To create a file in C++, you can use the `ofstream` class from the `<fstream>` header to open a file in write mode, as demonstrated in the following example:
#include <fstream>
int main() {
std::ofstream outfile("example.txt"); // Create and open a file named example.txt
outfile << "Hello, World!"; // Write to the file
outfile.close(); // Close the file
return 0;
}
Understanding File Streams in C++
In C++, file handling is accomplished using file streams. A file stream allows you to perform input and output operations on files, similar to how you handle standard input and output (like the console). The main types of file streams you will encounter are:
- `ofstream` - This is used for output file streams, meaning you can write data to files.
- `ifstream` - This stands for input file streams and is used for reading data from files.
- `fstream` - A combination of both input and output streams, allowing you to read from and write to files simultaneously.
Understanding these streams is crucial when learning how to create a file in C++ as they form the foundation of file manipulation in the language.
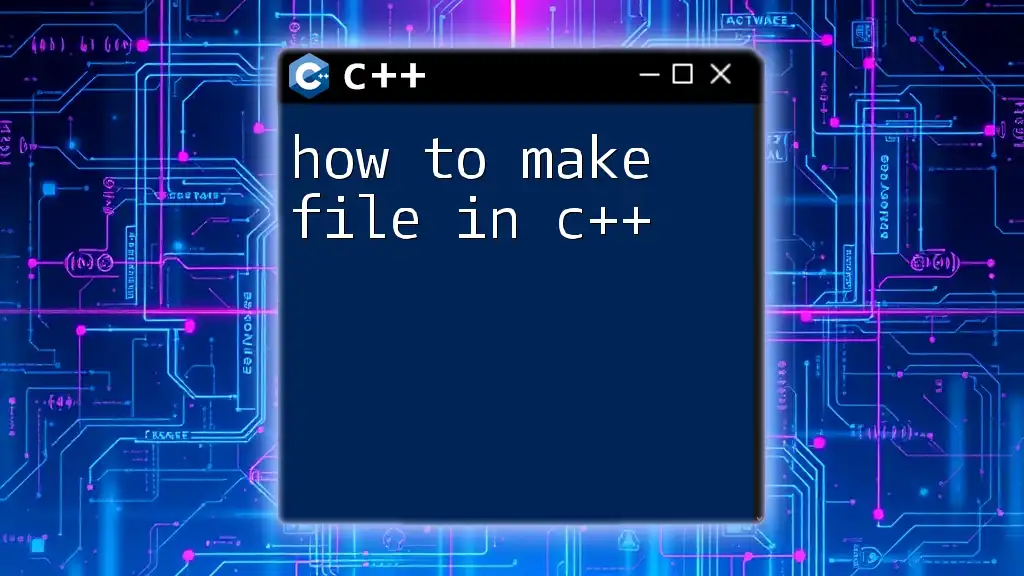
Setting Up Your Environment
Before you start creating files in C++, ensure that you have the appropriate libraries included at the top of your code. The fstream library is essential for file operations. Here’s how you set it up:
#include <iostream>
#include <fstream>
After including these headers, you can compile and run your C++ program using any integrated development environment (IDE) or compiler like g++, Visual Studio, or Code::Blocks. Make sure you save your changes before attempting to run the program.
