The C++ move assignment operator allows a class to transfer resources from one object to another efficiently, avoiding unnecessary copying, typically implemented when the left-hand object already has allocated resources.
#include <utility> // for std::move
class MyClass {
public:
MyClass(MyClass&& other) noexcept : data(other.data) {
other.data = nullptr; // Leave other in a valid state
}
MyClass& operator=(MyClass&& other) noexcept {
if (this != &other) {
delete data; // Clean up current resource
data = other.data; // Transfer ownership
other.data = nullptr; // Leave other in a valid state
}
return *this;
}
private:
int* data = nullptr; // Example resource
};
What is the Move Assignment Operator?
The C++ move assignment operator is a special member function that allows the assignment of resources from one object to another, transferring ownership of the resources without the overhead of copying. In other words, it provides a mechanism for "moving" instead of copying resource values, which can significantly improve performance, especially when dealing with large objects or dynamically allocated memory.
The main difference between the move assignment operator and the copy assignment operator lies in their behavior:
- The copy assignment operator creates a new copy of the resource, whereas the move assignment operator relinquishes ownership from an existing object without copying, making it more efficient.
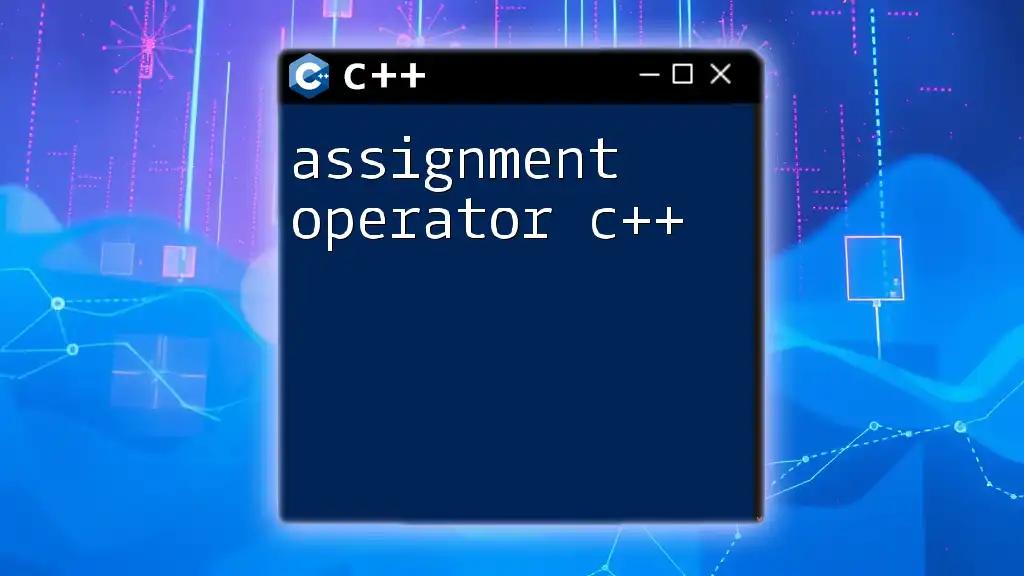
Syntax of the Move Assignment Operator
The syntax for defining a move assignment operator is straightforward. Here's what it typically looks like:
class MyClass {
public:
MyClass& operator=(MyClass&& other) noexcept;
};
The first key component is the use of `MyClass&&` as a parameter, indicating that this function accepts an rvalue reference, which allows for resource transfer. The `noexcept` specifier is used to indicate that this function does not throw exceptions, which is a critical aspect of performance in certain contexts.
