To run a C++ program, you first need to compile your code using a C++ compiler, such as g++, and then execute the generated executable file.
Here's a concise example:
// Compile the program
g++ -o myProgram myProgram.cpp
// Run the program
./myProgram
Understanding C++ and Its Development Environment
What is C++?
C++ is a powerful, high-level programming language that has become a foundational element in software development. Initially developed by Bjarne Stroustrup in the late 1970s, C++ extends the capabilities of the C language, providing features like classes, inheritance, and polymorphism. Its versatility makes it suitable for a wide array of applications, including systems programming, game development, and real-time simulation.
Setting Up Your C++ Environment
To start programming in C++, it’s essential to have a proper development environment set up.
Choosing a Compiler: Compilers are essential tools that translate your C++ code into machine language. Some popular compilers include:
- GCC (GNU Compiler Collection): Commonly used in Linux environments.
- Clang: Known for its speed and useful diagnostics.
- MSVC (Microsoft Visual C++): A staple for Windows application development.
Installing a Compiler: Here’s how to install GCC, for instance:
- Windows: Use MinGW or Cygwin for a UNIX-like environment.
- macOS: Install Homebrew and use the command `brew install gcc`.
- Linux: Use the package manager to install GCC by running `sudo apt-get install g++`.
Integrated Development Environments (IDEs): While you can write C++ code in text editors, IDEs like Code::Blocks, Visual Studio, and CLion provide features such as debugging tools and syntax highlighting that ease the coding experience.
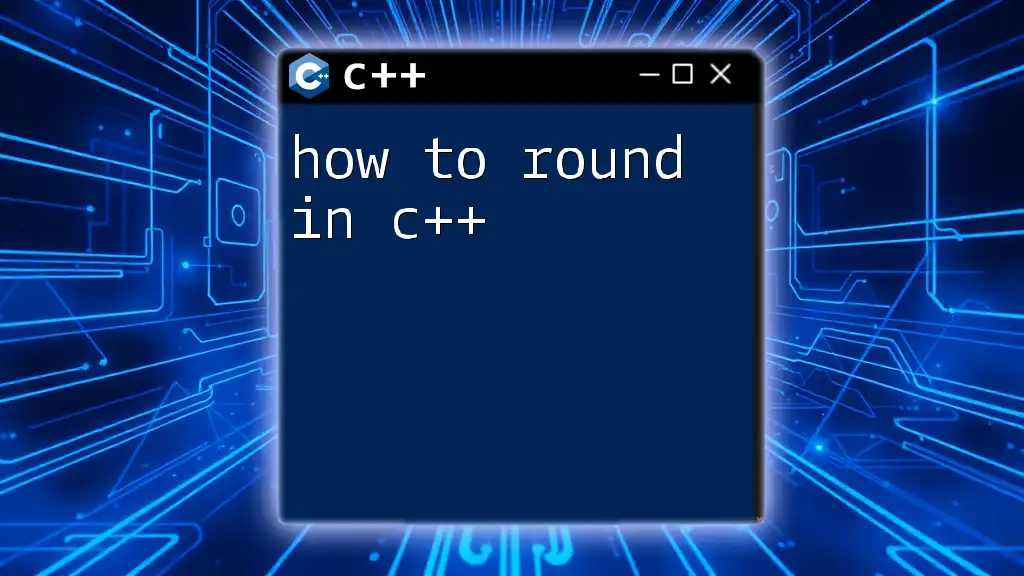
Writing a Simple C++ Program
Basic Structure of a C++ Program
Before running any code, it's crucial to understand its structure. A basic C++ program consists of header files, the main function, and necessary syntax. Here’s a template illustrating this:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This simple program demonstrates the basic setup, where `#include <iostream>` allows the program to use input-output functions.
Saving Your C++ File
Once the code is complete, it’s vital to save your file with the .cpp extension, such as `hello_world.cpp`. This informs the compiler that you are working with C++ code.