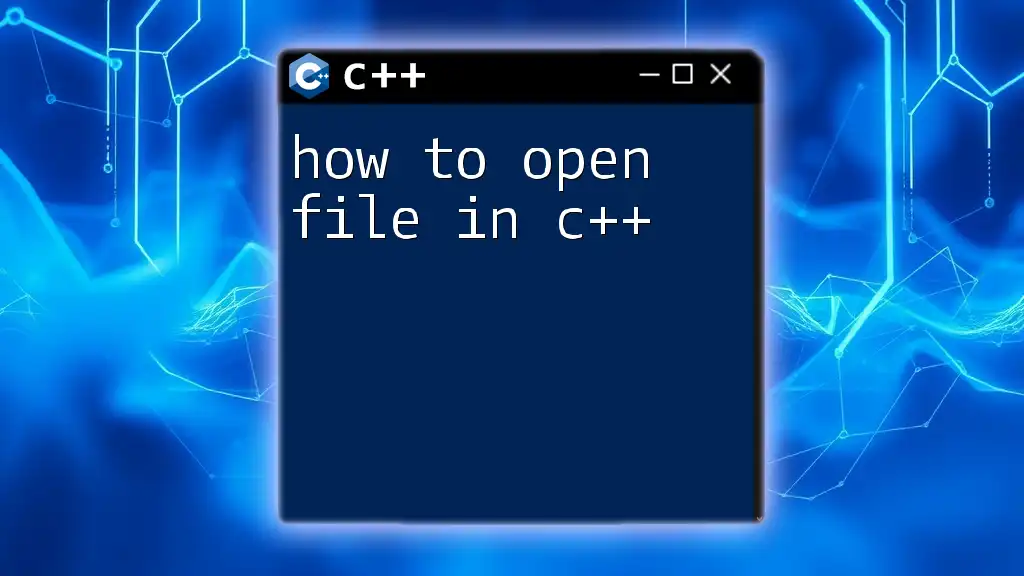
How to Create a File in C++
Overview of File Creation Methods
C++ offers several ways to create files, but using the `ofstream` class is the most straightforward method for beginners. Let’s explore how to effectively utilize it to accomplish your goal of creating a file in C++.
Using `ofstream` to Create a File
What is `ofstream`?
`ofstream` is specifically designed for creating and writing output files. It requires a file name as an argument to the constructor, which will dictate the file's name and destination.
To create a file using `ofstream`, consider the following example:
#include <fstream>
int main() {
std::ofstream myFile("example.txt");
if (myFile.is_open()) {
myFile << "Hello, World!";
myFile.close();
} else {
std::cout << "Unable to open file";
}
return 0;
}
In this code snippet:
- The `myFile` object is created using `std::ofstream` with "example.txt" as the file name. If this file does not exist, it will be created.
- The `is_open()` function checks whether the file was successfully opened.
- We write "Hello, World!" to the file using the output operator (`<<`).
- Finally, it's good practice to close the file using the `close()` method to free system resources.
This simple example illustrates the primary method of how to create a file in C++.
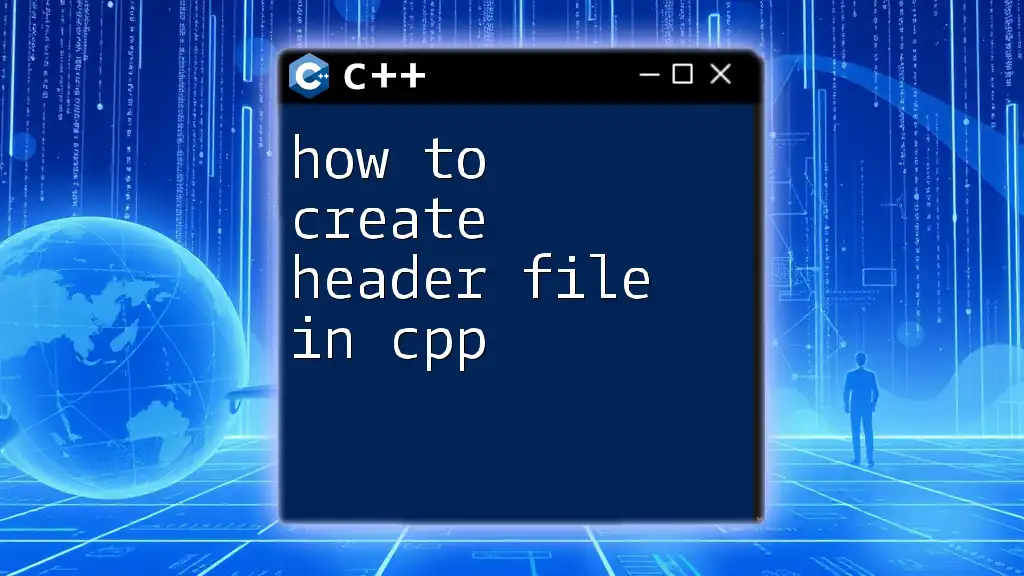
Confirming File Creation
Checking if the File Exists
Once a file has been created, it’s essential to confirm that it exists and operates correctly. You can use `ifstream` to verify the file's creation. Here’s how you can do it:
#include <fstream>
int main() {
std::ifstream infile("example.txt");
if (infile.good()) {
std::cout << "File created successfully!";
} else {
std::cout << "File does not exist.";
}
return 0;
}
In this code:
- `std::ifstream infile("example.txt");` attempts to open "example.txt" for reading.