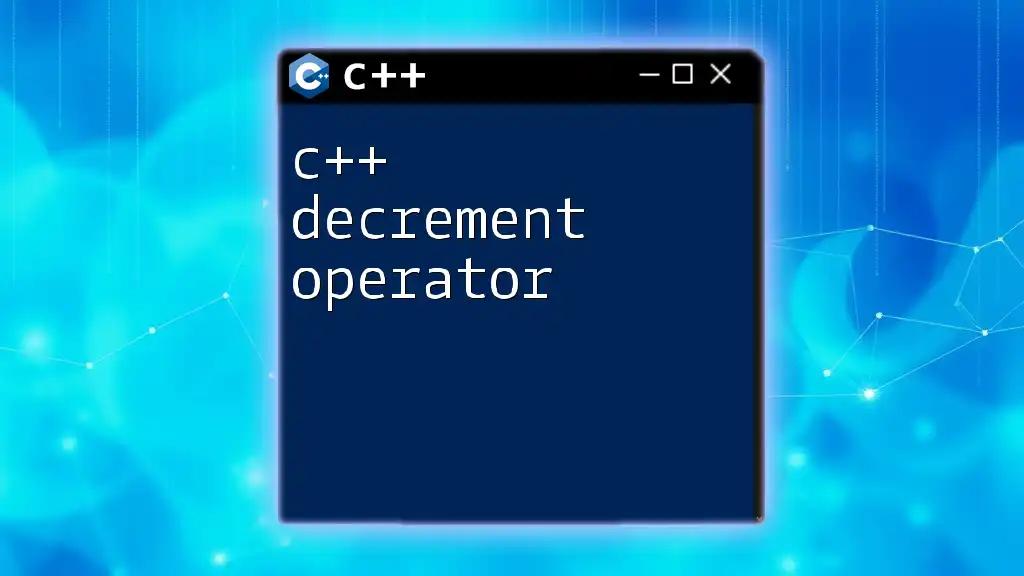
Implementing the Move Assignment Operator
Step 1: Checking Self-assignment
One of the essential first steps in implementing a move assignment operator is to check for self-assignment. This means verifying that the current object is not the same as the object being assigned to it. If self-assignment is not handled, it can lead to resource leaks or undefined behavior. Here's how you do it:
if (this == &other) return *this;
If the current object is the same as `other`, there is no need to proceed further, and you'll simply return `*this`.
Step 2: Releasing Resources
Before transferring resources, it is crucial to release any memory or other resources that the current object holds. Failing to do so can lead to memory leaks. Resource ownership refers to the idea that a class instance owns certain resources (like dynamically allocated memory), and it's the class's responsibility to free that memory when it's no longer needed.
To release resources, you can do something like this in your move assignment operator:
delete[] this->resource;
This line ensures that any previous resources are properly cleaned up.
Step 3: Transferring Resources
The actual transfer of resources is where the power of the move assignment operator comes into play. You essentially "steal" the resources from the `other` object and set the `other` object's resources to `nullptr`. This prevents double deletion issues when both the original and moved-from objects go out of scope:
this->resource = other.resource; // Transfer ownership
other.resource = nullptr; // Nullify the source
By nullifying `other.resource`, you ensure that the moved-from object does not accidentally delete the memory it no longer owns.
Step 4: Return `*this`
After successfully transferring the resources, the final step is to return a reference to `*this`. This allows for method chaining, where multiple operations can be performed on the same object in a single line.
