To parse a CSV file in C++, you can use the `ifstream` class to read the file line by line and the `stringstream` class to split each line into individual values based on a delimiter (usually a comma). Here's a simple example:
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <vector>
void parseCSV(const std::string& filename) {
std::ifstream file(filename);
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string value;
std::vector<std::string> row;
while (std::getline(ss, value, ',')) {
row.push_back(value);
}
// Process the row (for example, print values)
for (const auto& val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
}
int main() {
parseCSV("data.csv");
return 0;
}
Understanding CSV Files
What is a CSV File?
A CSV (Comma-Separated Values) file is a simple text format used to store tabular data. Each line in a CSV file corresponds to a row in the table, and within each line, the fields are separated by commas (or other delimiters). This format is widely adopted in data exchange because it is both human-readable and easily parsed by computer programs.
Structure of a CSV File
CSV files typically consist of two primary components:
- Headers: The first row often contains the names of the columns, which describe the data fields.
- Data Rows: Subsequent rows contain the actual data points, with each value corresponding to a header.
Beyond the basic structure, it's essential to note the following:
- Delimiters: While commas are the default, other characters, like semicolons or tabs, can separate the values. This flexibility can be significant when dealing with data that may contain commas.
- Quoting: If a field contains a newline or a delimiter, it is often enclosed within double quotes. Escape characters may be used to include quotes within fields.
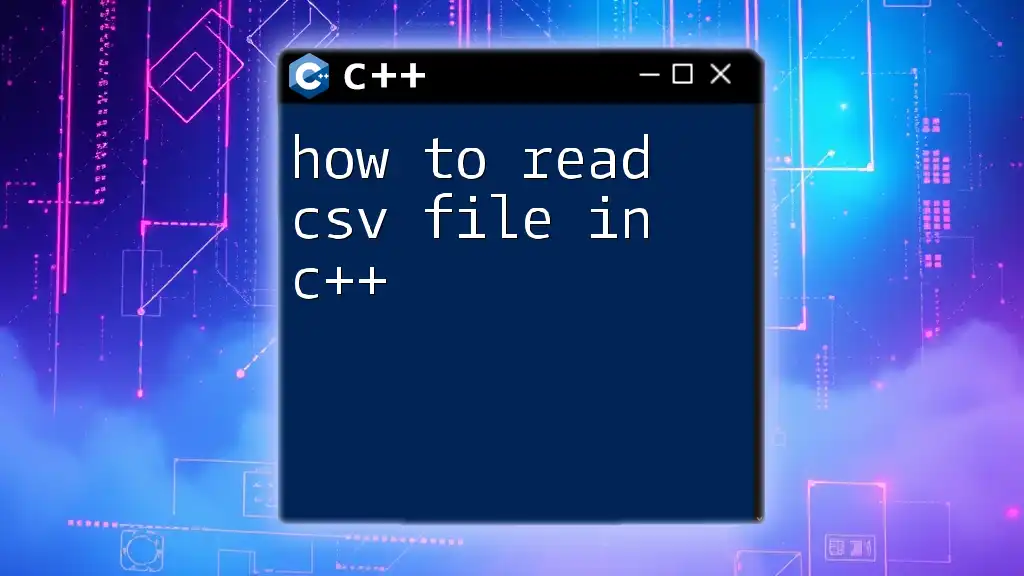
Setting Up the Environment
Required Tools and Libraries
To interact with CSV files, a basic C++ setup is sufficient. However, for more advanced needs, consider using libraries such as Boost for robust string and file handling or specialized libraries like RapidCSV or CSV-parser for simplified usage.
Creating a New C++ Project
Get started by creating a simple C++ project. Use any Integrated Development Environment (IDE) of your choice, such as Visual Studio, Code::Blocks, or even a text editor and command line. Ensure your project is set up with the necessary compiler and library paths.