- The `good()` function checks if the file opened correctly. If it does, the program outputs "File created successfully!", confirming your success in file creation.
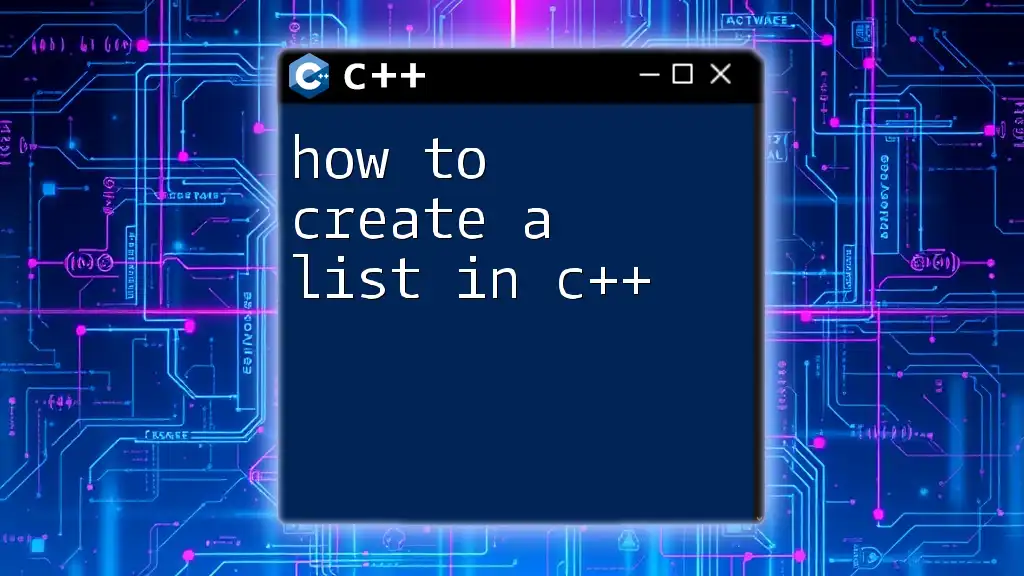
Appending to an Existing File
How to Append Data in C++
Appending data to an already existing file is another important aspect of file handling. When you want to add content without erasing the existing data, you can open the file in append mode using `std::ios::app`.
Here’s a simple code snippet that demonstrates how to append data to your file:
#include <fstream>
int main() {
std::ofstream myFile("example.txt", std::ios::app);
if (myFile.is_open()) {
myFile << "\nAppending new line!";
myFile.close();
}
return 0;
}
In this code:
- The file is opened in append mode by passing `std::ios::app` as a second argument to the constructor.
- Using the `<<` operator, you can seamlessly add a new line of text to the existing content of "example.txt".
