In C++, data structures such as arrays, vectors, and classes allow for the efficient organization and manipulation of data, enabling developers to create more complex and functional applications.
Here's a simple example using a vector in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Create a vector of integers
for (int number : numbers) { // Iterate through the vector
std::cout << number << " "; // Print each number
}
return 0;
}
Understanding Data Structures
What Are Data Structures?
Data structures are fundamental constructs in programming that dictate how data is stored, organized, and accessed. They allow for efficient data management and are essential for creating efficient algorithms. Understanding data structures is crucial for optimizing code performance and enhancing the overall functionality of software applications.
Types of Data Structures
Data structures can be broadly categorized into two types: linear and non-linear. Linear data structures maintain a sequential arrangement of elements, while non-linear structures allow for more complex relationships among data points.
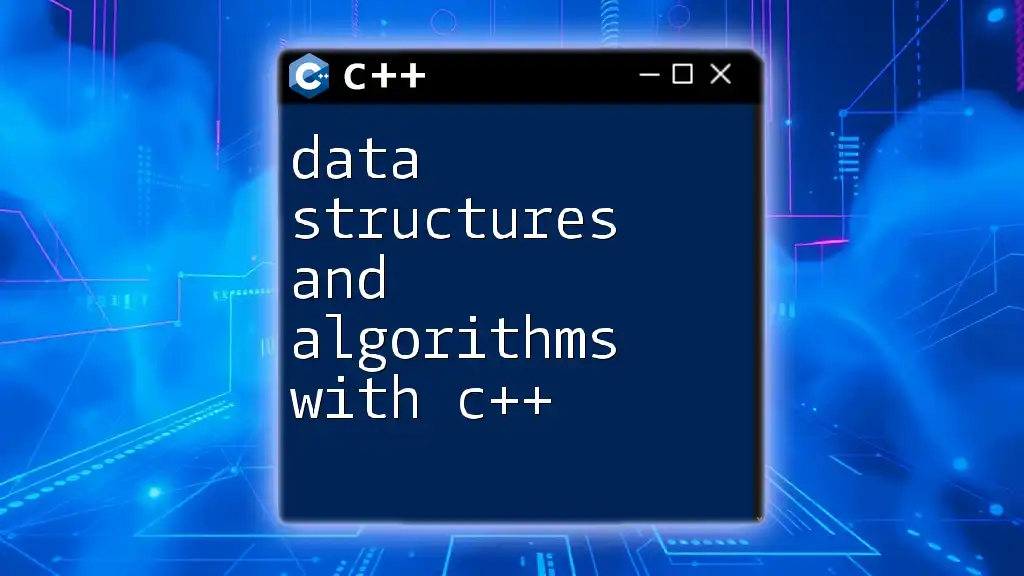
Linear Data Structures
Arrays
Arrays are a collection of elements identified by index or key. They are particularly useful for situations where you need to store multiple items of the same type.
Example:
int arr[5] = {1, 2, 3, 4, 5};
You can access and modify elements by their index:
arr[0] = 10; // Changing the first element to 10.
Keep in mind that arrays have a fixed size, which limits their flexibility in certain applications.
Linked Lists
Singly Linked List
A singly linked list is a dynamic data structure that consists of nodes, where each node contains data and a pointer to the next node.
Code Snippet:
struct Node {
int data;
Node* next;
};
To create and traverse a singly linked list, you can implement functions to add, delete, and display nodes, which allows for more dynamic memory management compared to arrays.
Doubly Linked List
In a doubly linked list, each node contains two pointers—one pointing to the next node and another pointing to the previous node. This allows for bidirectional traversal.
Adding and deleting nodes is more efficient in a doubly linked list, especially when working with large datasets.
Stacks
Stacks are Last-In-First-Out (LIFO) data structures, meaning the last element added will be the first one to be removed. They are useful in scenarios like evaluating expressions or managing function calls in programming.
Example:
#include <stack>
stack<int> myStack;
myStack.push(1); // Pushes 1 onto the stack.
myStack.pop(); // Removes the top element from the stack.
Queues
Queues are First-In-First-Out (FIFO) structures. They are ideal for scenarios such as task scheduling and buffering data.
Example:
#include <queue>
queue<int> myQueue;
myQueue.push(1); // Adds 1 to the back of the queue.
myQueue.pop(); // Removes the front element from the queue.
