C++ design patterns are reusable solutions to common problems in software design, enabling developers to create flexible and maintainable code.
Here's a simple example of the Singleton pattern in C++:
class Singleton {
private:
static Singleton* instance;
// Private constructor to prevent instantiation
Singleton() {}
public:
static Singleton* getInstance() {
if (!instance)
instance = new Singleton();
return instance;
}
};
Singleton* Singleton::instance = nullptr;
Overview of Design Patterns in C++
Design patterns are standardized solutions to common software design problems. Understanding them is crucial for developers looking to write efficient, reusable, and maintainable code. In C++, design patterns help in organizing code, promoting best practices, and fostering a clear architecture.
Categories of Design Patterns
Design patterns can be classified into three primary categories:
- Creational Patterns: These focus on object creation mechanisms, optimizing the instantiation process to create objects in a manner suitable for the situation.
- Structural Patterns: These deal with object composition and help in forming large structures from smaller objects, ensuring that if one part changes, the entire structure doesn't need to change.
- Behavioral Patterns: These focus on communication between objects, defining how they interact and collaborate to achieve a specific objective.
Common characteristics of design patterns include reusability, maintainability, and scalability, all of which contribute to higher code quality.
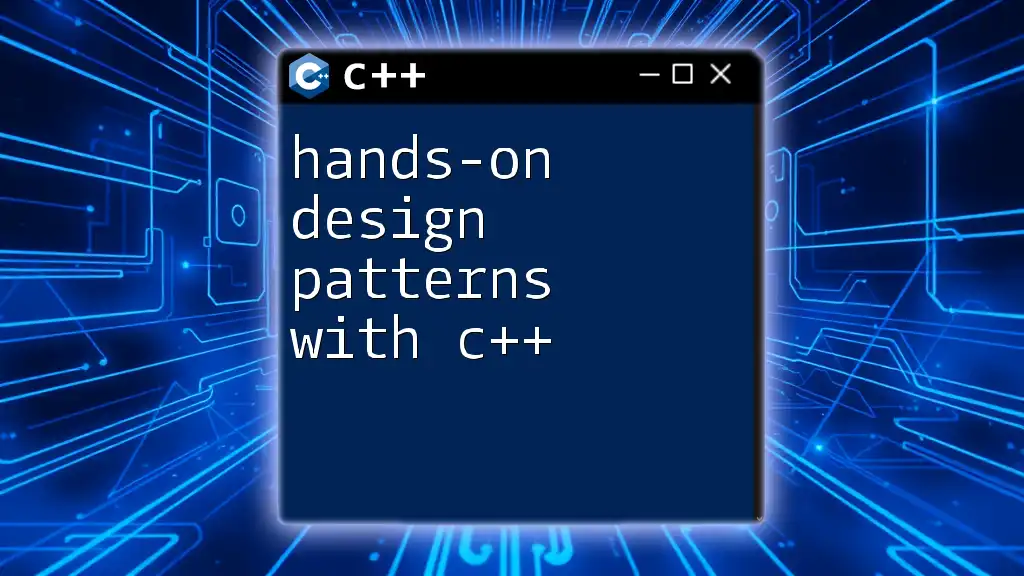
Creational Design Patterns in C++
Creational design patterns are best suited for scenarios where we need flexibility with object creation. Their primary goal is to help create objects in a way that satisfies the underlying design requirements.
Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This is particularly useful in scenarios where a centralized instance is needed, such as logging or configuration management.
class Singleton {
private:
static Singleton* instance;
Singleton() {}
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
// Initialization of the static member
Singleton* Singleton::instance = nullptr;
When to Use: The Singleton pattern is beneficial when exactly one instance of a class is needed throughout the lifecycle of an application. This can simplify coordination across various components, especially in settings where a shared resource is involved.
Factory Method Pattern
The Factory Method pattern provides an interface for creating an object but allows subclasses to alter the type of objects that will be created. This can significantly reduce dependencies and promote loose coupling.
class Product {
public:
virtual void use() = 0;
};
class ConcreteProductA : public Product {
public:
void use() override { /* implementation */ }
};
class Creator {
public:
virtual Product* factoryMethod() = 0;
};
class ConcreteCreatorA : public Creator {
public:
Product* factoryMethod() override {
return new ConcreteProductA();
}
};
When to Use: Use the Factory Method pattern when a client does not know ahead of time which class will be instantiated. Factories can help in managing product lifecycle and reduce direct dependencies between the client and the concrete classes.
