Data abstraction in C++ allows programmers to simplify complex problems by using classes and objects to hide unnecessary details, similar to how walls and mirrors can reflect and obscure our surroundings.
#include <iostream>
#include <string>
class Mirror {
public:
void reflect(const std::string& message) {
std::cout << "Reflection: " << message << std::endl;
}
};
class Wall {
private:
Mirror mirror;
public:
void showReflection(const std::string& message) {
mirror.reflect(message);
}
};
int main() {
Wall wall;
wall.showReflection("Hello, World!");
return 0;
}
Understanding Data Abstraction
What is Data Abstraction?
Data abstraction is a fundamental concept in programming that involves simplifying complex realities by modeling classes based on the essential properties and behaviors relevant to a particular problem. In C++, data abstraction enables developers to focus on defining the "what" and "how" of data structures without needing to delve into the intricate details of how these operations are implemented. By abstracting data, you create a clear interface that hides unnecessary complexities, thus allowing for cleaner and more maintainable code.
Key Concepts of Data Abstraction
Classes and Objects: At the core of C++ lies the idea of classes and objects. A class serves as a blueprint for creating objects, encapsulating data for the object and methods to manipulate that data. This separation of data and functionality forms the basis for data abstraction.
Encapsulation: One of the cornerstones of data abstraction is encapsulation. It protects the data within a class from unauthorized access and modification. By providing a controlled interface—public interfaces, both methods and properties—you maintain the integrity of the object.
Interface vs. Implementation: It’s important to differentiate between the interface (what the class does) and its implementation (how it performs its tasks). A well-designed class offers a clear interface, allowing users to interact with complex functionality without needing to understand its workings.
Example of Data Abstraction in C++
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
In this example, the `Shape` class defines an interface for all shapes without defining how they are drawn. This level of abstraction allows for the creation of other classes that implement the `draw` function in various ways, such as `Circle`, `Square`, or `Triangle`, each fulfilling the contract established by the `Shape` class.
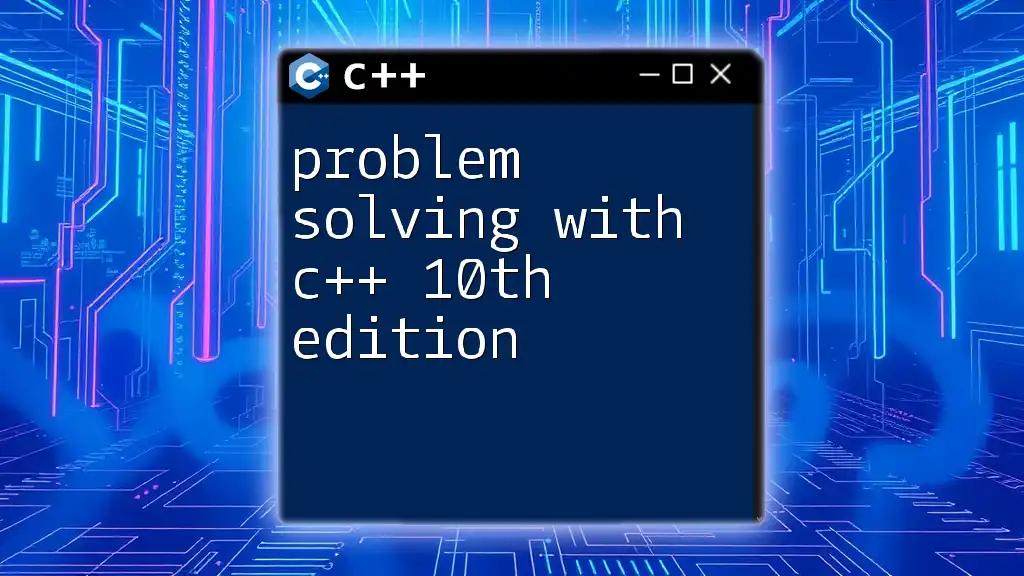
Walls and Mirrors: Concept Explained
What are Walls and Mirrors?
