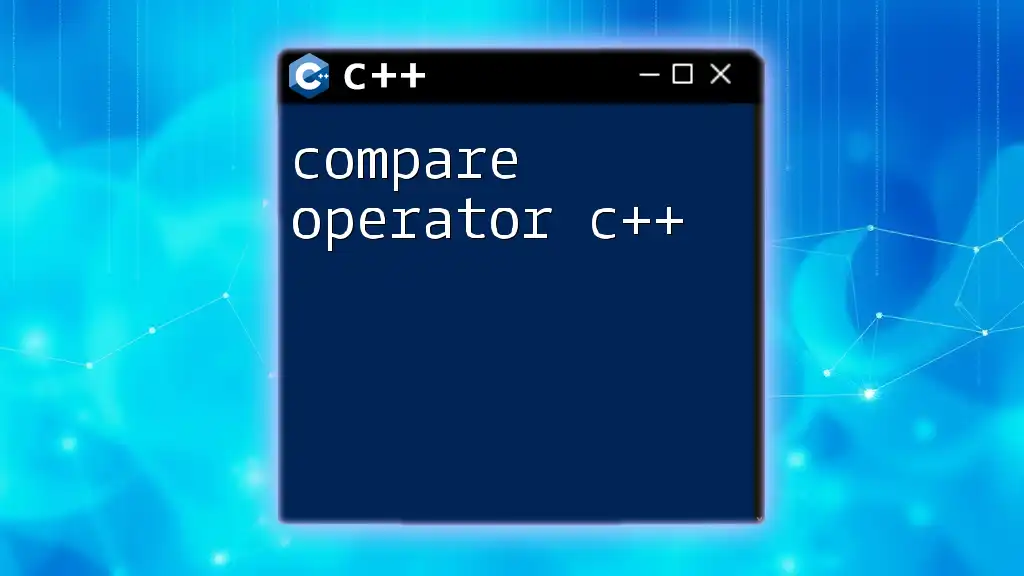
How to Use the Modulus Operator in C++
Basic Syntax of the C++ Modulo Operator
To execute a modulus operation, simply use `a % b`. The result will always be non-negative when `a` is non-negative. Be mindful that if `b` is 0, it will lead to a runtime error since division by zero is undefined.
Examples of Using the Mod Operator in C++
Example 1: Simple Modulus Calculation
Here’s a basic demonstration of the modulus operator:
int a = 10;
int b = 3;
int result = a % b; // result will be 1
Explanation: In this example, `10` divided by `3` gives us a quotient of `3` and a remainder of `1`, hence `result` is `1`.
Example 2: Evaluating Even and Odd Numbers
One practical application of the modulus operator is to check the parity of a number:
int number = 5;
if (number % 2 == 0) {
cout << "Even";
} else {
cout << "Odd"; // Output: Odd
}
Explanation: The condition checks if the number divided by `2` yields a remainder of `0`. If yes, it’s even; otherwise, it’s odd.
Advanced Applications of the Modulus in C++
Circular Arrays
In certain data structures like circular arrays, the modulus operator can help in wrapping around the indices:
const int size = 5;
int circularArray[size] = {1, 2, 3, 4, 5};
// Simulating circular index
for (int i = 0; i < 10; ++i) {
cout << circularArray[i % size] << " "; // Outputs: 1 2 3 4 5 1 2 3 4 5
}
Explanation: In this loop, using `i % size` allows the index to wrap around the array length, creating a continuous loop through the array.
Hashing Algorithms
The modulus operator is frequently used in implementing hash functions:
int hashFunction(int key) {
const int tableSize = 10;
return key % tableSize;
}
Explanation: This function takes a key and computes its hash by returning its equivalent index within a predefined hash table size.
