Hacking with C++ involves utilizing the language's powerful features to develop software tools for security testing and exploit development.
#include <iostream>
#include <string>
int main() {
std::string password;
std::cout << "Enter the password: ";
std::cin >> password;
if(password == "securepassword") {
std::cout << "Access Granted!" << std::endl;
} else {
std::cout << "Access Denied!" << std::endl;
}
return 0;
}
Understanding Hacking Ethics
The Legal Considerations
In the realm of hacking, understanding the distinction between ethical hacking and malicious hacking is crucial. Ethical hacking involves probing systems for vulnerabilities with permission to strengthen security. It helps organizations identify potential weaknesses before malicious hackers do. On the other hand, malicious hacking refers to unauthorized intrusions focused on exploiting systems for personal gain or causing harm. It’s important to familiarize yourself with cybersecurity laws to avoid severe penalties and criminal charges.
Responsible Disclosure
When you identify a vulnerability, understanding the process of responsible disclosure is vital. Responsible disclosure refers to the ethical practice of informing the affected parties about discovered vulnerabilities, allowing them to rectify the flaws before malicious entities exploit them. Follow best practices, such as providing a detailed report of the vulnerability and giving the organization sufficient time to respond before making the vulnerability public.
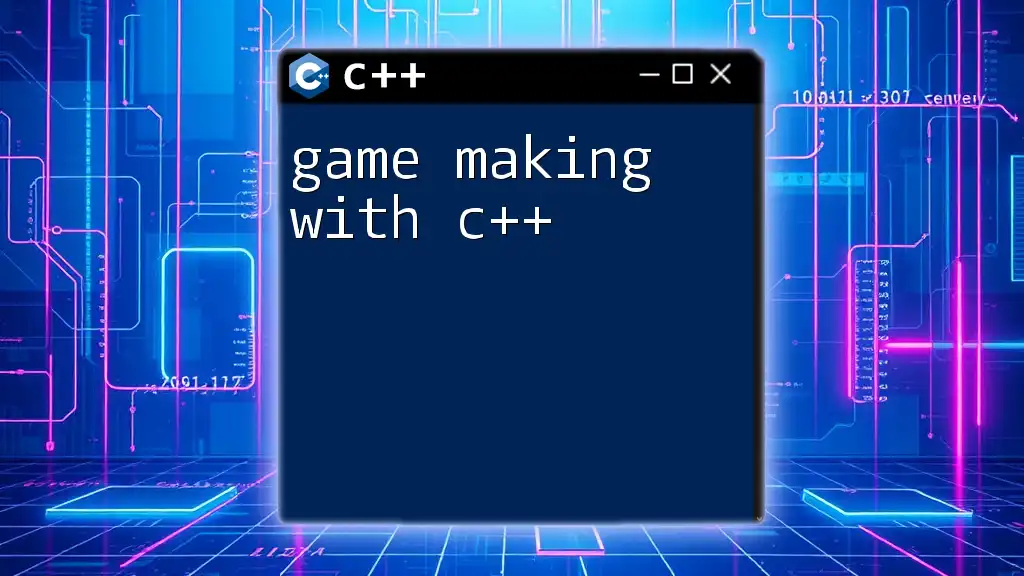
Basics of C++ for Hacking
Why C++ in Hacking?
C++ is a powerful programming language ...
-
Performance and memory manipulation advantages: C++ provides low-level memory access, making it suitable for tasks requiring direct manipulation of system resources. This capability is especially useful in developing exploits and understanding how programs interact with memory.
-
Comparison with other programming languages: While languages such as Python and JavaScript are popular in hacking circles, C++ offers speed and efficiency that is unmatched for certain tasks, specifically those that require close interaction with hardware and system resources.
Setting Up Your C++ Environment
To start your journey in hacking with C++, you'll need a proper development environment. There are several recommended IDEs and tools, including:
- Visual Studio: A comprehensive IDE for Windows development.
- Code::Blocks: A free and open-source IDE that supports multiple compilers.
- g++ (GNU Compiler Collection): Ideal for Linux users, providing a robust command-line environment.
Setting up the environment is straightforward. For example, installing `g++` on a Linux system can be done through:
sudo apt install g++
Now you are ready to write and compile your C++ programs!
