In C++, an assignment statement is used to assign a value to a variable, typically using the equals sign (`=`) to store data in the variable's memory location.
int x = 5; // Assigns the value 5 to the variable x
Understanding Assignment Statements
What is an Assignment Statement?
A C++ assignment statement is a fundamental instruction that assigns a value or expression to a variable. The basic syntax for an assignment statement is as follows:
variable = expression;
In this syntax, the expression on the right-hand side is evaluated, and its result is stored in the variable on the left-hand side. This feature enables developers to control the state of their programs effectively.
Purpose of Assignment Statements
Assignment statements are pivotal in C++ programming, serving several purposes. They allow you to:
- Store values in variables, which makes it easier to manipulate and access data.
- Update the state and values of variables during program execution.
- Provide the foundation for building control structures and implementing algorithms that rely on variable values.
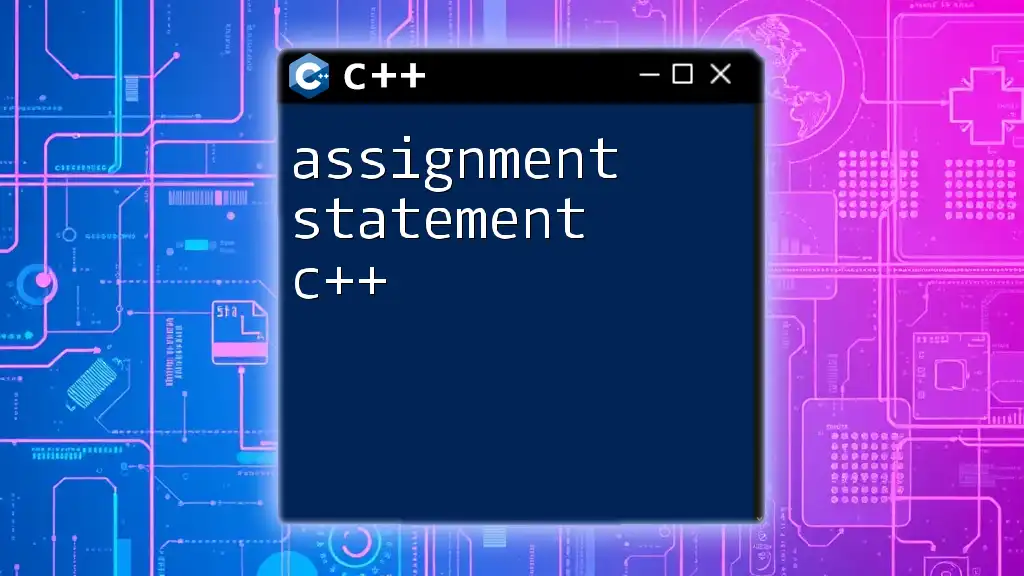
Syntax of Assignment Statements
Basic Syntax
The simplest form of assignment in C++ is the straightforward assignment, where a value is assigned directly to a variable. For instance:
int x = 10; // Assigns 10 to x
In this example, the integer variable `x` holds the value `10`. The syntax is both clear and straightforward, making C++ accessible to beginners.
Components of an Assignment
Understanding the components of an assignment statement is essential:
- Variable: A named storage location that holds data, like `x` in the example above.
- Operator: The assignment operator `=` indicates that the value on the right should be assigned to the variable on the left.
- Expression: This can be a number, variable, or more complex expressions, such as mathematical operations.
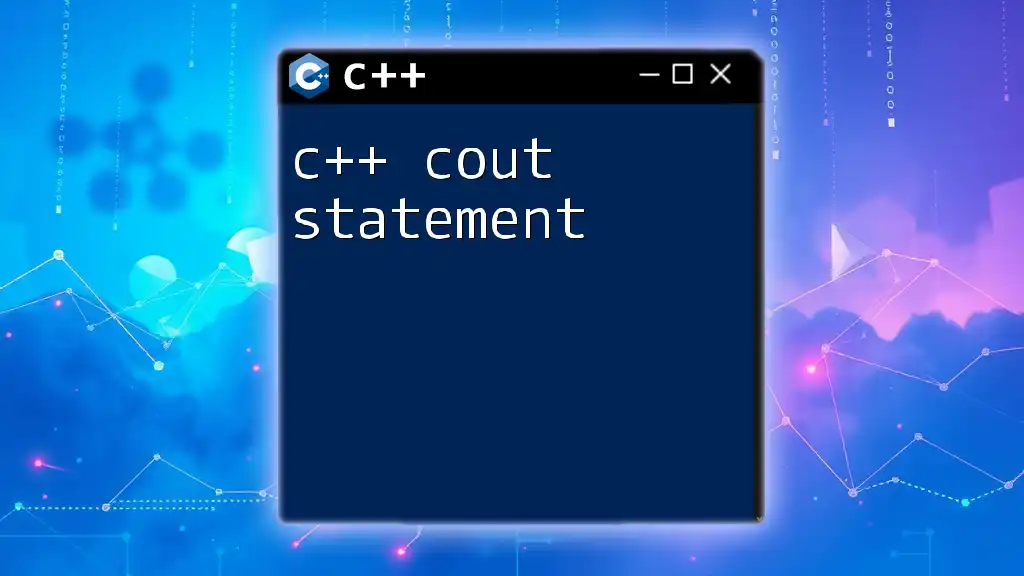
Types of Assignment Statements
Simple Assignment
The simple assignment is the most basic form, where a single value is assigned to a variable:
int a = 5; // Assigns 5 to a
This operation initializes `a` with the value `5`, which can be used later in calculations or functions.
