To type the mathematical constant π (pi) in C++, you can use the predefined constant `M_PI` from the `cmath` library, as shown in the following code snippet:
#include <iostream>
#include <cmath>
int main() {
std::cout << "Value of pi: " << M_PI << std::endl;
return 0;
}
Understanding Pi and Its Importance in C++
What is Pi?
Pi (π) is a mathematical constant that represents the ratio of a circle's circumference to its diameter. This irrational number has a decimal expansion that never ends or repeats, beginning with the classical value of approximately 3.14159. Pi plays a vital role in various calculations involving geometry, trigonometry, and physics, making it essential for developers who work with mathematical problems.
The Role of Pi in Programming
Constants like Pi are crucial in programming, as they provide a reliable reference point for numerous mathematical operations. Whether you’re calculating the circumference of a circle in a geometry application or simulating waveforms in a physics engine, having access to Pi can significantly simplify your code and improve accuracy. Real-world applications of Pi in programming include:
- Geometry calculations (area, perimeter, etc.)
- Physics simulations (e.g., rotation, oscillation)
- Engineering applications (e.g., design of circular structures)
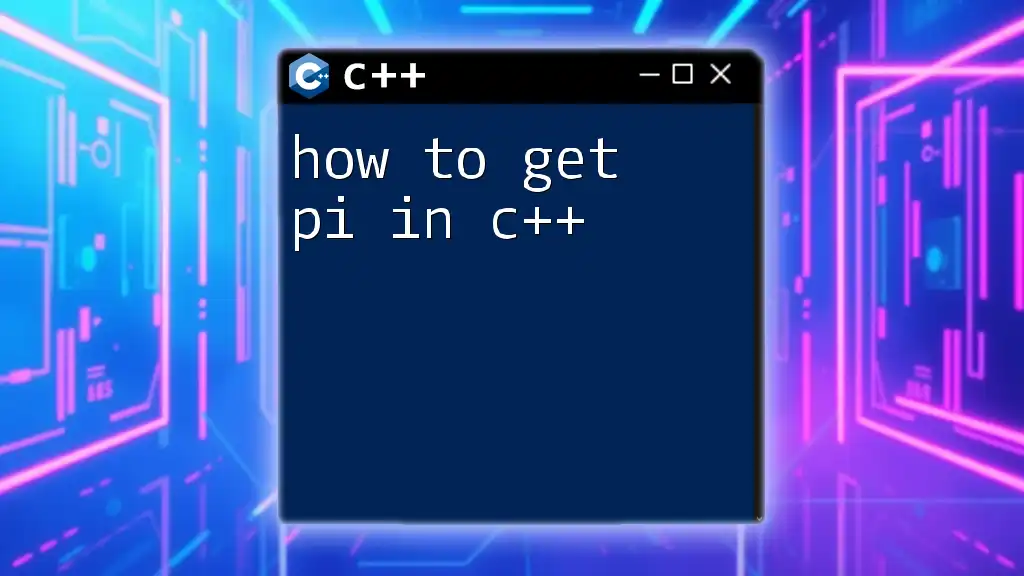
How to Use Pi in C++
Introduction to Mathematical Constants in C++
To make use of Pi in C++, you must be familiar with the `cmath` library, which provides a range of mathematical functions and constants. This library simplifies your coding needs and improves readability by eliminating repetitive calculations.
Using Pi as a Constant
Defining Pi in Your Code
You have the option to define the Pi constant directly within your code. This is a straightforward way to ensure that all calculations using Pi reference the same value. Here's how you can do it:
const double PI = 3.141592653589793;
In this example, the keyword `const` indicates that the value of PI cannot be modified after it is defined. This practice helps prevent accidental changes, ensuring accuracy throughout your code.
Using C++ Built-in Constants
In C++17 and later, you can take advantage of predefined constants available in the `<numbers>` header. This approach gives you a built-in representation of Pi with improved precision:
#include <numbers>
double pi = std::numbers::pi;
This method reduces the risk of introducing errors from manual definitions and centralizes the management of mathematical constants within the standard library.