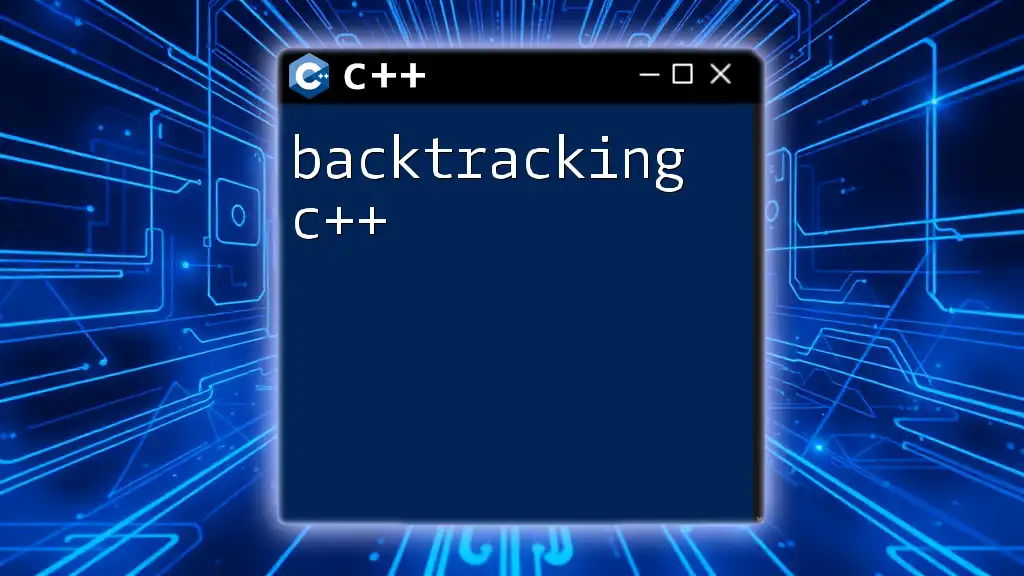
C++ Fundamentals for Security Professionals
Key Concepts
Understanding the foundational concepts of C++ is essential for hacking.
-
Variables and Data Types: Grasping how to efficiently use variables and types helps in managing memory resources effectively, which is crucial for certain hacking techniques.
-
Control Structures: Using control structures like loops and conditionals helps to create dynamic scripts that respond to different scenarios when probing for vulnerabilities.
Pointers and Memory Management
One of the most important aspects of C++ relevant to hacking is memory manipulation through pointers. Pointers allow for direct access to memory addresses, enabling you to change the values stored at those addresses. For example:
int* ptr = new int; // Dynamically allocating memory
*ptr = 10; // Assigning value
delete ptr; // Freeing memory
This snippet shows how pointers can be used to manage memory allocation effectively. Understanding pointers is key to tasks like buffer overflows and other exploits.
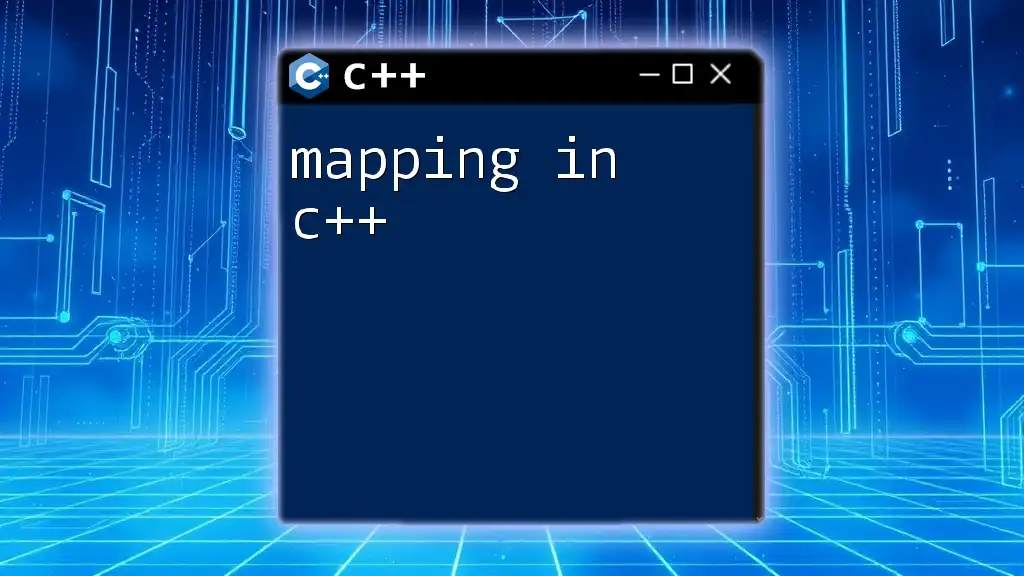
Networking Fundamentals with C++
Socket Programming
Networking is at the heart of many hacking activities, and understanding socket programming is pivotal. A socket acts as an endpoint for sending or receiving data across a computer network.
