To install C++ on Visual Studio, download the Visual Studio Installer, select the "Desktop development with C++" workload, and follow the installation prompts.
// Example of a simple C++ program
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Why Choose Visual Studio for C++ Development?
Visual Studio stands out as a premier Integrated Development Environment (IDE) for C++ developers due to its user-friendly interface and robust features. This IDE offers a plethora of tools that enhance productivity and streamline the coding process.
-
User-Friendly Interface: Visual Studio's intuitive layout makes it accessible for beginners while providing powerful capabilities for experienced developers. The organized menu options and easy navigation ensure that users can focus on coding rather than getting lost in settings.
-
Robust Features: Visual Studio comes equipped with features like IntelliSense, which provides code suggestions and context-aware completions, as well as advanced debugging capabilities that simplify identifying and fixing errors in your code. Additionally, project management tools allow you to efficiently organize your work.
-
Community and Support: With an extensive community backing Visual Studio, a vast array of resources—including documentation, forums, and tutorials—are available. This sense of community helps users troubleshoot and find solutions to common problems easily.
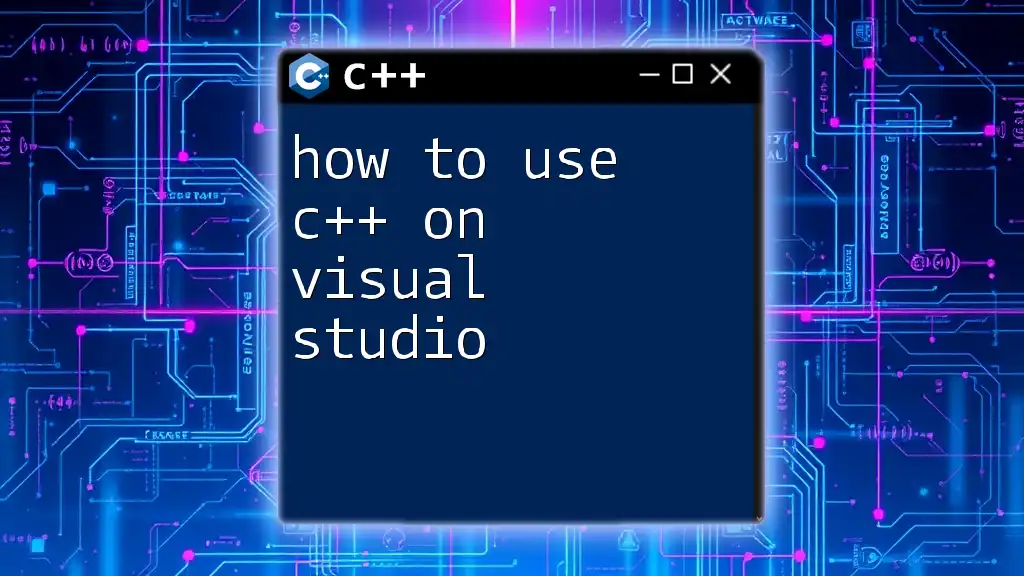
Pre-requisites for Installation
Before diving into the installation process, it’s essential to ensure that your system meets the necessary requirements.
-
System Requirements: Visual Studio has specific minimum and recommended specifications, such as:
- Operating System: Windows 10 or later
- RAM: At least 4 GB of RAM (8 GB recommended)
- Hard Drive: Minimum of 3 GB of available space
-
Account Setup: To download and use Visual Studio, you are required to set up a Microsoft account. This account not only grants you access to the download but also provides additional benefits like cloud services and collaborative tools.
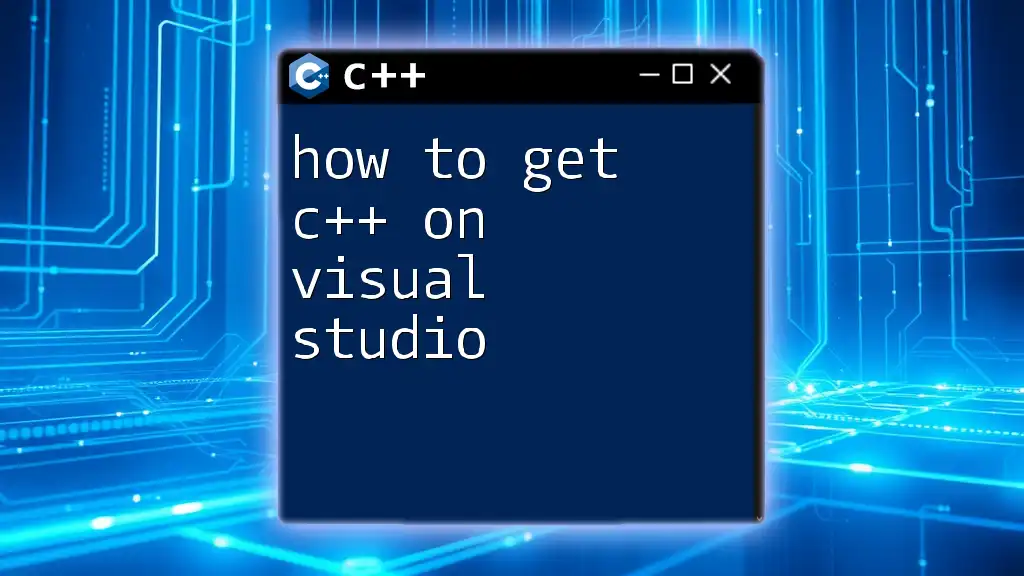
Step-by-Step Guide to Install Visual Studio
Downloading Visual Studio
To begin, navigate to the official Visual Studio [download page](https://visualstudio.microsoft.com/). Here, you will find different versions of Visual Studio: Community, Professional, and Enterprise.
