A game engine built with C++ allows developers to create high-performance games by utilizing low-level programming capabilities to manage resources, graphics, and gameplay mechanics efficiently.
Here's a simple code snippet demonstrating how to initialize a graphical window using the SFML library, a popular choice for game development in C++:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Game Engine Example");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
// Game rendering goes here
window.display();
}
return 0;
}
What is a Game Engine?
A game engine is a robust software framework designed to facilitate the development and creation of video games. It provides developers with a suite of tools and services to manage complex tasks such as rendering graphics, handling physics, processing user input, and managing audio. The rise of game engines has democratized game development, allowing both indie creators and large studios to produce high-quality games more efficiently.
Historically, game engines evolved from specialized software created for individual games to comprehensive tools that can be used across multiple projects. This shift has enabled a more modular development process, where teams can focus on content creation rather than the underlying engine architecture.
Core components of a game engine include:
- Rendering Engine: Responsible for displaying graphics, rendering 2D or 3D representations of game objects and environments.
- Physics Engine: Simulates real-world physics to calculate and manage interactions, movements, and collisions between objects.
- Audio Engine: Handles sound effects and background music, allowing for immersive audio experiences.
- Input Handling: Captures and processes user input from devices like keyboards, controllers, and touchscreens.
- Scripting and Game Logic: Provides the means for developers to implement game rules and object behaviors using scripting languages or C++.
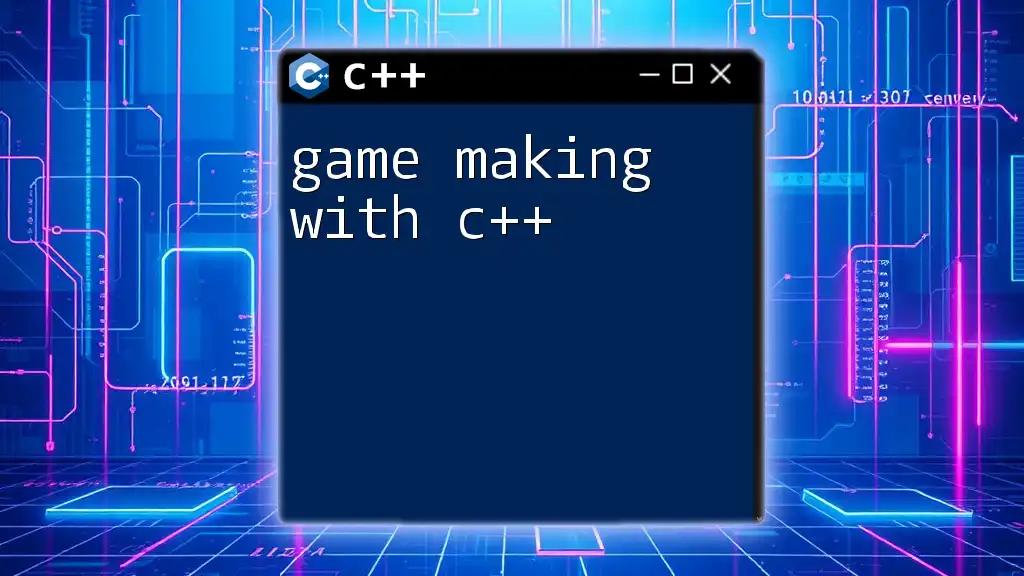
Why Use C++ for Game Engines?
C++ is a preferred language for game engine development due to several compelling reasons:
Performance and Efficiency: C++ is known for its high performance, allowing developers to write code that runs efficiently, which is crucial for resource-intensive applications like games. This is especially important in high-performance settings, such as first-person shooters or real-time strategy games, where frame rates and response times can greatly impact player experience.
Low-Level Memory Management: C++ gives developers fine-grained control over memory management, allowing them to manage resources optimally. This capability is vital in game development, as poor memory management can lead to performance bottlenecks or crashes.
Established Industry Standard: Many well-known game engines, including Unreal Engine and CryEngine, are built using C++. Working in this environment provides access to a vast array of tutorial resources, libraries, and community support.
Large Community and Resources: The extensive C++ developer community contributes a wealth of knowledge, tools, and libraries that can be leveraged to simplify game development.
Compatibility with Other Languages and APIs: C++ can easily work alongside other programming languages and vital graphics APIs like OpenGL and DirectX, allowing developers to create powerful and flexible gaming solutions.