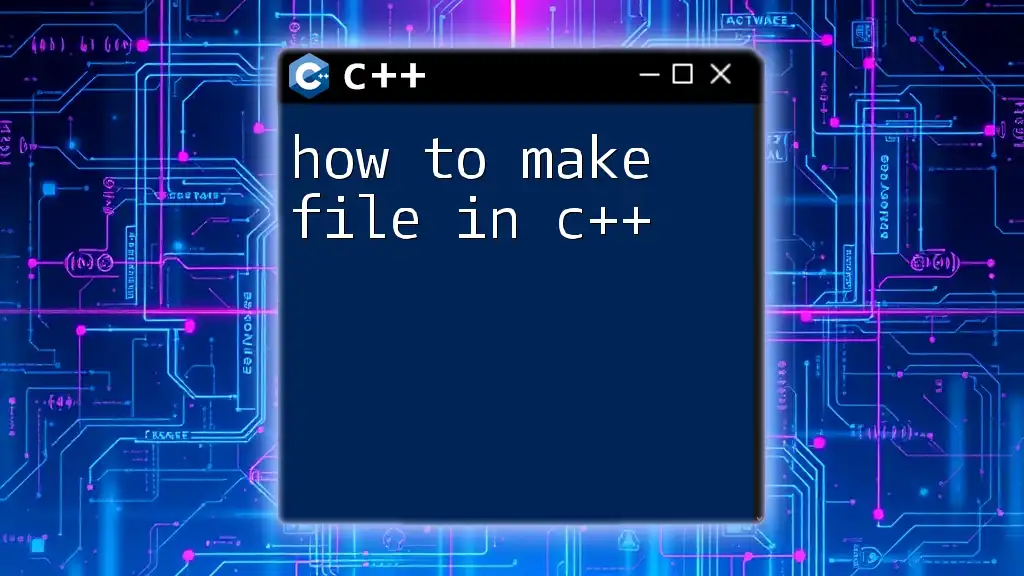
Basic CSV Parsing in C++
Reading a File
To read a CSV file in C++, we typically utilize file input/output functionality. Here's how you can open a file for reading:
#include <fstream>
#include <iostream>
#include <string>
std::ifstream inputFile("data.csv");
if (!inputFile) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
In this snippet, we include essential headers, open the CSV file, and check for errors in opening the file.
Parsing the Data
Using `getline()` for Row Reading
The `getline()` function is crucial for reading a file line by line. Each line will represent a row in the CSV. Below is an example of using `getline()` within a loop to process each row:
std::string row;
while (std::getline(inputFile, row)) {
// Process each row here
}
Within this loop, you can focus on tokenizing the row into individual cells.
Tokenizing a Row into Columns
To split each row into columns, we can utilize the `std::istringstream` class from the `<sstream>` header. Here’s how:
#include <sstream>
std::istringstream ss(row);
std::string cell;
while (std::getline(ss, cell, ',')) {
// Process each cell
}
In this code, we create a string stream from the row and use `getline()` again to retrieve each cell by specifying the delimiter (a comma in this case).
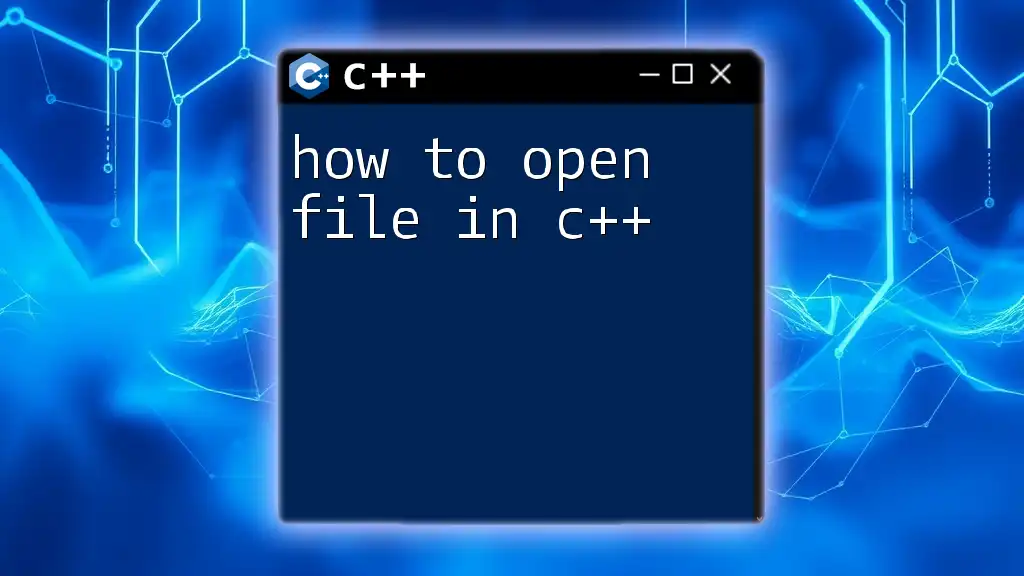
Handling Advanced Cases
Handling Different Delimiters
Sometimes, CSV files may use different delimiters. To handle this, simply replace the delimiter argument in the `getline()` function:
while (std::getline(ss, cell, ';')) {
// Process cell for semi-colon separated values
}
This approach allows for flexibility in parsing.