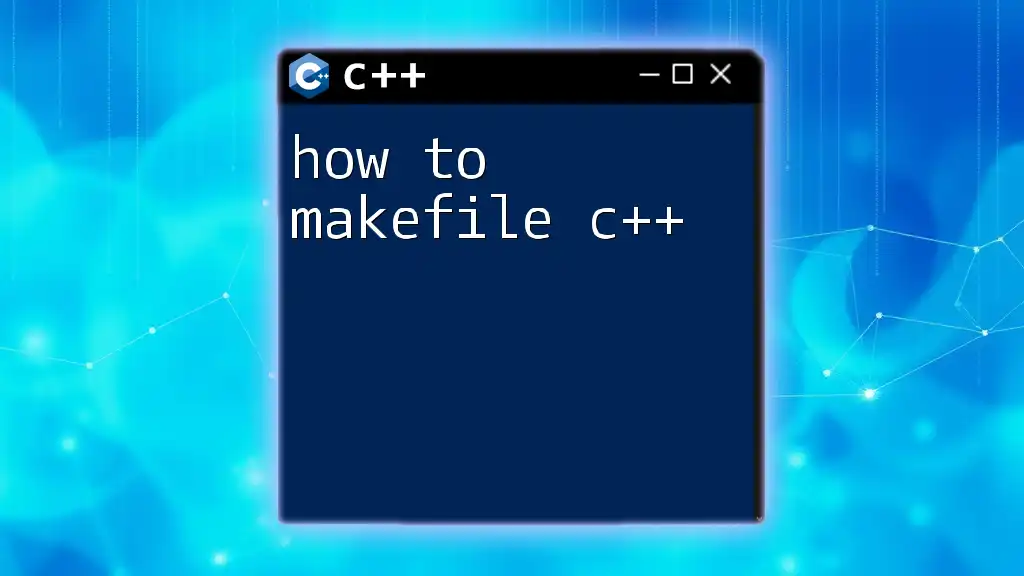
Error Handling While Creating a File
Best Practices for Error Handling
When working with files, it's essential to handle potential errors gracefully. A robust way to handle exceptions in file operations is by using `try-catch` blocks. This ensures that your program does not crash unexpectedly.
Here's how to implement error handling while creating a file:
#include <fstream>
#include <iostream>
int main() {
try {
std::ofstream myFile("example.txt");
if (!myFile) {
throw std::ios_base::failure("Failed to open file");
}
// Rest of the file operations...
} catch (const std::ios_base::failure& e) {
std::cerr << e.what() << '\n';
}
return 0;
}
Explanation of the code:
- Within the `try` block, we attempt to create the file. If it fails, we throw an exception with a descriptive error message.
- The catch block catches the exception and prints the error message, helping you understand what went wrong.
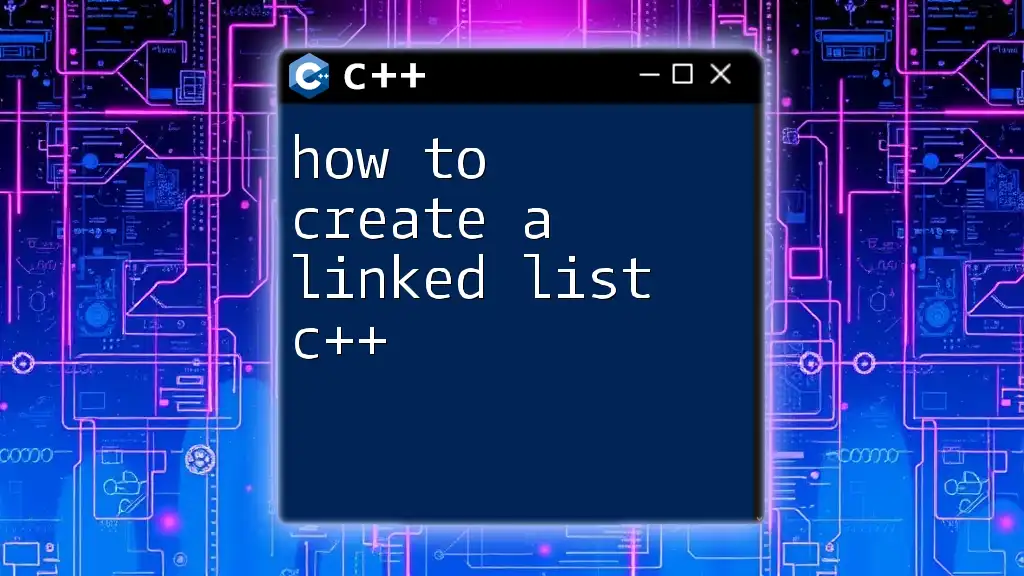
Conclusion
Creating a file in C++ is a fundamental programming skill that is essential for managing data effectively. By utilizing the `ofstream`, you can easily create and manipulate files, as we've seen through various examples. Remember to always check for successful file creation and implement error handling to make your programs robust.
With this knowledge, you're now equipped to explore more advanced file handling techniques and enhance your C++ projects. Happy coding!