To create an object in C++, you first need to define a class and then instantiate it using the class name followed by the object name and an optional constructor call.
class MyClass {
public:
MyClass() { /* constructor code */ }
};
MyClass myObject; // Creating an object of MyClass
Understanding C++ Classes and Objects
What is a Class?
In C++, a class serves as a blueprint for creating objects. It encapsulates data for the object and methods to manipulate that data, thereby supporting the principle of encapsulation. Through classes, you can model real-world entities, making it easier to manage and interact with complex data.
Creating a Simple Class
For example, let's create a simple class to represent a `Car`.
class Car {
public:
std::string model;
int year;
void displayInfo() {
std::cout << "Model: " << model << ", Year: " << year << std::endl;
}
};
Explanation of Class Members
In the code above:
- `model` and `year` are class members or attributes that hold specific information about a car.
- The method `displayInfo()` prints the attributes of the car object to the console.
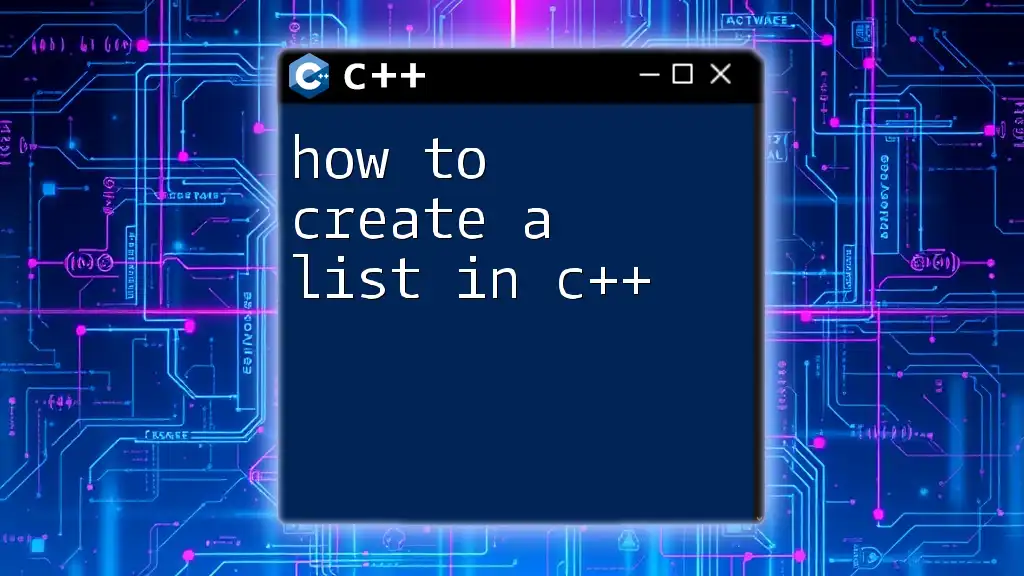
How to Create an Object in C++
Syntax of Object Creation
When it comes to learning how to create an object in C++, the syntax is straightforward. C++ allows you to create objects in two main ways: stack allocation and heap allocation.
Creating an Object Using Stack Allocation
Definition and Benefits
Stack allocation allows you to instantiate an object automatically when it goes out of scope. This is advantageous due to automatic memory management, ensuring that memory is freed up without needing explicit deallocation.
Example of Stack Allocation
int main() {
Car myCar; // Creating an object
myCar.model = "Toyota";
myCar.year = 2020;
myCar.displayInfo(); // Output: Model: Toyota, Year: 2020
return 0;
}
Explanation of the Example
In this example, `myCar` is created on the stack. When `main()` finishes its execution, the object is automatically destroyed, and memory is reclaimed. Thus, you don’t have to worry about memory leaks in this case.
Creating an Object Using Heap Allocation
Definition and Benefits
In contrast, heap allocation involves using dynamic memory, providing flexibility for managing the object’s lifetime. You will need to explicitly allocate and deallocate memory when using this method.