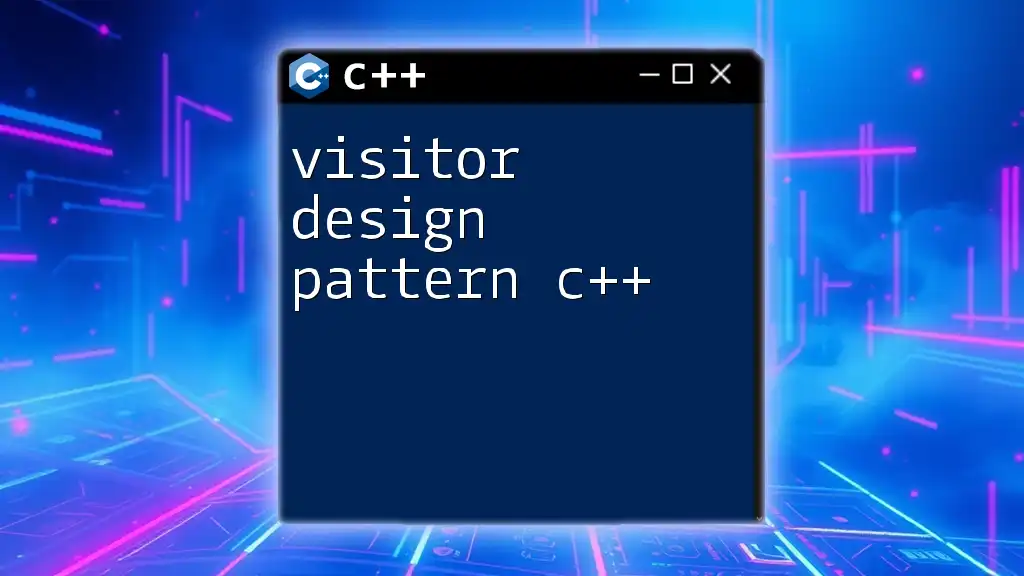
Structural Design Patterns in C++
Structural patterns are designed to simplify the relationships between different objects. They facilitate efficient organization of classes and objects into larger structures while ensuring manageable and scalable code.
Adapter Pattern
The Adapter pattern allows two incompatible interfaces to work together by acting as a bridge between them. This is especially useful when integrating new components without altering existing code.
class Target {
public:
virtual void request() = 0;
};
class Adaptee {
public:
void specificRequest() { /* implementation */ }
};
class Adapter : public Target {
private:
Adaptee* adaptee;
public:
Adapter(Adaptee* a) : adaptee(a) {}
void request() override { adaptee->specificRequest(); }
};
When to Use: The Adapter pattern is particularly useful when you're trying to integrate a new interface into an existing system without modifying your existing codebase. It's applicable when dealing with legacy systems or when using external libraries.
Composite Pattern
The Composite pattern allows you to compose objects into tree structures to represent part-whole hierarchies. This pattern treats both individual objects and compositions uniformly, enabling clients to interact with them seamlessly.
class Component {
public:
virtual void operation() = 0;
};
class Leaf : public Component {
public:
void operation() override { /* implementation */ }
};
class Composite : public Component {
private:
vector<Component*> children;
public:
void operation() override {
for (auto child : children) {
child->operation();
}
}
};
When to Use: Use the Composite pattern when you need to represent part-whole hierarchies of objects. It simplifies the client code as it can uniformly manage individual objects and composites, providing greater flexibility.
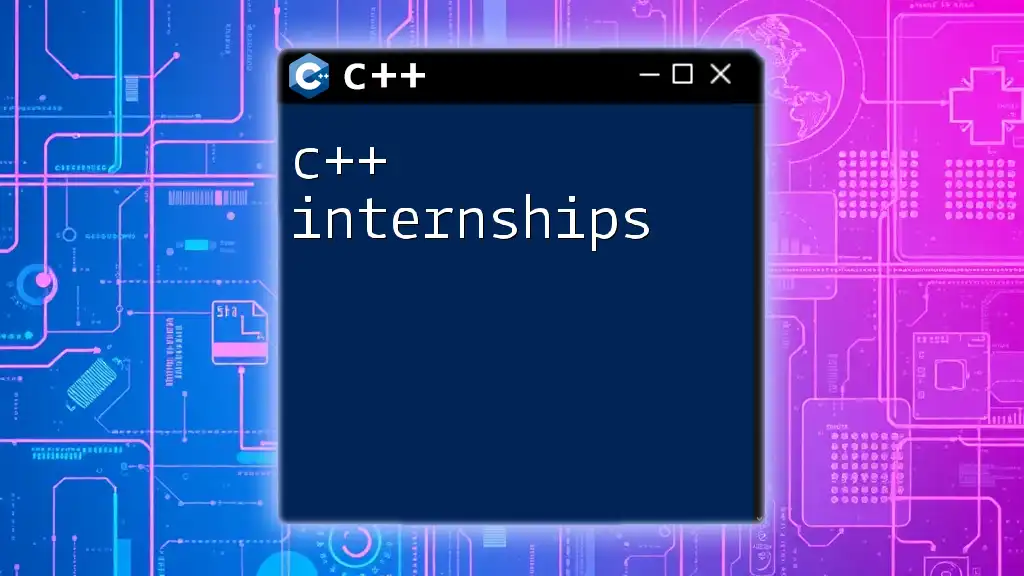
Behavioral Design Patterns in C++
Behavioral patterns define how objects communicate with each other. They facilitate better communication and cooperation between complex objects, defining the manners in which objects interact in a clear and defined manner.
