To run a C++ program in Visual Studio, create a new project, write your code in the editor, and click on the 'Start' button or press F5 to execute it. Here's a simple example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Your Environment
Installing Visual Studio
To get started, the first step is to download and install Visual Studio. Visit the [Visual Studio website](https://visualstudio.microsoft.com/) and choose the version that best suits your development needs (Community, Professional, or Enterprise). For most beginners and learners, the Community version is free and fully featured.
During the installation process, make sure to select the C++ development workload. This option ensures that all necessary tools for C++ programming are installed, including the C++ compiler and libraries.
Creating Your First C++ Project
Once Visual Studio is installed, you will need to create a new C++ project. Open Visual Studio and navigate to the home window. Click on "Create a new project."
In the “Create a new project” dialog, you can apply filters to find the right template by selecting "C++" from the languages dropdown. Choose "Console App" as this is the most common project type for simple C++ programs.
After selecting the template, you'll be prompted to give your project a name and specify its location. Once this is set up, click on "Create".
Now, you are ready to start coding in Visual Studio!
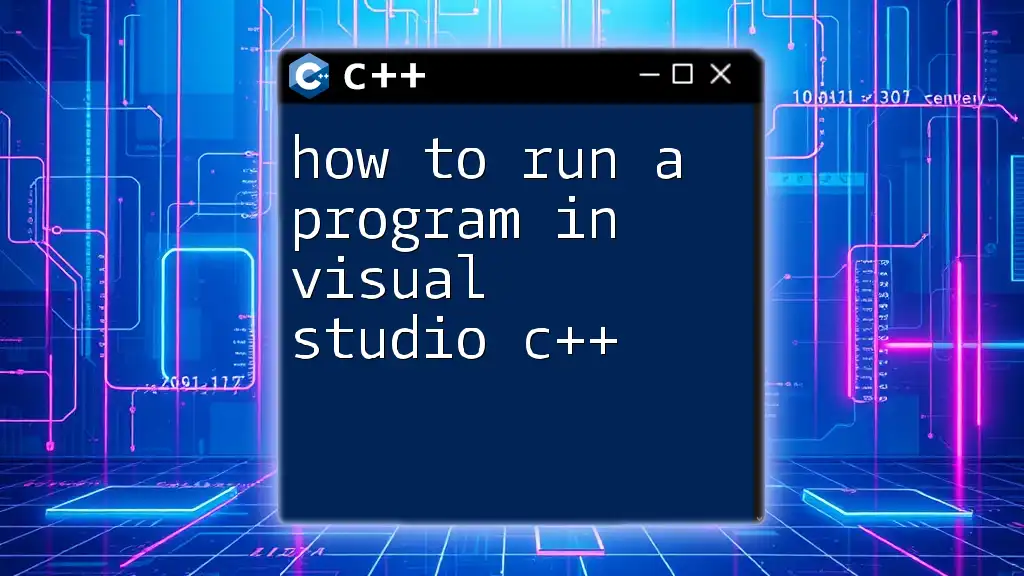
Writing a Simple C++ Program
Basic C++ Program Structure
To understand how to run a C++ program in Visual Studio, it's essential to know the basic structure of a C++ program. The elements typically include `#include`, the `main()` function, and the `return` statement.
Here’s a simple program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Adding Your Code in Visual Studio
With your project created, you should see a editor interface open. The main `.cpp` file (likely named `ProjectName.cpp`) will already be open. You can simply copy and paste the example code above into the code editor. Take a moment to review each line:
- `#include <iostream>` allows the program to use input and output stream objects, such as `std::cout`.
- The `main()` function serves as the entry point of every C++ program.
- `std::cout << "Hello, World!" << std::endl;` prints the string to the console and ends the line.
