To input a string in C++, you can use the `std::cin` function along with the `std::string` type to read user input.
#include <iostream>
#include <string>
int main() {
std::string input;
std::cout << "Enter a string: ";
std::cin >> input;
std::cout << "You entered: " << input << std::endl;
return 0;
}
Understanding Strings in C++
Definition of Strings in C++
In C++, a string is fundamentally a sequence of characters. This can be represented in two primary forms: C-style strings and C++ STL (Standard Template Library) strings.
- C-style strings are essentially arrays of characters terminated with a null character (`'\0'`).
- C++ STL strings, represented by the `std::string` class, provide a more flexible and abstract way to handle text, allowing for dynamic size, easier manipulation, and built-in functions.
Example of both types:
char cString[] = "Hello, C-style!";
std::string cppString = "Hello, C++!";
Difference Between C-style Strings and C++ Strings
The most notable difference lies in memory management and capabilities. C-style strings require you to manage memory manually and are prone to buffer overflows if not handled carefully. In contrast, C++ strings (i.e., `std::string`) automatically manage memory, resize themselves as needed, and come packed with helpful methods for string manipulation.
For example, consider the following ways C++ strings are more convenient:
- Automatic memory management: You don't need to specify a size in advance.
- Built-in functionalities: Methods like `length()`, `substr()`, and `find()` greatly simplify operations.
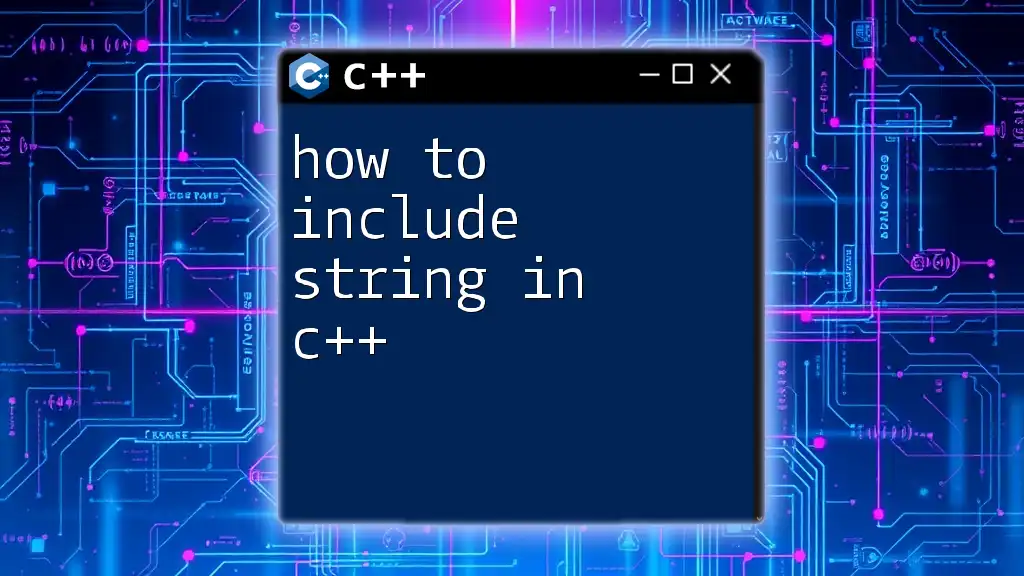
Methods for Inputting Strings in C++
Using `cin`
One of the simplest ways to handle input in C++ is through the `std::cin` stream. Using `cin` to input strings is straightforward but does come with its limitations—most importantly, it stops reading at the first whitespace.
Here’s how you can use it:
std::string name;
std::cout << "Enter your name: ";
std::cin >> name; // Stops at the first whitespace
In this example, entering "John Doe" will only accept "John" as the input because `cin` stops reading at the space.
Using `getline()`
When you need to capture an entire line—including spaces—the `std::getline()` function is your best friend. It allows you to input strings without limitations on whitespace.
