Hands-on design patterns with C++ involve practical implementation of common design patterns to improve code organization, reusability, and maintainability; for example, using the Singleton pattern ensures a class has only one instance and provides a global point of access to it:
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private constructor
public:
static Singleton* getInstance() {
if (!instance)
instance = new Singleton();
return instance;
}
};
// Initialize static member
Singleton* Singleton::instance = nullptr;
What Are Design Patterns?
Design patterns are standard solutions to common problems in software design. They provide a set of best practices and guidelines that can be followed to improve code structure and maintainability. By utilizing design patterns, developers can save time and effort by leveraging established methodologies, which ultimately leads to more robust and scalable applications.

Benefits of Using Design Patterns
Implementing design patterns in your C++ projects presents several advantages:
- Enhance Code Reusability: Patterns promote a modular approach, allowing developers to reuse components across different projects.
- Improve Code Maintainability: A well-structured codebase that adheres to recognized patterns is easier to maintain and adapt as requirements evolve.
- Facilitate Better Communication Among Developers: Patterns serve as a shared language for developers, enabling them to discuss design decisions more effectively.
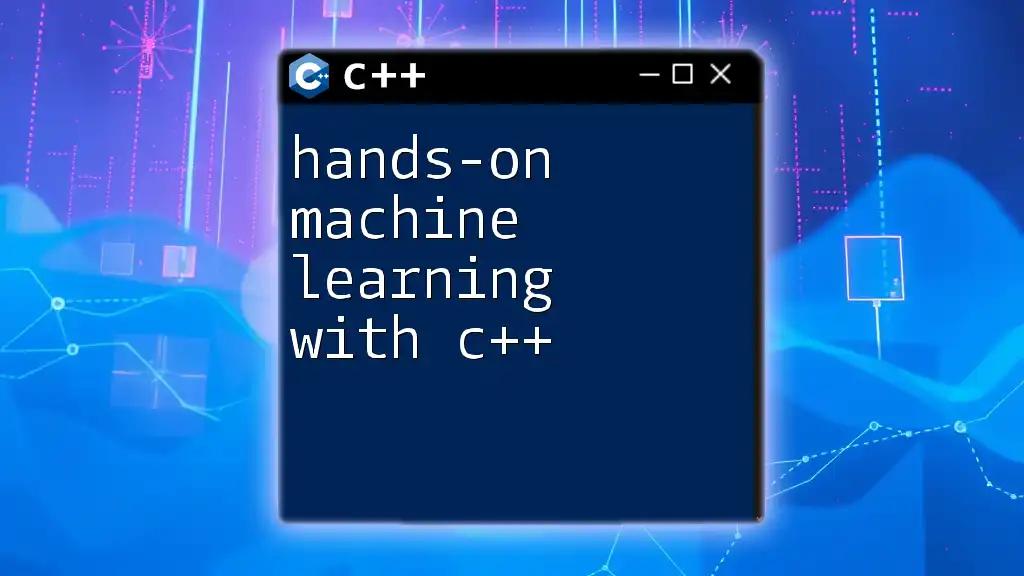
Overview of Design Patterns
Understanding the different types of design patterns is crucial for effectively utilizing them in C++. Design patterns are typically categorized into three groups:
Creational Patterns
These patterns are concerned with the way objects are created. They provide mechanisms to create objects in a manner suitable for the situation.
Structural Patterns
Structural patterns deal with object composition, allowing you to form large structures while keeping those structures flexible and efficient.
Behavioral Patterns
Behavioral patterns are focused on communication between objects, defining how objects interact and manage workflows.
Common Misconceptions
It’s important to clarify that design patterns are not merely code templates. They are conceptual tools that guide developers in solving design challenges, and their application should be tailored to the specific needs of a project.
