A linked list in C++ can be created by defining a structure for the nodes and then linking them together using pointers.
#include <iostream>
struct Node {
int data;
Node* next;
};
int main() {
Node* head = new Node(); // Create head node
head->data = 1; // Assign value
head->next = nullptr; // End of list
Node* second = new Node(); // Create second node
second->data = 2;
second->next = nullptr;
head->next = second; // Link head to second node
// Output linked list
Node* temp = head;
while(temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
return 0;
}
Understanding Linked Lists
What is a Linked List?
A linked list is a data structure used for organizing and storing data in a linear fashion, allowing for efficient insertion and deletion of elements. Unlike arrays, which have a fixed size, linked lists are dynamic and can grow or shrink in size as needed.
There are several types of linked lists you should be aware of:
- Singly Linked List: Each node contains a single link to the next node. Traversal can only be done in one direction.
- Doubly Linked List: Each node has links to both the next and the previous nodes, allowing for more flexibility in traversal.
- Circular Linked List: The last node points back to the first node, creating a circular structure that allows for continuous traversal.
Benefits of Using a Linked List
Linked lists offer several advantages over traditional arrays, including:
- Dynamic Size: They can grow and shrink in size quickly, avoiding the need for a predetermined size.
- Efficient Insertions and Deletions: Inserting or deleting nodes (at the beginning, end, or middle) can be done without shifting elements, making these operations faster compared to arrays.
- Use Cases: Linked lists are particularly useful in applications like dynamic memory allocation, implementing stacks and queues, and manipulating polynomial expressions efficiently.
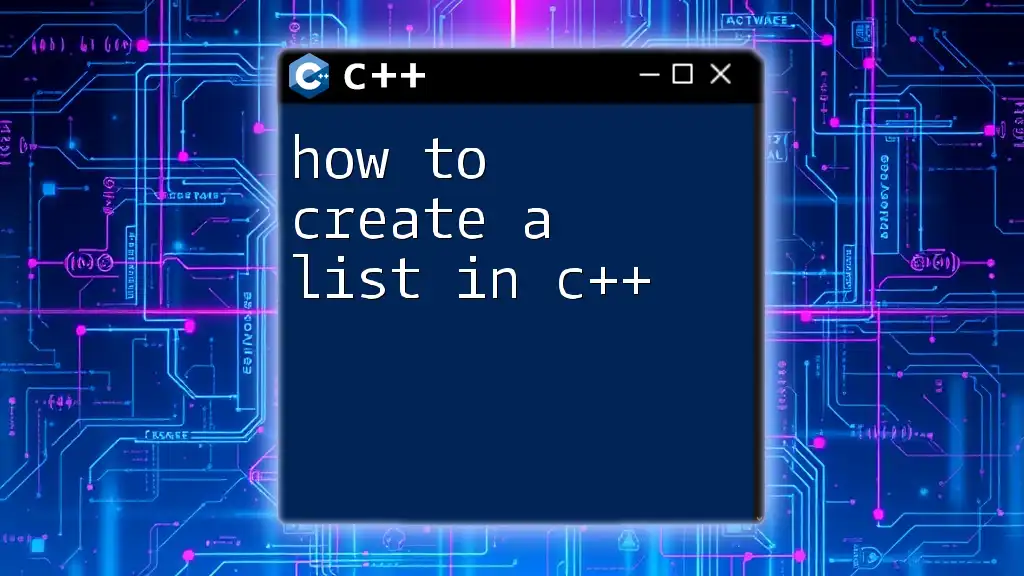
How to Create a Linked List in C++
Defining a Node Structure
At the heart of any linked list is the node. A node holds the data and a pointer to the next node in the sequence. In C++, you can define a simple node structure as follows:
struct Node {
int data;
Node* next; // Pointer to the next node
};
Initializing a Linked List
Before you can manipulate a linked list, you need to initialize it. Here’s how to create an empty linked list by setting the head pointer to `nullptr`:
Node* head = nullptr; // Empty list
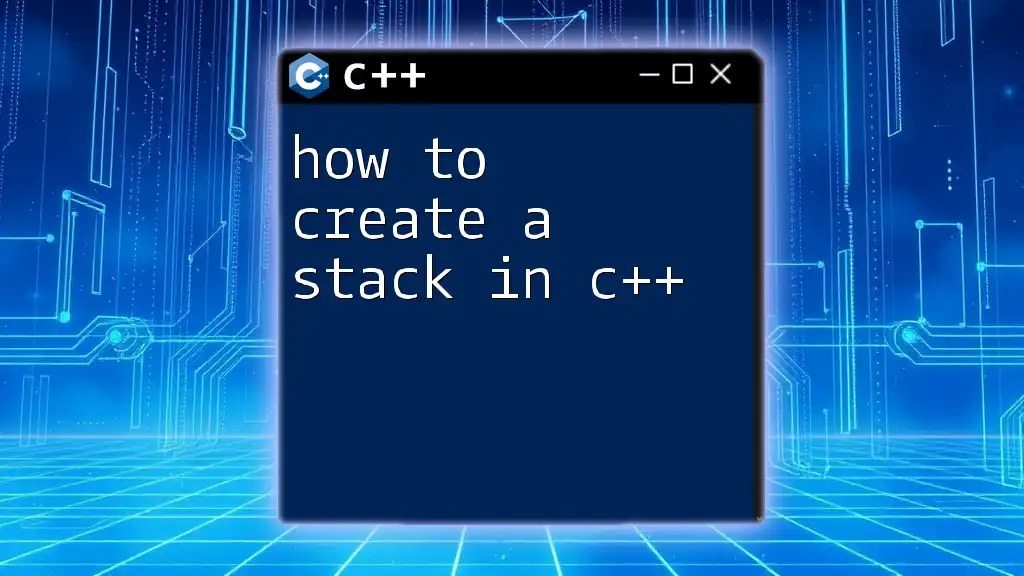
Implementing Basic Linked List Operations
Adding a Node to the Linked List
Adding nodes to a linked list can be done in multiple ways depending on where you want the new node to be inserted.
Insertion at the Beginning
To insert a new node at the start of the list, you can use the following function:
void insertAtBeginning(Node** head_ref, int new_data) {
Node* new_node = new Node();
new_node->data = new_data; // Set the new node's data
new_node->next = (*head_ref); // Link the new node to the previous head
(*head_ref) = new_node; // Move the head to point to the new node
}
Insertion at the End