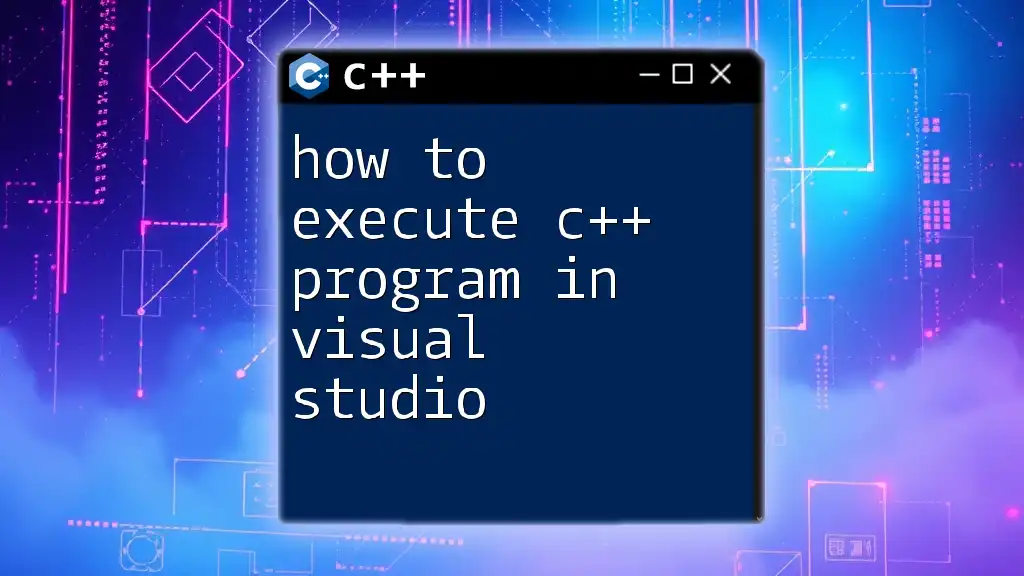
Building Your C++ Program
Understanding the Build Process
When you’re ready to see your program in action, you need to build it. The build process compiles the code into an executable (.exe) file. There are typically two configurations available: Debug and Release.
- Debug Mode: Ideal for development, it includes debugging support, allowing you to troubleshoot your code.
- Release Mode: Optimized for performance, this configuration is used for the final product with fewer debugging tools.
Building in Visual Studio
To build your program, navigate to the Menu Bar at the top and select Build > Build Solution. This process compiles your code.
If there are any errors in your code, they will be displayed in the Error List panel. A successful build will show a message indicating a successful build; make sure to address errors accordingly.
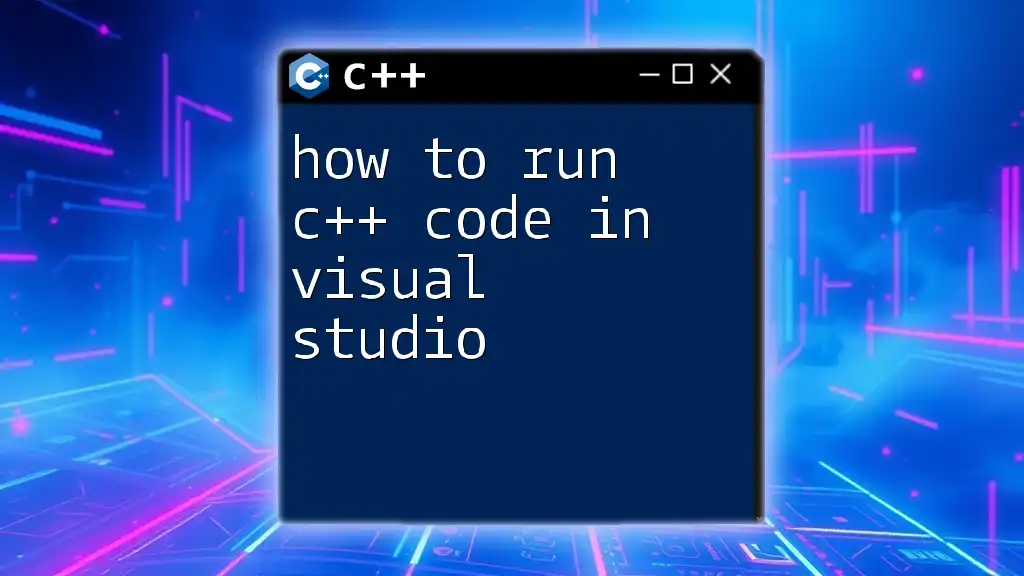
Running Your C++ Program
Executing Your Program in Debug Mode
To run your program in Debug Mode, press F5 or click on the green Start Debugging button in the toolbar. This action launches your program, allowing you to see the output in the console window.
