The "or" statement in C++ is a logical operator that evaluates to true if at least one of its operands is true, and it can be used to combine multiple conditional expressions.
if (condition1 || condition2) {
// Execute this block if either condition1 or condition2 is true
}
Understanding the Basics of the Or Statement in C++
What is an "Or" Statement?
An "Or" statement in C++ is a logical operator that evaluates two boolean expressions. If either expression is true, the overall condition becomes true. This operator is essential in decision-making processes, allowing the program to branch based on multiple conditions.
Logical operators form a backbone of control structures in programming. By using the "Or" statement, a programmer can create versatile and robust conditions in their code. This functionality is invaluable in scenarios where multiple criteria must be checked before executing particular code blocks.
Syntax of the Or Statement in C++
The syntax for the "Or" statement in C++ is straightforward. You use the `||` symbol to denote the logical OR. The basic structure looks like this:
if (condition1 || condition2) {
// code to execute if either condition is true
}
This structure serves as the foundation for using "Or" statements effectively in your code, allowing for clear and readable conditions.
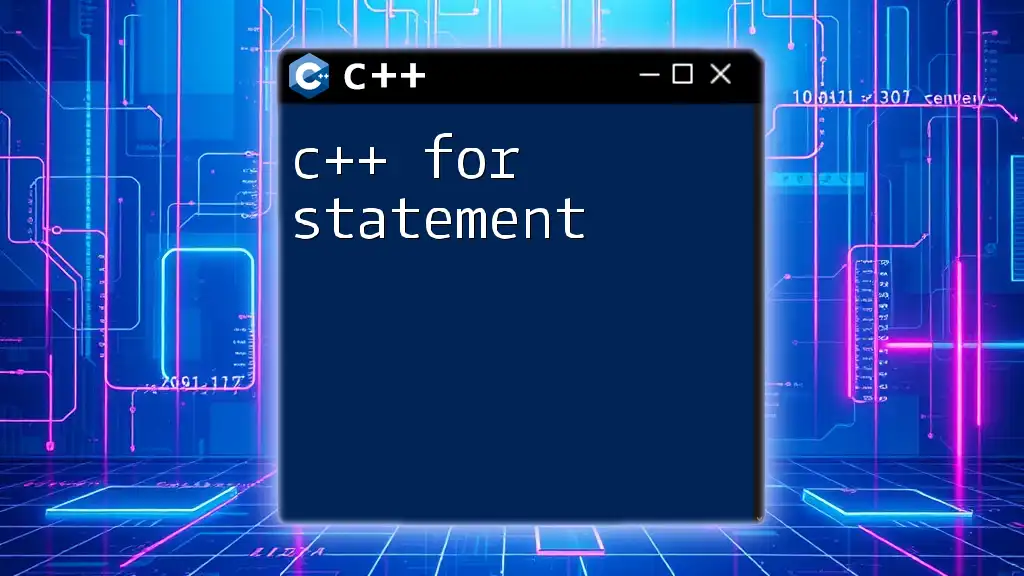
How the "Or" Statement Works
Logical Operators Overview
Understanding how various logical operators work is fundamental when using the "Or" statement. C++ provides three primary logical operators:
- `&&` (And): Returns true only if both operands are true.
- `||` (Or): Returns true if at least one of the operands is true.
- `!` (Not): Inverts the truth value of its operand.
Knowing how these operators work together can help you create more complex logical conditions.
Evaluating the "Or" Expression
The "Or" operator performs a truth evaluation by checking the values of the operands. If at least one of the operands evaluates to true, the entire expression is true. Notably, C++ employs short-circuit evaluation for the "Or" operator. This means that if the first condition is true, the second condition is not evaluated because the overall expression is already determined to be true.
Here’s a simple example to illustrate this:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a || b) {
cout << "At least one of them is true!" << endl;
}
return 0;
}
In this code, the output will be "At least one of them is true!" because `a` is true, regardless of the value of `b`.
