To start coding in C++, install a compiler like GCC or Clang, create a simple program that prints "Hello, World!" to the console, and compile it using your terminal.
Here's a basic example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
Setting Up Your Development Environment
Choosing an IDE or Text Editor
To embark on your journey of learning how to start coding in C++, the first step is setting up your development environment. An Integrated Development Environment (IDE) or a text editor provides the tools you need to write, compile, and debug your C++ code. Some popular options include:
- Visual Studio: Best for Windows users, offering a comprehensive toolset with a robust debugger.
- Code::Blocks: A free, open-source IDE suitable for various platforms.
- Dev-C++: Lightweight and easy to use for beginners.
- Online Compilers: Websites like repl.it or ideone.com provide an instant online coding experience without the need for installation.
Follow the installation instructions provided on their respective websites to ensure a smooth setup.
Installing a Compiler
A compiler transforms your C++ code into executable programs. One of the most popular compilers is GCC (GNU Compiler Collection). To install GCC on Linux, use the terminal and type:
sudo apt-get install g++
For Windows, you can install Visual Studio, which comes with the MSVC compiler. Be sure to set the compiler path in your IDE.
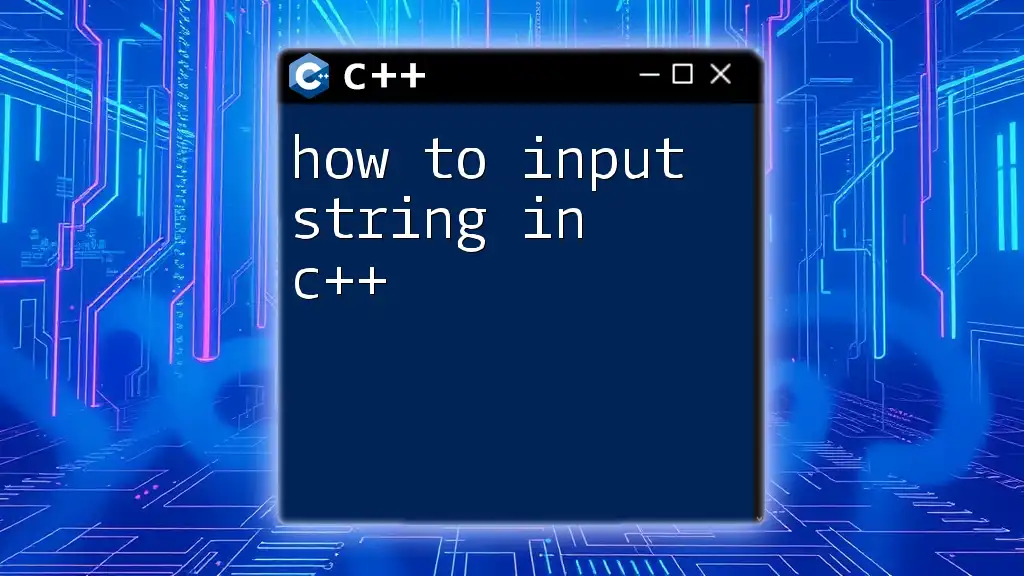
Basic Concepts of C++
Understanding C++ Syntax
Basic Structure of a C++ Program
Understanding the basic structure of a C++ program is essential in how to start coding in C++. Here’s a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` is an include directive, allowing access to input/output stream functionalities.
- `int main()` is the main function, where execution begins.
- `std::cout << "Hello, World!" << std::endl;` outputs text to the console.
- `return 0;` signifies successful completion of the program.
Variables and Data Types
What are Variables?
In C++, a variable is a storage location identified by a name. It can hold data values. Their purpose is to maintain state between different parts of your code. Here’s how to declare some variables:
int age = 25;
float height = 5.8;
char initial = 'A';
Different Data Types in C++
C++ supports various data types, and understanding these is crucial for effective coding:
- int: Integer type. Example: `int score = 100;`
- float: Floating-point number. Example: `float weight = 70.5;`
- char: Represents a single character. Example: `char grade = 'A';`
- double: Double-precision floating-point. Example: `double pi = 3.141592;`
- string: Series of characters. Example: `std::string name = "Alice";`
Get comfortable with these data types to excel in your programming.