Example of Heap Allocation
int main() {
Car* myCar = new Car(); // Creating an object on heap
myCar->model = "Honda";
myCar->year = 2022;
myCar->displayInfo(); // Output: Model: Honda, Year: 2022
delete myCar; // Freeing memory at the end
return 0;
}
Explanation of the Example
Here, `myCar` is allocated on the heap using the `new` keyword. The arrow (`->`) operator is used to access the object's members. It's important to remember to deallocate memory with `delete` to prevent memory leaks, ensuring efficient memory use.
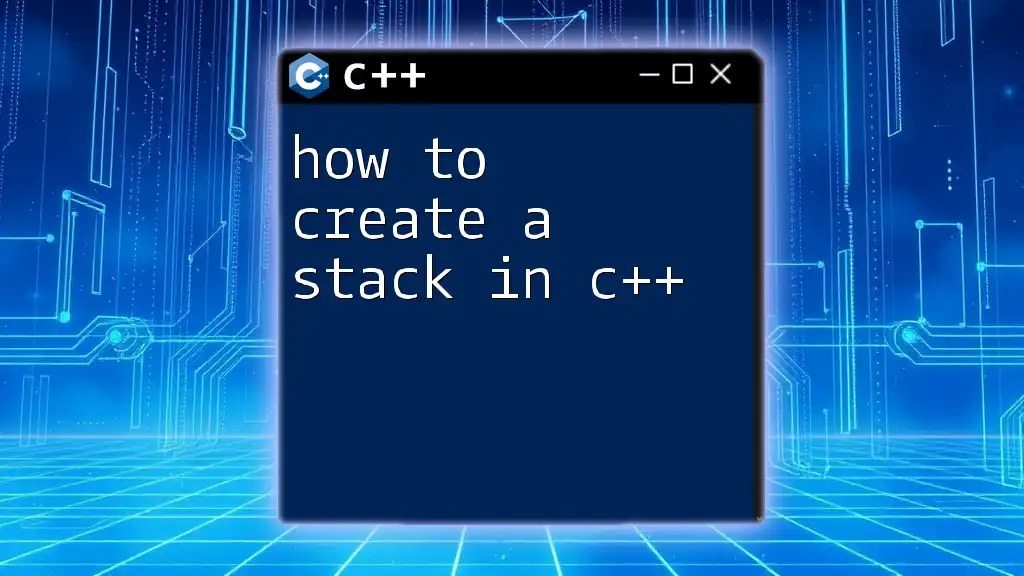
Working with Multiple Objects
Creating Multiple Objects
Creating multiple objects from the same class is common and easy to accomplish. This technique can be useful when modeling a collection of similar items, like a fleet of cars.
Example of Multiple Objects
int main() {
Car car1; // Stack allocation for car1
car1.model = "Ford";
car1.year = 2019;
Car* car2 = new Car(); // Heap allocation for car2
car2->model = "Tesla";
car2->year = 2021;
car1.displayInfo(); // Output: Model: Ford, Year: 2019
car2->displayInfo(); // Output: Model: Tesla, Year: 2021
delete car2; // Clean up heap memory
return 0;
}
Discussion on Object States
In this example, `car1` is allocated on the stack, while `car2` is allocated on the heap. Both objects maintain separate states, allowing them to function independently. This demonstrates how multiple instances of a class can encapsulate unique data.
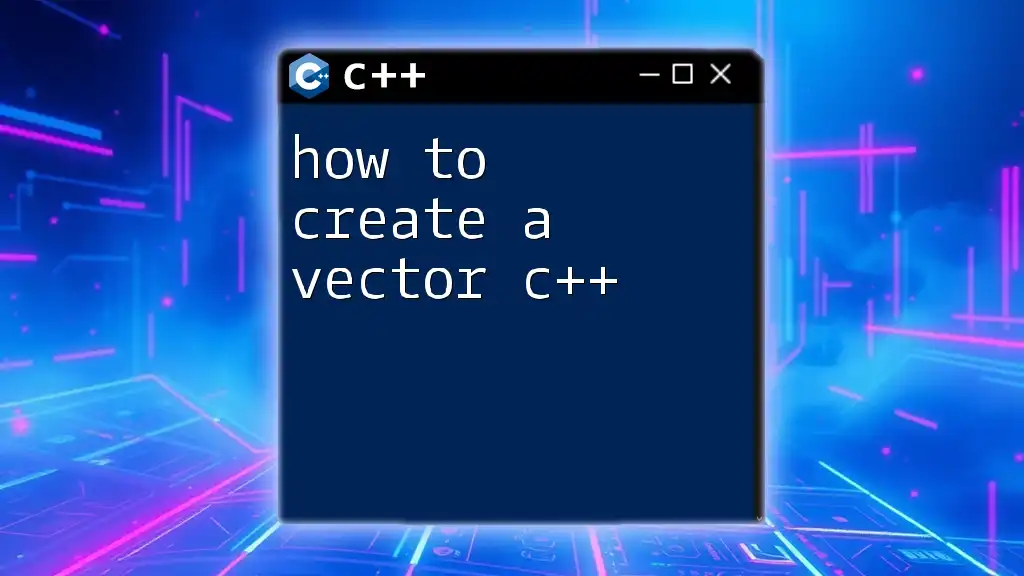
Understanding Constructors and Destructors
What is a Constructor?
