In C++, classes serve as blueprints for creating objects, encapsulating data and functions that operate on that data within a single unit.
class Car {
public:
string brand;
int year;
void display() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2020;
myCar.display();
return 0;
}
What is a Class in C++?
Definition of a Class
A class in C++ serves as a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data. The key features of classes include:
- Encapsulation: Binding data and methods into a single unit.
- Abstraction: Hiding complex implementations while exposing simple interfaces.
- Inheritance: Enabling new classes to derive properties and behaviors from existing classes.
- Polymorphism: Allowing methods to do different things based on the object they are acting upon.
Syntax of a Class
The syntax for defining a class in C++ is straightforward. Here's how you can structure it:
class ClassName {
// Access specifiers
public:
// Member variables and methods
};
Access specifiers play a vital role in defining the visibility of class members. You can declare members as public, private, or protected:
- Public members can be accessed from outside the class.
- Private members can only be accessed by methods within the class.
- Protected members can be accessed in derived classes.
Example of a Simple Class
Consider the following class definition for a `Car`:
class Car {
public:
string brand;
string model;
int year;
void display() {
cout << brand << " " << model << " " << year << endl;
}
};
In this example, the `Car` class contains three public member variables (`brand`, `model`, and `year`) and a public method `display()`. This method will print the car's details, showcasing how functions can be encapsulated within a class.
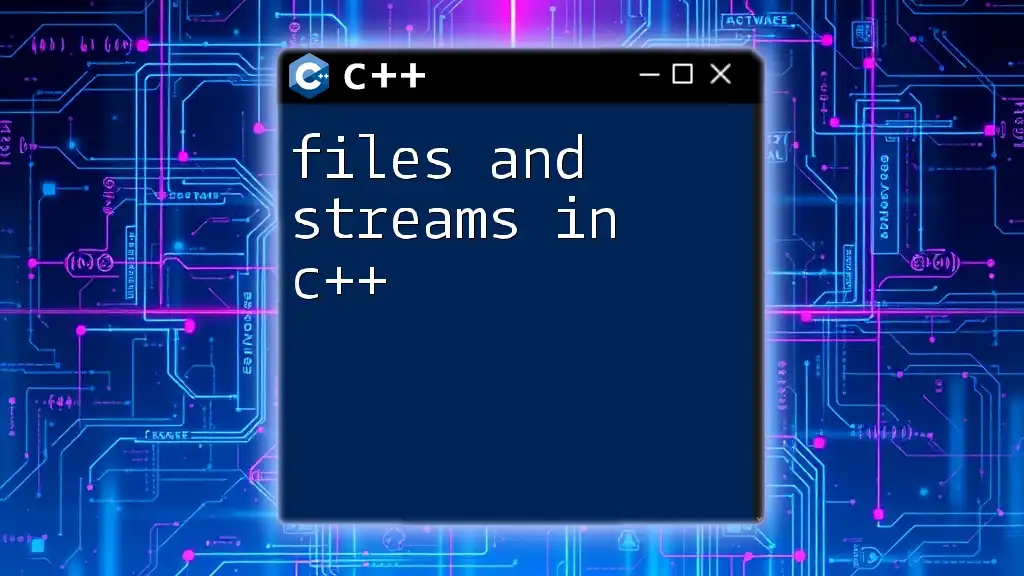
What is an Object in C++?
Definition of an Object
An object in C++ is simply an instance of a class. Objects are essential in object-oriented programming as they represent real-world entities that encapsulate both data and behavior. Understanding objects is fundamental when working with classes and objects in C++.
Creating Objects
To create an object from a class, you need to simply declare it like so:
Car myCar; // Creating an object of Car
In this example, `myCar` is an instance of the `Car` class.
Example of Object Creation and Initialization
You can initialize the properties of your `myCar` object as follows:
Car myCar;
myCar.brand = "Toyota";
myCar.model = "Corolla";
myCar.year = 2020;
myCar.display(); // Output: Toyota Corolla 2020
By accessing the member variables, you can set values specific to that object. The `display()` method allows you to see the properties of `myCar`.
