The assignment operator in C++ (`=`) is used to assign the value of the right-hand operand to the left-hand operand. Here’s a simple example:
int a = 5; // Assigns the value 5 to the variable a
What is the Assignment Operator?
The assignment operator in C++ is a fundamental operator that allows you to assign values to variables. Its most common form is the equal sign (`=`), which is not only used for assigning values but also acts as a means to equate values in expressions. Understanding the assignment operator is crucial for effective programming in C++, as it is used extensively throughout your code.

How the Assignment Operator Works
The Basic Functionality
The primary function of the assignment operator is to transfer a value from one variable to another. This can be seen as a copying action where the destination variable receives the value from the source variable.
For example:
int a = 5;
int b;
b = a; // b now holds the value of a, which is 5
Here, `b` takes the value of `a`, demonstrating a simple variable assignment.
Assignment Operator Mechanics
Internally, the assignment operator involves copying the value from the right side of the operator to the left side. It's crucial to understand that value assignment means copying the actual value, whereas reference assignment references the same memory location.
When you assign one object to another, you need to consider whether you're using a data type that allows copying (like simple data types) or one that may require a more complex behavior (such as object types).
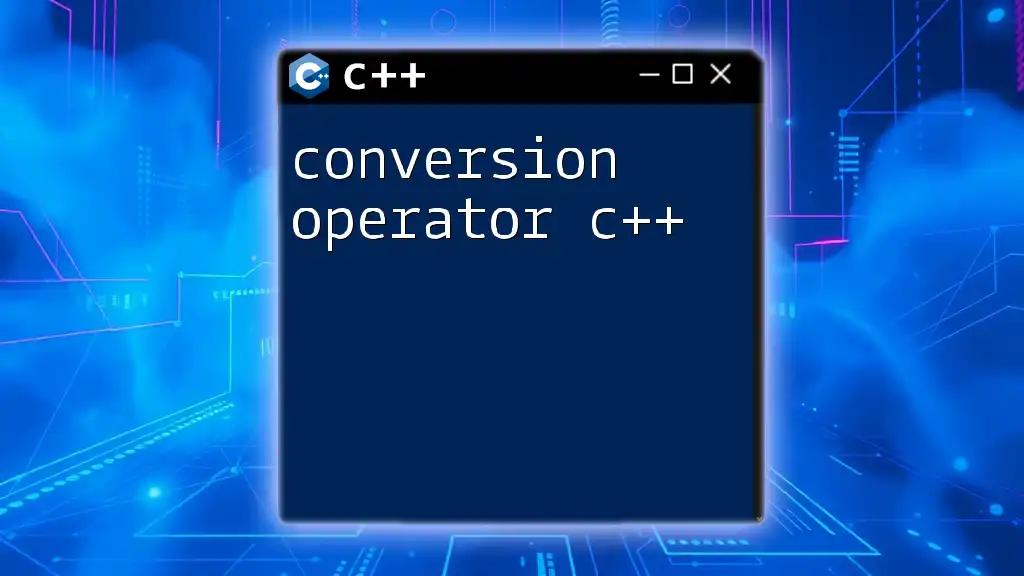
Assigning Different Data Types
Integer Assignment
Assigning values between integer variables is straightforward. The assignment operator duplicates the integer value from one variable to another.
int x = 10;
int y = x; // y is now 10, an integer assignment.
Float and Double Assignment
In C++, you can also assign floating-point values. Careful attention must be paid to data types to avoid precision issues.
float f = 10.5f;
double d = f; // d is now 10.5, demonstrating a float to double assignment.
String Assignment
Assigning strings involves copying the value of one string object into another. C++ provides a convenient way to handle string assignments through its `std::string` class.
std::string str1 = "Hello";
std::string str2 = str1; // str2 is "Hello", showing string assignment.

The Role of the Assignment Operator with Classes
Adding Assignment Operator Overloading
In C++, you can overload the assignment operator to customize its behavior for your own classes. This is particularly useful for managing resources or implementing special copy mechanics.
For instance, this example illustrates how to define a class with an overloaded assignment operator:
class MyClass {
private:
int value;
public:
MyClass(int val) : value(val) {}
MyClass& operator=(const MyClass& other) {
if (this != &other) {
value = other.value; // Perform the assignment if not self-assigning
}
return *this; // Return the current instance
}
};
In this code, `MyClass` has an overloaded `operator=` that carefully checks for self-assignment before copying the data.
Importance of Copy Assignment
Copy assignment helps manage how resources are moved or shared among objects. Especially when dealing with dynamic memory, handling copy semantics appropriately can prevent memory leaks and ensure that your program functions accurately and efficiently.
