Problem solving with C++ involves using its rich set of features and libraries to efficiently develop algorithms that address various computational challenges.
Here’s a simple example that demonstrates how to find the maximum value in an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {3, 5, 1, 8, 7};
int maxVal = arr[0];
for (int i = 1; i < sizeof(arr)/sizeof(arr[0]); i++) {
if (arr[i] > maxVal) {
maxVal = arr[i];
}
}
cout << "Maximum value in the array is: " << maxVal << endl;
return 0;
}
Understanding Problem Solving
What is Problem Solving?
Problem solving in programming involves identifying a challenge and devising a methodical approach to overcome it. In the realm of problem solving with C++, the language’s robust features enable developers to efficiently tackle complex issues. C++ combines the power of object-oriented programming with procedural programming, allowing for flexible and scalable solutions.
Importance of Problem Solving Skills
Developing strong problem-solving skills is essential for programmers. These skills not only improve coding efficiency but also cultivate critical thinking. In various domains—be it in software development, game design, or data analysis—C++ provides a cornerstone for creating innovative solutions. Mastery in problem solving empowers programmers to translate concepts into operational code effortlessly.
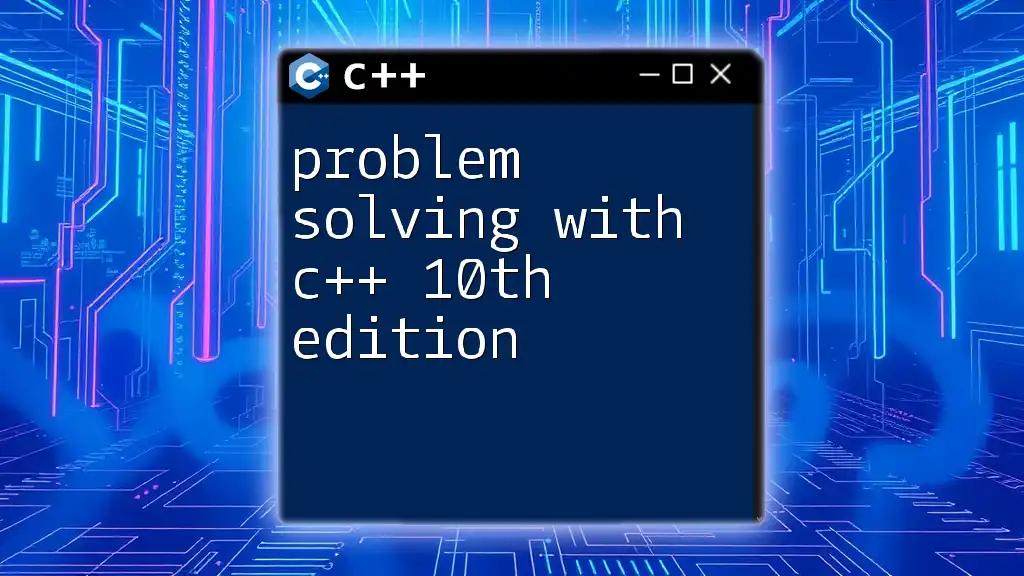
Steps to Effective Problem Solving in C++
Defining the Problem
Accurately defining the problem is the first step in effective problem solving. This involves a thorough analysis of what needs to be resolved. One popular technique for clarification is brainstorming. Take the time to write out the problem statement to provide a clear roadmap for the ensuing steps.
Algorithm Development
An algorithm is a step-by-step procedure designed to execute a task. Creating a clear and logical algorithm is crucial to solving any problem. Here’s a simple example of an algorithm to find the maximum among three numbers:
- Take input of three numbers, `a`, `b`, and `c`.
- Compare the numbers using conditional statements.
- Return the greatest number.