Chained Assignment
Chained assignments allow multiple variables to be assigned the same value in a single statement. For instance:
int x, y, z;
x = y = z = 10; // All variables are assigned the value 10
In this example, all three variables `x`, `y`, and `z` are set to `10`. This technique is useful for initializing multiple variables at once.
Compound Assignment
Compound assignment operators simplify expressions by combining an arithmetic operation with an assignment. Here are some examples:
int num = 10;
num += 5; // Equivalent to num = num + 5
In the snippet above, `num` is increased by `5`, demonstrating a concise way to update a variable's value without repeating its name.
Multi-variable Assignment
In C++, you can assign values to multiple variables using the same syntax. Here's an illustrative example:
int x, y, z;
x = y = z = 1; // Assigns 1 to x, y, and z
This practice is handy when you want to initialize several variables to the same value at once.
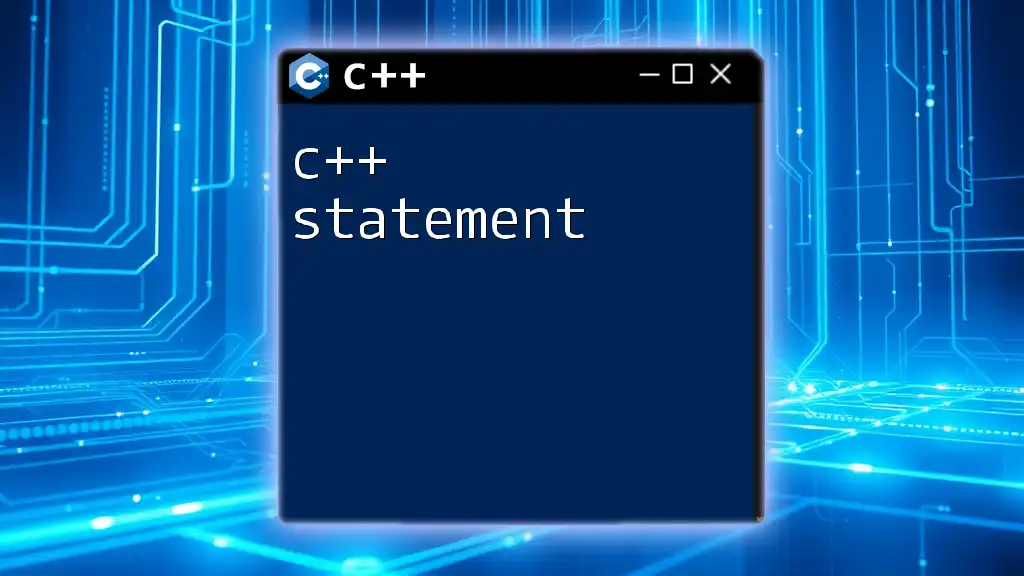
Special Cases in Assignment Statements
Assigning Different Data Types
C++ allows for implicit type conversion during assignment, which can be convenient. For example:
float f = 10; // Implicitly converts the integer 10 to a float
In this case, the integer `10` is automatically converted to a float type and assigned to the variable `f`. However, developers should be cautious about this behavior to avoid unexpected results due to type mismatches.
Using Constants
To enhance code clarity and prevent accidental changes, you can use constants in assignment statements with the `const` keyword. Here’s an example:
const int MAX_VALUE = 100;
Using a constant achieves two things: it assigns the value `100` to `MAX_VALUE`, and it communicates to anyone reading the code that this value should not be altered.