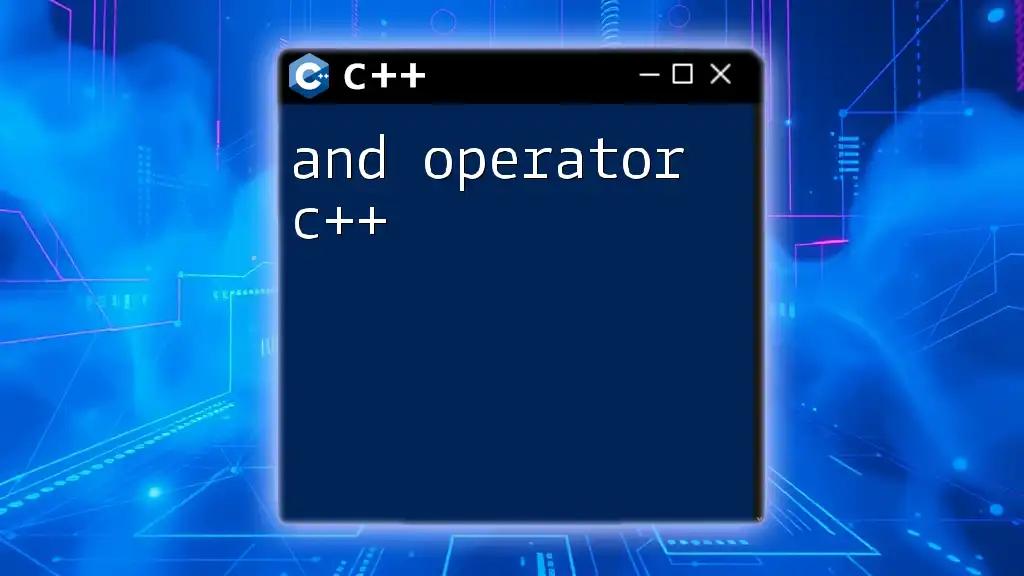
Common Mistakes When Using the Assignment Operator
Trying to Assign to Constants
One common mistake is attempting to assign a value to a constant variable. Constants cannot be modified after initialization, leading to compilation errors.
const int a = 10;
a = 20; // Error: assignment of read-only location
This error highlights the immutable nature of constant variables in C++.
Self Assignment Pitfalls
Another pitfall is failing to check for self-assignment, which can lead to unintended behaviors. It's essential to guard against this in your overloaded operators.
When you perform an assignment like:
MyClass obj1(5);
obj1 = obj1; // Potential issue if not controlled
If `operator=` is not implemented correctly, you might end up in an undefined state within the object.
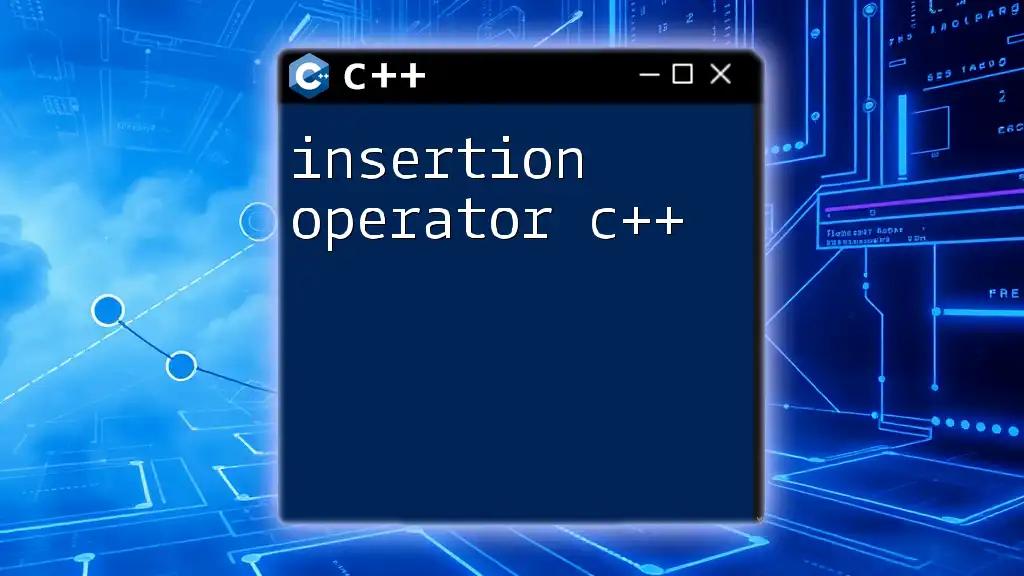
Advanced Topics
Chained Assignments
C++ allows for chained assignments, which can be a powerful feature:
int a, b, c;
a = b = c = 10; // all variables get the value of 10
In this case, the assignment is processed from right to left, with each variable receiving the value of 10 in one concise statement.