Converting this algorithm into C++ code can enhance understanding and clarity:
int maxOfThree(int a, int b, int c) {
return (a > b) ? (a > c ? a : c) : (b > c ? b : c);
}
Writing Pseudocode
Pseudocode provides a way to write an algorithm in plain English, enabling developers to focus on the logic without worrying about syntax. For the previous example, the pseudocode would look something like this:
FUNCTION maxOfThree(a, b, c)
IF a is greater than b AND a is greater than c THEN
RETURN a
ELSE IF b is greater than c THEN
RETURN b
ELSE
RETURN c
Translating Algorithms to C++
Next, learn to translate your well-defined algorithms and pseudocode into actual C++ code. Pay attention to the syntax and structure of C++. Proper indentation and formatting will make your code more readable for others and for yourself.

C++ Fundamentals for Problem Solving
Data Types and Variables
C++ provides a variety of data types including `int`, `float`, `double`, `char`, and `string`. Choosing the appropriate data type is essential because it affects memory usage and performance. For instance, if you’re counting whole numbers, you should use `int`, while for decimal numbers, `float` or `double` are more appropriate.
Control Structures
Control structures such as if statements, switch cases, and loops are vital for decision-making in C++. They allow for conditional operations and repeated executions. Here's a simple example of a `for loop` that prints numbers from 0 to 9:
for (int i = 0; i < 10; i++) {
std::cout << i << std::endl;
}
Functions and Modular Programming
Functions in C++ enable modular programming, allowing for code reusability and simplification. By defining functions, you limit complexity and can focus on smaller, manageable pieces of code. Functions also make it easier to test individual components of your program.
Arrays and Strings
Arrays allow for the storage of multiple values in a single variable, while strings let you handle textual data. Understanding how to manipulate these data structures can significantly streamline your problem-solving process. For example, consider the task of reversing a string:
#include <string>
#include <algorithm>
std::string reverseString(const std::string &str) {
std::string reversedStr = str;
std::reverse(reversedStr.begin(), reversedStr.end());
return reversedStr;
}
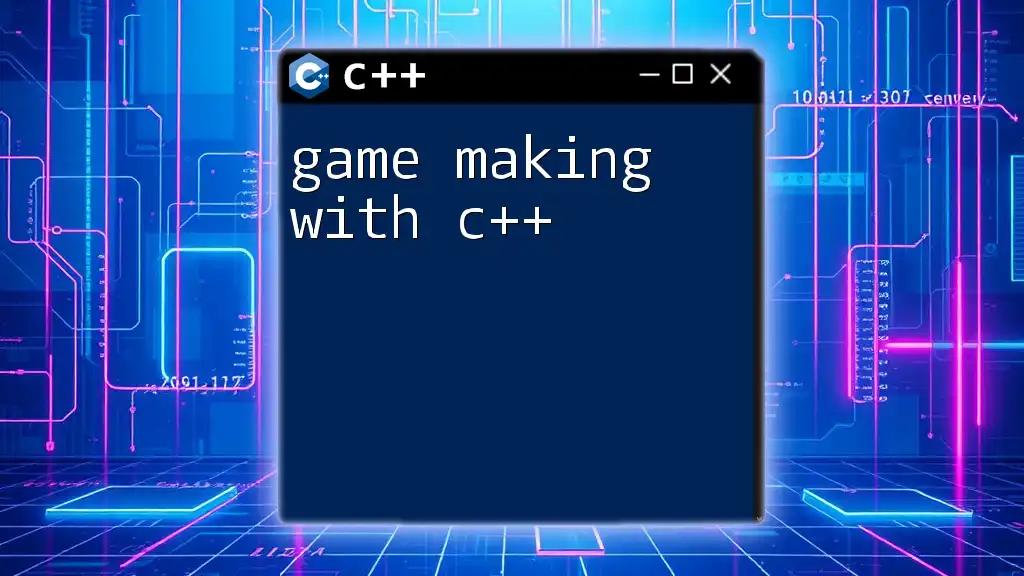
Common Problem-Solving Techniques in C++
Divide and Conquer