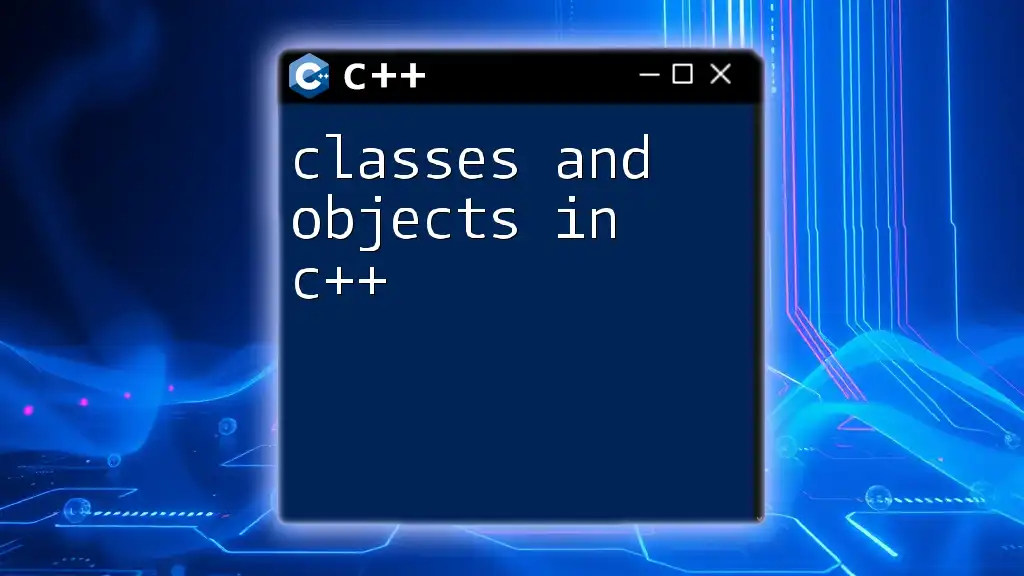
Non-linear Data Structures
Trees
Binary Trees
Binary trees are structures where each node has at most two children, referred to as the left and right child. They are widely used in applications like search algorithms and hierarchical data representation.
Example:
struct TreeNode {
int value;
TreeNode* left;
TreeNode* right;
};
You can traverse a binary tree using different methods, such as pre-order, in-order, or post-order traversal, which each serve different purposes based on your needs.
Binary Search Trees (BST)
Binary search trees enhance functionality by maintaining order—specifically, for any given node, the left subtree contains nodes with lesser values, and the right subtree contains nodes with greater values. This makes searching, inserting, and deleting nodes efficient.
Example:
- Adding elements to a BST can be done using a recursive function that respects the ordering principle of trees.
Heaps
Heaps are complete binary trees that satisfy the heap property. In a min-heap, for instance, the key at each node is less than or equal to the keys of its children. Heaps are commonly used in implementing priority queues.
Code Snippet:
#include <queue>
priority_queue<int> maxHeap; // Max-heap implementation
These structures facilitate quick access to the highest or lowest values, which is particularly advantageous in numerous algorithms.
Graphs
Graphs consist of a set of vertices connected by edges. They are versatile structures used to model relationships and networks, such as social connections and traffic systems.
Graphs can be represented using two primary methods: an adjacency list (more space-efficient for sparse graphs) or an adjacency matrix (better for dense graphs).