To create basic TCP sockets in C++, you can use the following framework:
#include <sys/socket.h>
#include <netinet/in.h>
// Example: Creating a socket
int sockfd = socket(AF_INET, SOCK_STREAM, 0);
This code snippet initializes a TCP socket which can then be used to connect to servers or services.
Network Protocols
Familiarizing yourself with the common protocols utilized in hacking is essential. Protocols like HTTP and FTP serve different purposes but are crucial for understanding how data is transferred over networks. Delve into their structures and how they can be manipulated to exploit weaknesses in applications.
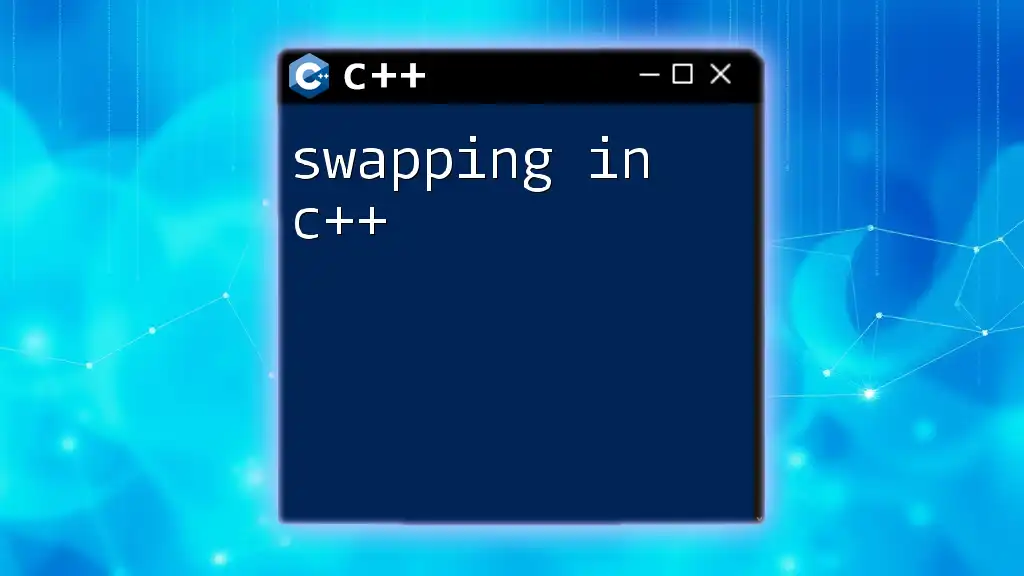
Hacking Techniques with C++
Writing a Simple Keylogger
Creating a keylogger is an intriguing exercise in understanding how keystrokes can be captured. However, it's essential to emphasize the ethical considerations—only use such techniques on authorized systems.
Here’s an outline of a simple C++ keylogger:
#include <windows.h>
// Example: Simple key hook
LRESULT CALLBACK KeyboardProc(int nCode, WPARAM wParam, LPARAM lParam) {
// Logger implementation
}
This code initializes a keyboard hook that captures keystrokes. Proper implementation can reveal significant insights into user behavior.
Creating a Basic Network Sniffer
Understanding how to capture and analyze network traffic is a fundamental skill for any hacker. A network sniffer monitors network packets flowing through the system.
// Example: Using raw sockets to capture packets
This simple code leverages raw sockets to intercept packets sent over the network. Analyzing these packets can reveal sensitive information such as plain-text credentials.
Exploit Development
Exploit development focuses on uncovering and taking advantage of vulnerabilities in software. Common vulnerabilities include buffer overflows and race conditions.
For example, consider this simple buffer overflow vulnerability:
void vulnerableFunction() {
char buffer[50];
gets(buffer); // Dangerous function
}
The use of `gets()` permits writes beyond the allocated buffer, leading to potential exploitation. Understanding these vulnerabilities and how to exploit them is crucial for sharpening your hacking skills.