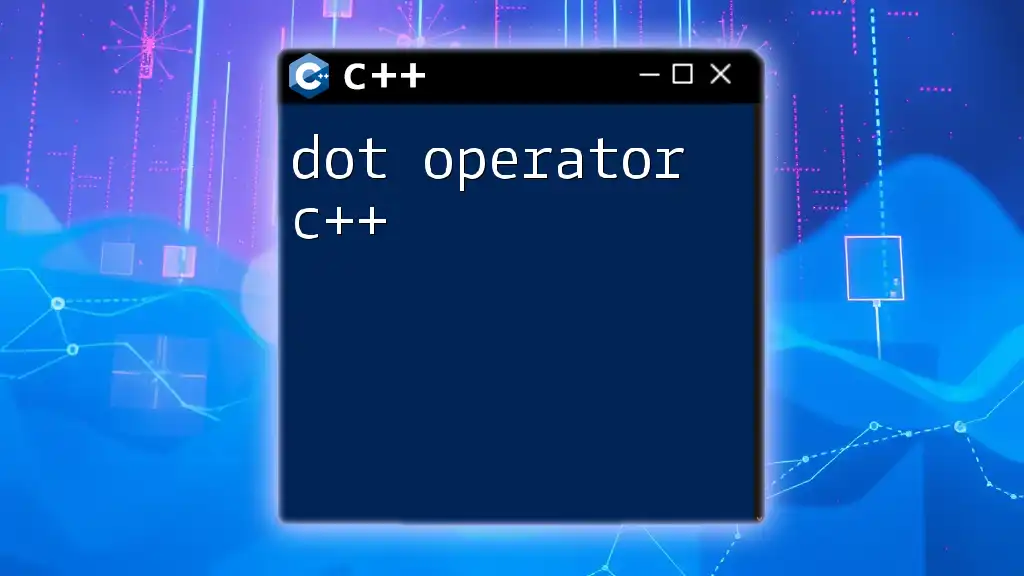
Common Mistakes Using the Modulus Operator in C++
Division by Zero
One of the essential points to remember is that attempting to calculate a modulus with a divisor of `0` leads to runtime errors. Always ensure that the divisor is non-zero before performing the operation.
Negative Numbers
Another common pitfall is the unexpected behavior when using negative numbers. The result of the modulus operator can yield a negative result in some languages, but in C++, it follows the sign of the numerator:
int a = -10;
int b = 3;
int result = a % b; // result will be -1
Here, although `-10 % 3` is mathematically `-1`, it can lead to confusion if not properly understood.
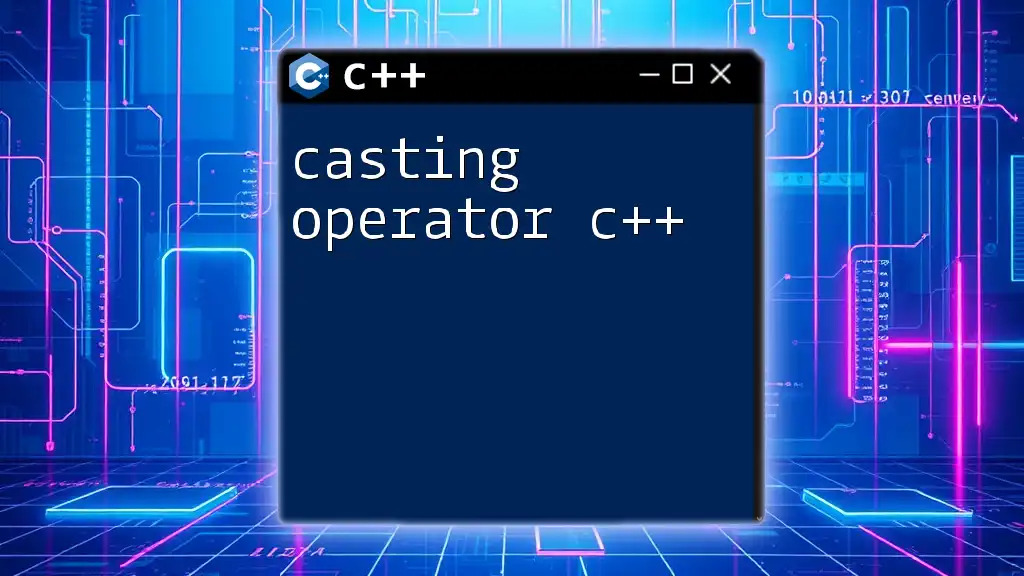
Debugging Tips for Using the Mod Operator in C++
When working with the modulus operator, consider these troubleshooting tactics:
- Check for Division by Zero: Always validate that your denominator is not zero before performing the modulus operation.
