The question mark operator, also known as the ternary operator, in C++ is a concise way to evaluate a condition and return one of two values based on the result.
Here’s a simple example:
#include <iostream>
int main() {
int a = 10, b = 20;
int max = (a > b) ? a : b; // returns a if true, otherwise returns b
std::cout << "The maximum value is: " << max << std::endl;
return 0;
}
Understanding the C++ Question Mark Operator
What is the Ternary Operator?
The question mark operator in C++ is commonly known as the ternary operator, because it takes three operands. It serves as a compact alternative to traditional if-else statements. This operator facilitates conditional logic in a concise manner and utilizes the following syntax:
condition ? expression1 : expression2
In this construct, if the `condition` evaluates to `true`, `expression1` is executed; otherwise, `expression2` is executed. This brings a notable reduction in code length and often enhances readability when used appropriately.
Why Use the Ternary Operator?
The primary reasons to use the question mark operator in C++ include:
- Breavity: The operator allows you to make decisions in a single line, reducing the need for multiple lines of code typically associated with if-else constructs.
- Clarity in Simple Cases: For straightforward conditions, using the ternary operator can clarify the intent without cluttering the code.
However, it’s essential to use this operator judiciously; too much complexity can lead to confusion. In many cases, if the decision-making logic involves several conditions, a traditional if-else statement might be preferable for clarity.
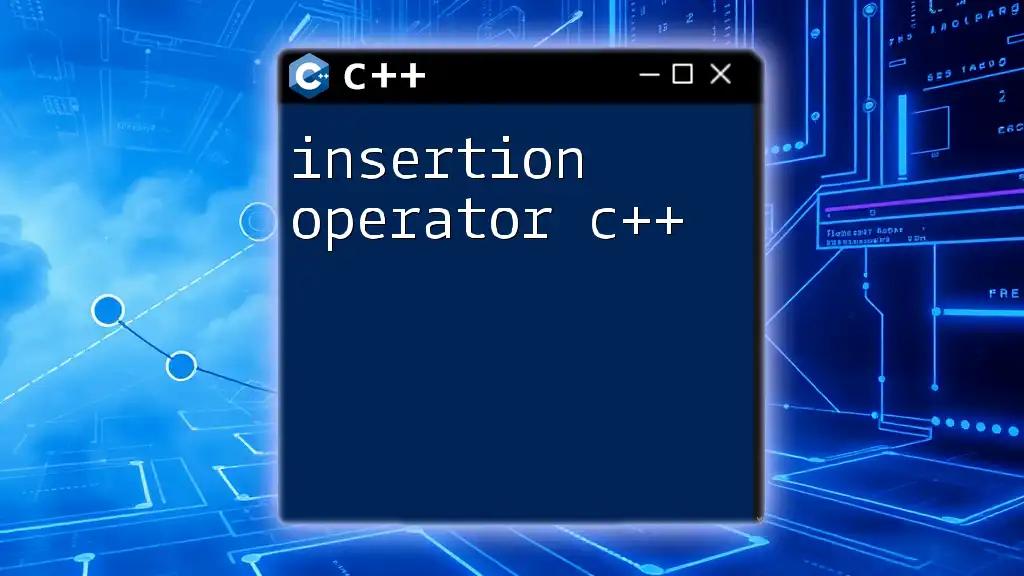
How to Use the Question Mark Operator in C++
Basic Syntax and Examples
Understanding the syntax is the first step to effectively utilizing the question mark operator. Consider this example:
int a = 10, b = 20;
int max = (a > b) ? a : b;
In this code snippet, `max` will be assigned the value of `b` because `a` is not greater than `b`. By unpacking this logic:
- Condition: `a > b` evaluates to `false`.
- Result: Hence, `b` is assigned to `max`.
Nested Ternary Operators
A powerful feature of the question mark operator is its ability to nest conditions. However, nesting should be used thoughtfully. Here’s an example of nested ternary operators:
int score = 85;
char grade = (score >= 90) ? 'A' :
(score >= 80) ? 'B' :
(score >= 70) ? 'C' : 'D';
In this example, `grade` is determined based on the value of `score` through a series of conditions evaluated in order. The breakdown of this nesting looks like this:
- If `score` is 90 or above, `grade` is 'A'.
- If `score` is between 80 and 89, `grade` is 'B'.
- If `score` is between 70 and 79, `grade` is 'C'.
- For any score below 70, `grade` becomes 'D'.