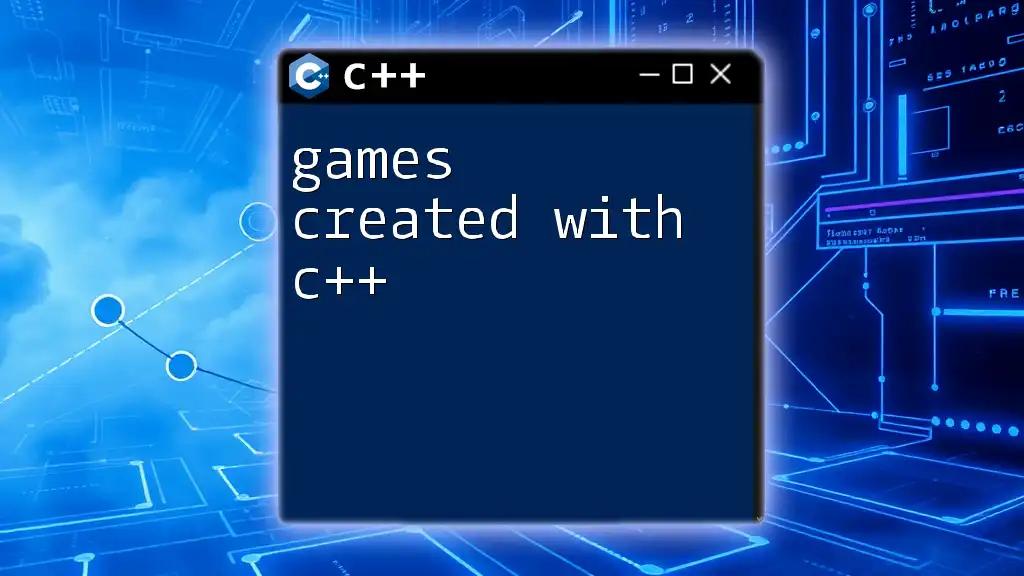
Popular C++ Game Engines
Unreal Engine
Unreal Engine is one of the most recognized C++ game engines available. Developed by Epic Games, it is renowned for its stunning graphics capabilities and versatile toolset.
Features include a complete suite for 3D modeling, animation, and world creation, as well as a visual scripting language (Blueprints) that allows developers without deep programming knowledge to create complex interactions.
For example, below is a simple C++ snippet that showcases how to implement character movement:
void AMyCharacter::Tick(float DeltaTime) {
Super::Tick(DeltaTime);
FVector Forward = GetActorForwardVector();
AddMovementInput(Forward, 1.0f); // Move forward
}
CryEngine
CryEngine prioritizes exceptional graphics and realistic physics. Its capabilities make it particularly attractive for developers building open-world games.
In addition to excellent rendering capabilities, CryEngine offers comprehensive tools for asset management and environmental design.
Here’s a snippet to apply physics to an object in CryEngine:
void MyEntity::OnUpdate(float deltaTime) {
Vec3 direction = GetForwardDir();
GetPhysics()->AddForce(direction * forceAmount);
}
Godot Engine
While primarily known for its user-friendly design, Godot allows for C++ integration through GDNative. This flexibility means developers can harness C++ performance for the core engine while using Godot’s built-in scripting languages for rapid prototyping.
An example showing how to implement a basic game object in Godot might look like this:
void MyGameObject::_process(float delta) {
position.y -= delta * gravity; // Apply gravity
set_position(position); // Update position
}
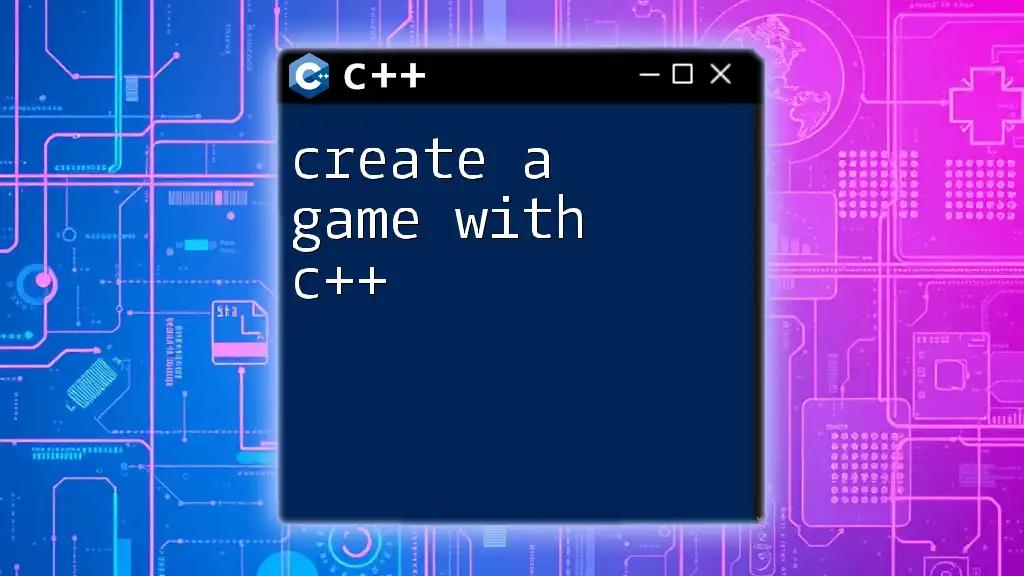
Getting Started with Developing a Game Engine in C++
Embarking on the journey to create a game engine in C++ requires a solid grip on various key concepts that form the backbone of game creation.