Best Practices for Assignment Operators
When implementing assignment operators, always consider the following best practices:
- Ensure you handle self-assignment elegantly.
- Return a reference to the current object (`*this`) for chained assignments to work seamlessly.
- Properly manage resources, particularly if your class manages dynamic memory.
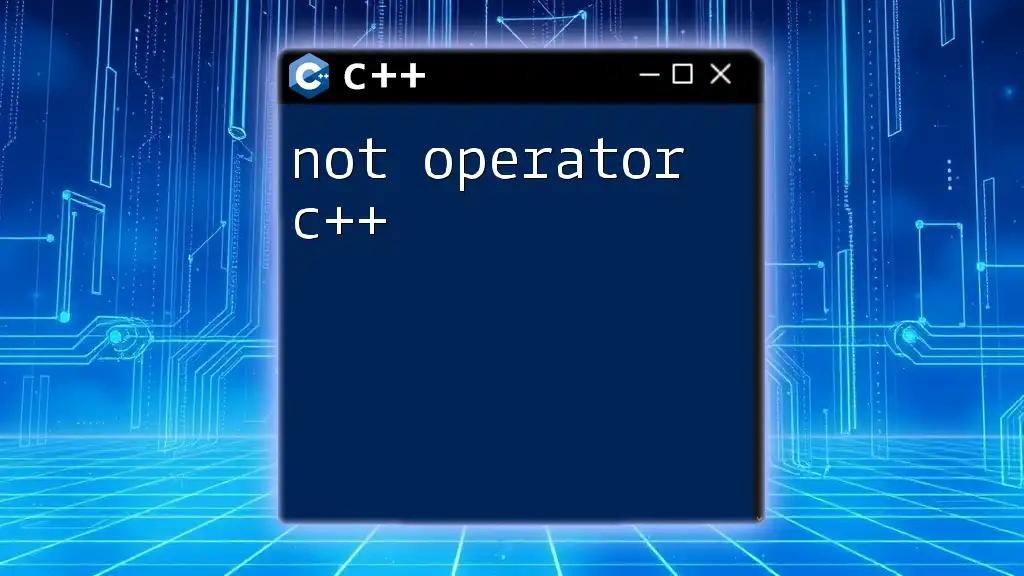
Conclusion
The assignment operator in C++ is a foundational element that plays a critical role in value assignment. Mastering the use of the assignment operator, including how it operates with different data types, classes, and potential pitfalls, is essential for efficient programming. By utilizing best practices and comprehending the nuances of assignment semantics, you can build robust and efficient C++ applications.
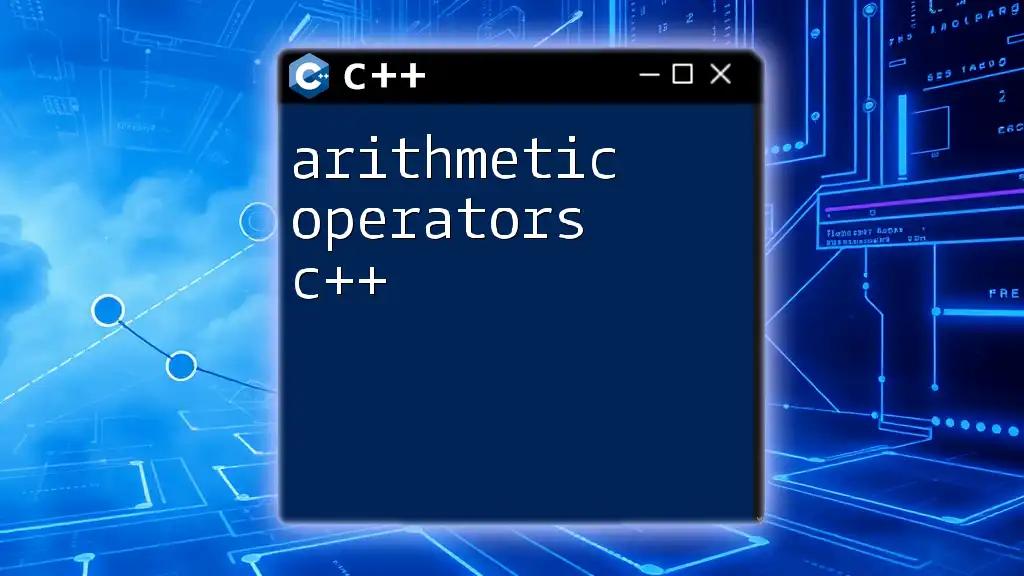
Further Resources
To deepen your understanding of C++ operators, including the assignment operator, consider exploring additional reading materials, documentation, and practical coding challenges that reinforce these concepts. An iterative approach to learning—combining theory with practical application—will solidify your mastery of the assignment operator and enhance your coding prowess.