If everything is set correctly, you should see:
Hello, World!
This means your program has compiled successfully and executed as intended!
Running Your Program in Release Mode
Once you are comfortable with Debug Mode, you might want to test your program in Release Mode to see how it performs. To switch to Release Mode, look for the configuration dropdown in the toolbar (this is usually set to Debug) and select Release.
After switching, repeat the previous step by pressing F5 or choosing Start Without Debugging (Ctrl + F5). This will run the executable without the debug tools active, allowing you to witness its performance in a real-world scenario.
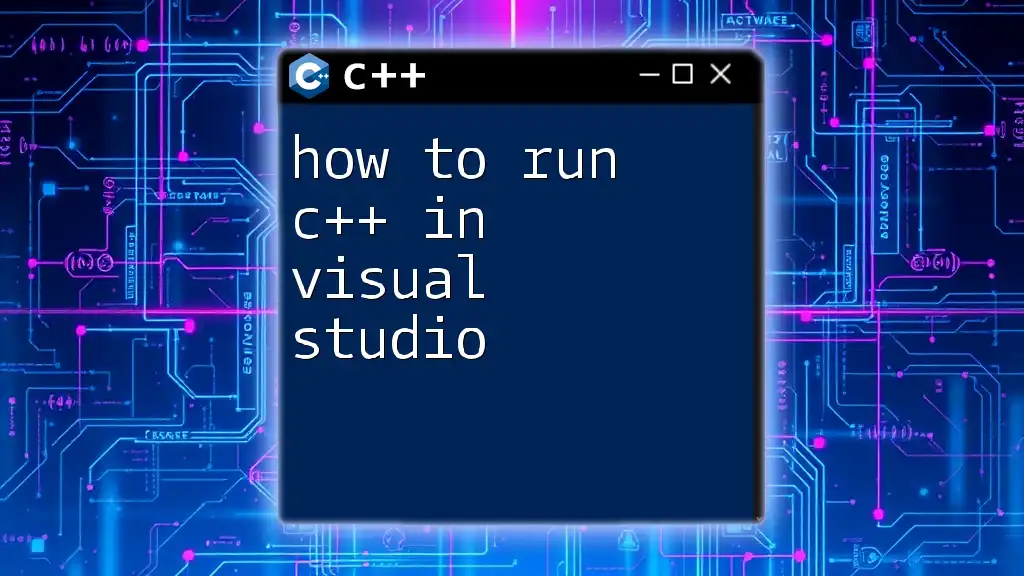
Debugging Your C++ Program
Features of the Visual Studio Debugger
When programming, encountering bugs is inevitable. Thankfully, Visual Studio comes equipped with an advanced debugging toolset. The debugger allows you to inspect variables, set breakpoints, and watch for changes in real-time.
Using the debugger effectively can greatly enhance your development experience, saving you time in fixing errors.
Step-by-Step Debugging Guide
You can initiate debugging by placing a breakpoint. To do this, simply click in the gray margin to the left of a line number in your code. A red dot will appear, indicating a breakpoint.
When you run the program in Debug Mode, execution will pause at your breakpoint, allowing you to inspect variable values directly. For example, if you place a breakpoint right after defining a variable, you can hover over the variable to see its current value.
If you need to debug the simple program previously mentioned:
int main() {
int x = 0;
std::cout << "Value of x: " << x << std::endl; // Debug and check if x is intended
return 0;
}
This way, when stopped at the breakpoint, you can ensure that `x` is being initialized correctly before it is printed.