Key Concepts to Understand
It is essential to grasp object-oriented programming principles, as they enable code reusability and modular design. Understanding the game loop is crucial, as it drives the game's updates and frame rendering. Moreover, mastering scene management helps maintain and organize game states and assets efficiently.
Basic Structure of a C++ Game Engine
Creating a basic game engine structure lays the foundation for further development. The main loop of your engine will process game logic, handle user input, and render graphics. A simple overview of the game loop is as follows:
while (gameIsRunning) {
processInput();
updateGameLogic();
render();
}
Handling Game Objects
In a game engine, game objects are central. These are the entities that compose your game world, such as characters, enemies, or items. To model a basic game object, we could create a class like this:
class GameObject {
public:
void update();
void render();
private:
int positionX;
int positionY;
};
This code snippet demonstrates the essential structure of a game object that can be expanded with additional properties and methods for movement, rendering, and interactivity.
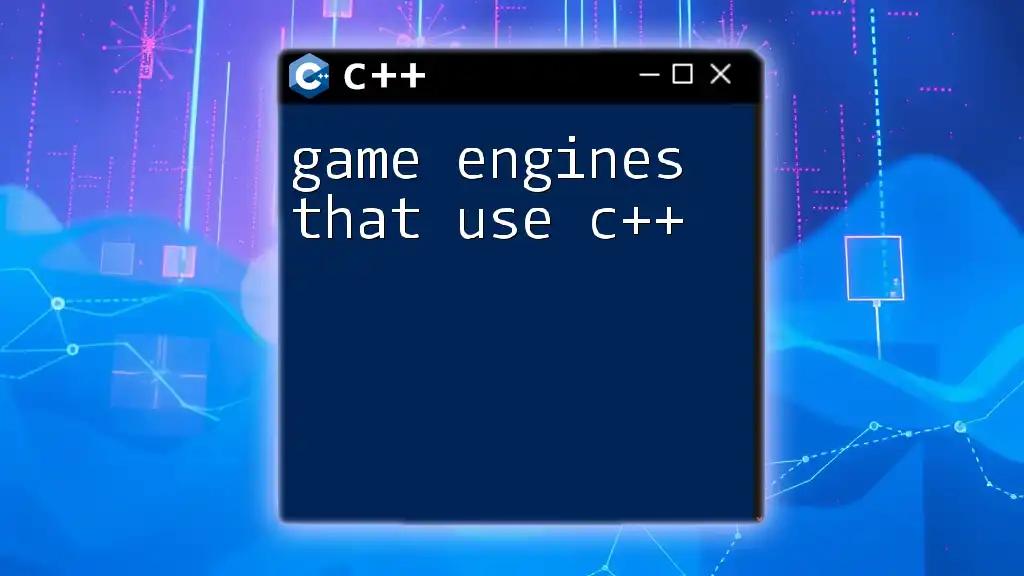
Building a Simple Game Engine: Step-by-Step
Environment Setup
Before diving into C++ game engine development, setting up a suitable development environment is essential. Recommended IDEs include Visual Studio, CLion, or even lightweight options like Code::Blocks. Familiarize yourself with common dependencies like OpenGL, DirectX, or SDL, depending on the graphics API you choose to use.