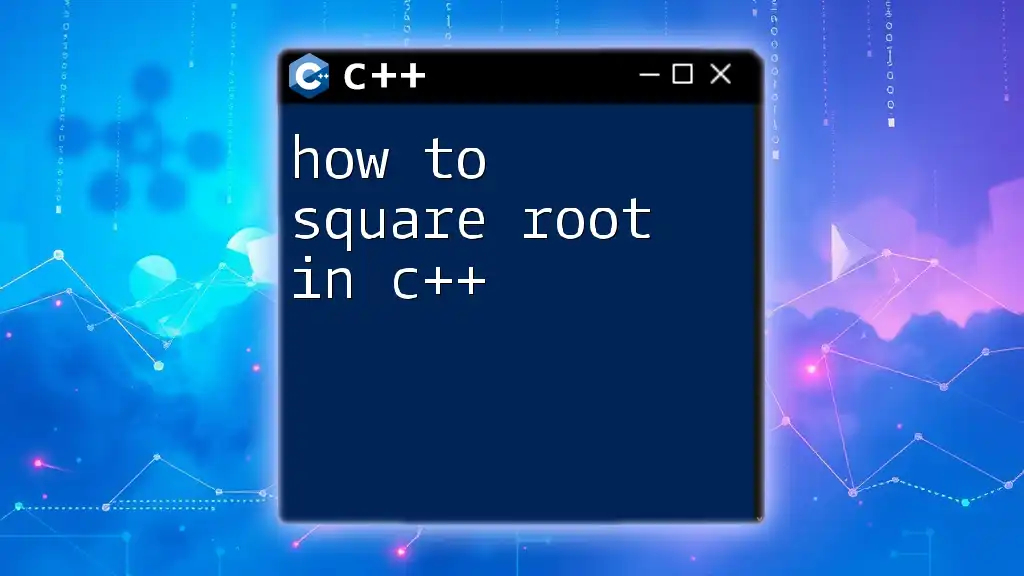
Control Structures
Conditional Statements
If-Else Statements
Control structures allow for decision-making in code. An if-else statement can guide your code's flow based on conditions. Here’s an example:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
In this snippet, if the value of `age` is 18 or more, it outputs "Adult"; otherwise, it outputs "Minor". This structure is a building block for more complex logic.
Loops
For Loop
Loops enable you to execute a block of code multiple times. A for loop is generally used when the number of iterations is known:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
This loop will print numbers 0 to 4. Understanding loops is vital in learning how to iterate through data or repeat operations efficiently.
While Loop
A while loop executes as long as a given condition remains true. Example:
int i = 0;
while (i < 5) {
std::cout << i << std::endl;
i++;
}
This loop will also output numbers 0 to 4, with each iteration incrementing `i` until it reaches 5.
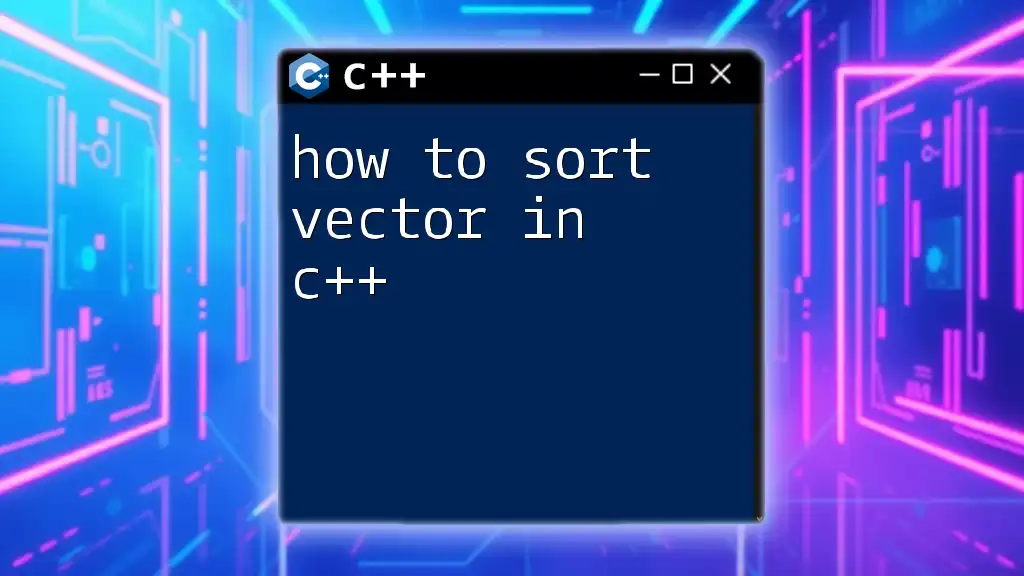
Functions
What are Functions?
Functions are a way to encapsulate code for reusability and organization. They allow you to perform specific tasks without duplicating code.
Creating Your First Function
Here’s a simple function to add two numbers:
int add(int a, int b) {
return a + b;
}
You call this function by using:
int sum = add(5, 3);
std::cout << "Sum is: " << sum << std::endl;
Understanding functions can vastly improve your coding efficiency as you break down complex problems.