A constructor is a special member function of a class that is invoked when an object of the class is created. It sets initial values for the object, ensuring that your object is in a valid state from the moment of creation.
Example of Constructor
class Car {
public:
std::string model;
int year;
Car(std::string m, int y) { // Parameterized constructor
model = m;
year = y;
}
};
What is a Destructor?
A destructor, on the other hand, is called when an object is destroyed. It is primarily used to free resources that the object may have acquired during its lifetime, such as dynamically allocated memory.
Example of Destructor
class Car {
public:
~Car() { // Destructor
// Cleanup logic can go here.
}
};
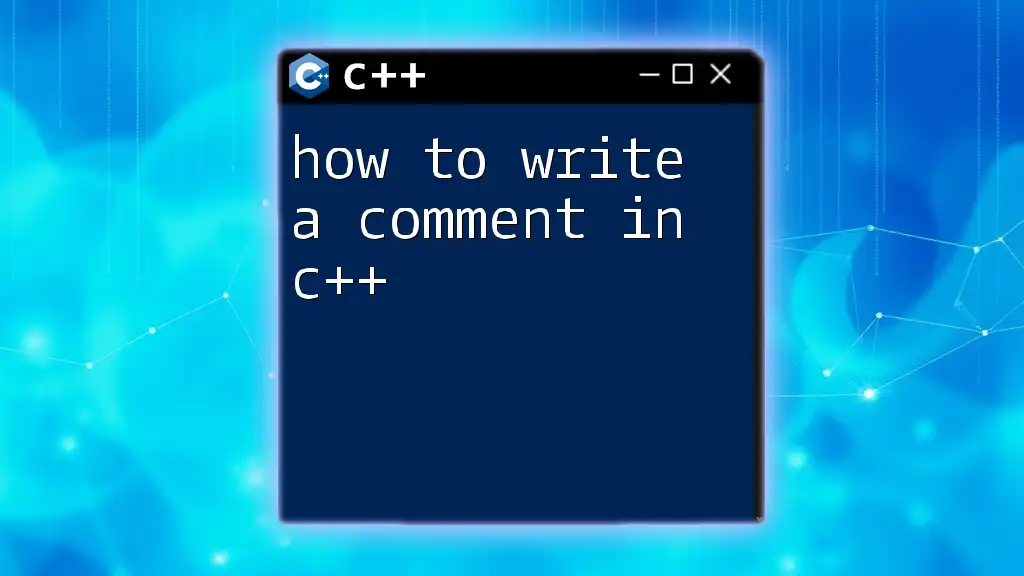
Conclusion
Understanding how to create an object in C++ is fundamental in mastering this powerful language. By learning about class design, constructors, destructors, and the differences between stack and heap allocation, you will grasp the foundational concepts of object-oriented programming.
Being proficient in creating and managing objects will lay the groundwork for more advanced C++ features. Embrace hands-on practice, experiment with the examples provided, and continue to explore the rich world of C++ programming.