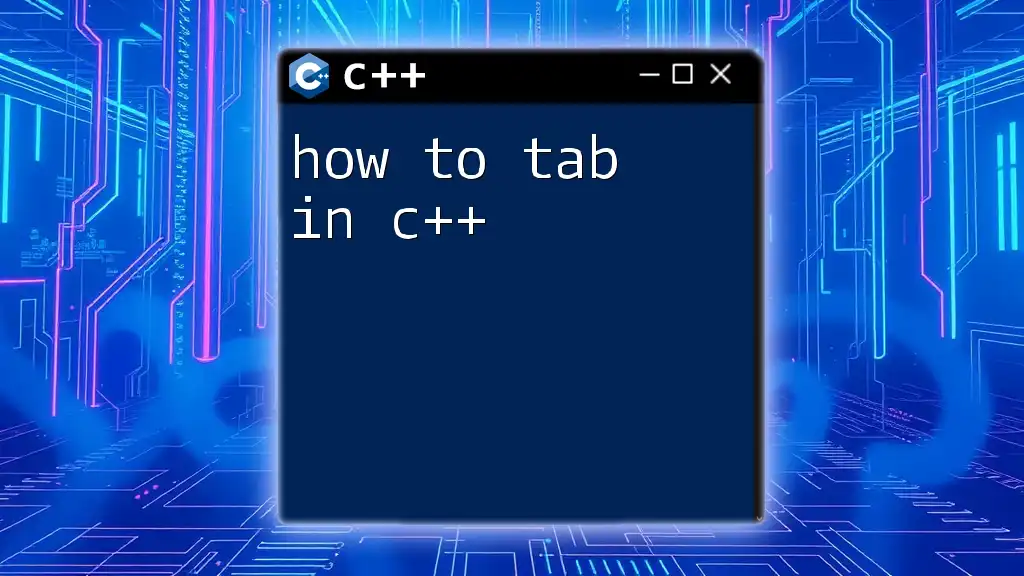
Compiling and Running C++ Code
What Does It Mean to Compile?
Compiling is the process of transforming your C++ code into machine-readable format. The compiler checks for errors, optimizing and producing an executable file that the system can run.
How to Compile C++ Files in Terminal
To compile a C++ file using GCC, you would use the terminal. The general command is:
g++ hello_world.cpp -o hello_world
In this command:
- `g++` is the compiler.
- `hello_world.cpp` is your source file.
- `-o hello_world` specifies the name of the output file.
How to Run C++ Program in Terminal
Once compiled, you can execute the program. For UNIX-like systems, run:
./hello_world
For Windows, you would execute:
hello_world.exe
Expected Output: Upon execution, the terminal should display "Hello, World!", indicating that the program ran successfully.
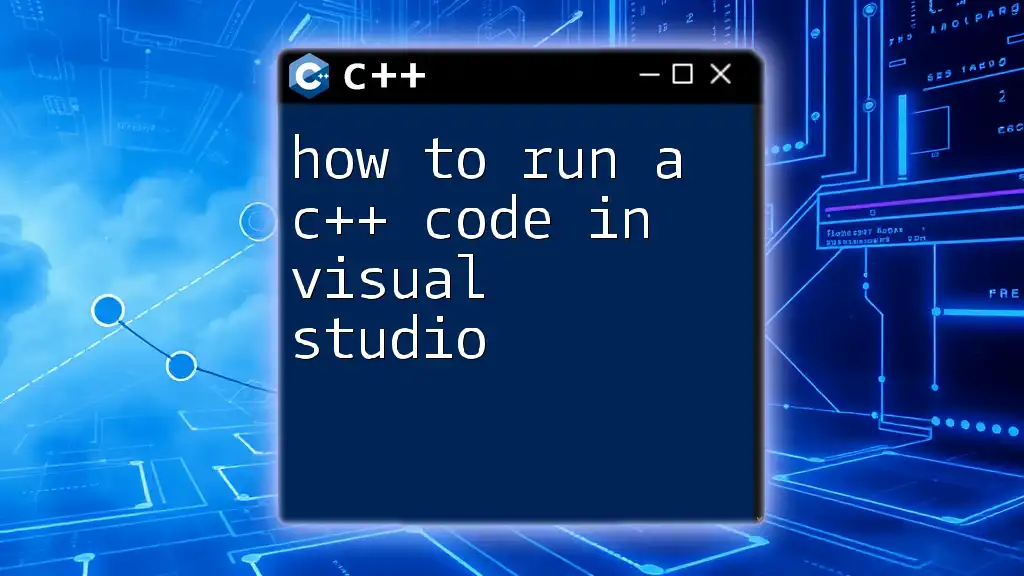
Running C++ Files in Different Environments
How to Run C++ Files in Linux and macOS Terminal
The steps to compile and run C++ files in Linux or Mac are similar:
- Compile:
g++ my_program.cpp -o my_program
- Run:
./my_program
How to Run C++ Files in Windows Command Prompt
In Windows, ensure you are in the directory containing your `.cpp` file, then compile and run using:
- Compile:
g++ my_program.cpp -o my_program
- Run:
my_program.exe
This will execute your compiled C++ code within the command prompt.