- Logical Errors in Conditions: When using the modulus in conditional statements (like checking even or odd), ensure your logic reflects your intent clearly.
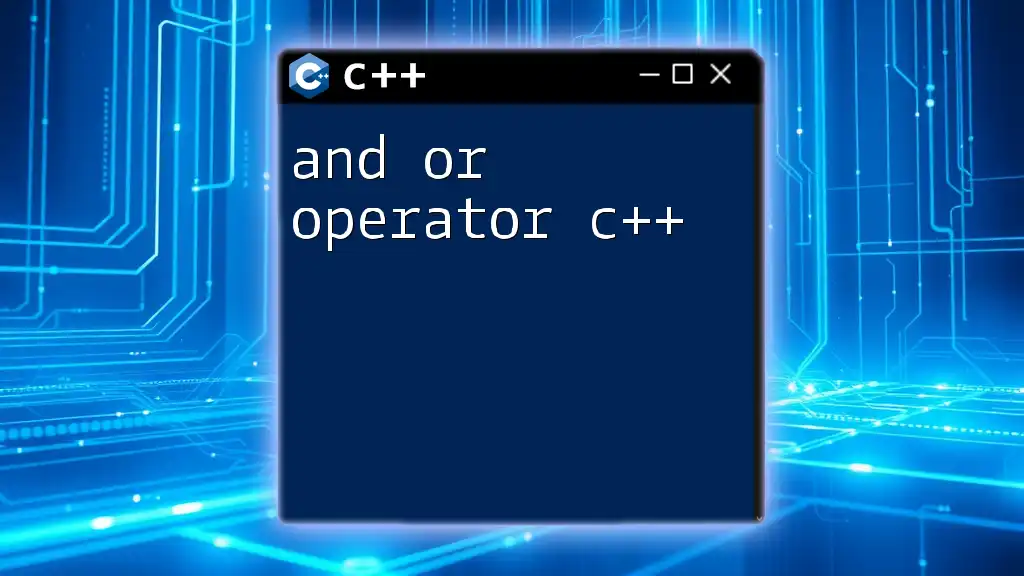
Recap of Key Points
The modulus operator in C++ is an indispensable tool for calculating remainders in integer division, helping implement various algorithms and mathematical operations. Its simplicity and versatility make it essential for both beginners and experienced programmers alike.
Encouragement to Practice: Try creating your own examples using the modulus operator. Practice will enhance your understanding and proficiency with this important programming tool.