Dealing with Quoted Values
CSV parsing can get complicated when fields contain quoted values. A quoted field can contain commas without being split into multiple fields. Here’s a basic outline of how to handle such cases:
std::string parseCell(std::string& cell) {
// Strip quotes and handle escape characters
if (cell.front() == '"' && cell.back() == '"') {
cell = cell.substr(1, cell.size() - 2);
}
return cell;
}
// In the tokenization loop
std::string parsedCell = parseCell(cell);
By wrapping the cell processing in a dedicated function, you create a clean, reusable method that manages quoted cells effectively.
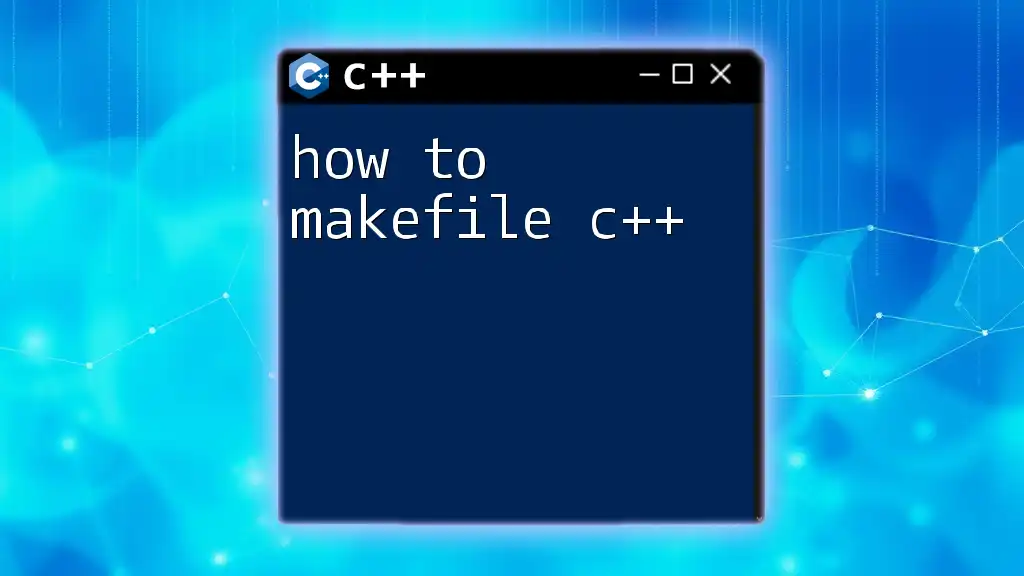
Error Handling and Edge Cases
Common Parsing Errors
During parsing, you may encounter several issues:
- Missing values: Some rows may have missing data, leading to inconsistencies.
- Extra delimiters: Occasionally, some rows may include additional delimiters, causing incorrect tokenization.
Implementing Error Handling
Implementing integrity checks for each row can help in handling these issues effectively. For example:
if (numberOfFields != expectedNumberOfFields) {
std::cerr << "Row has an incorrect number of fields!" << std::endl;
continue; // Skip to the next row
}
By validating row data before processing it, you can gracefully manage errors without crashing your application.