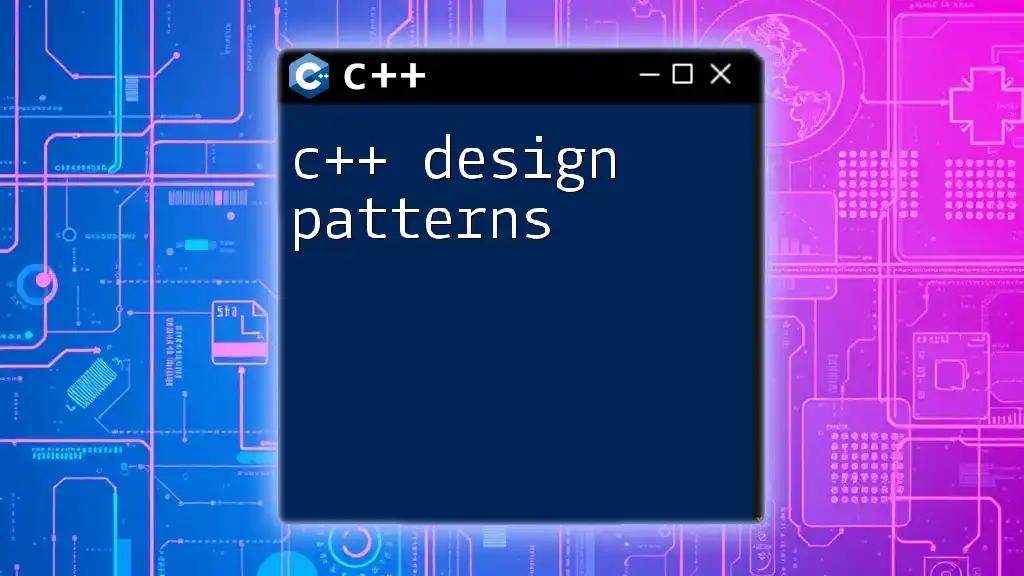
Creational Design Patterns
Factory Method
The Factory Method is a creational pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. This isolates the instantiation process from the client and encapsulates object creation.
Example in C++:
class Product {
public:
virtual void use() = 0;
};
class ConcreteProductA : public Product {
public:
void use() override {
// Implementation for ConcreteProductA
}
};
class Creator {
public:
virtual Product* factoryMethod() = 0;
};
class ConcreteCreatorA : public Creator {
public:
Product* factoryMethod() override {
return new ConcreteProductA();
}
};
When to Use: Use the Factory Method when you want to delegate the responsibility of object creation to subclasses. This is particularly useful when your code needs to work with a variety of related products that share a common interface.
Singleton Pattern
The Singleton Pattern ensures a class has only one instance while providing a global access point to this instance. This is crucial in scenarios where a single shared resource is required throughout an application.
Example in C++:
class Singleton {
private:
static Singleton* instance;
Singleton() {} // Private constructor
public:
static Singleton* getInstance() {
if (!instance) {
instance = new Singleton();
}
return instance;
}
};
When to Use: Implement the Singleton Pattern when you need to restrict instantiation of a class to a single object. This is common in use cases such as logging where maintaining one instance avoids resource conflicts.
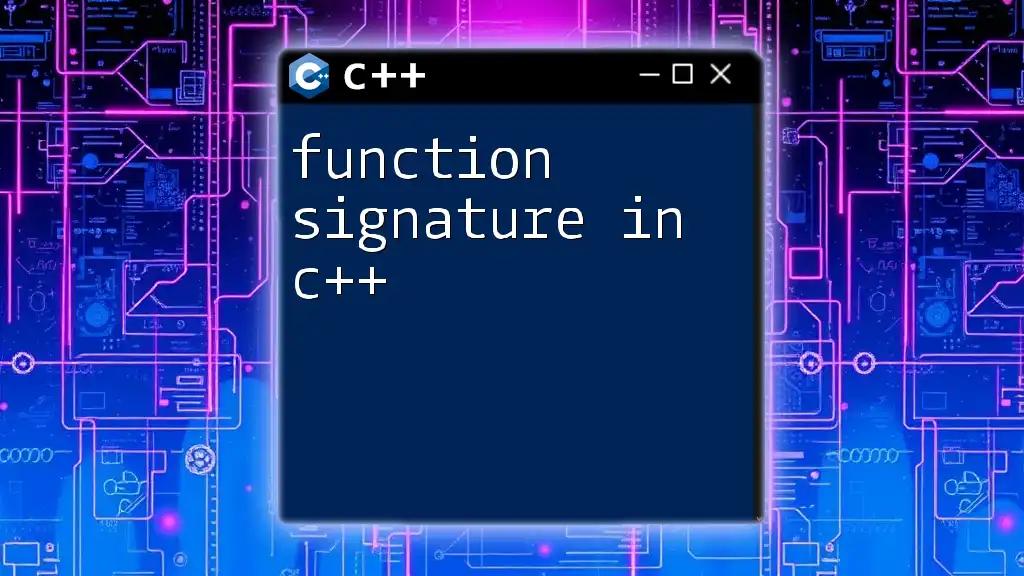
Structural Design Patterns
Adapter Pattern
The Adapter Pattern allows incompatible interfaces to work together. It involves creating an adapter that acts as a bridge between two incompatible interfaces.
