To code C++ in Visual Studio, simply create a new C++ project, write your code in the editor, and use the debugger to run and test your program, as shown in the example below:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Setting Up Your Environment
Installing Visual Studio
To start coding in C++, you first need to install Visual Studio. Begin by visiting the official Visual Studio website and selecting the appropriate version for your needs.
The Community Edition is free and ideal for individual developers, students, and open-source projects. In contrast, Professional and Enterprise editions offer additional features for teams and enterprises, but they come at a cost.
Follow these steps to install Visual Studio:
- Download the Installer: Click on the download button for the Community Edition.
- Run the Installer: Open the downloaded file and follow the prompts.
- Select the Workloads: When prompted to choose workloads, ensure you select the Desktop Development with C++ workload. This option installs all the necessary tools for C++ development.
Selecting the C++ Workload
A workload in Visual Studio groups related tools and packages together. For C++ programming, selecting the "Desktop Development with C++" workload will install components like the C++ compiler, libraries, and tools essential for building applications.
Make sure to review any optional components that may further enhance your C++ development environment.
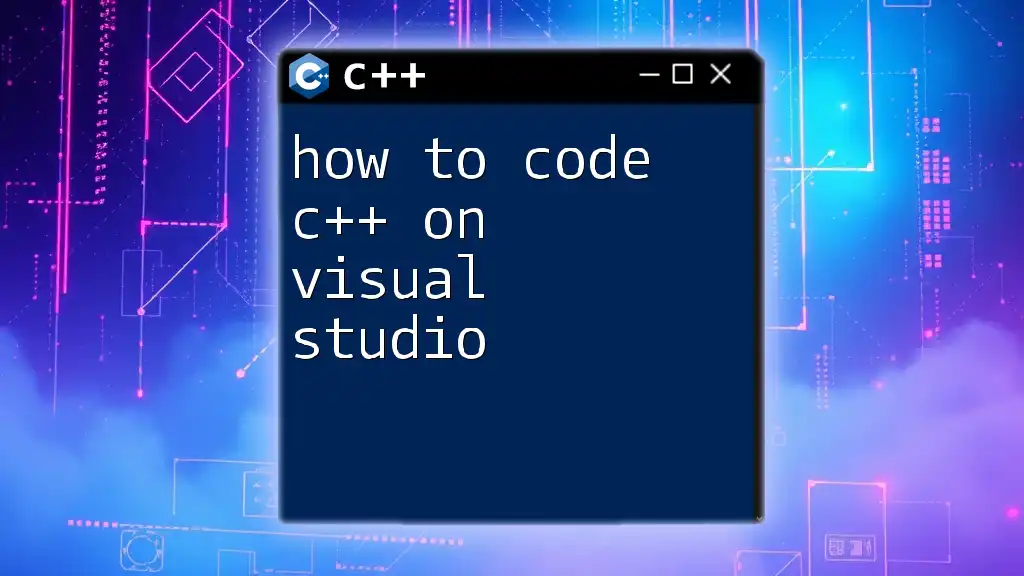
Creating Your First C++ Project
Launching Visual Studio
Once your installation is complete, launch Visual Studio from your applications menu or desktop shortcut. You will be greeted with the Start Window, where you can manage your projects.
Starting a New Project
To create a new C++ project:
- Click on the Create a new project button.
- In the project template selection, type “C++” in the search bar.
- Choose C++ Console Application from the list.
- Click Next.
Now, you can name your project. For example, you might call it `HelloWorld`. Choose a suitable location for your project files, and click Create. Visual Studio will generate a basic project structure for you.
Understanding Project Structure
Your newly created project will contain various files:
- Source Files: This is where you write your C++ code, typically with a `.cpp` extension.
- Header Files: Files with a `.h` extension used for declarations and definitions.
- Solution Files: A `.sln` file that organizes your projects and their configurations within Visual Studio.
Familiarize yourself with the Solution Explorer on the right side of the screen. It allows you to navigate through your project files easily.