The walls and mirrors concept serves as a powerful metaphor for problem-solving in programming. Walls represent constraints—boundaries that define the limits of the problem. Mirrors, on the other hand, assist in reflecting on existing solutions, helping programmers adapt and reuse code effectively. Understanding and utilizing these concepts in C++ can significantly enhance your problem-solving approach.
Applying the Walls and Mirrors Strategy
Walls: When faced with a programming challenge, identifying the boundaries of the problem is critical. These walls can take various forms, such as performance constraints, memory limits, or functional requirements. By recognizing these constraints, you can better navigate your solution space.
Mirrors: Reflecting on existing solutions is equally crucial. Sometimes, when encountering a new problem, a similar one may have already been solved. Utilizing previous knowledge, code, or libraries allows you to develop solutions more quickly and without reinventing the wheel.
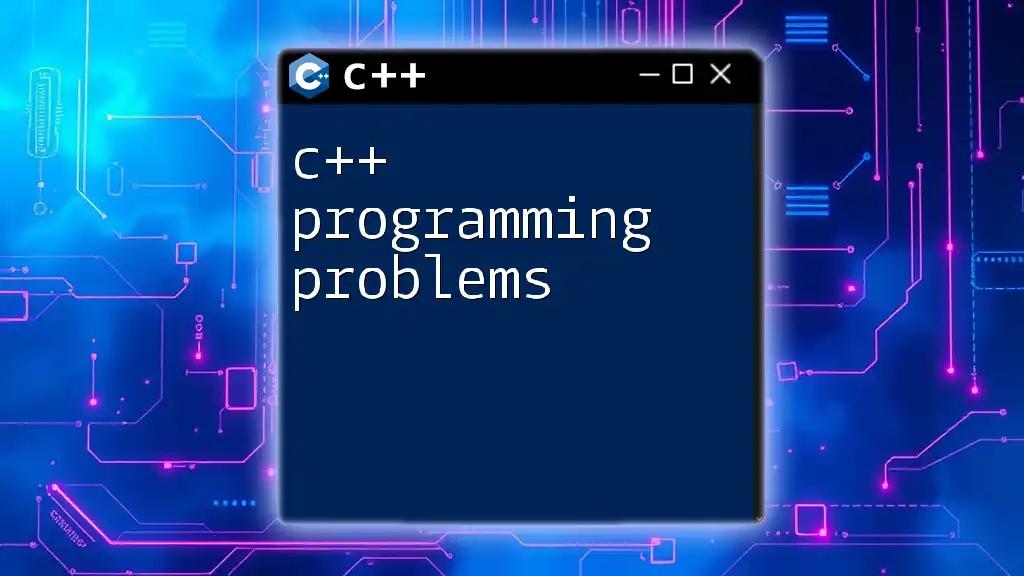
Problem Solving in C++ through Data Abstraction
Analyzing a Problem: Recognizing Boundaries (Walls)
To effectively analyze a problem using C++, start by defining its scope. Ask critical questions to identify key constraints:
- What is the expected input and output?
- Are there performance limitations I need to consider?
- What functionalities must be included and what can be excluded?
By setting these boundaries, you create a structured environment that simplifies the problem-solving process, making it easier to construct your solution.
Creating Solutions: Using Mirrors to Reflect
Once the problem boundaries are established, the next step is to leverage existing solutions and concepts:
- Review similar problems you have tackled before.
- Determine if any existing classes or libraries can be adapted for your current needs.
By reflecting on these assets, you minimize duplication of effort and build solutions based on solid foundations.

Practical Example: Building a Simple C++ Application
Problem Statement
Imagine we want to create a simple application for drawing various geometric shapes based on user input.
Step-by-Step Solution
Step 1: Define the Problem
The problem involves creating multiple shapes that draw themselves when instructed. The walls of our problem are defined by:
- Each shape should encapsulate its attributes (e.g., width, height).
- Shapes must provide a way to draw themselves.