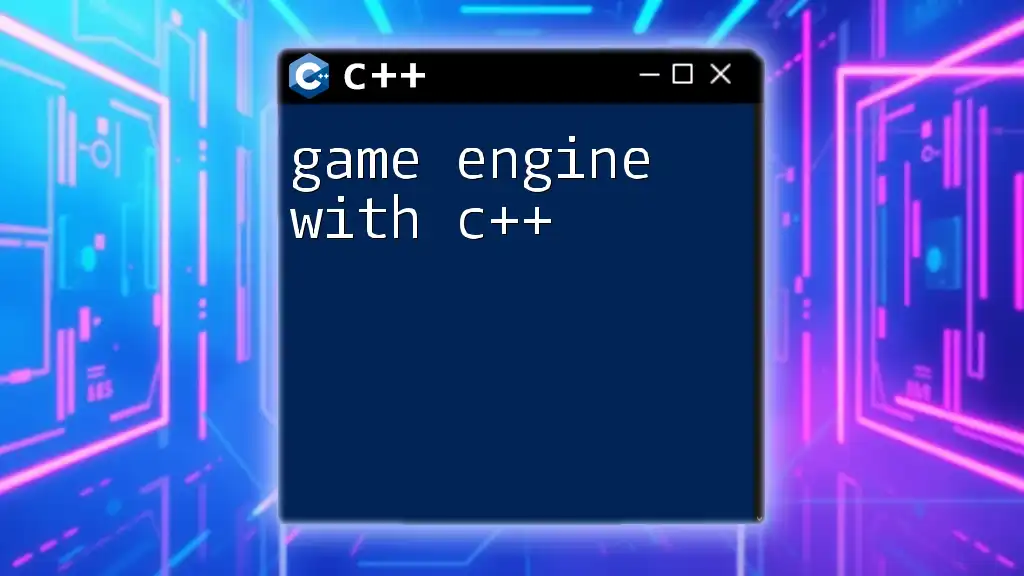
Tools and Libraries for C++ Hackers
Leveraging Open Source Libraries
Utilizing open-source libraries can extend the functionality of your hacking tools. Boost and OpenCV are noteworthy libraries. Boost provides a wealth of libraries that improve programming productivity, while OpenCV might help with image processing tasks in specific hacking scenarios.
Common Tools Built in C++
Familiarizing yourself with established hacking tools like Metasploit and Nmap expands your toolkit significantly. Investigating their source code will provide insights into well-structured techniques you can adopt for your projects.
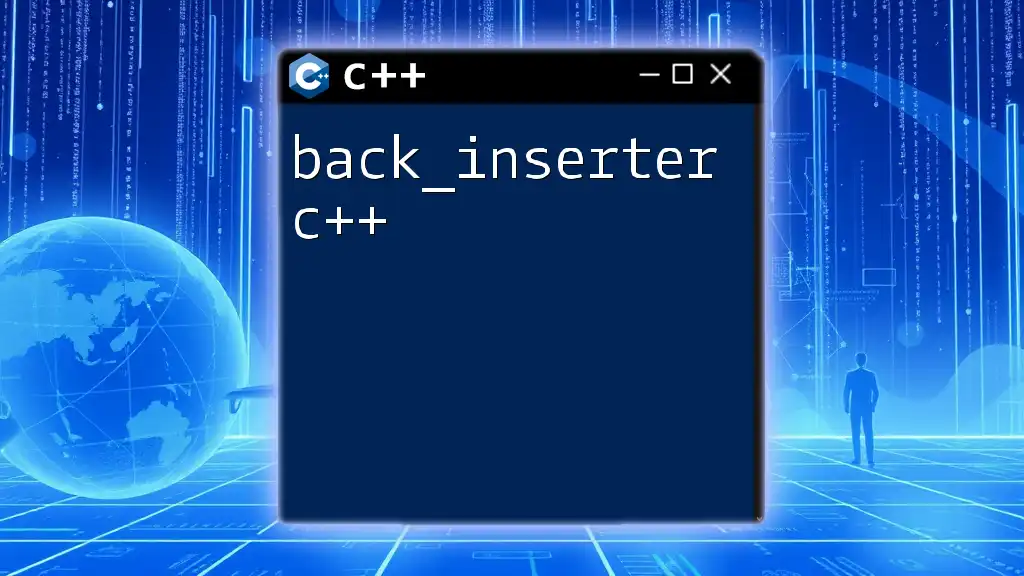
Learning Resources
Books and Online Courses
To enhance your hacking skills, consider investing your time in quality literature and online courses. Books like "Black Hat C Programming" and "The Art of Software Security Assessment" offer deep dives into advanced C++ nuances and security assessments.
Online platforms such as Coursera, Udacity, and Udemy host numerous courses aimed at cybersecurity and ethical hacking, often featuring C++ modules.
Communities and Forums
Joining active communities helps you share knowledge and learn from others. Websites like Stack Overflow or GitHub serve as excellent resources for assistance and collaboration. Engaging with like-minded individuals can also foster growth in your hacking capabilities.