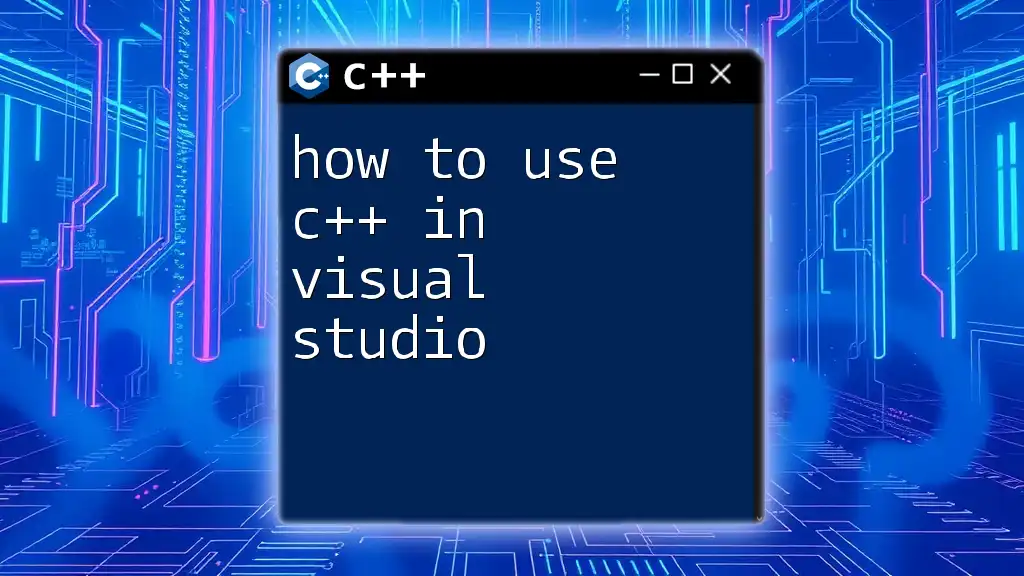
Writing Your First C++ Program
Basic Structure of a C++ Program
Every C++ program consists of a main function, where execution begins. Here’s a simple structure of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Adding Your Code
Open the `main.cpp` file (or similar) that Visual Studio created for you. Delete any default code inside and copy the provided code snippet into it.
This code includes the necessary header file and uses `std::cout` to print "Hello, World!" to the console. The `return 0;` statement indicates that the program has ended successfully.
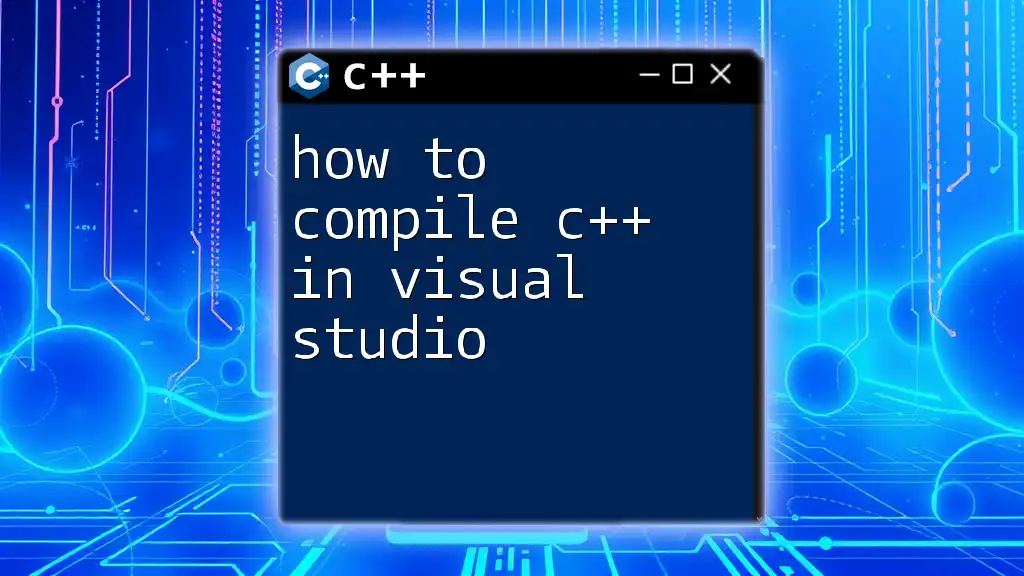
Compiling and Running Your Program
Understanding the Build Process
C++ code must be compiled into an executable format before it can run. When you hit the Build button (or press `Ctrl + Shift + B`), Visual Studio compiles your code.
- Compiling converts your source code into object code.
- Linking combines the object code with libraries to produce an executable.
Running Your Program
After successfully building your project, you can run it:
- Click on the Start button (green arrow) or press `Ctrl + F5` to execute your program.
- The console window will pop up displaying "Hello, World!"
Take a moment to observe the Output Window; it shows the results of your program or any compilation errors that may occur.