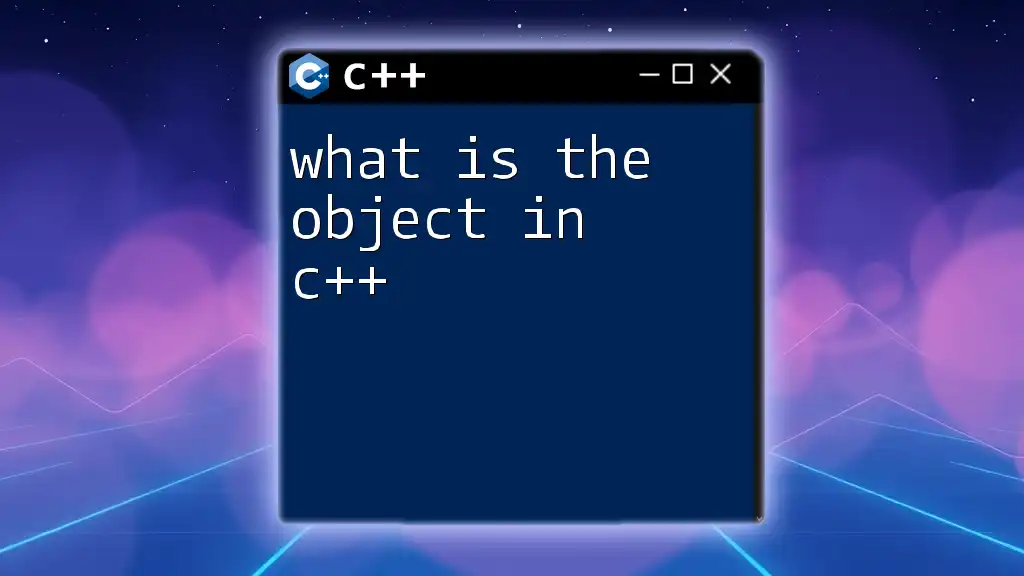
The Relationship Between Classes and Objects in C++
Classes as Blueprints
Classes serve as blueprints from which objects are created. Every object instantiated from a class will have its own set of member variables defined by that class. If the class definition changes (say, adding a new member variable), all subsequent objects created from that class will include this new variable.
Objects as Instances of Classes
One of the core aspects of classes and objects in C++ is that you can create multiple objects from a single class. Each object can hold different values, showcasing their individuality.
Car car1;
Car car2;
car1.brand = "Honda";
car2.brand = "Ford";
In this example, `car1` and `car2` are two independent objects of the `Car` class, each possessing their own set of data.
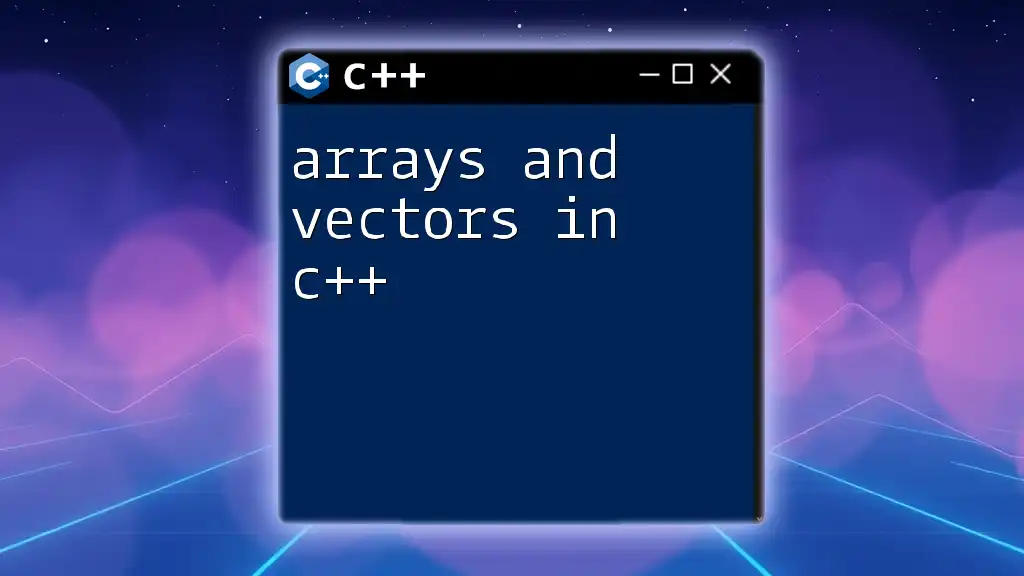
Class Members: Variables and Methods
Member Variables
Member variables are the attributes of a class. They hold the state of the object. Here’s how you can structure member variables in a simple `Student` class:
class Student {
public:
string name;
int age;
};
Each instance of `Student` will have its own `name` and `age`, demonstrating individual states controlled by the class structure.
Member Functions
Member functions define the behavior of the class. They can manipulate member variables and can be invoked through an object. Consider the following example of a `Calculator` class:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
This simple example shows a method `add()` that takes two integers and returns their sum. You could invoke this method through an object of the Calculator class, which showcases how class functionality can be encapsulated.