The divide and conquer strategy works by breaking down a problem into smaller sub-problems, solving them independently, and combining their solutions. One well-known application of this technique is the Merge Sort Algorithm. Here’s an illustrative C++ code snippet:
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
// Merging the sorted halves happens here
}
}
Dynamic Programming
Dynamic programming is an optimization technique that is particularly useful for solving problems that can be broken down into overlapping subproblems. A classic example is calculating the Fibonacci sequence:
int fibonacci(int n) {
int fib[n + 2]; // Extra space for the 0th case
fib[0] = 0;
fib[1] = 1;
for (int i = 2; i <= n; i++) {
fib[i] = fib[i - 1] + fib[i - 2];
}
return fib[n];
}
Backtracking
Backtracking is a powerful method used to solve problems incrementally. It involves making a series of choices and, if a choice leads to an invalid state, it steps back and tries alternative options. One well-known example of backtracking is solving the N-Queens Problem:
bool solveNQUtil(int board[N][N], int col) {
if (col >= N)
return true;
for (int i = 0; i < N; i++) {
if (isSafe(board, i, col)) {
board[i][col] = 1;
if (solveNQUtil(board, col + 1) == true)
return true;
board[i][col] = 0; // backtrack
}
}
return false;
}
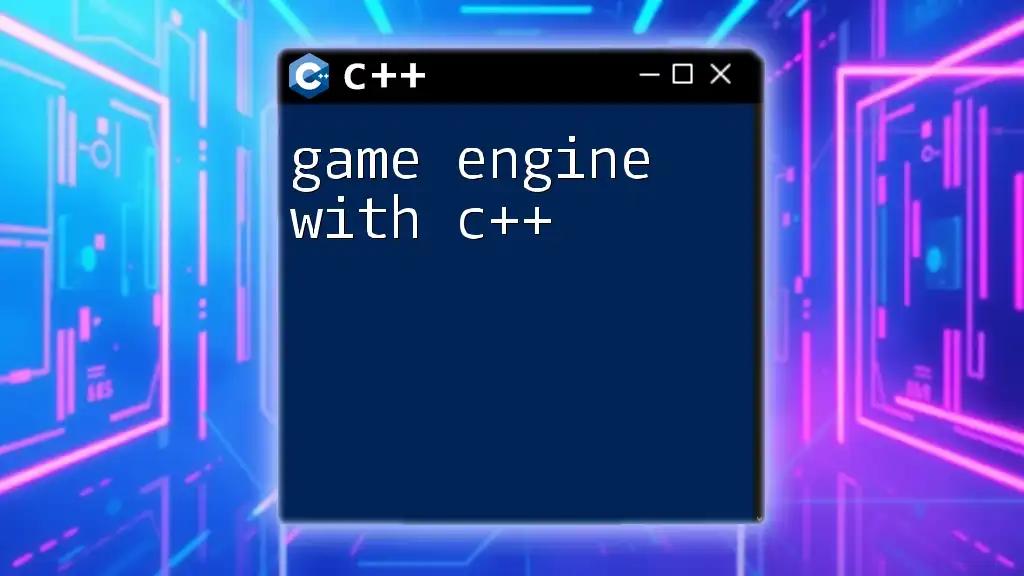
Testing and Debugging
Importance of Testing
Testing is a critical phase in the software development lifecycle. It allows you to validate that your code meets the requirements and behaves as expected. Common types of testing include unit tests, integration tests, and system tests. When done correctly, testing helps in identifying bugs and improving code quality.
Common Debugging Techniques
Effective debugging requires a systematic approach. Utilize tools like `gdb` for C++ to track down issues in your code. Here’s a simple gdb command sequence to help get you started:
- Compile with debugging information: `g++ -g your_program.cpp -o your_program`
- Start gdb: `gdb ./your_program`
- Set breakpoints: `break main`
- Run the program: `run`

Conclusion
Mastering problem solving with C++ is an enriching journey that vastly enhances your programming skills. By following the outlined steps and understanding the foundational elements, you’ll develop a robust problem-solving mindset. Remember, practice is key, and continual learning will equip you with the necessary tools to face programming challenges with confidence.