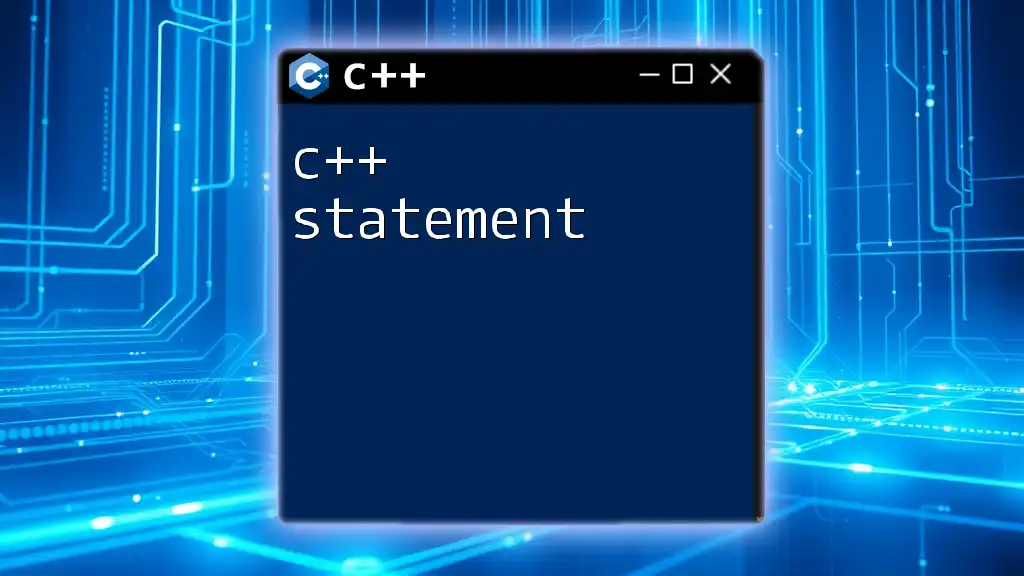
Practical Applications of the Or Statement
Using the Or Statement in Conditions
The practical uses of the "Or" statement are plentiful, especially in if statements. You can quickly check multiple conditions without nesting several if-statements, which improves readability and efficiency.
Consider the following example:
#include <iostream>
using namespace std;
int main() {
int x = 5;
int y = 10;
if (x < 10 || y < 5) {
cout << "At least one condition is true." << endl;
}
return 0;
}
In this scenario, the program will display "At least one condition is true." because although `y` is not less than 5, `x` satisfies the condition.
Combining Or and And Statements
Combining "Or" with "And" statements allows you to create complex conditions that can efficiently evaluate multiple logical paths.
For instance:
#include <iostream>
using namespace std;
int main() {
bool x = true;
bool y = false;
bool z = true;
if (x || (y && z)) {
cout << "Combination works!" << endl;
}
return 0;
}
Here, the program checks if `x` is true, and because it is, the inner `y && z` expression is irrelevant due to short-circuit behavior. This allows for robust and flexible condition checking.
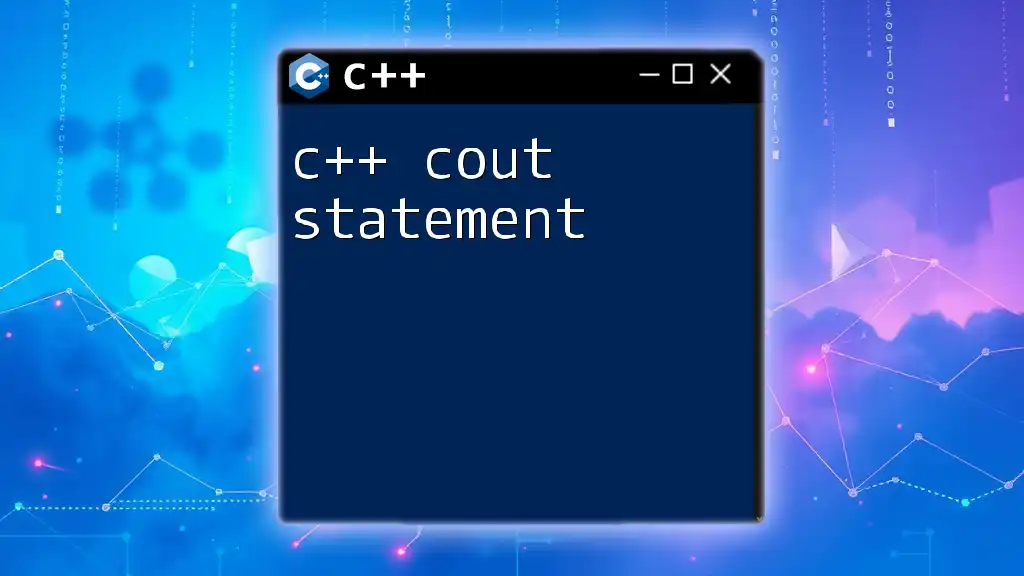
Common Mistakes Using the Or Statement
Logical Errors in Code
Common pitfalls occur when misusing logical operators. For instance, using `||` unintentionally when the intention was to use `&&` can lead to unexpected behavior in the program. Ensuring clarity and understanding of what each operator does is critical.
Best Practices for Using the Or Statement
One of the best practices when using the "Or" statement is to utilize parentheses to group conditions logically. Doing so greatly enhances the readability of your code. For example:
if ((a < 10 && b > 5) || c)
This expression makes it explicit which conditions are evaluated first, making your intentions clearer.