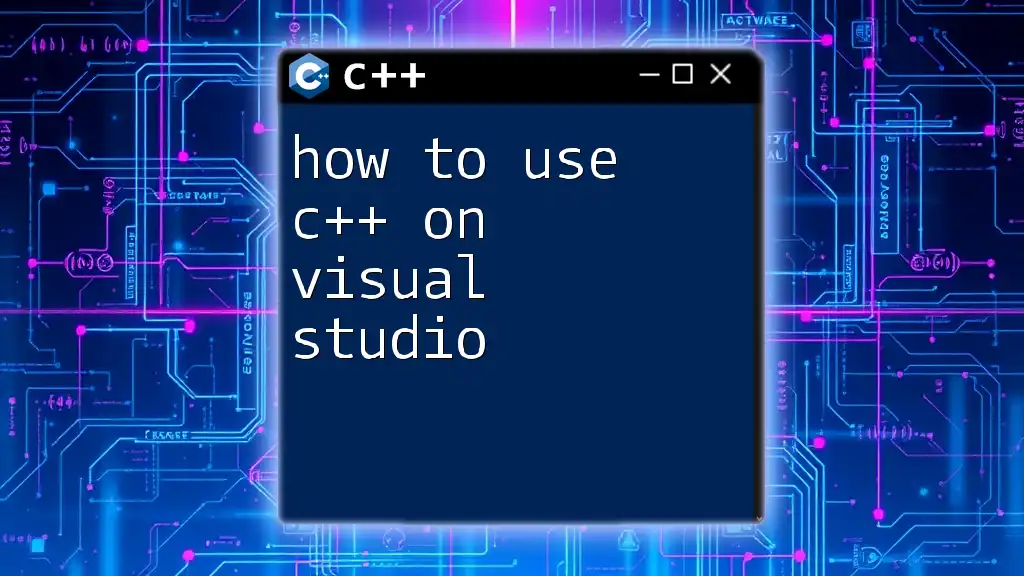
Debugging Your C++ Code in Visual Studio
Introduction to Debugging
Debugging is an essential part of software development. It involves identifying and fixing errors in your code. Visual Studio provides powerful debugging tools to help you troubleshoot issues efficiently.
Using Breakpoints
Breakpoints allow you to pause program execution at a specific line of code so you can inspect variables and the program's state. To set a breakpoint:
- Click in the left margin next to the line of code where you want to pause.
- A red dot will appear, indicating a breakpoint is set.
Next, run your program in debug mode by pressing `F5`. The execution will stop at your breakpoint, allowing you to investigate your program's behavior.
Inspecting Variables and Using Watches
While paused, you can inspect variable values in the Locals window. You can also add variables to a Watch:
- Right-click on a variable and select Add Watch.
- The Watch window will display the variable's current value, helping you identify issues.
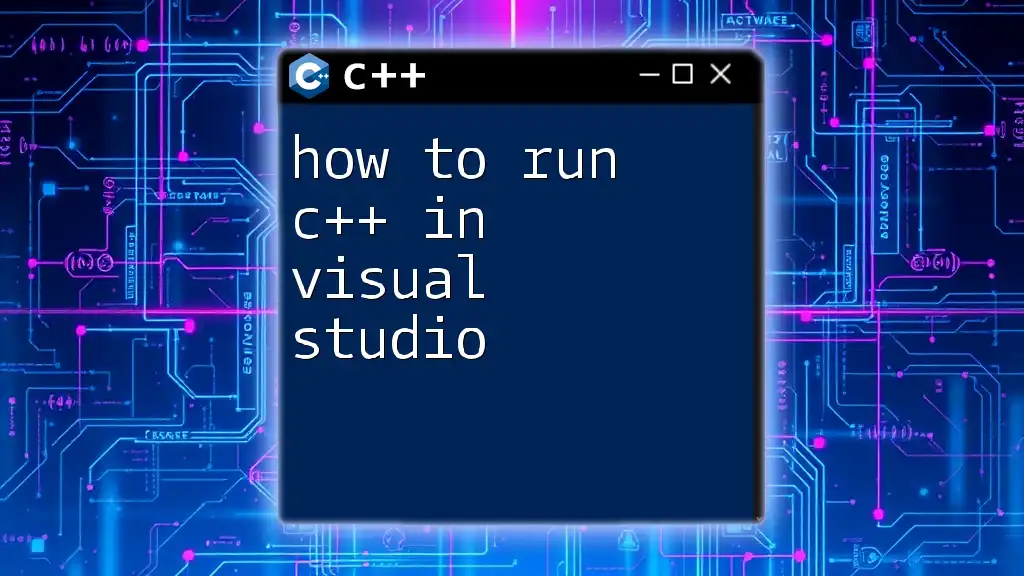
Enhancing Your C++ Skills
Utilizing IntelliSense
IntelliSense is a feature in Visual Studio that provides intelligent code suggestions, autocompletion, and inline documentation. This tool is incredibly valuable as you write C++ code, helping you learn syntax and available functions more efficiently.
Start typing a command, and IntelliSense will display relevant options. This can save time and reduce errors in your coding.
Exploring Visual Studio Extensions
Visual Studio supports a wide range of extensions that can enhance your C++ development experience:
- Visual Assist: A powerful tool that improves code navigation and refactoring.