Example in C++:
class Target {
public:
virtual void request() = 0;
};
class Adaptee {
public:
void specificRequest() {
// Implementation specific to Adaptee
}
};
class Adapter : public Target {
private:
Adaptee* adaptee;
public:
Adapter(Adaptee* a) : adaptee(a) {}
void request() override {
adaptee->specificRequest();
}
};
When to Use: Use the Adapter Pattern when you want to integrate a new system with an existing one without completely rewriting the legacy code, or when dealing with a third-party library that doesn’t match your application's interface.
Composite Pattern
The Composite Pattern is used to treat individual objects and compositions of objects uniformly. This is especially useful when working with tree structures.
Example in C++:
class Component {
public:
virtual void operation() = 0; // Common interface
};
class Leaf : public Component {
public:
void operation() override {
// Leaf-specific implementation
}
};
class Composite : public Component {
private:
std::vector<Component*> children;
public:
void add(Component* component) {
children.push_back(component);
}
void operation() override {
for (auto& child : children) {
child->operation(); // Delegate call to children
}
}
};
When to Use: Implement the Composite Pattern when you need to work with a tree-like structure, allowing clients to treat individual objects and compositions of objects uniformly.
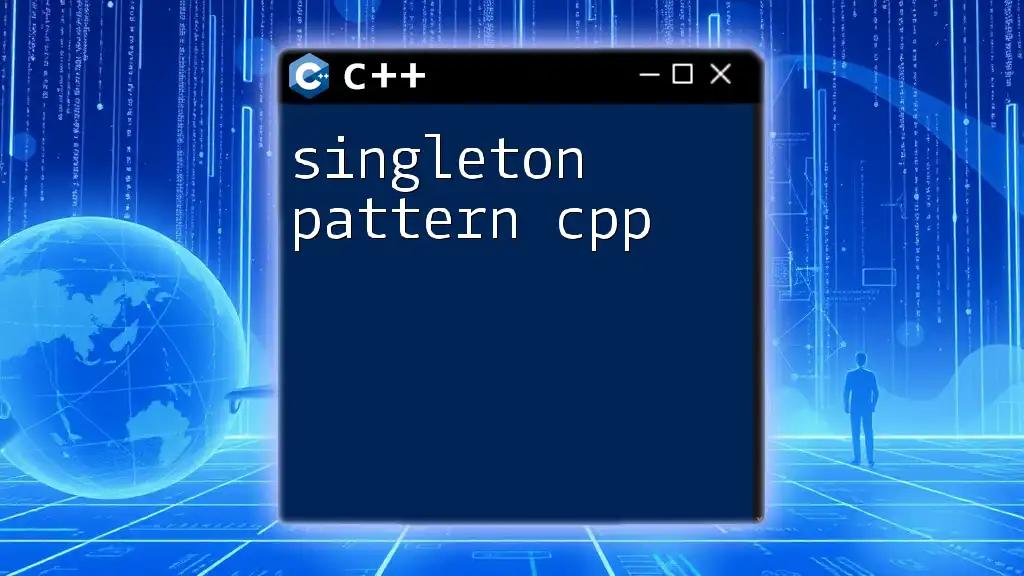
Behavioral Design Patterns
Observer Pattern
The Observer Pattern defines a one-to-many dependency between objects. When one object changes state, all its dependents are notified and updated automatically.