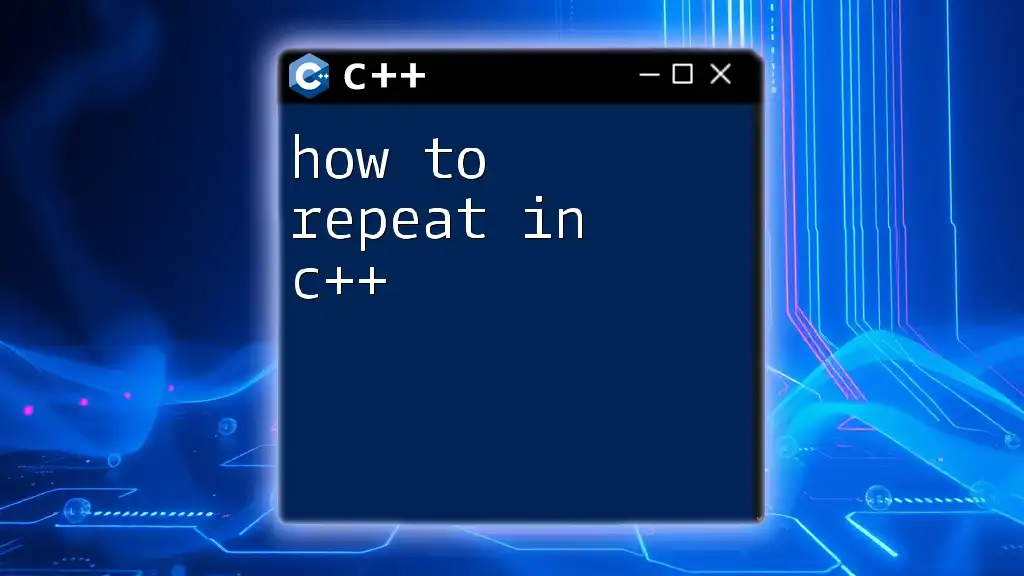
Practical Applications of Pi in C++
Calculating the Circumference of a Circle
Using Pi in calculations is most commonly demonstrated when determining the circumference of a circle. The formula for calculating circumference is:
Circumference = 2 * π * radius.
Here's a code example that illustrates this:
#include <iostream>
const double PI = 3.141592653589793;
double calculateCircumference(double radius) {
return 2 * PI * radius;
}
int main() {
double radius = 5.0;
std::cout << "Circumference: " << calculateCircumference(radius) << std::endl;
return 0;
}
In this code snippet, we define a function `calculateCircumference` that takes the radius as an argument and returns the calculated circumference. The `main` function demonstrates how to use this function, producing an output of the circumference based on the radius provided.
Calculating the Area of a Circle
Similar to calculating circumference, Pi is also used to find the area of a circle. The formula for area is:
Area = π * radius².
Here's how you can implement this formula in your code:
double calculateArea(double radius) {
return PI * radius * radius;
}
This function follows the same logic as the circumference calculation, showcasing the versatility of the Pi constant in various mathematical functions.