Observer Pattern
The Observer pattern is a behavioral pattern that defines a one-to-many dependency between objects. When one object changes state, all registered observers are notified and updated automatically.
class Observer {
public:
virtual void update() = 0;
};
class Subject {
private:
vector<Observer*> observers;
public:
void attach(Observer* o) { observers.push_back(o); }
void notify() {
for (auto observer : observers) {
observer->update();
}
}
};
When to Use: The Observer pattern is useful when changes in one object must trigger reactions in other objects. Typical use cases include implementing event systems and maintaining consistency in application states.
Strategy Pattern
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern allows the algorithm to vary independently from the clients that use it.
class Strategy {
public:
virtual void execute() = 0;
};
class ConcreteStrategyA : public Strategy {
public:
void execute() override { /* implementation */ }
};
class Context {
private:
Strategy* strategy;
public:
void setStrategy(Strategy* s) { strategy = s; }
void executeStrategy() { strategy->execute(); }
};
When to Use: Use the Strategy pattern when you want to be able to select which algorithm to use at runtime without altering the code that uses it. This promotes cleaner, more manageable code and enhances flexibility.
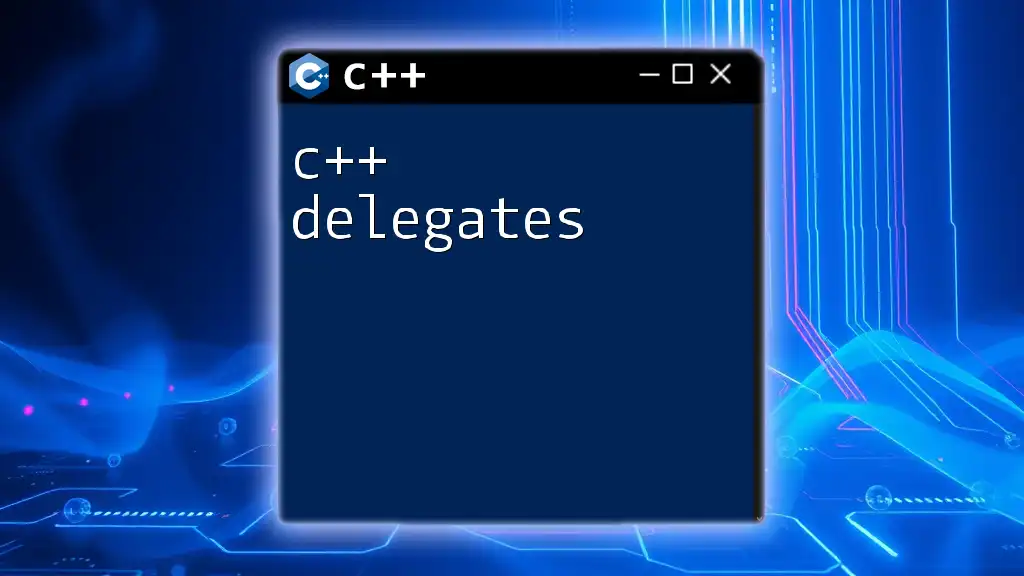
Conclusion
Understanding C++ design patterns is crucial for any developer looking to create robust, maintainable, and scalable applications. By leveraging design patterns such as Singleton, Factory Method, Adapter, Composite, Observer, and Strategy, you ensure that your code is easy to understand and adapt over time.
Learning and implementing these patterns in practice will not only enhance your programming skills but also enrich the quality of the software you develop. Don't hesitate to dive deeper into these patterns and explore their potential in your C++ projects!