Example in C++:
class Observer {
public:
virtual void update() = 0; // Update method
};
class Subject {
private:
std::vector<Observer*> observers;
public:
void attach(Observer* observer) {
observers.push_back(observer); // Add an observer
}
void notify() {
for (auto& observer : observers) {
observer->update(); // Notify all observers
}
}
};
When to Use: The Observer Pattern is ideal in situations where changes in one object require updates in others, such as in event handling systems or data binding contexts.
Strategy Pattern
The Strategy Pattern is a behavioral pattern that defines a family of algorithms, encapsulates each one, and makes them interchangeable. It lets the algorithm vary independently from the clients that use it.
Example in C++:
class Strategy {
public:
virtual void execute() = 0; // Strategy interface
};
class ConcreteStrategyA : public Strategy {
public:
void execute() override {
// Implementation for ConcreteStrategyA
}
};
class Context {
private:
Strategy* strategy; // Reference to a strategy object
public:
void setStrategy(Strategy* newStrategy) {
strategy = newStrategy; // Set a new strategy
}
void executeStrategy() {
strategy->execute(); // Execute the strategy
}
};
When to Use: Use the Strategy Pattern when you want to select an algorithm at runtime, enabling flexibility in choosing how to accomplish a task without altering the context that uses it.

Conclusion
Throughout this comprehensive guide on hands-on design patterns with C++, we explored various patterns and their implementations. Design patterns not only enhance code organization but also foster better collaboration among developers. They offer timeless solutions that, when applied diligently, can significantly improve software quality and agility.
Practice is key. To master these concepts, implement them in your projects, adapting them to your unique challenges. Dive deeper into design patterns and enrich your C++ programming skills, allowing you to build powerful and maintainable software applications.
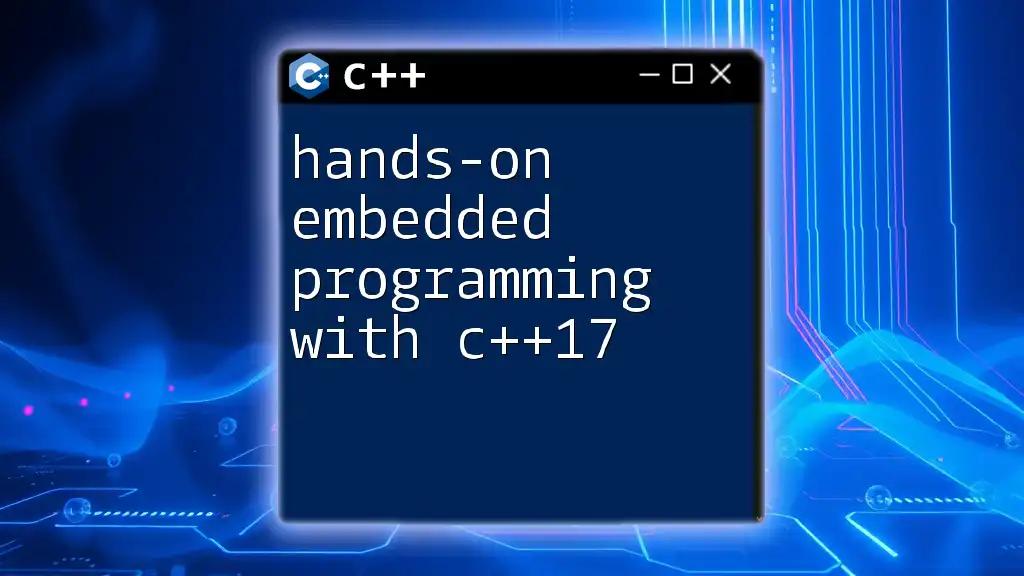
Additional Resources
To further your understanding of design patterns in C++, consider exploring reputable books, engaging with online tutorials, and participating in developer communities and forums where you can discuss and share experiences with others interested in design patterns.