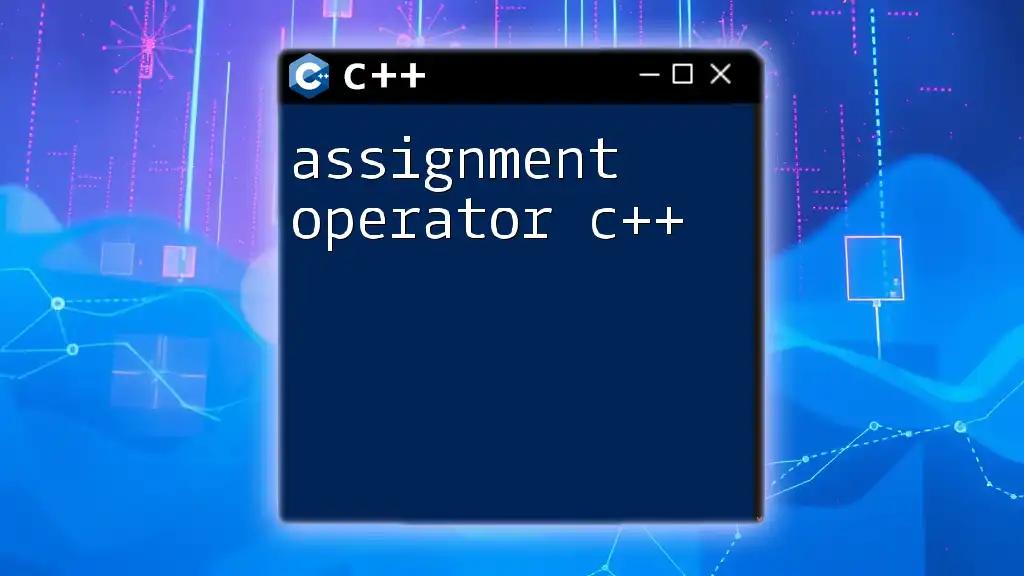
Common Use Cases for the C++ Question Mark Operator
Conditional Assignment
The question mark operator is handy for making quick, conditional assignments. Here’s a typical example:
bool isLoggedIn = true;
string message = isLoggedIn ? "Welcome Back!" : "Please Log In";
In this scenario, `message` will either greet the user appropriately or prompt them to log in, depending on the value of `isLoggedIn`.
Simplifying Return Statements
Another practical application of the question mark operator is in simplifying the return values in functions. Consider the following:
string getStatus(bool isActive) {
return isActive ? "Active" : "Inactive";
}
This concise function directly returns a string based on the boolean `isActive`, showcasing how the question mark operator can enhance function readability.

Best Practices for Using the Question Mark Operator
Readability Matters
While the question mark operator can tighten up your code, readability should always be a priority. When writing more complex conditional logic, if it begins to obscure the intent of your code, it is better to opt for traditional if-else constructs.
Comparison to If-Else Statements
The decision to use the ternary operator rather than an if-else statement can often come down to context. If the logic is simple, the question mark operator provides a clean solution. But if multiple conditions or actions are involved, employing standard if-else statements not only maintains readability but makes debugging easier.
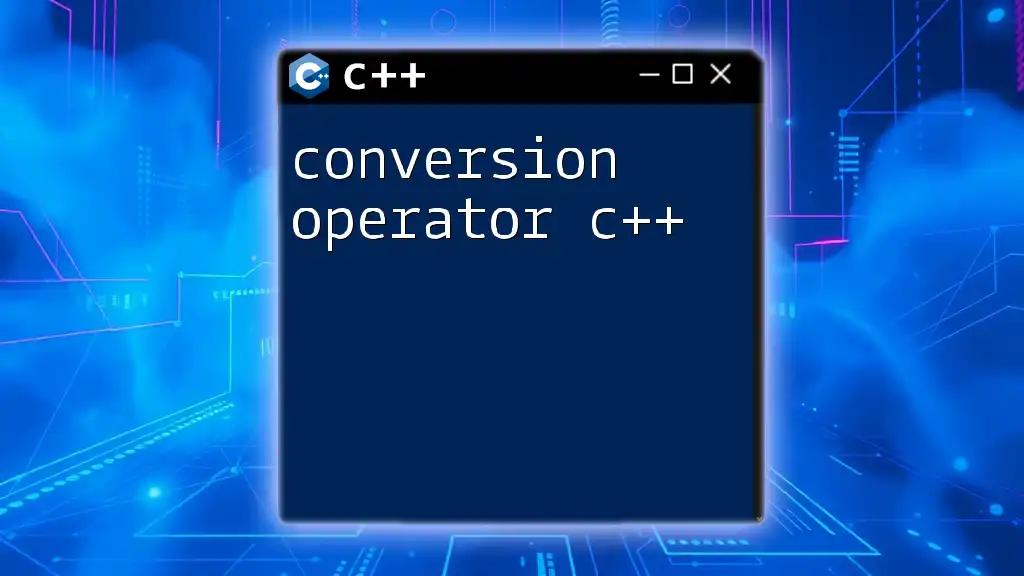
Conclusion
Mastering the question mark operator in C++ offers programmers a powerful tool for creating compact, efficient, and readable conditional statements. However, it is essential to balance its use against the potential for complexity. Embrace this operator for simple conditions and explore how it can improve both your code's clarity and performance.