Creating the Engine Framework
Drafting the overall architecture involves defining modules for each core component mentioned earlier. Begin with a rendering engine; whether you choose OpenGL or DirectX, ensure you grasp the necessary libraries and functions for effective implementation.
Integrating Physics and Collision Detection
Developing a physics system can start with basic concepts. Here’s an example of how to apply gravity to an object:
void applyGravity(GameObject& object) {
object.positionY -= GRAVITY * deltaTime; // Apply gravitational force
}
Implementing collision detection can begin with bounding box comparisons, allowing your game objects to interact meaningfully when they overlap in the game world.
Adding User Input Handling
Capturing user input is crucial for immersive gameplay. Implement an example of basic input handling like this:
if (isKeyPressed('W')) {
player.moveUp(); // Player moves upward when 'W' is pressed
}
This example illustrates how simple input processing can lead to immediate gameplay response.
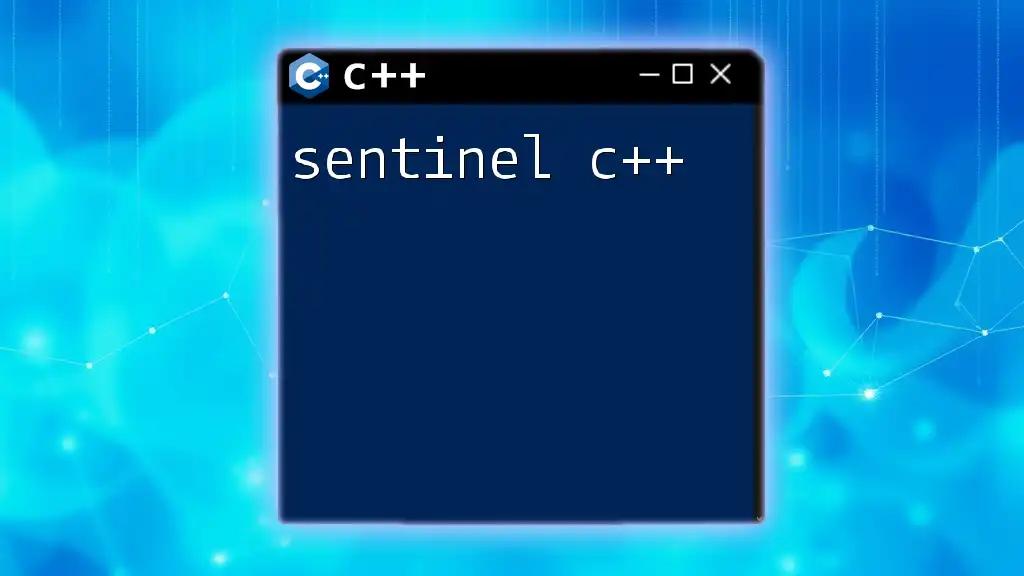
Best Practices for C++ Game Engine Development
To ensure your game engine is robust and maintainable, adopt these best practices:
Code Organization and Architecture: Structure your code for modularity, separating concerns within different classes or modules. This will improve maintainability and clarity.
Memory Management Strategies: Be vigilant about managing memory, especially with frequent scene loads and object instantiations. Utilize smart pointers where appropriate to manage resource lifecycles efficiently.
Performance Optimization Techniques: Focus on optimization from the outset. Profile your engine regularly to identify performance bottlenecks and address them proactively.
Handling Cross-Platform Development: Aim for a codebase that can run smoothly across platforms without heavy modifications. Use portable libraries and avoid system-dependent code when possible.