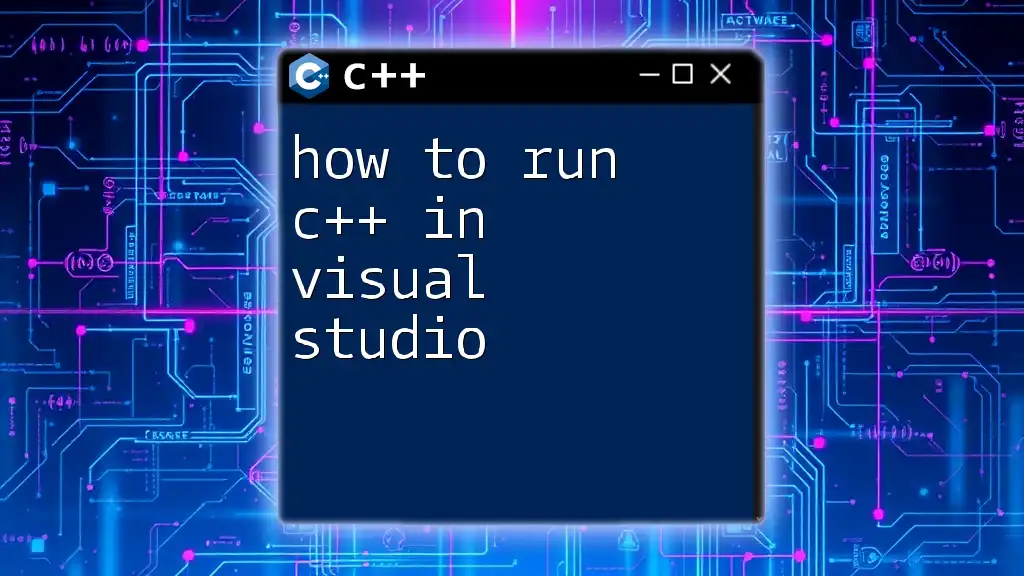
Troubleshooting Common Issues
Common Compilation Errors
Compilation errors can arise due to syntax mistakes, missing semicolons, or mismatched types. Here are some common errors:
- Syntax Error: Often indicated by red lines in your IDE, ensure all syntax is correct.
- Linker Errors: These occur if you've declared functions but failed to define them. Fix these by ensuring all functions in your code are properly defined.
Runtime Errors
Runtime errors happen during program execution, possibly due to logic errors like division by zero or accessing elements out of an array's bounds. Utilize debugging tools in your IDE or add print statements in your code to track down the issues.