return *this; // Return current object
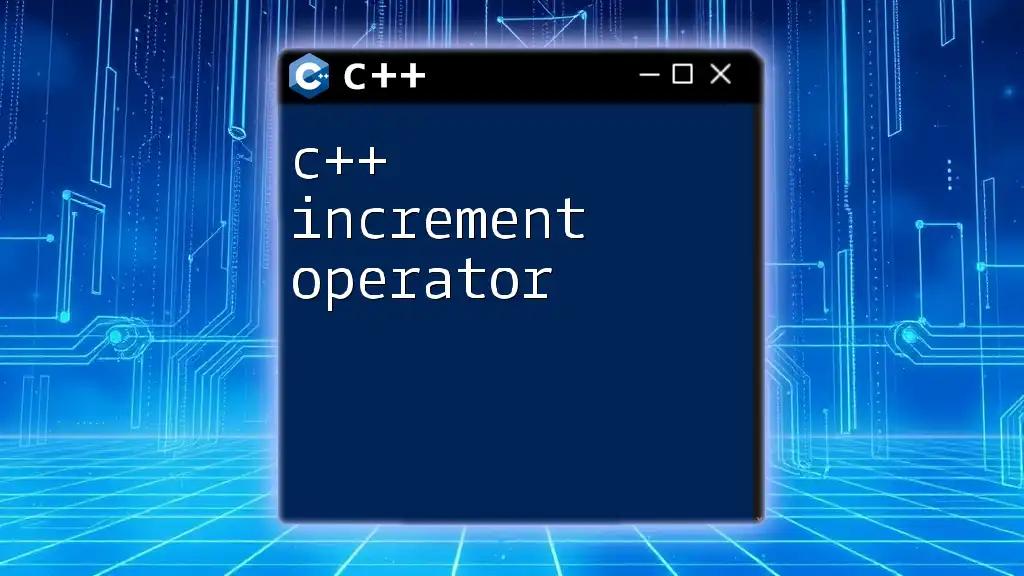
Complete Example of a Move Assignment Operator
Here’s an example of a complete implementation of a class that includes a move assignment operator. The class manages a dynamic array of integers:
class MyClass {
private:
int* resource;
public:
MyClass(int size) : resource(new int[size]) {}
~MyClass() { delete[] resource; }
// Move Assignment Operator
MyClass& operator=(MyClass&& other) noexcept {
if (this == &other) return *this; // Check for self-assignment
delete[] resource; // Release old resources
resource = other.resource; // Transfer ownership
other.resource = nullptr; // Nullify the source
return *this; // Return current object
}
};
This implementation showcases a typical use case of the move assignment operator with key considerations such as self-assignment check, resource management, and correct object state maintenance.
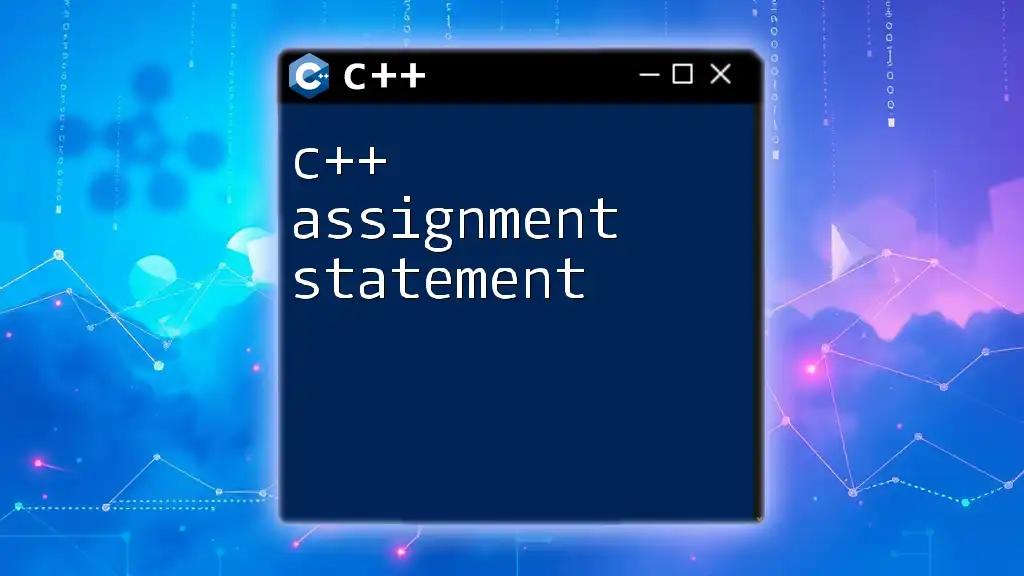
Best Practices for Using Move Assignment
When utilizing the C++ move assignment operator, keep the following best practices in mind:
- Prefer Move Over Copy: Use move semantics whenever you find yourself working with objects that are expensive to copy. This helps maximize performance, particularly with resource-heavy classes.