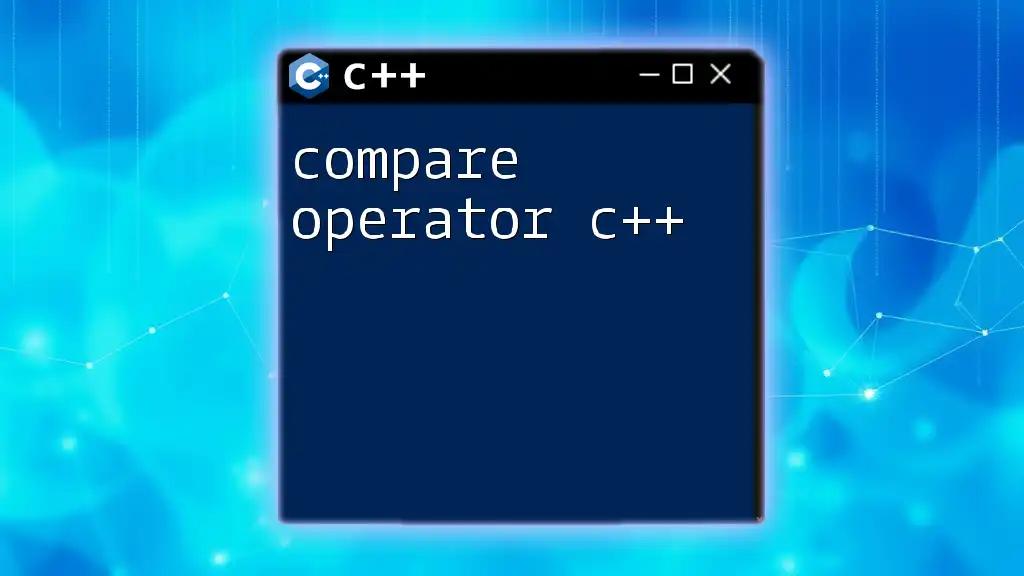
Additional Resources
For those looking to deepen their understanding of the question mark operator and C++ in general, consider utilizing online compilers for real-time coding practice, and delve into recommended books and articles that focus on C++ programming techniques and best practices.
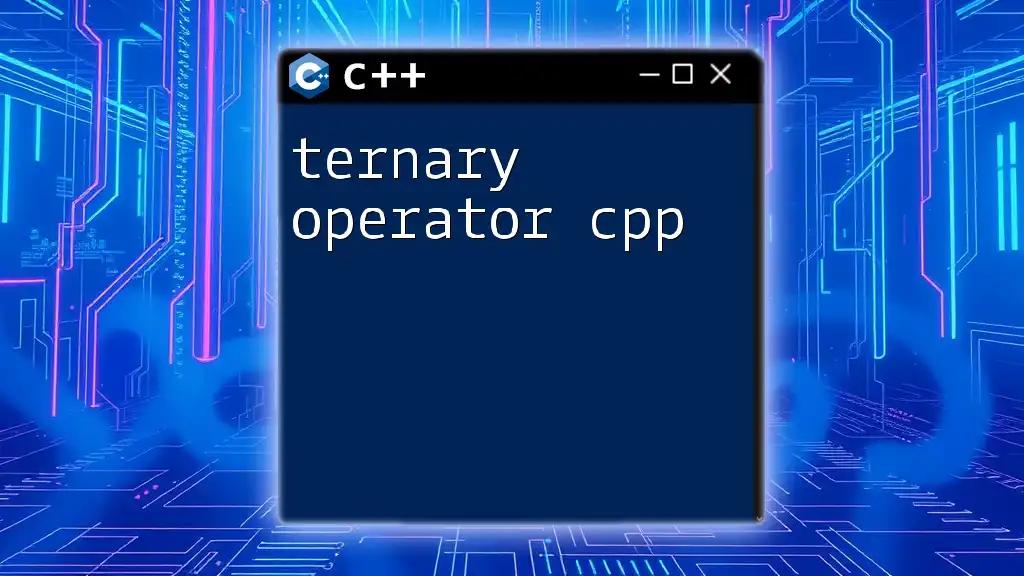
FAQs About the C++ Question Mark Operator
What happens if the condition is false?
When the condition evaluates to `false`, the question mark operator skips the first expression and executes the second expression instead. Thus, only one of the expressions is evaluated based on the outcome of the condition.
Can you use the question mark operator for types other than ints?
Absolutely! The question mark operator can be used with any data type. It's versatile and can handle various types from integers to strings, as long as the expressions yield compatible types.
What are alternative operators in C++?
C++ features a range of operators, including logical operators (like && and ||), bitwise operators (like & and |), and arithmetic operators (like +, -, *, and /). Understanding these can enhance your programming proficiency beyond just conditional statements.