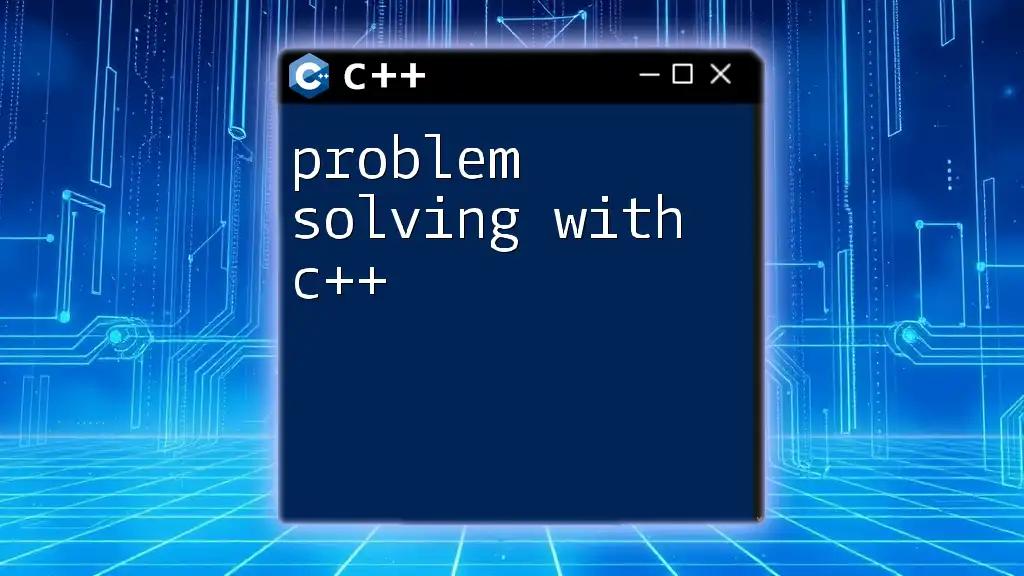
Case Studies: Successful Games Built on C++ Engines
Analyzing established titles can provide valuable insights into effective C++ game engine development.
Fortnite, developed on Unreal Engine, showcases Whats possible with C++. Its dynamic gameplay mechanics and vibrant graphical style can be attributed to Unreal Engine's robust capabilities.
Far Cry, leveraging CryEngine, presents a stunning open-world experience powered by advanced graphics and physics systems, demonstrating how powerful C++ engines can drive visual fidelity.
Hollow Knight created its engine with C++, emphasizing how bespoke engines can deliver unique artistic visions through finely-honed design practices.
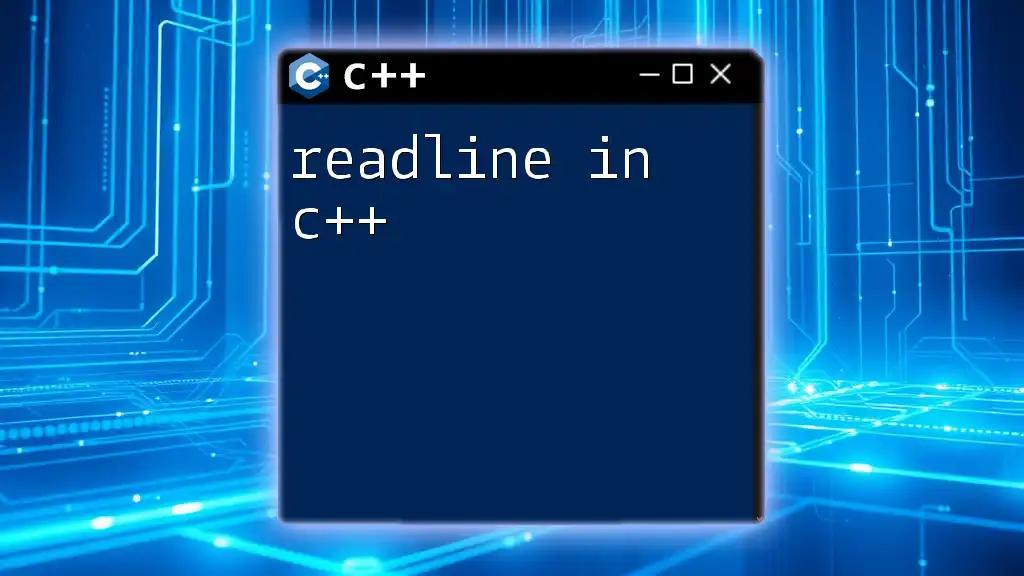
Future Trends in C++ Game Engine Development
As technology evolves, several trends are emerging within the realm of C++ game engine development:
Emerging Technologies: The integration of ray tracing, virtual reality, and augmented reality provides exciting opportunities to push the boundaries of gameplay and immersion.
The Role of AI and Machine Learning: Developing intelligent characters and adaptive systems is becoming easier with advances in AI. Incorporating machine learning can lead to more dynamic and responsive gameplay experiences.
Predictions for the Future of C++ in Game Engines: C++ will likely remain a core language for performance-sensitive areas, even as new languages gain popularity. Innovations in compilers and tooling will continue to enhance the C++ developer experience.