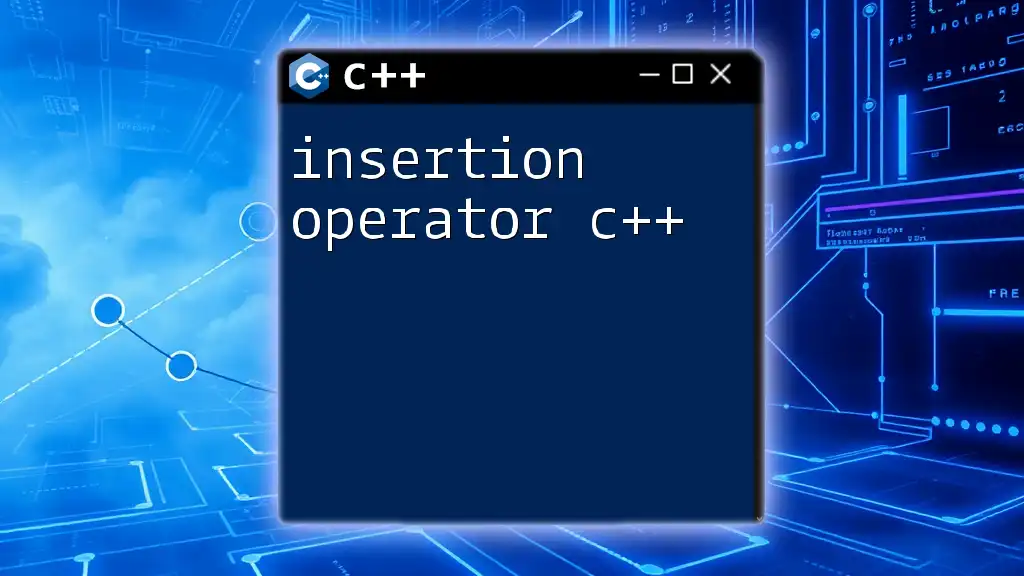
Additional Resources
Further reading on the modulus operator in C++ can help deepen your understanding. Consider checking documentation, online tutorials, or programming books that cover advanced topics in C++.
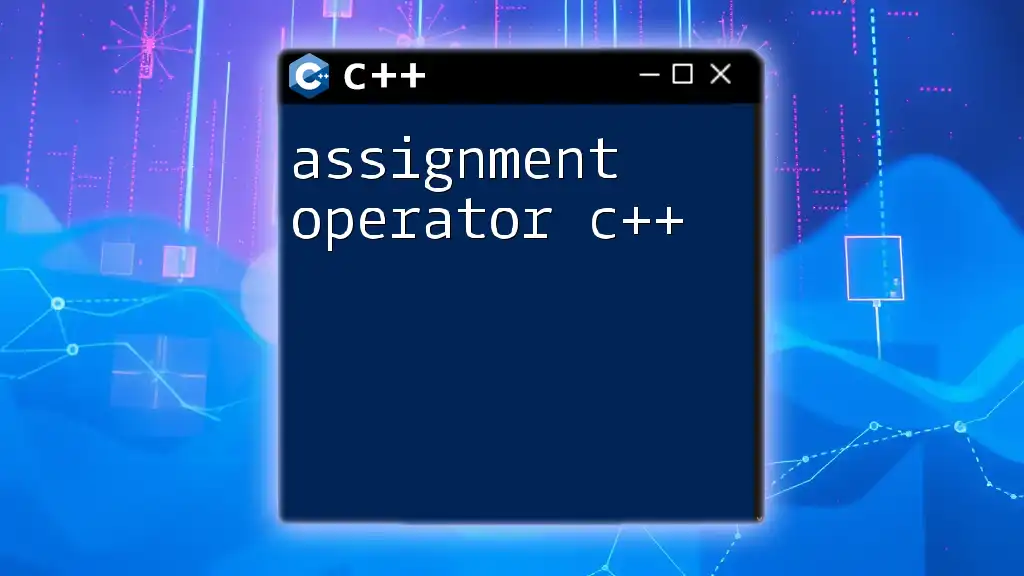
FAQs about Modulus in C++
What is the modulo function in C++?
The modulo function allows you to determine the remainder when one integer is divided by another, forming the basis of many mathematical computations and programming logic.
Can I use modulus with floating-point numbers?
While the modulus operator is primarily designed for integers, C++ does include alternative ways to work with floating-point numbers using functions from libraries such as `<cmath>`.
How does the C++ modulus operator handle negatives?
In C++, the result of the modulus operator takes the sign of the first operand. Thus, negative results may occur if the first operand is negative. Understanding this behavior is crucial for accurate mathematical operations.