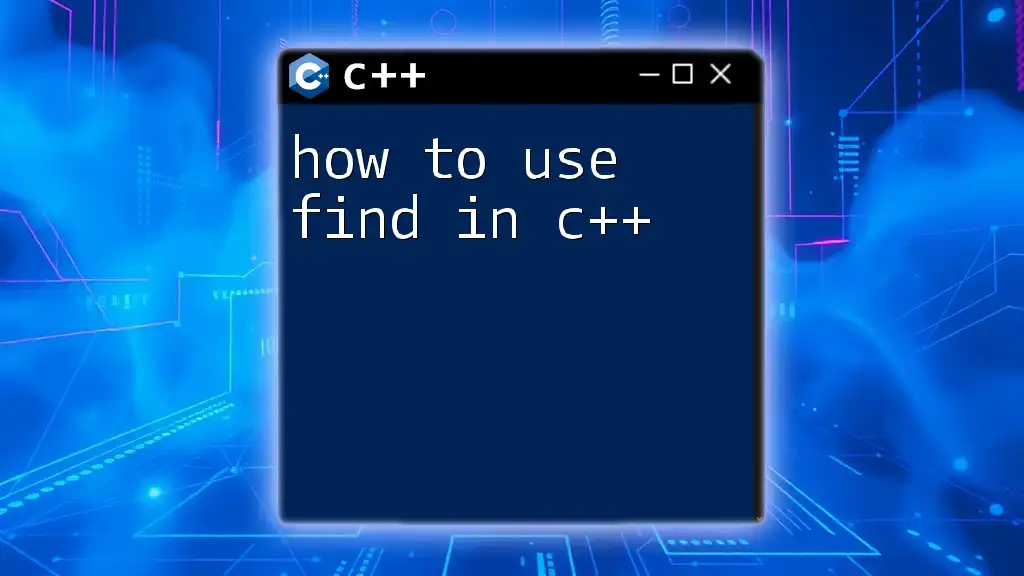
Practical Applications of CSV Parsing
Creating a Simple CSV Reader
You can develop a simple CSV reader class to encapsulate the parsing logic. Below is an example skeleton of a CSV reader class:
class CSVReader {
public:
void parse(const std::string& filename);
};
This class can include member functions for file reading, row parsing, and error checking, promoting modular design.
Example Use Cases
Parsing CSV files can find applications in:
- Data analysis: Extracting and analyzing large datasets.
- Importing into databases: Streamlining data import processes when populating a database.
- Batch processing: Handling multiple record entries efficiently.
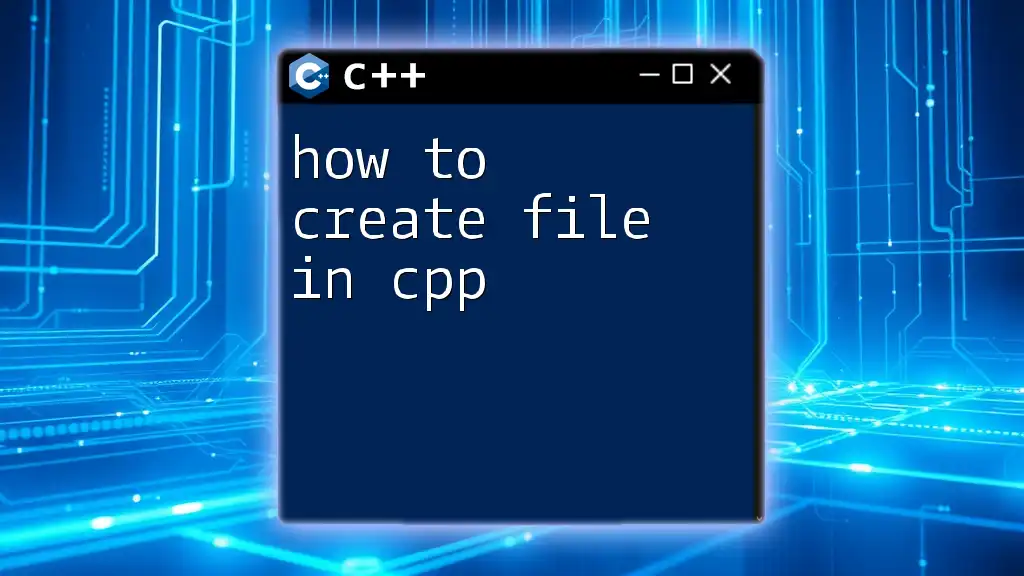
Performance Considerations
Optimize for Large CSV Files
When dealing with large files, consider:
- Efficient file reading techniques: Avoid loading the entire file into memory. Process it line by line as shown earlier.
- Memory management strategies: Allocate memory only when necessary and release it promptly to prevent leaks.
Using Libraries for Performance Boost
Libraries like Boost or RapidCSV can significantly enhance performance and usability, offering advanced features for CSV parsing, like built-in error handling and fast read/write operations.