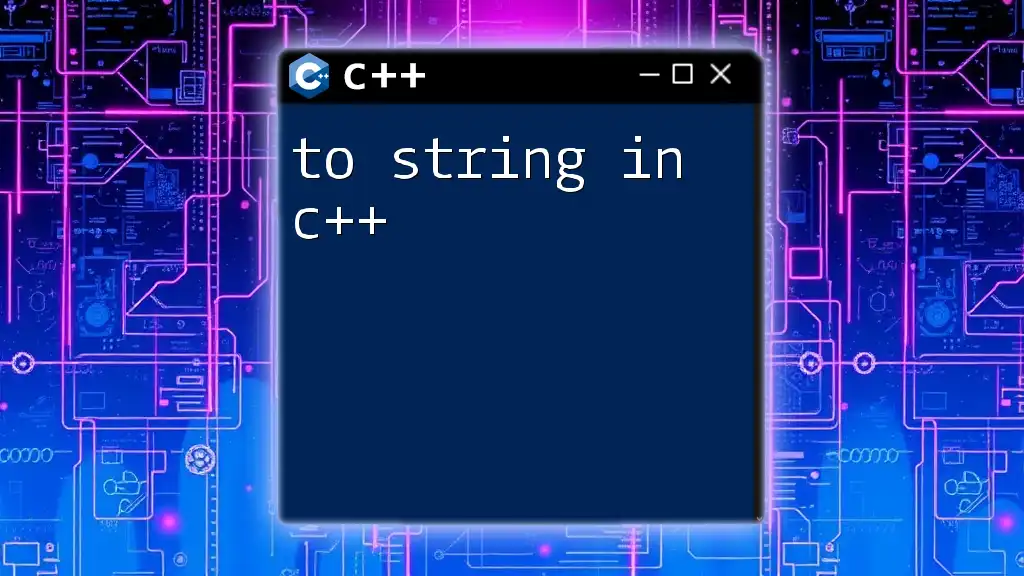
Object-Oriented Programming in C++
Introduction to OOP Concepts
C++ is an object-oriented programming language, which means it allows you to use classes and objects as fundamental building blocks. OOP concepts such as encapsulation, inheritance, and polymorphism help organize and manage code complexity.
Creating a Class
Here’s a simple class definition:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In the above code:
- The class keyword defines a new class.
- `public` allows the function `bark()` to be accessible from outside the class.
You can create an object of this class and call its function like this:
Dog myDog;
myDog.bark();
This snippet showcases the power of OOP in C++, enabling abstraction of real-world entities.
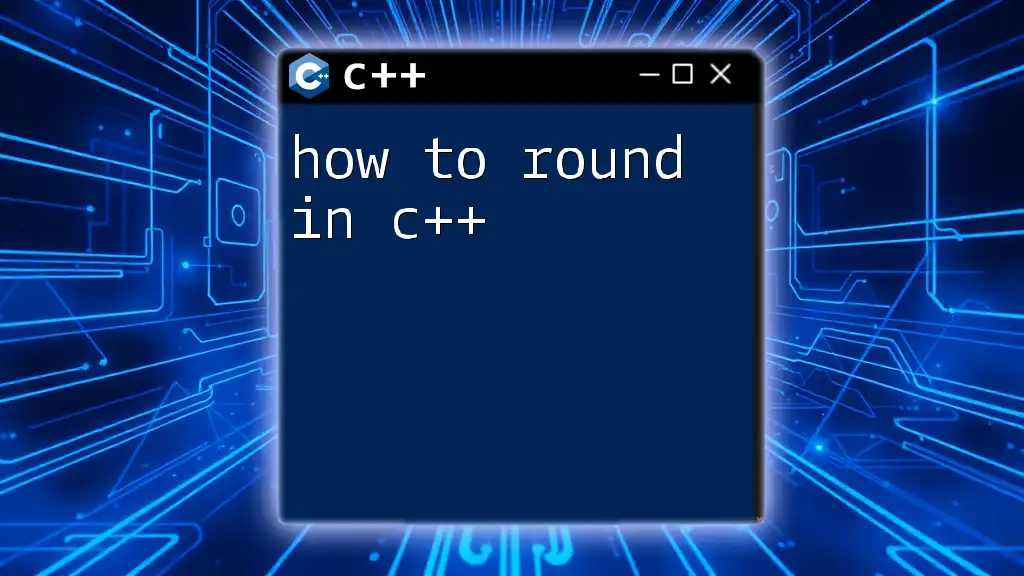
Debugging and Best Practices
Common Errors and How to Fix Them
As you learn how to start coding in C++, you will inevitably encounter errors. Common types include:
- Syntax Errors: Mistakes in spelling or punctuation.
- Runtime Errors: Problems that arise while executing the program (e.g., division by zero).
- Logical Errors: The program runs, but the output is not as expected.
Using a debugger tool within your IDE can help isolate these issues, making it easier to fix them.
Best Coding Practices
Writing clean, maintainable code is fundamental. Here are some best practices:
- Comment your code: Explain what complex code does for future reference.
- Consistent indentation and naming conventions: Use meaningful variable names and maintain a consistent style; it makes your code easier to read.