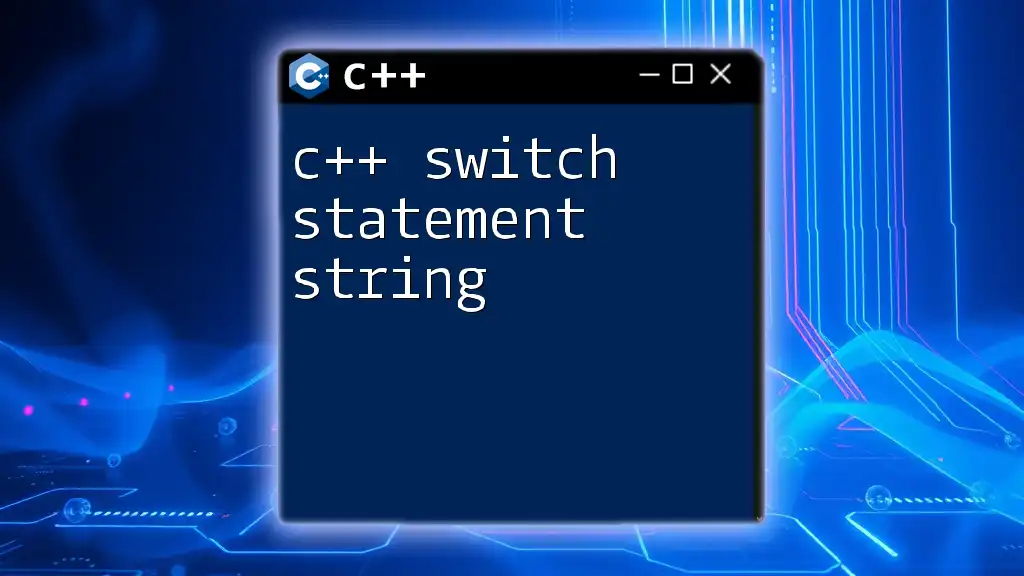
Common Errors and Troubleshooting
Common Mistakes
One common mistake with assignment statements is confusing assignment (`=`) with comparison (`==`). Consider the following incorrect usage:
if (x = 5) { // Incorrect: This assigns 5 to x, leading to logical error
}
The correct way to compare would be to use the `==` operator:
if (x == 5) {
}
Debugging Tips
To check for assignment-related errors, always review your conditional statements to ensure you are using the right operators. Utilize debugging tools such as IDE debuggers, print statements, or logging to track variable values throughout your program execution.
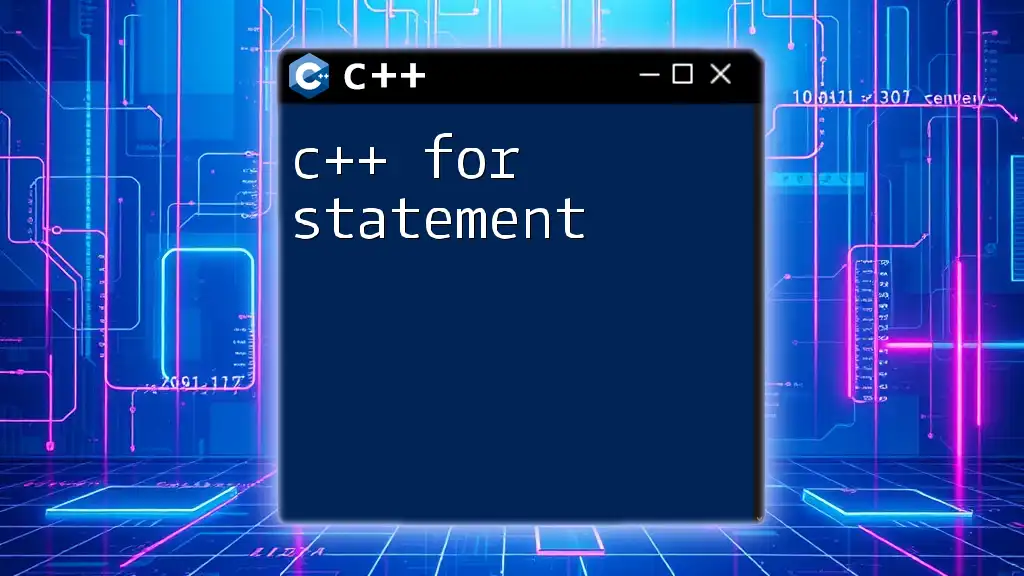
Practical Applications of Assignment Statements
Using in Conditional Statements
Assignment statements can sometimes cause errors in control flow, particularly within conditional statements. For example:
if (score = 100) { // This will always evaluate to true (score is set to 100)
// Code block executing if score is 100
}
Instead of assigning `100` to `score`, you should compare it against `100` like so:
if (score == 100) {
// Code block only executes if score is indeed 100
}
Loops and Assignment
Assignment statements play a crucial role inside loops by modifying variables that control loop execution. Here's an example of a loop that continually updates a variable:
for (int i = 0; i < 10; i++) {
x = i; // Updates the value of x in each iteration
}
In this snippet, the value of `x` is updated with the current loop index `i` in each iteration, demonstrating how assignments are integral to loop behavior.