To use `getline()` effectively, consider the following:
std::string fullInput;
std::cout << "Enter a full sentence: ";
std::getline(std::cin, fullInput);
Unlike `cin`, `getline` will accept "John Doe" in its entirety.
Reading Strings with Specific Length
Sometimes, it’s necessary to limit the string length to enhance security or conform to specific requirements. You can achieve this using `getline()` in combination with a specified length.
Here’s how to read input while enforcing a maximum length:
std::string limitedInput(100, '\0'); // Preallocate 100 characters
std::cout << "Enter input (max 100 characters): ";
std::cin.getline(&limitedInput[0], 100);
This code snippet allows the user to input a string while ensuring it does not exceed 100 characters, preventing buffer overflow and managing user input effectively.
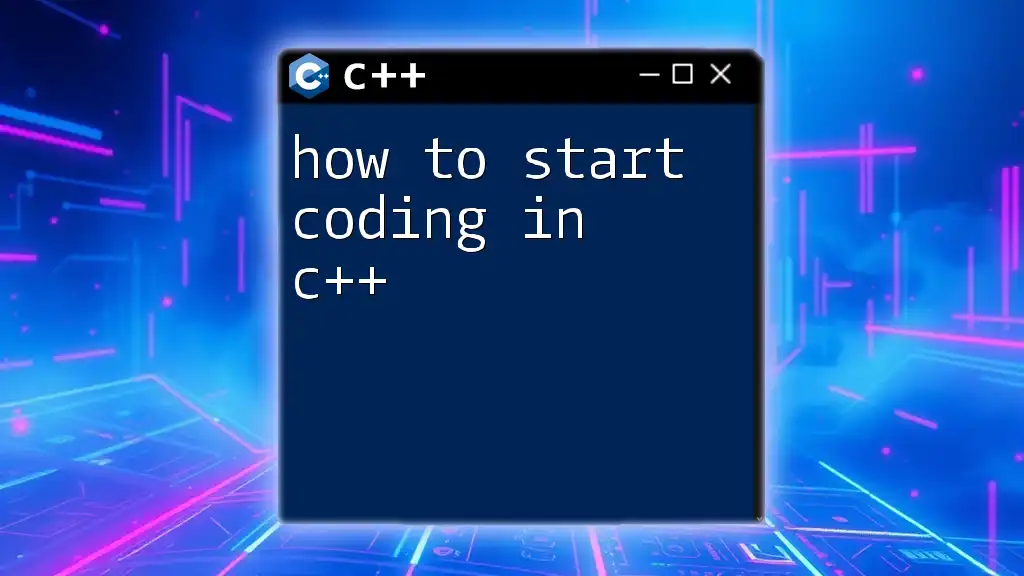
Common Pitfalls in String Input
Handling Whitespace
When mixing `cin` and `getline()`, one common pitfall arises from the handling of whitespace characters. Particularly, if you've used `cin` before calling `getline()`, the newline character left in the input buffer can lead to unexpected behavior.
To mitigate this, use:
std::cin.ignore(); // This ignores the leftover newline character
This command effectively clears out any pending input, ensuring your subsequent `getline()` captures fresh input.
Input Validation
It’s crucial to validate input to ensure that you receive correct and expected data from users. Sometimes, users may enter empty strings or unwanted characters.
Here's a simple loop to verify valid input:
std::string userInput;
while (true) {
std::cout << "Enter a valid input: ";
std::getline(std::cin, userInput);
if (!userInput.empty()) break; // Check for empty input
}
This approach ensures you prompt the user repeatedly until they provide a satisfactory response, promoting robust data handling in your applications.