- Use `noexcept`: Always mark your move assignment operator as `noexcept` unless you explicitly need it to throw. This allows the compiler to make optimizations and is crucial when using standard library containers that rely on move semantics.
- Understand the Rule of Five: With C++11 and beyond, if you define a move assignment operator, you should also consider defining a move constructor, copy constructor, copy assignment operator, and destructor to ensure proper resource management.
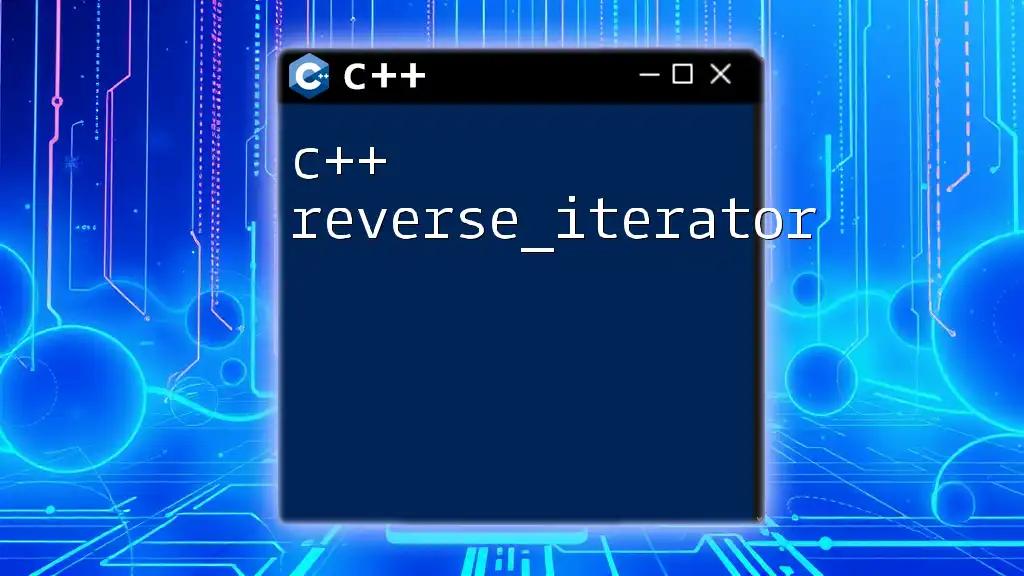
Common Mistakes to Avoid
When implementing the C++ move assignment operator, it’s crucial to avoid common pitfalls:
- Forgetting Self-assignment Check: Neglecting this check may lead to issues when an object is assigned to itself.
- Not Releasing Resources Correctly: Failing to properly release resources could cause memory leaks.
- Using Moved-from Objects: After an object has been moved from, it should only be destructed or assigned to again; using it can lead to undefined behavior.
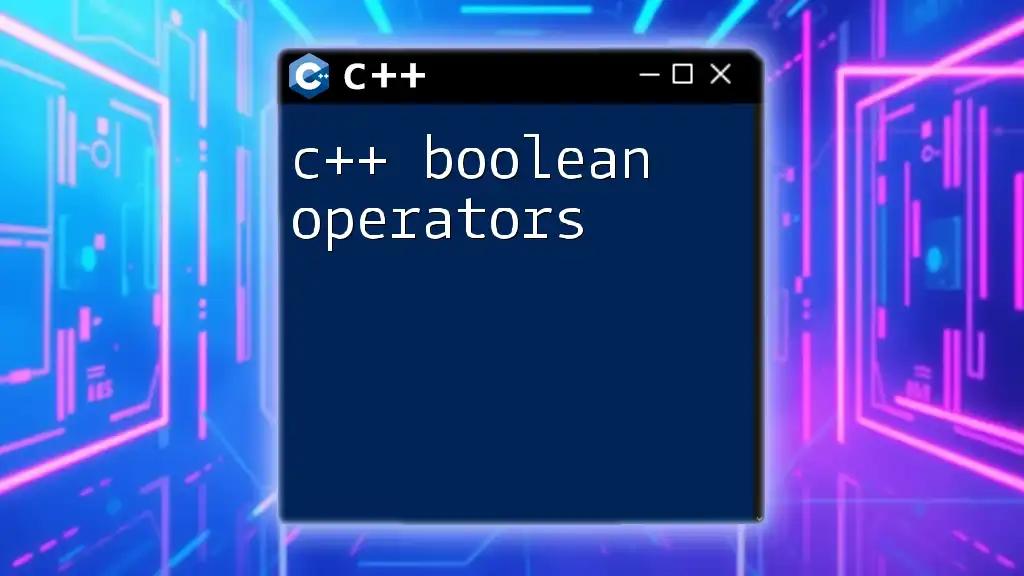
Performance Benefits of Move Assignment Operator
Implementing a move assignment operator can significantly improve performance. When resources are moved rather than copied, the operation becomes much faster, as the underlying data is merely transferred, often represented by a simple pointer operation rather than duplicating memory. This is particularly evident with large objects or containers, where unnecessary copying can become a substantial overhead.