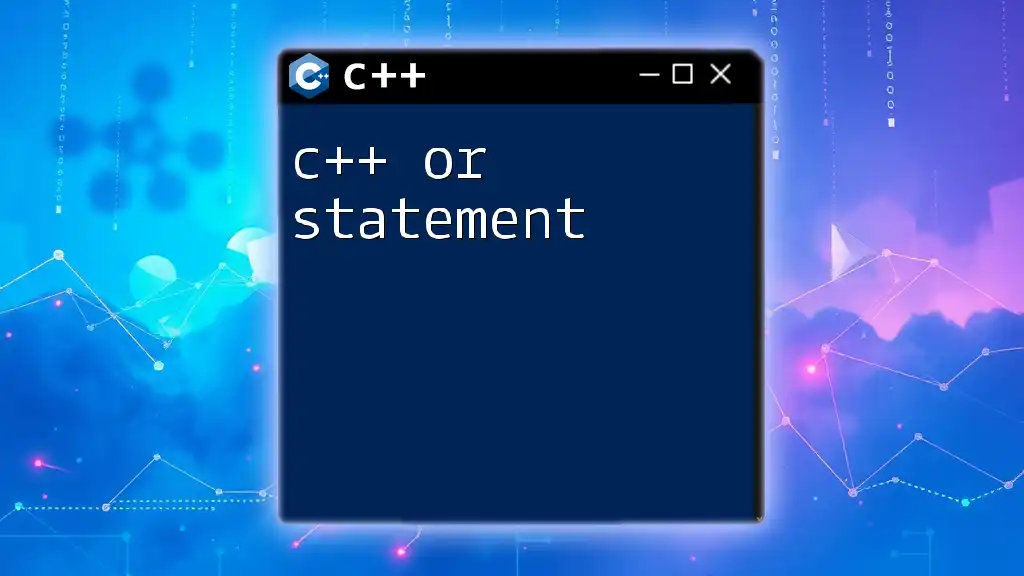
Conclusion
In summary, C++ assignment statements are essential for managing the values stored in variables. Understanding their syntax, types, and applications allows developers to write more effective and efficient code. Mastering assignment statements opens the door to more complex programming concepts and structures, reinforcing the foundational principles of C++. For those eager to enhance their skills, practice with various examples and assignments is highly recommended!
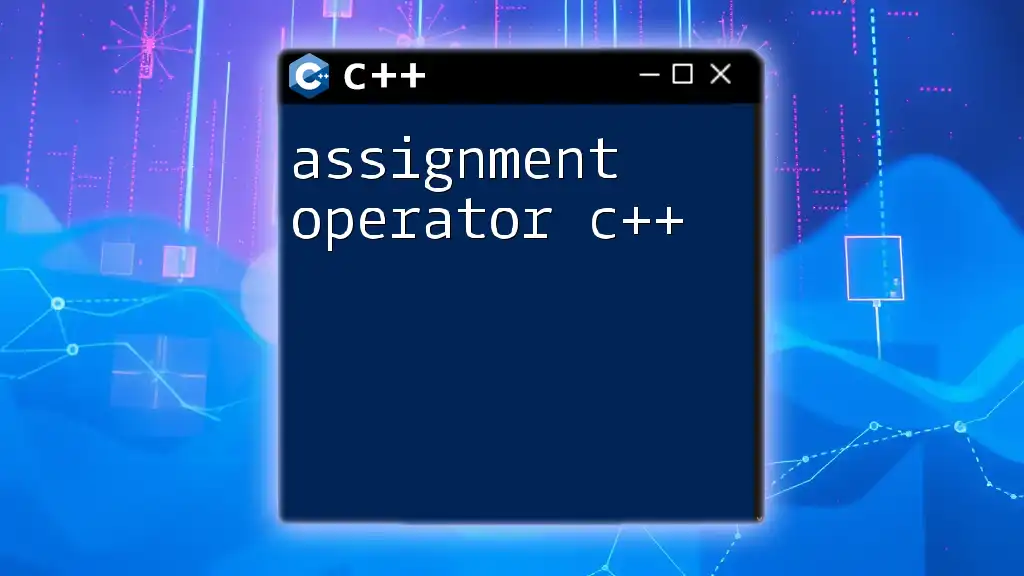
Additional Resources
To further your understanding of assignment statements and C++ programming, consider exploring these resources:
- Recommended books on C++ that cover both fundamental and advanced topics.
- Online platforms and coding challenges that provide practical experience and problem-solving opportunities.
- Links to the official C++ documentation for an in-depth discussion of operators, variables, and data types.