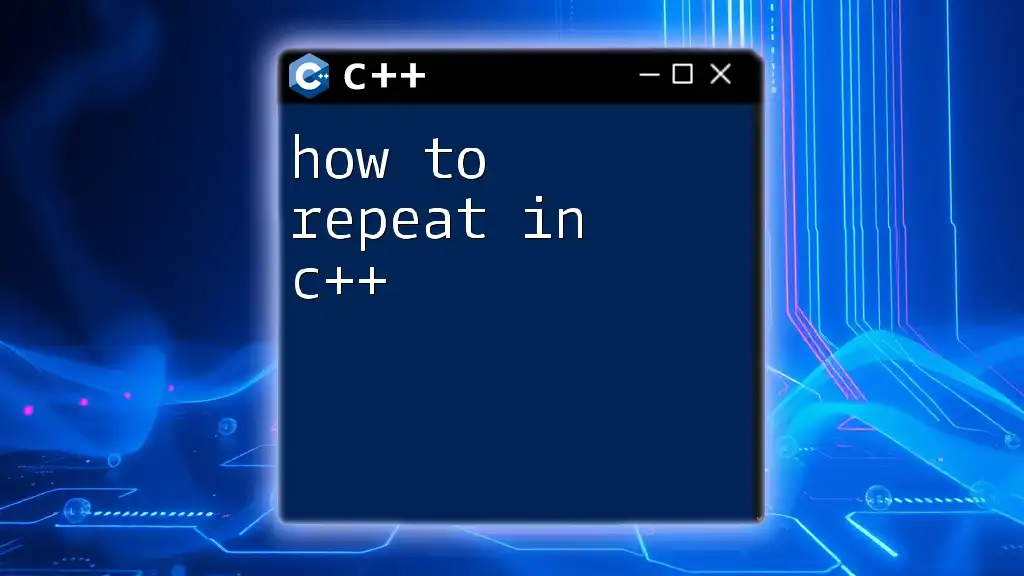
Additional Resources
Leverage online documentation, tutorials, and community forums to deepen your understanding and resolve any queries you may have on your C++ journey. Engaging with other learners or experienced developers will enhance your learning experience and provide valuable insights.
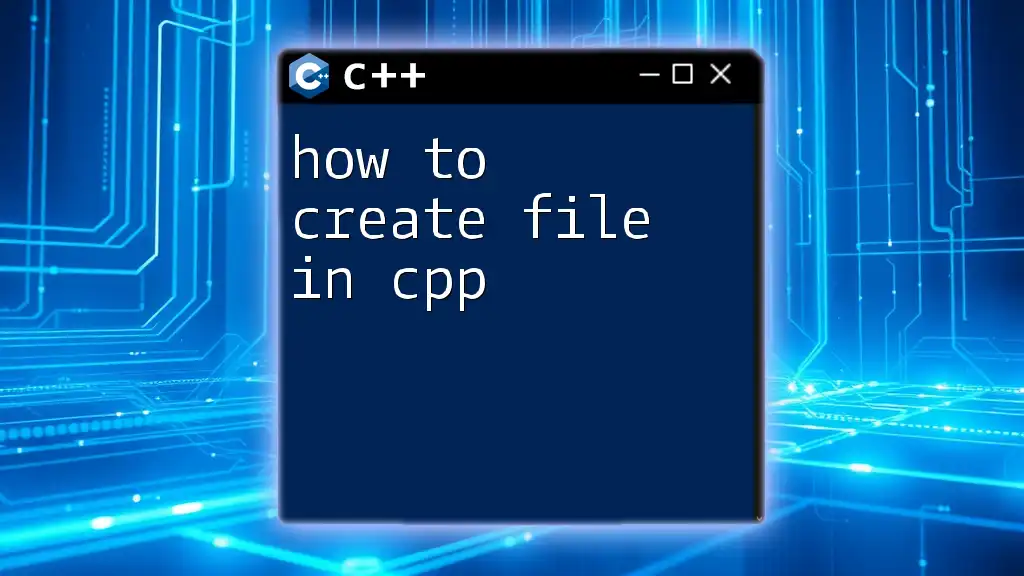
Frequently Asked Questions (FAQs)
-
What are the differences between stack and heap allocation? Stack allocation is automatic and faster but limited in size, while heap allocation is more flexible but requires manual memory management.
-
When should I use constructors and destructors? Always utilize constructors to initialize your objects and destructors to clean up any resources used, especially when working with dynamic memory.