- C++ Code Snippets: Helps you easily insert commonly used code patterns.
You can find and install extensions through the Extensions menu within Visual Studio.
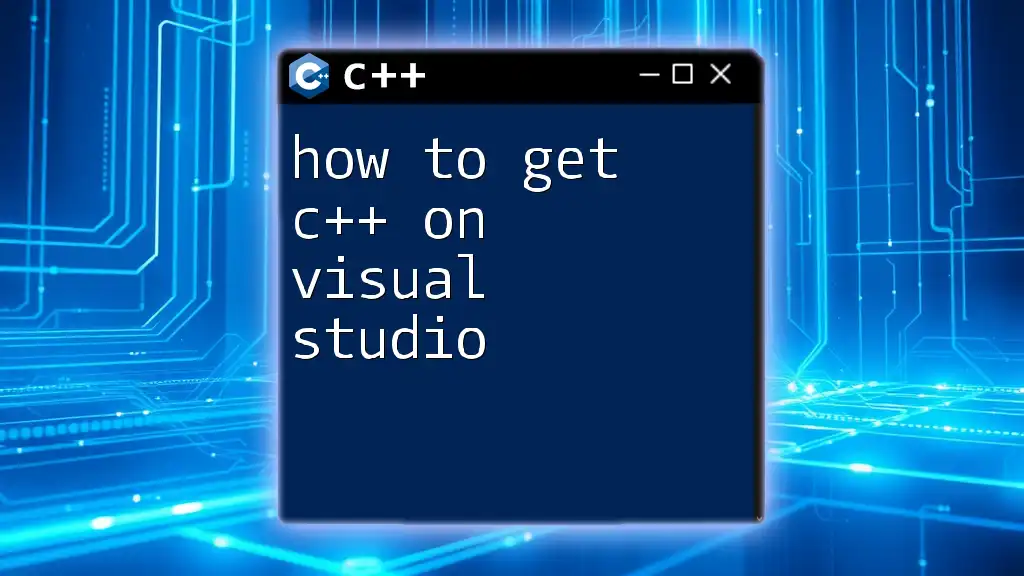
Conclusion
You’ve now learned how to code C++ in Visual Studio, from setting up your environment and creating your first project to running your program and debugging it effectively. Take the time to explore advanced features and continue to build on your C++ knowledge!
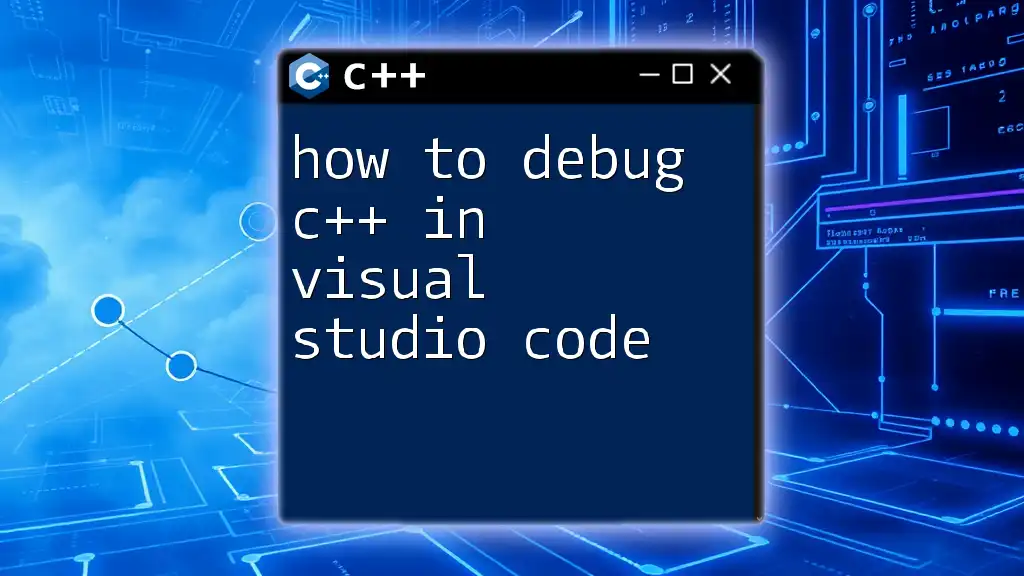
Additional Resources
Learning Platforms for C++
To further enhance your skills, consider enrolling in online courses on platforms like Coursera, Udacity, or Udemy. Many reputable YouTube channels also provide tutorials that can guide you through more intricate concepts in C++ programming.
Community and Support
If you encounter challenges, turn to online forums such as Stack Overflow, the C++ sections on Reddit, or the official Microsoft documentation to seek help and connect with fellow developers. Happy coding!