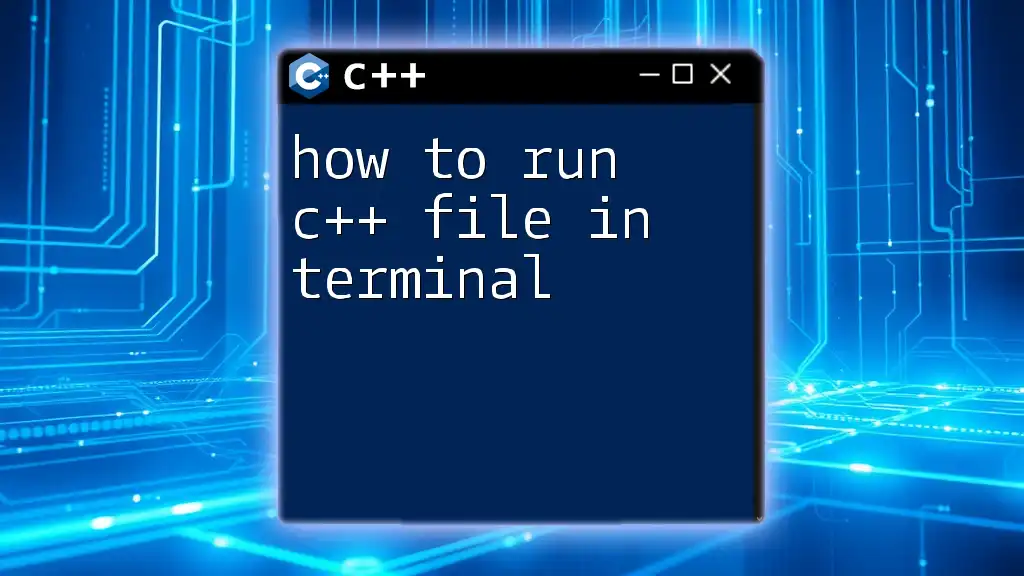
Additional Tips and Best Practices
Improving Your C++ Coding Skills
To enhance your C++ skills:
- Online Courses: Platforms like Coursera and Udemy have comprehensive C++ courses.
- Books: Look for recommendations like "The C++ Programming Language" by Bjarne Stroustrup.
- Forums and Communities: Engage in communities like Stack Overflow for discussions and troubleshooting tips.
Advanced: Build and Execute C++ Files
For larger projects, managing compilation through build systems or `Makefile` can simplify the process:
all: my_program
my_program: my_program.o support.o
g++ -o my_program my_program.o support.o
my_program.o: my_program.cpp
g++ -c my_program.cpp
support.o: support.cpp
g++ -c support.cpp
Using `make`, you can compile your entire project with a single command, which optimizes development efficiency.
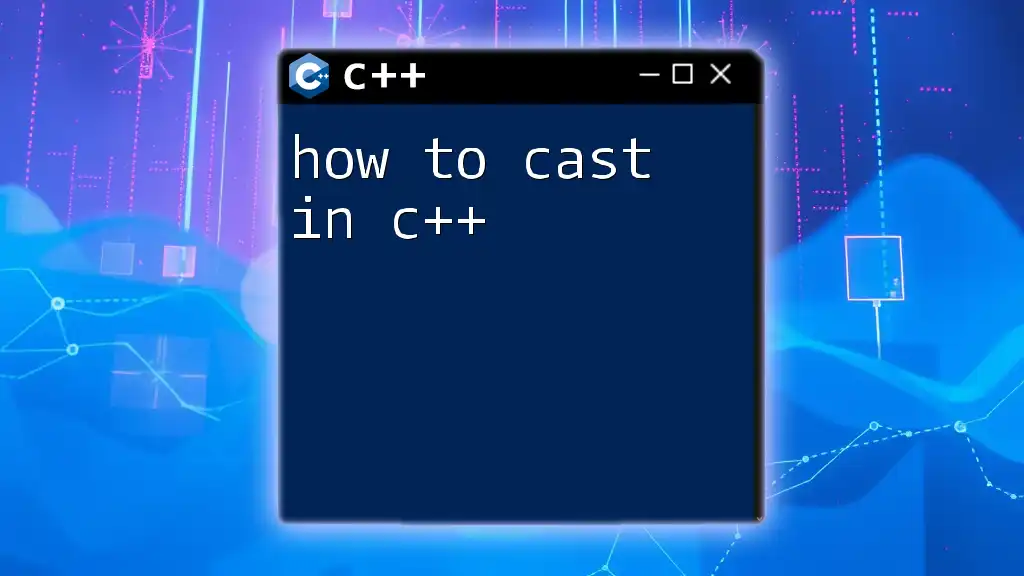
Conclusion
Recap of Key Concepts
Through this guide, you’ve learned not only how to run in C++ but also about the essential tools and structures necessary for programming. By practicing the different commands, compilation processes, and executions, you can confidently start your journey into C++ programming.
Call to Action
Share your experiences with running C++ code in the comments. Don't forget to follow for more tutorials and in-depth articles that will continue to elevate your programming skills!