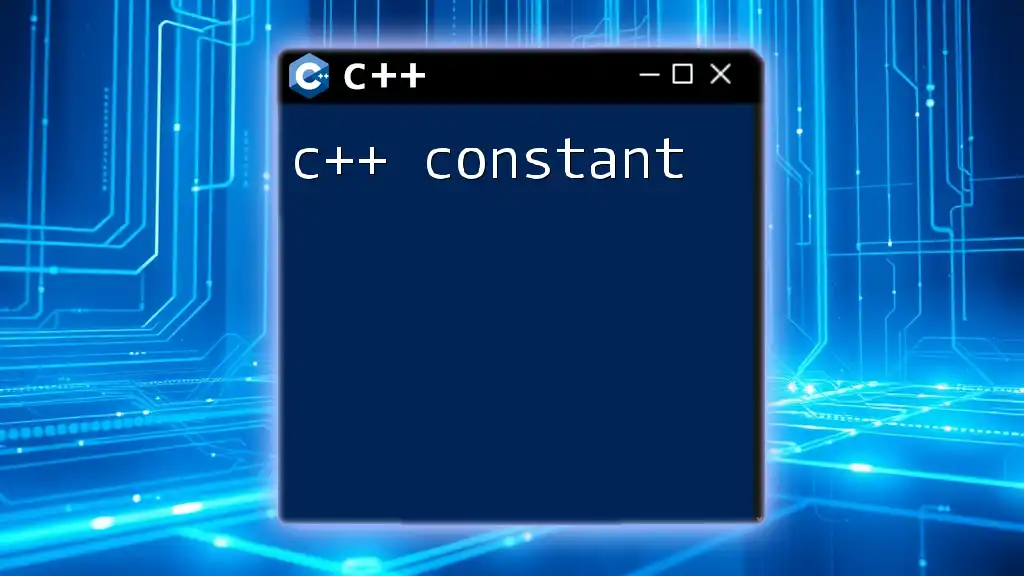
Comparison of Or Statement with Other Logical Operators
Differences Between Or and And Statements
The "And" statement (`&&`) requires all conditions to be true for the overall expression to evaluate as true, which contrasts with the "Or" statement. Understanding when to use these operators is crucial in controlling the flow of your program.
For example:
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 10;
if (a < 10 && b > 5) {
cout << "Both conditions are true!" << endl;
}
return 0;
}
This will output "Both conditions are true!" only if both conditions about `a` and `b` are satisfied.
When to Use Or vs And
In practical programming scenarios, use the "Or" statement when you want to proceed with an action if at least one condition is satisfied. Conversely, use the "And" statement when the goal requires multiple conditions to be true concurrently. Understanding the logic behind these operators will empower you to write more efficient and effective code.
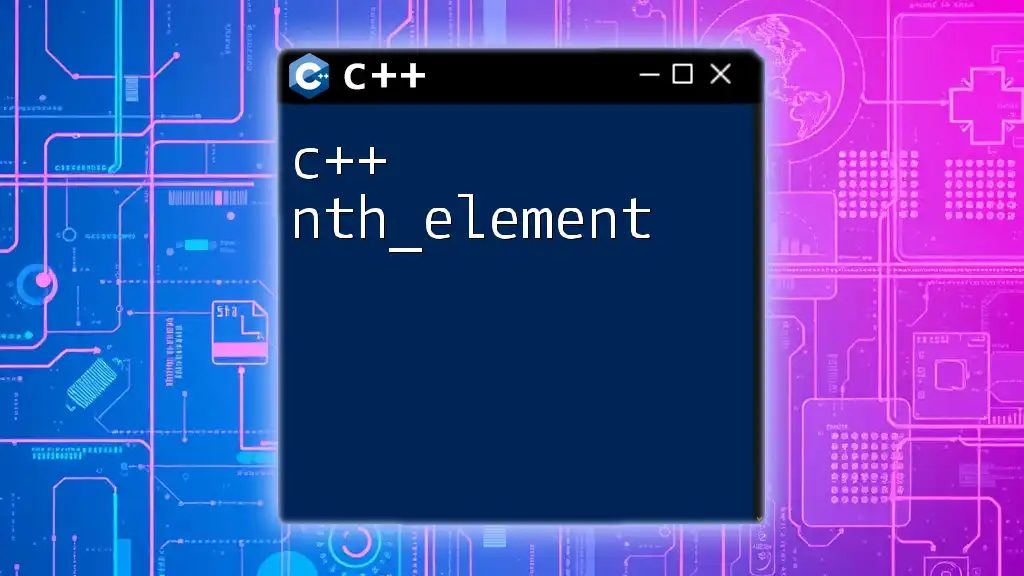
Conclusion
Recap of Key Points
The C++ "Or statement" serves as a robust tool for decision-making in your code. Its utility in checking multiple conditions concurrently makes it indispensable in programming logic. For best practices, always strive for clarity and logical grouping.
Further Reading & Resources
To deepen your understanding of logical operators, consult additional resources or practice exercises focused on advanced C++ topics. Engaging with varied code challenges will enhance your skills and comprehension of the "Or statement" and its counterparts.