To append a node at the end of the list, the function below can be used:
void insertAtEnd(Node** head_ref, int new_data) {
Node* new_node = new Node();
Node* last = *head_ref;
new_node->data = new_data; // Set the new node's data
new_node->next = nullptr; // New node will be the last one, so it points to nullptr
if (*head_ref == nullptr) { // If the list is empty, make the new node the head
*head_ref = new_node;
return;
}
// Otherwise, traverse to the last node
while (last->next != nullptr) {
last = last->next;
}
last->next = new_node; // Link the last node to the new node
}
Insertion After a Given Node
If you want to insert a new node after a specific node in the list, here's how you can do it:
void insertAfter(Node* prev_node, int new_data) {
if (prev_node == nullptr) {
std::cout << "The given previous node cannot be NULL.";
return;
}
Node* new_node = new Node();
new_node->data = new_data; // Set the new node's data
new_node->next = prev_node->next; // Link the new node to the next node
prev_node->next = new_node; // Make the previous node point to the new node
}
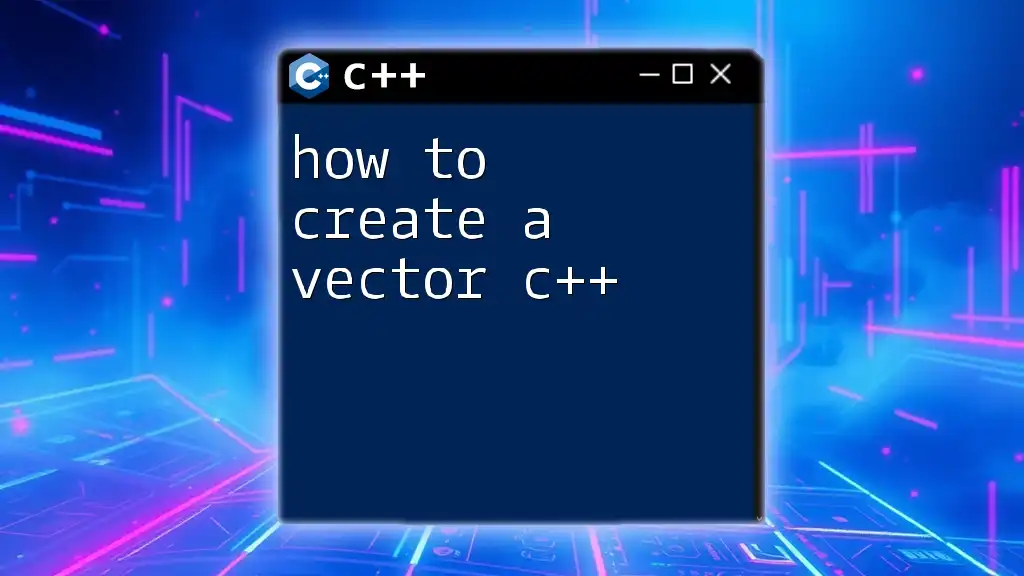
Traversing a Linked List
Displaying the Elements
To traverse and display each element in the linked list, you can create a function like this:
void displayList(Node* node) {
while (node != nullptr) {
std::cout << node->data << " "; // Print the node's data
node = node->next; // Move to the next node
}
}
This simple function allows you to see the contents of your linked list efficiently.
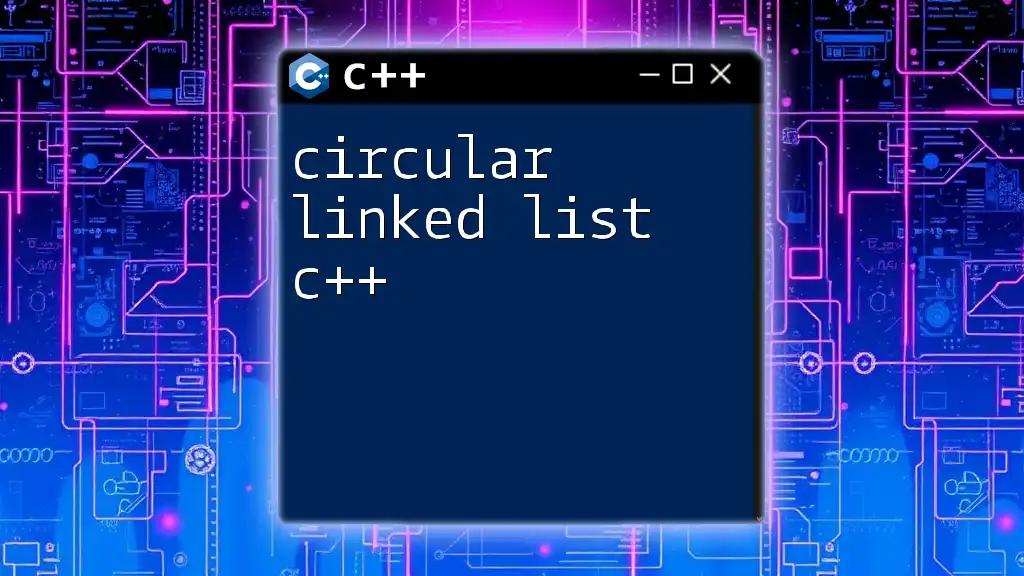