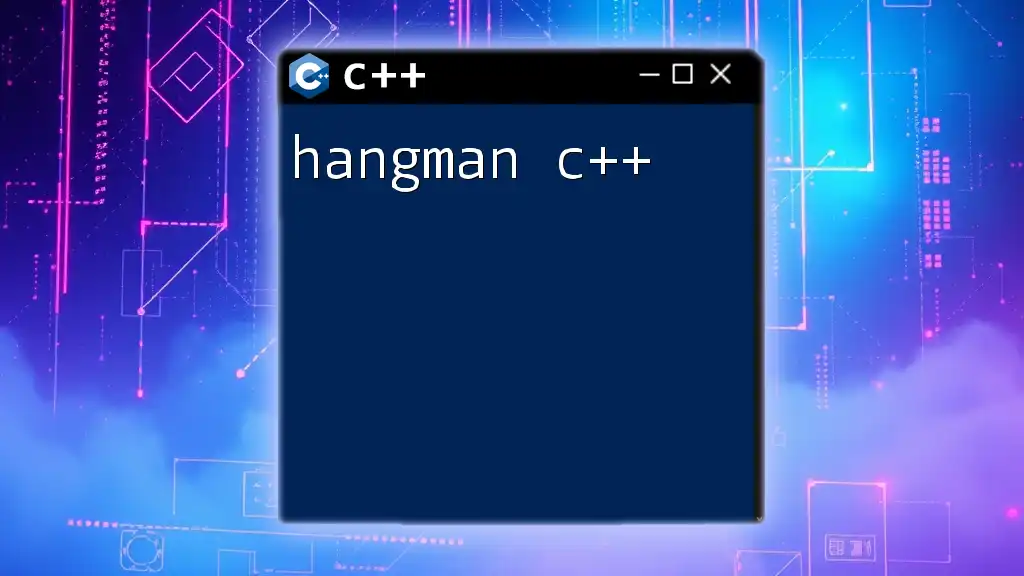
Ethical Hacking Certifications
Certification Overview
Certifications play a pivotal role in establishing credibility in cybersecurity. Certified Ethical Hacker (CEH) and CompTIA Security+ are reputable certifications that validate your skills and knowledge in hacking and security.
Preparing for the Certification Exam
Preparing for certification exams requires discipline and strategic planning. Focus on study materials, practice tests, and review courses that cover essential knowledge areas. Utilize online resources and diverse study groups to enhance your understanding significantly.
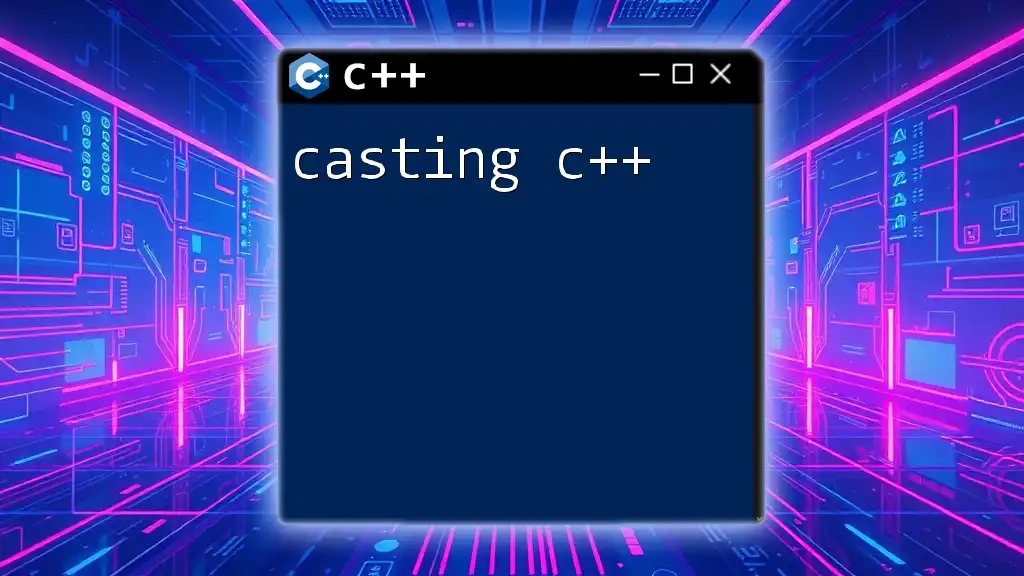
Conclusion
The Future of Hacking with C++
As technology advances, so does the landscape of hacking and cybersecurity. Hacking with C++ is an emerging field with boundless possibilities. Being adept in C++ not only equips you to challenge current technologies but also prepares you to tackle the future challenges of the cybersecurity world.
Call to Action
We encourage you to join a C++ and cybersecurity community, actively participate in discussions, and refine your coding skills. Enroll in training services to further your education in C++ and ethical hacking, empowering you to excel in this exciting field.