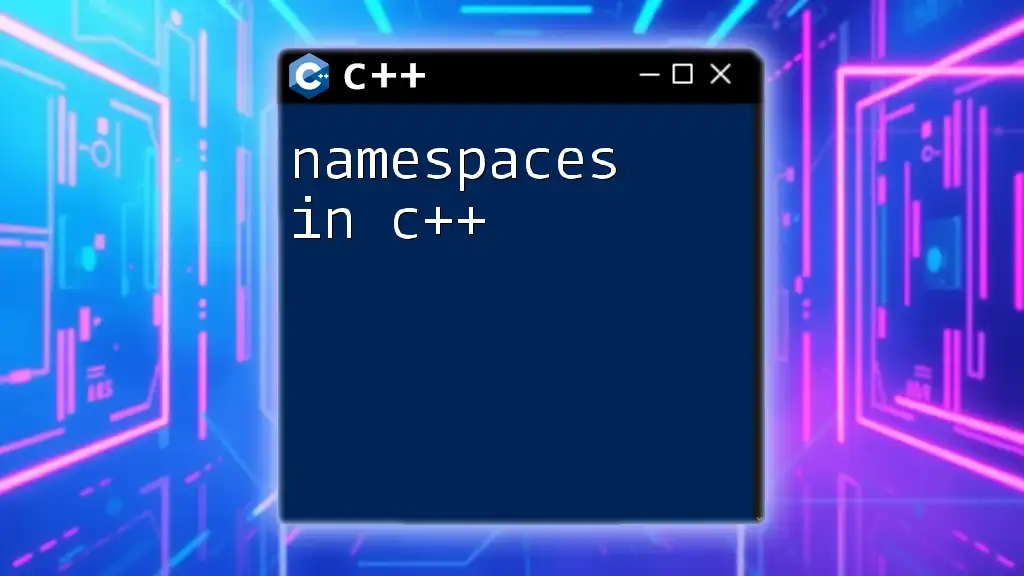
Access Modifiers in C++
Understanding Access Control
Access modifiers control the visibility of class members. Using access controls effectively is crucial in maintaining data integrity, allowing for encapsulation to protect an object's internal state.
Examples of Access Modifiers
You can declare public and private members to illustrate their differences:
class Person {
public:
string name; // Public
};
In this example, the `name` variable is public, meaning it can be accessed outside the class.
For a more restricted access, consider a `BankAccount` class:
class BankAccount {
private:
double balance; // Private
public:
void deposit(double amount) {
balance += amount; // Accessing private member
}
};
In this code snippet, `balance` is private and can only be manipulated through the public `deposit` method, providing a clear example of encapsulation.
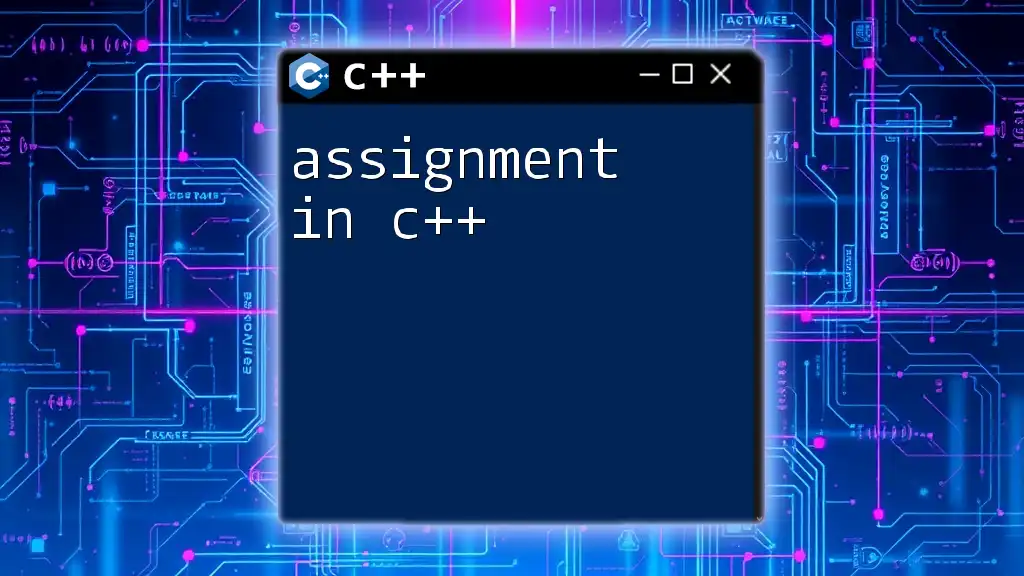
Constructors and Destructors in C++
Introduction to Constructors
A constructor is a special member function that is automatically called when an object of the class is created. Its primary purpose is to initialize objects with default values or with data provided by the user.
class Book {
public:
string title;
Book(string t) { title = t; } // Constructor
};
Here, the `Book` class has a constructor that initializes the `title` member.