Step 2: Data Abstraction Approach
You need to design an abstract base class that defines the `draw` behavior for all shapes:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
Next, create derived classes that implement this interface:
class Rectangle : public Shape {
private:
int width, height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
void draw() override {
// Logic for drawing rectangle
std::cout << "Drawing Rectangle of width " << width << " and height " << height << std::endl;
}
};
class Circle : public Shape {
private:
int radius;
public:
Circle(int r) : radius(r) {}
void draw() override {
// Logic for drawing circle
std::cout << "Drawing Circle of radius " << radius << std::endl;
}
};
In this code, classes `Rectangle` and `Circle` define specific implementations of the abstract `draw` method, demonstrating how abstraction allows for diverse functionalities under a unified interface.
Step 3: Implementing the Solution
With these classes defined, you can create a function to manage shape drawing:
void drawShape(Shape* shape) {
shape->draw(); // Call the draw method
}
Testing the Application
Proper testing ensures that the implementation is working as intended. You might write simple tests such as:
Shape* rect = new Rectangle(5, 10);
Shape* circ = new Circle(7);
drawShape(rect);
drawShape(circ);
These tests validate that the shapes are being drawn correctly according to their definitions.
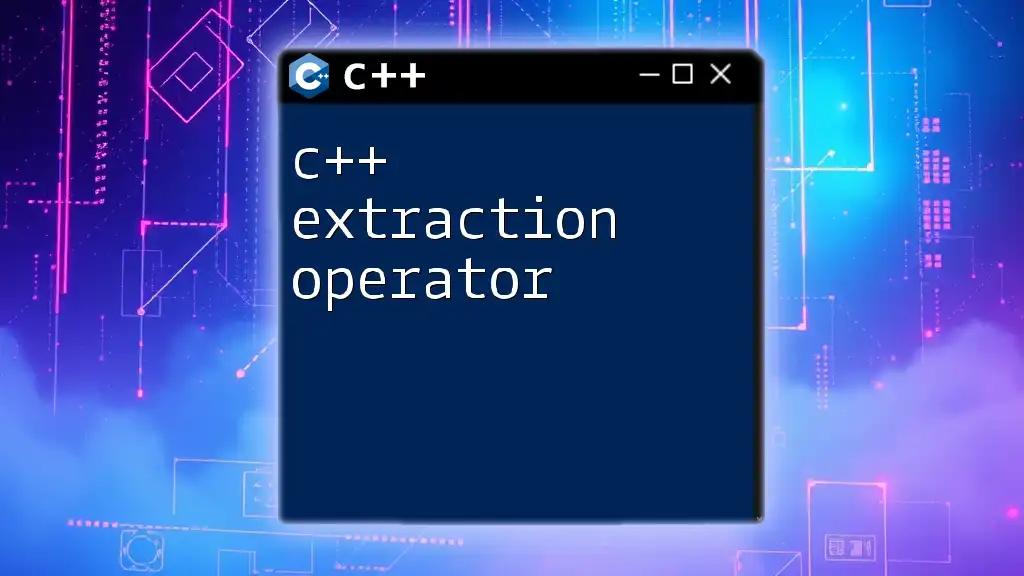
Enhancing Problem-Solving Skills with Practice
Exercises to Improve Your Skills
To sharpen your problem-solving skills, consider practicing the following exercises:
- Design and implement a new shape class, such as a Triangle or Hexagon.
- Explore C++ libraries such as STL or Boost to find reusable components.
- Analyze different problems, identifying walls and exploring mirroring strategies in your solutions.
Additional Resources
To further enhance your understanding of data abstraction and problem-solving in C++, consider exploring the following resources:
- Books: "Effective C++" by Scott Meyers, "The C++ Programming Language" by Bjarne Stroustrup.
- Online Courses: Platforms like Coursera, Udacity, or edX offering comprehensive C++ programming courses.

Conclusion
Data abstraction in C++ is a critical concept that empowers developers to build sophisticated applications while simplifying complexity. By adopting the walls and mirrors strategy, programmers can enhance their problem-solving capabilities, allowing them to navigate constraints and adapt existing solutions effectively. Embrace these principles in your C++ journey, and you’ll find that programming becomes not just a task but an enjoyable challenge filled with endless opportunities.