Conclusion
In summary, mastering the C++ move assignment operator is vital for writing efficient and robust C++ code. This operator allows developers to optimize resource management and performance in their applications. By adhering to best practices and understanding the principles behind move semantics, you can improve your C++ programming skills significantly.
Frequently Asked Questions (FAQs)
What happens if I don't implement the move assignment operator?
If a move assignment operator is not implemented, the compiler will generate a default copy assignment operator, which may lead to inefficiencies when handling large or complex objects.
Can I use the move assignment operator with built-in types?
Yes, the move assignment operator can be used with built-in types, but you may not see significant performance gains since built-in types are already lightweight.
What is the difference between the move assignment operator and the copy assignment operator?
The move assignment operator transfers ownership without creating a copy of the resources, while the copy assignment operator creates a new copy, which can be more expensive in terms of performance.
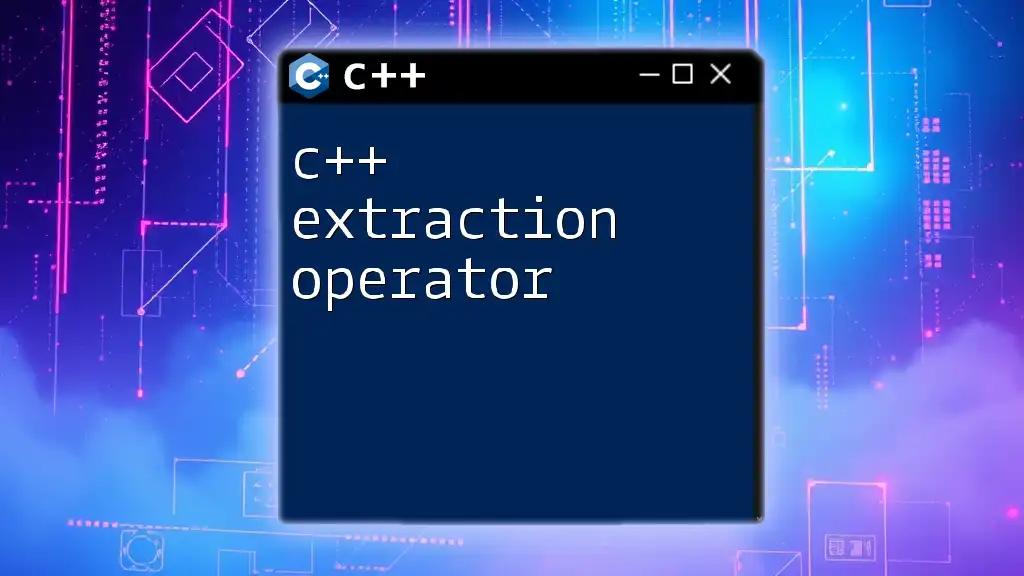
Additional Resources
For further exploration into C++ and advanced topics akin to the move assignment operator, consider referring to official C++ documentation, online tutorials, and comprehensive programming books that delve deep into C++ memory management and resource handling.