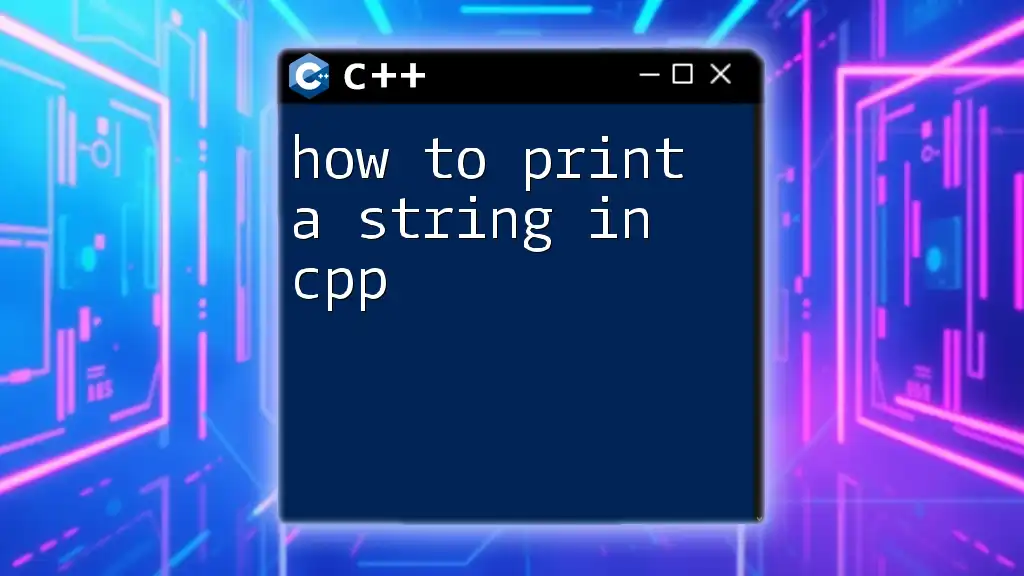
Conclusion
Understanding how to input string in C++ is essential for effective programming. By mastering different methods like `cin` and `getline()`, and recognizing common pitfalls, you’ll be able to handle user input with confidence.
Practice these examples, experiment with variations, and feel free to explore advanced data handling techniques to enhance your skills in string management further. Whether for simple applications or intricate systems, string input is a fundamental skill to polish in your C++ journey.
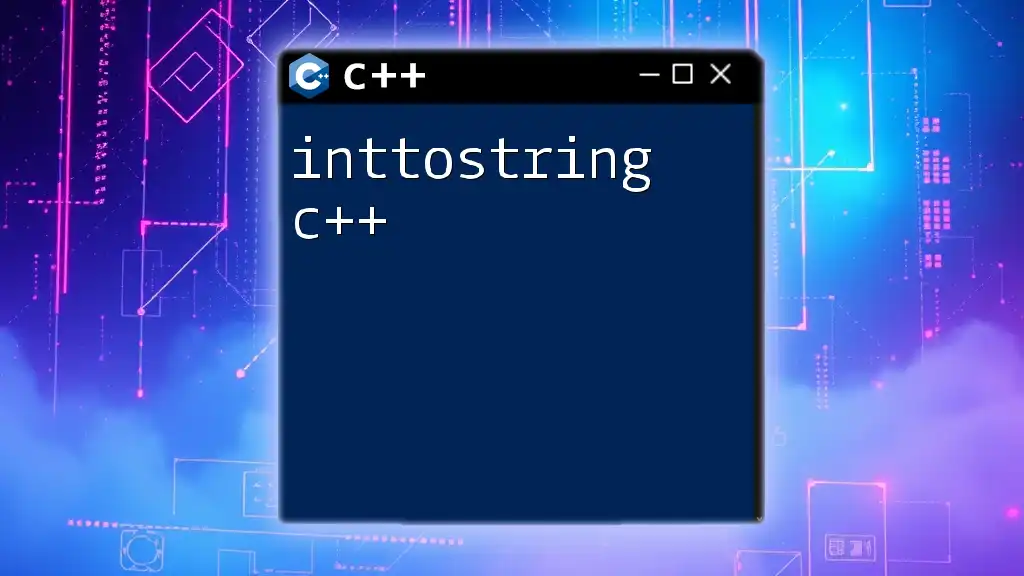
Further Resources
To deepen your understanding of string input in C++, consider exploring books, online courses, or forums dedicated to C++. Additionally, consult cppreference.com for comprehensive documentation that can aid in your learning.