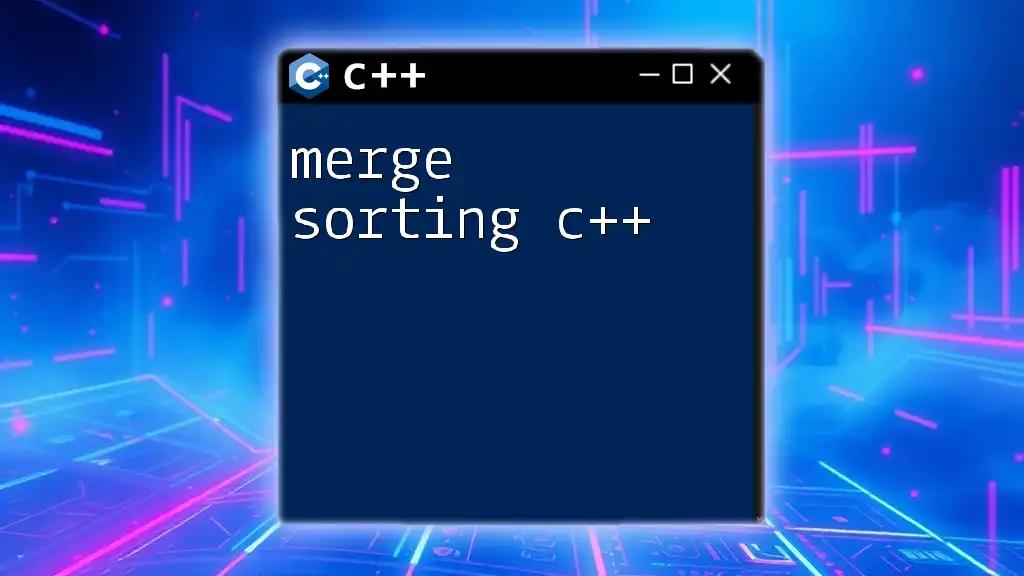
Additional Resources
Consider exploring recommended books, online courses, and vibrant programming communities to further enhance your learning. Websites like LeetCode and HackerRank offer an excellent platform for practicing and honing your skills.
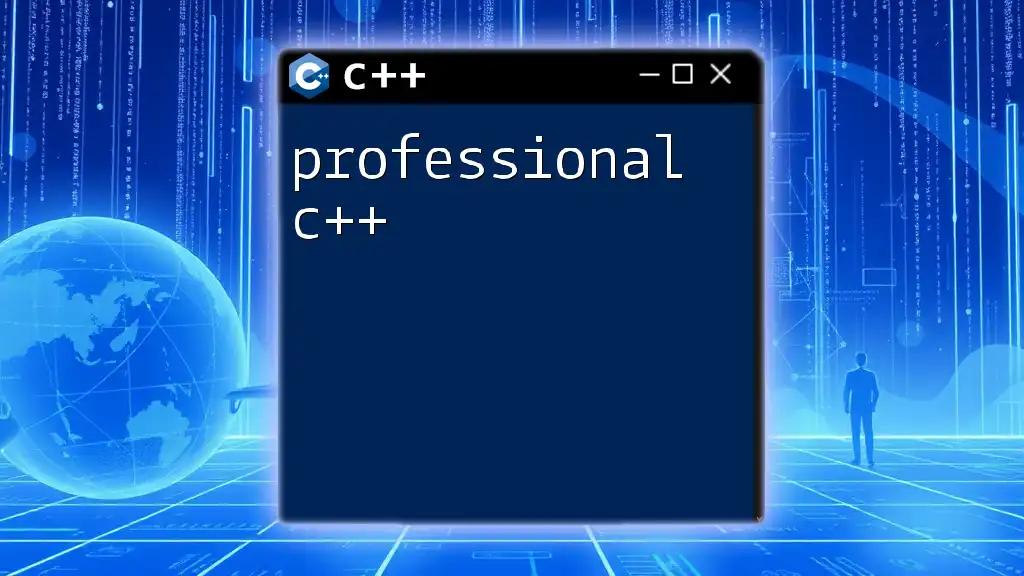
Call to Action
We encourage you to dive into C++ problem-solving exercises and share your insights and challenges in the comments below. Your journey in mastering C++ could inspire others in the community!