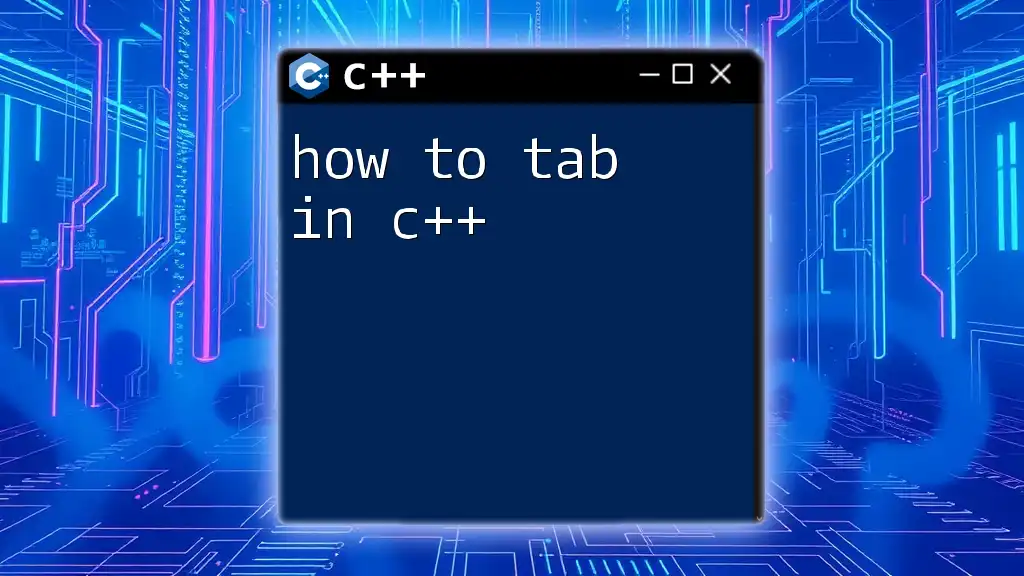
Best Practices in Using Pi in Your Code
Avoid Hardcoding Values
One of the essential best practices when programming is to avoid hardcoding numerical constants. Instead, define a constant for Pi and use it throughout your code. This approach enhances clarity and maintainability; if you ever need to update the value of Pi, you can do so in one place.
Use of Standard Libraries
Utilizing standard libraries is another excellent practice. Not only does it improve the readability of your code, but it also reduces the chance of human error. Incorporating libraries like `<numbers>` allows you to leverage built-in constants, potentially increasing precision without additional effort on your part.
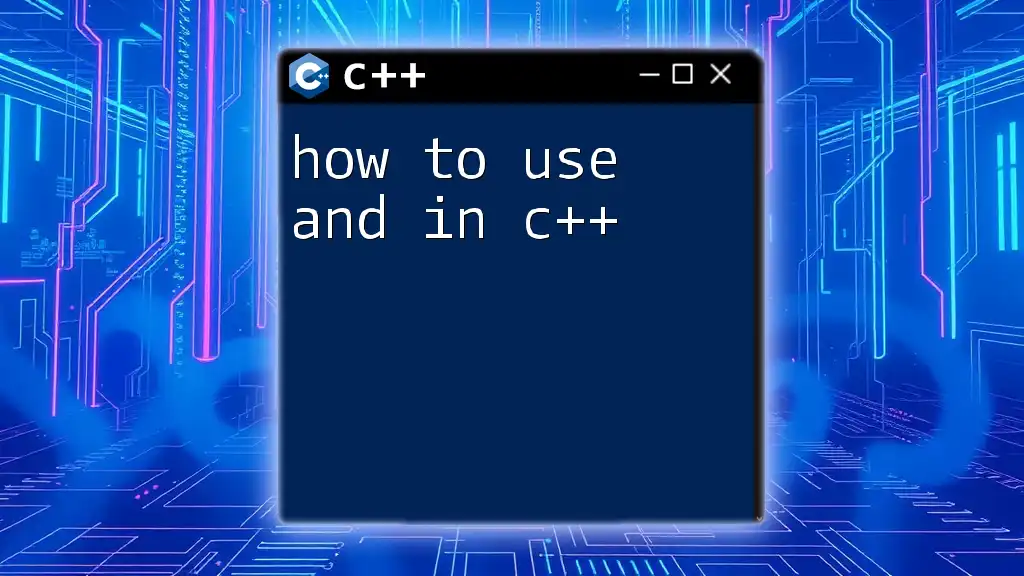
Conclusion
In summary, learning how to type pi in C++ opens up a wealth of opportunities for mathematical computations in your programming projects. By defining Pi as a constant or using built-in library access, you can perform complex calculations with confidence and accuracy. As you explore more advanced applications, think about how you can incorporate Pi into your various programming endeavors.
Feel free to experiment with these examples and explore additional resources for further learning about the fascinating world of C++ programming!