Understanding Destructors
Conversely, a destructor is invoked when an object is destroyed, allowing for cleanup tasks, like deallocating resources.
class Demo {
public:
~Demo() { // Destructor
// Clean up resources
}
};
Destructors are essential in managing dynamic memory, ensuring that allocated memory is properly released to avoid leaks.
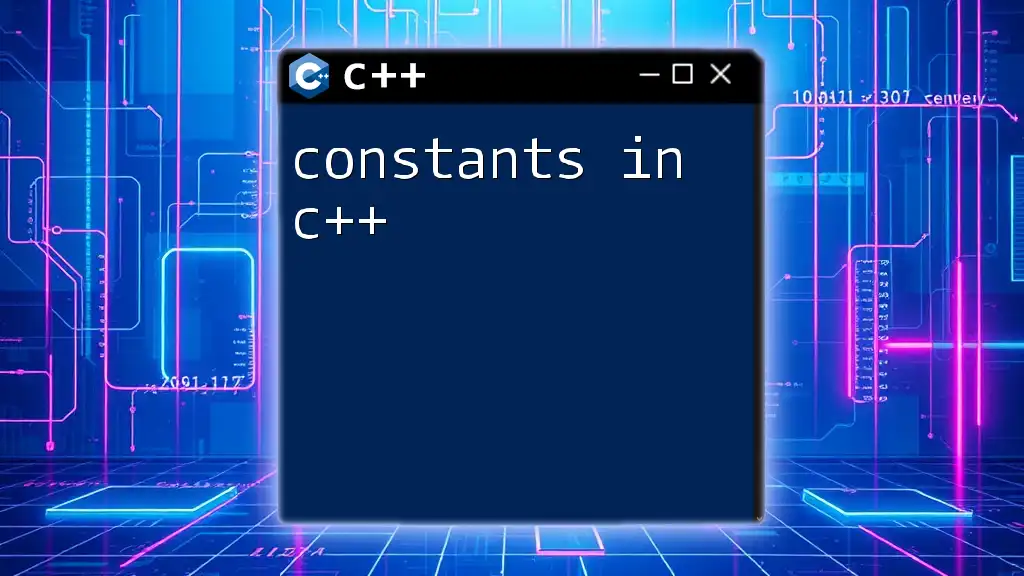
Conclusion
Understanding classes and objects in C++ is fundamental to mastering object-oriented programming. Classes act as blueprints, while objects are instances that hold data defined by these blueprints. Embracing the concepts of member variables, methods, access modifiers, constructors, and destructors will not only enhance your programming skills but also pave the way for more complex applications. As you practice creating and manipulating classes and objects in C++, your proficiency will grow, enabling you to construct powerful and efficient code.
Now is the time to dive into your projects and bring your classes and objects in C++ to life!
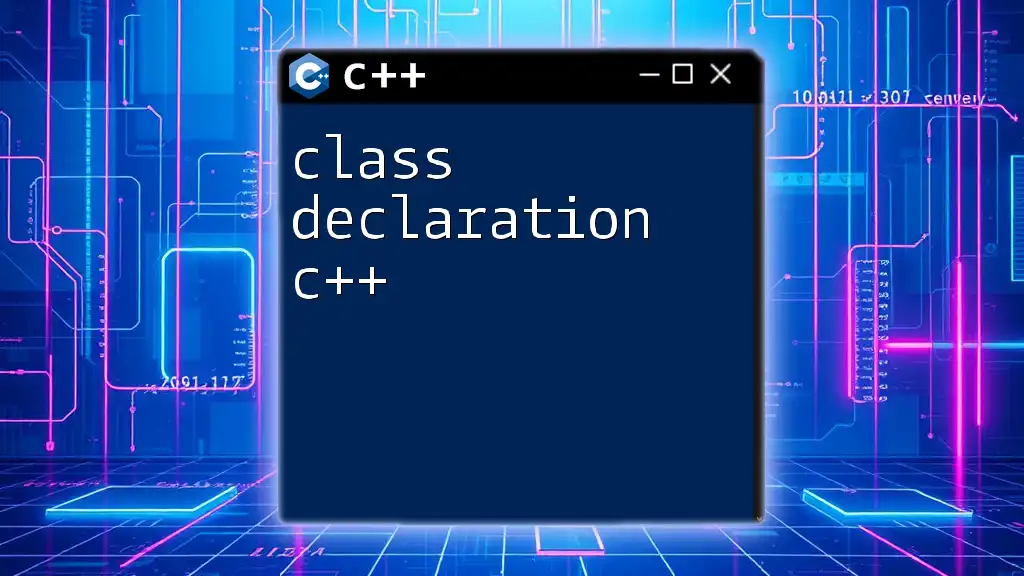
Additional Resources
- Official C++ documentation
- Recommended books and online courses on C++
- Suggested further reading about object-oriented programming concepts