Deleting Nodes from a Linked List
Deleting a Node by Key
Removing a node from a linked list involves searching for the node containing the specified key and adjusting the pointers accordingly. Here’s a function to accomplish that:
void deleteNode(Node** head_ref, int key) {
Node* temp = *head_ref, *prev = nullptr; // Initialize temp and prev pointers
// If the head node itself holds the key to be deleted
if (temp != nullptr && temp->data == key) {
*head_ref = temp->next; // Move the head to the next node
delete temp; // Free memory of the old head
return;
}
// Search for the key to be deleted
while (temp != nullptr && temp->data != key) {
prev = temp; // Keep track of the previous node
temp = temp->next;
}
// Key was not present in linked list
if (temp == nullptr) return;
prev->next = temp->next; // Unlink the node from the list
delete temp; // Free memory
}
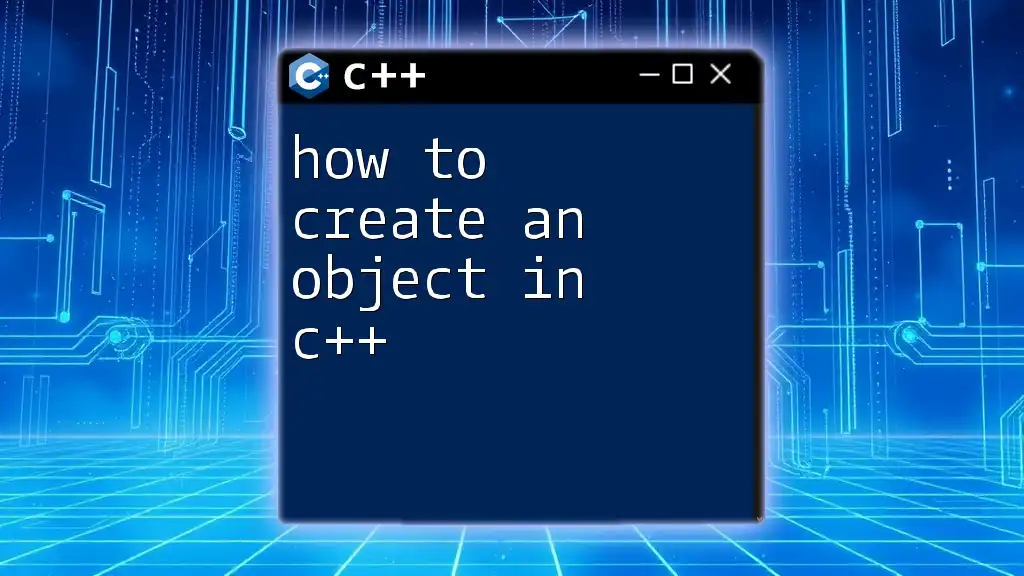
Other Linked List Variations
Doubly Linked Lists
A doubly linked list allows traversal in both directions by maintaining a pointer to the previous node in addition to the next one. This structure offers greater flexibility and makes certain operations, such as deletion, easier as you can access both ends of the list.
Circular Linked Lists
In a circular linked list, the last node points back to the first node, creating a circular structure that eliminates the notion of a "tail." This feature allows for continuous traversal which can be beneficial in applications where the end of the list is frequently revisited.