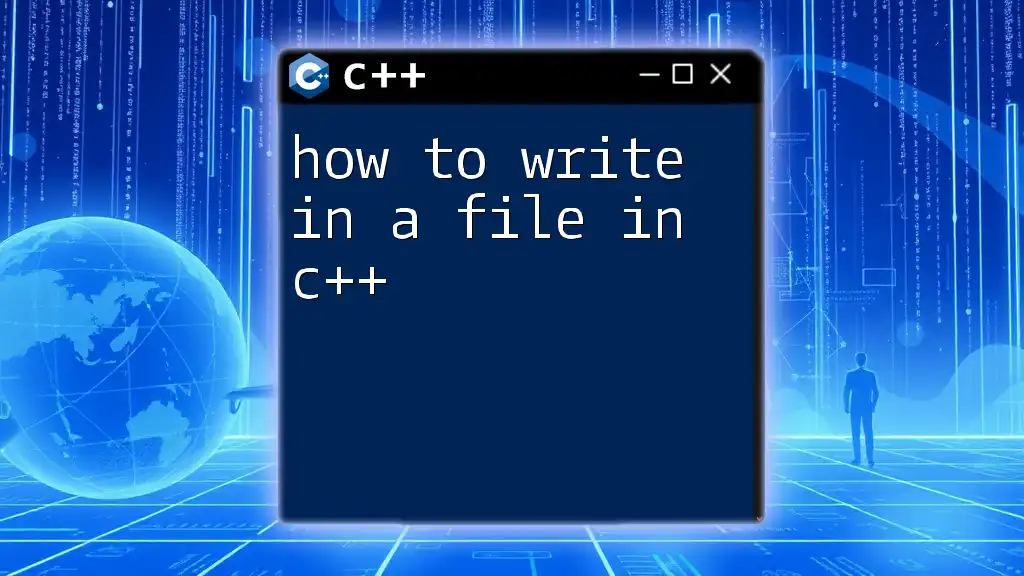
Conclusion
In this comprehensive guide, we've explored how to parse CSV files in C++, covering everything from the structure of CSV files to practical applications and performance considerations. The techniques discussed provide a solid foundation for working with CSV data, allowing you to tailor your parsing solutions to meet specific project needs.
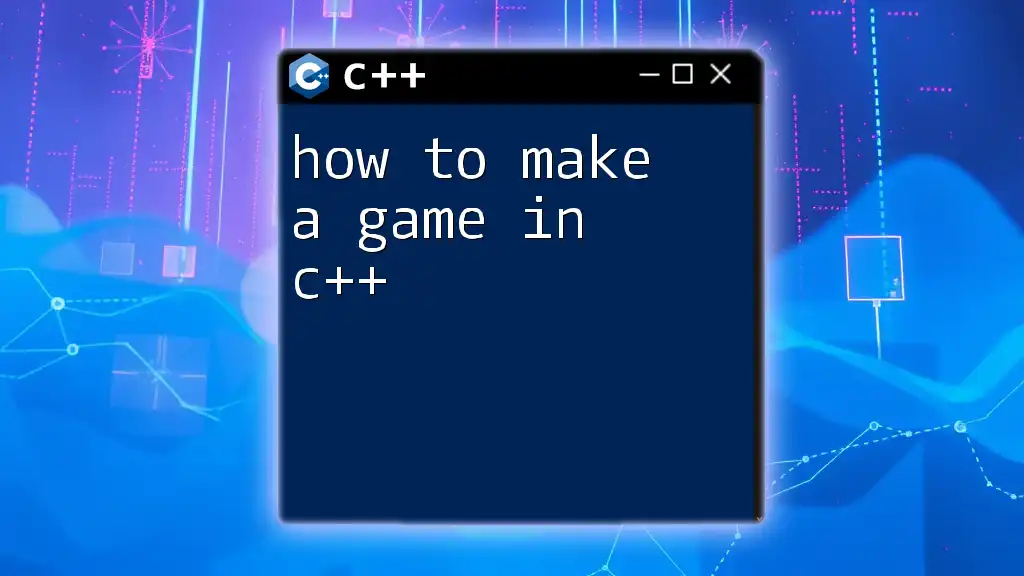
Call to Action
Now that you are equipped with the essential knowledge on how to parse csv file in c++, I encourage you to implement your parser and share your experiences or questions in the comments. Happy coding!