- Modularize your code: Break down large programs into functions or classes to enhance readability and reusability.
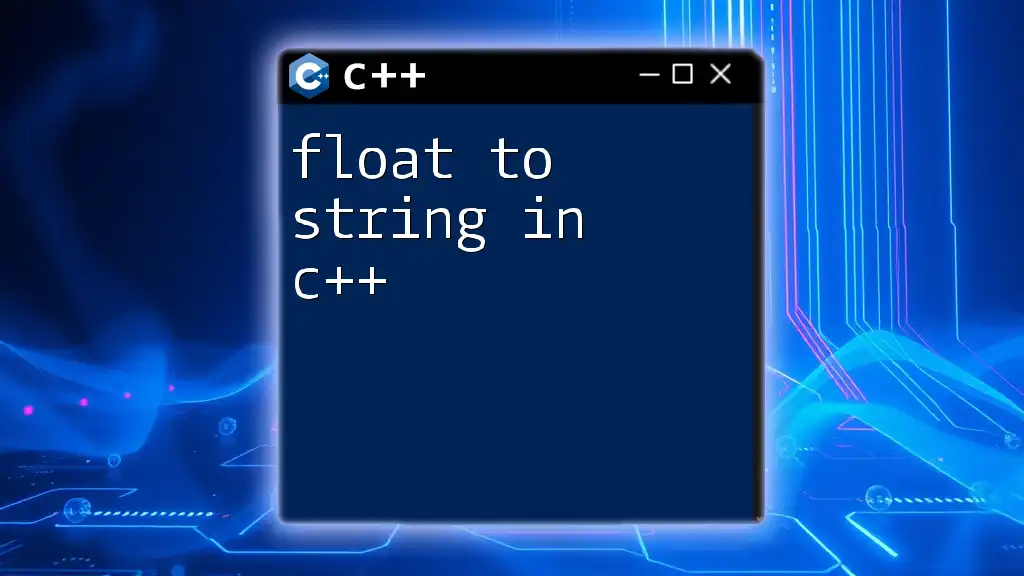
Resources for Further Learning
Online Tutorials and Courses
As you progress, you may want to tap into additional resources. Websites like:
- Codecademy
- Udemy
- Coursera
offer courses tailored to both beginners and advanced C++ programmers.
Books and Publications
Reading books can deepen your understanding and provide structured learning. Some recommended titles include:
- "C++ Primer" by Stanley B. Lippman
- "Effective C++" by Scott Meyers
- "The C++ Programming Language" by Bjarne Stroustrup
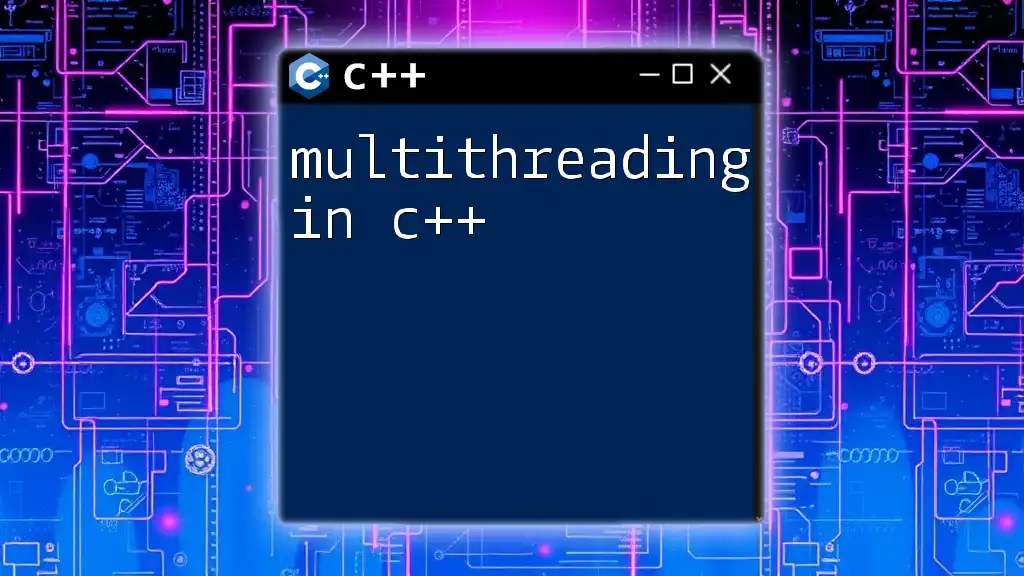
Conclusion
By now, you should have a solid start on how to start coding in C++. From setting up your environment to understanding basic syntax, control structures, functions, and OOP concepts, you have the foundational knowledge required to progress further in your programming journey. Remember, the key to mastering programming is consistent practice and continual learning. Embrace the challenges ahead, and happy coding!