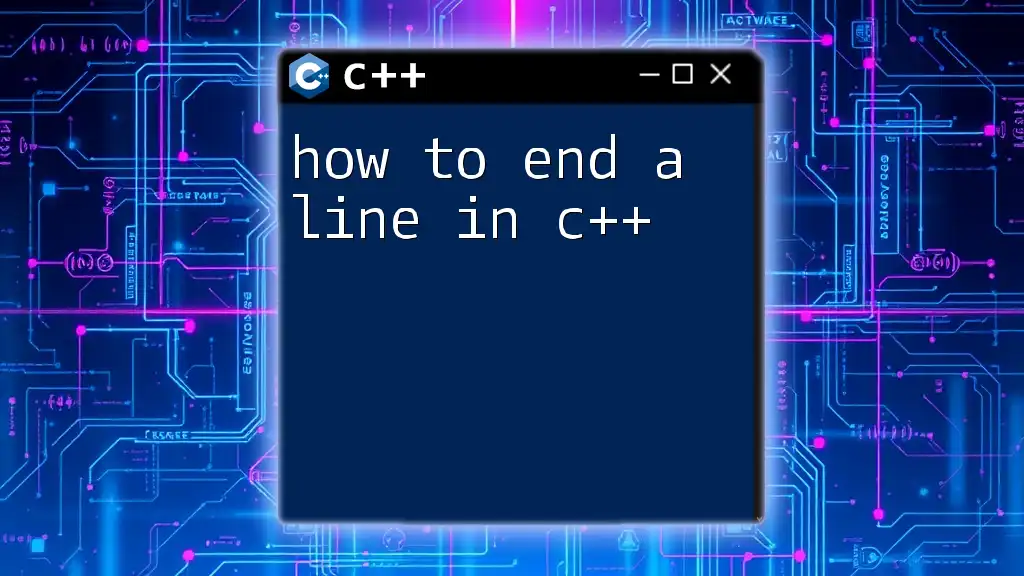
Conclusion
In this guide, we've discussed in-depth how to create a linked list in C++ and implement various operations such as insertion, traversal, and deletion. Understanding linked lists is essential for anyone looking to delve into advanced data structures and algorithms. With practice in these concepts, you'll be well-equipped to handle a variety of programming challenges that utilize linked lists effectively.
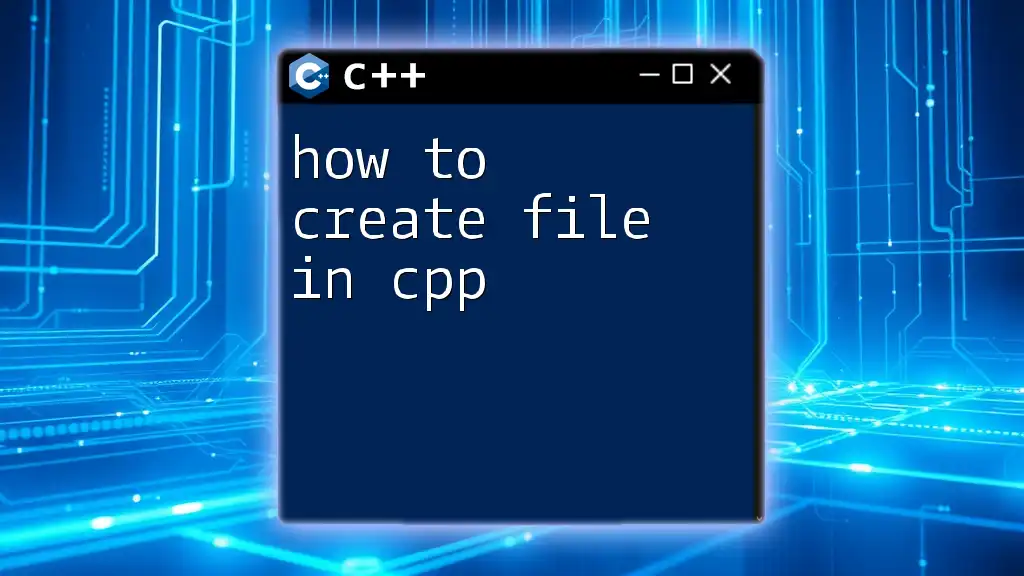
Additional Resources
To gain a deeper understanding and further explore linked lists in C++, consider looking into advanced data structure practices, algorithm analysis, and real-world applications. There are numerous excellent resources available that can help you master this crucial topic.