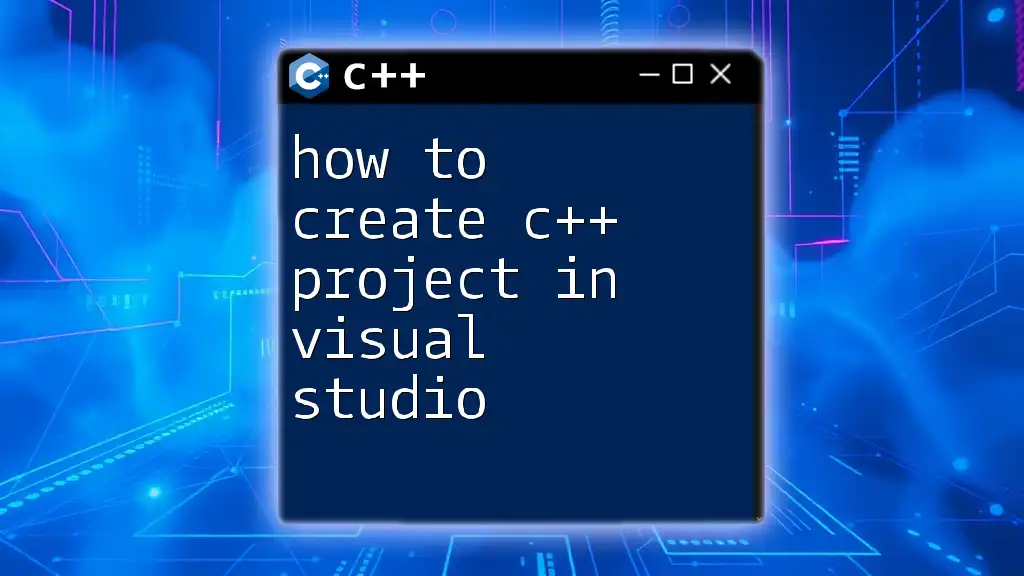
Tips for Efficient C++ Development in Visual Studio
Keyboard Shortcuts for C++ Programming
Familiarizing yourself with keyboard shortcuts can significantly speed up your workflow in Visual Studio. Here are some essential shortcuts:
- F5: Start debugging.
- Ctrl + F5: Run without debugging.
- Ctrl + Shift + B: Build the solution.
- F9: Toggle breakpoint.
Best Practices for Organizing C++ Projects
Maintaining an organized project is crucial. Use clear naming conventions for files and folders. Group related files together to avoid confusion.
Additionally, write comments in your code to explain complex logic; this practice aids both your future self and any collaborators in understanding your work.
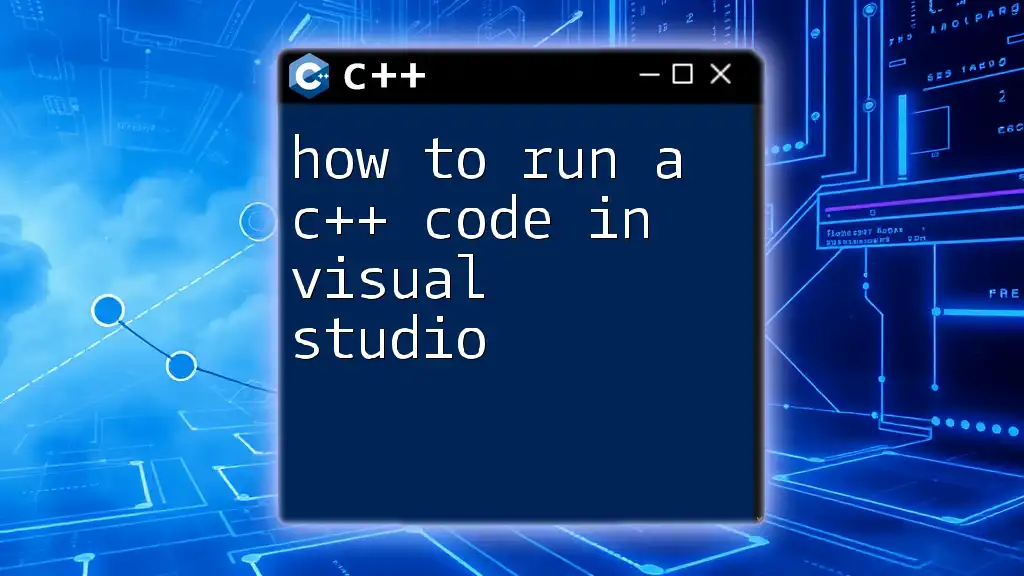
Conclusion
By following this guide on how to run a C++ program in Visual Studio, you've equipped yourself with the fundamental steps necessary to create, build, and execute C++ programs in one of the most powerful IDEs available. Don't hesitate to experiment with more complex C++ projects as you refine your skills!
In case of any lingering questions, you're encouraged to leave comments or reach out for further clarification. Exploring additional resources or tutorials on C++ can also help enhance your learning experience.