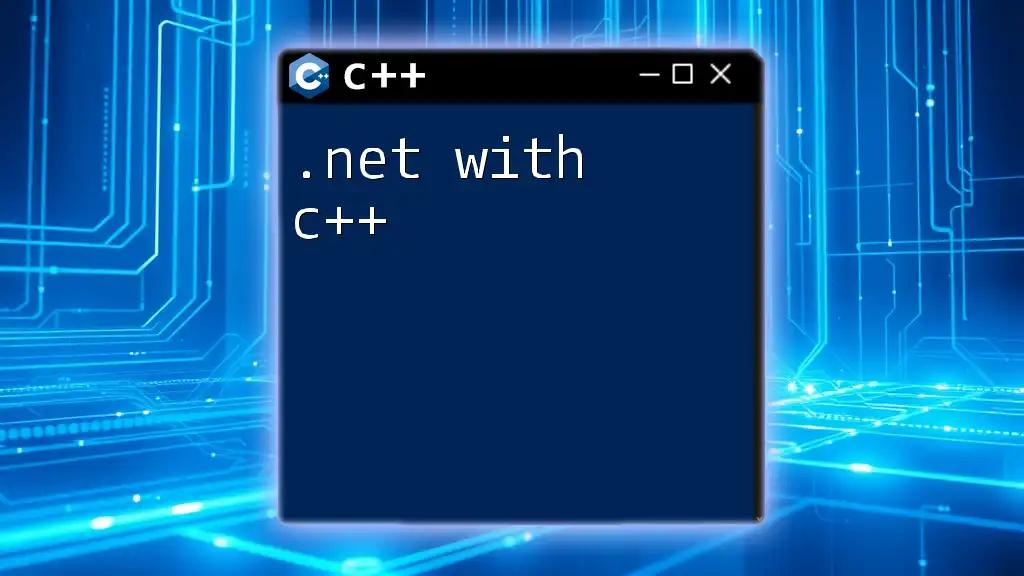
Conclusion
The significance of game engines with C++ cannot be overstated. Their effectiveness in producing high-quality games underpins much of the gaming industry’s success. As aspiring developers begin their journeys, understanding and mastering C++ in game engine development opens doors to endless possibilities for creativity and innovation.
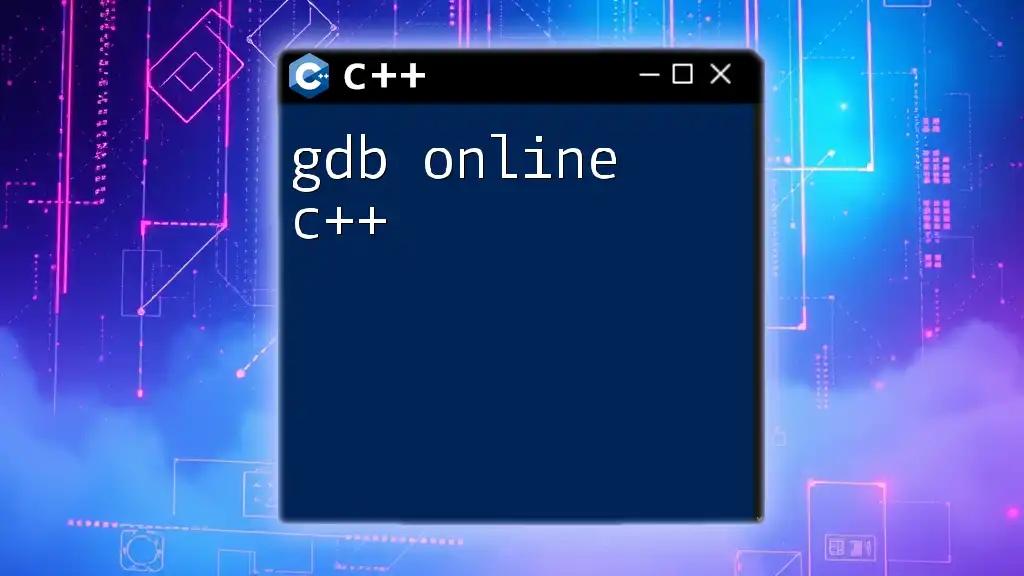
Resources
To further your knowledge in C++ game engine development, consider the following resources:
- Recommended Books on C++: Delve deeper into your C++ skills with foundational literature.
- Online C++ Game Development Courses: Engage in targeted learning through structured courses.
- Forums and Community Resources: Join discussions, ask questions, and share your experiences with other developers to foster a collaborative learning environment.
Start building your dream game engine today, and contribute to the ever-evolving landscape of game development!