Example: Using an adjacency list:
vector<vector<int>> adjList(n); // where n is the number of vertices
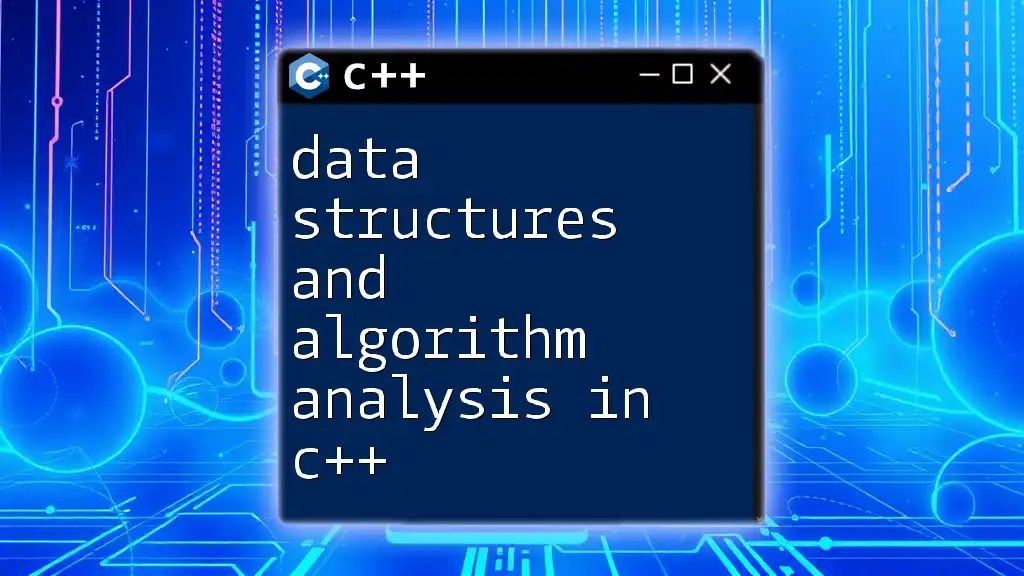
Other Important C++ Objects
Classes and Objects
C++ is an object-oriented programming language, meaning it allows the creation of classes that encapsulate data and functionalities together. This structure supports data abstraction and helps to manage complex problems more effectively.
Example:
class Box {
public:
int length;
int width;
int height;
int volume() {
return length * width * height;
}
};
By defining classes, you can create multiple instances (objects) with attributes and behaviors, further enhancing data structures into meaningful, real-world representations.
Templates
Templates enable the creation of generic data structures that can work with any data type. This increases the flexibility of your code and allows for reusability.
Example: Generic Stack Implementation:
template <typename T>
class MyStack {
private:
vector<T> elements;
public:
void push(T element) {
elements.push_back(element);
}
T pop() {
T elem = elements.back();
elements.pop_back();
return elem;
}
};
Smart Pointers
Smart pointers help manage dynamic memory in C++, ensuring that memory leaks do not occur when using data structures. They automatically handle memory deallocation when an object goes out of scope, promoting safer programming practices.
Example:
#include <memory>
std::unique_ptr<Node> nodePtr(new Node());
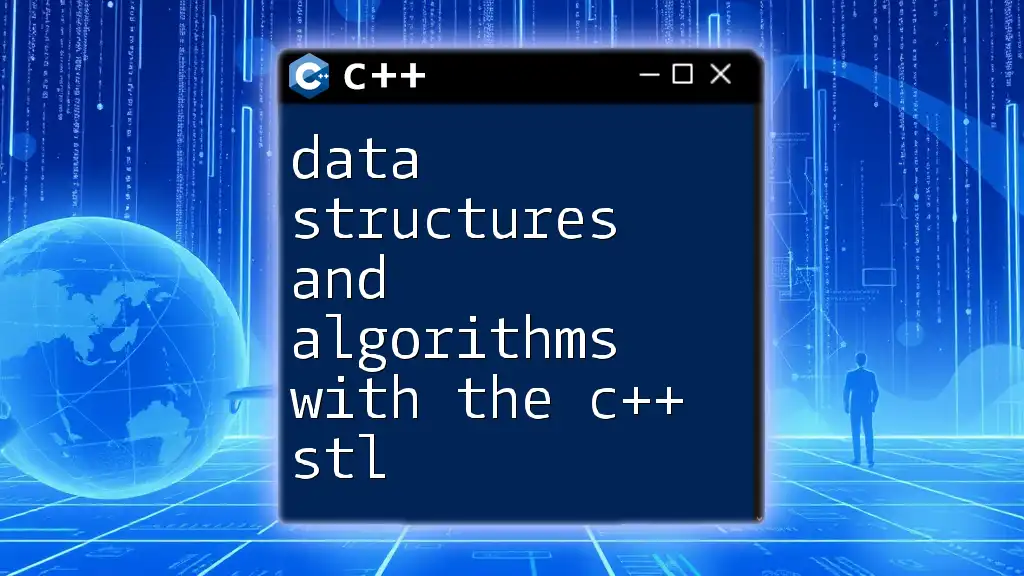
Conclusion
In summary, understanding data structures and other objects using C++ is integral for any programmer aiming to develop efficient and robust applications. By leveraging arrays, linked lists, trees, graphs, and other C++ constructs, you can enhance your programming skills significantly. As you practice with these data structures, remember to experiment, as hands-on experience is invaluable for mastering these concepts. Embrace these foundational elements, and continually seek to implement them in your projects for better organization and management of your data.