- Choosing the Right Version: For beginners or individual developers, the Community edition is an excellent choice as it is free and contains most of the essential features you will need for C++ development.
Installation Process
-
Run the Installer: After downloading the installer, locate the downloaded file (typically in your Downloads folder) and double-click to run it. This action starts the installation process.
-
Select Workloads: Once the installer is up, you'll see an options menu with different workloads.
- Choosing C++ Development: Check the box for Desktop development with C++. This selection will install the necessary tools, libraries, and templates required for C++ programming.
- Additional Components: You might also want to select other optional components like the Windows 10 SDK and C++ CMake tools for Windows for a more comprehensive development environment.
-
Installation Location and Options: If you wish to change the default installation path, you can do so in this step. Customizing other installation settings is also available, allowing you to tailor the installation according to your preferences.
-
Complete Installation: Click the install button and allow the installer to complete the process. It might take some time depending on your internet connection and hardware capabilities.
Initial Setup
Once the installation concludes, you will be prompted to launch Visual Studio for the first time.
-
Launching Visual Studio for the First Time: Upon opening, you will encounter a start window with various options for project types. It's an excellent opportunity to familiarize yourself with the interface.
-
Sign In with Microsoft Account: Signing in with your Microsoft account enhances your experience by allowing access to additional features. This includes syncing settings across devices, accessing cloud services, and receiving updates easily.
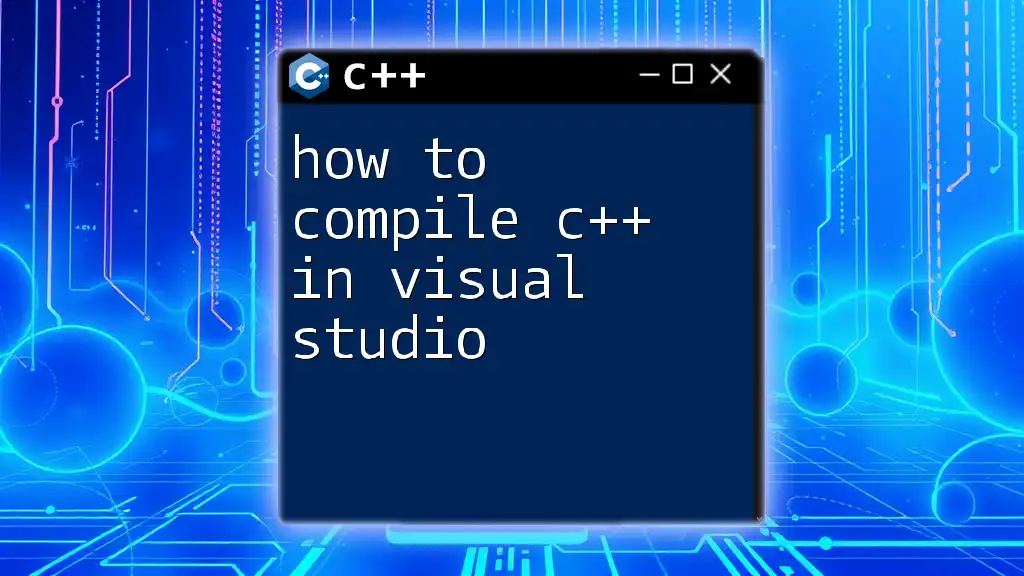
Creating Your First C++ Project
Starting a New Project
To start developing in C++, you first need to create a new project.
-
Navigating the Start Window: The start window of Visual Studio provides you with the option to create a new project, open an existing project, or access recent projects. Click on Create a new project.
-
Creating a Console Application: In the project template search, type "Console App" and select Console App (C++). This template is ideal for writing C++ programs that run in a command-line interface.
Writing Your First C++ Program
Now, let’s write a simple C++ program.
- Code Example: Here’s a classic "Hello, World!" program:
#include <iostream> // Include library for input/output
int main() {
std::cout << "Hello, World!"; // Print the message to the console
return 0; // Return success code
}
In this example, the `#include <iostream>` line is a directive that includes the necessary library for input and output operations in C++. The `main()` function is the entry point of every C++ program.
Building and Running the Project
-
Build the Application: To compile the code, click on the Build option in the menu bar and then select Build Solution. If everything is correct, the system will compile without errors.
-
Running the Application: With the project built, you can now run it. Click on the Start Debugging button or press `F5` on your keyboard. The console window will open, displaying "Hello, World!" on the screen.
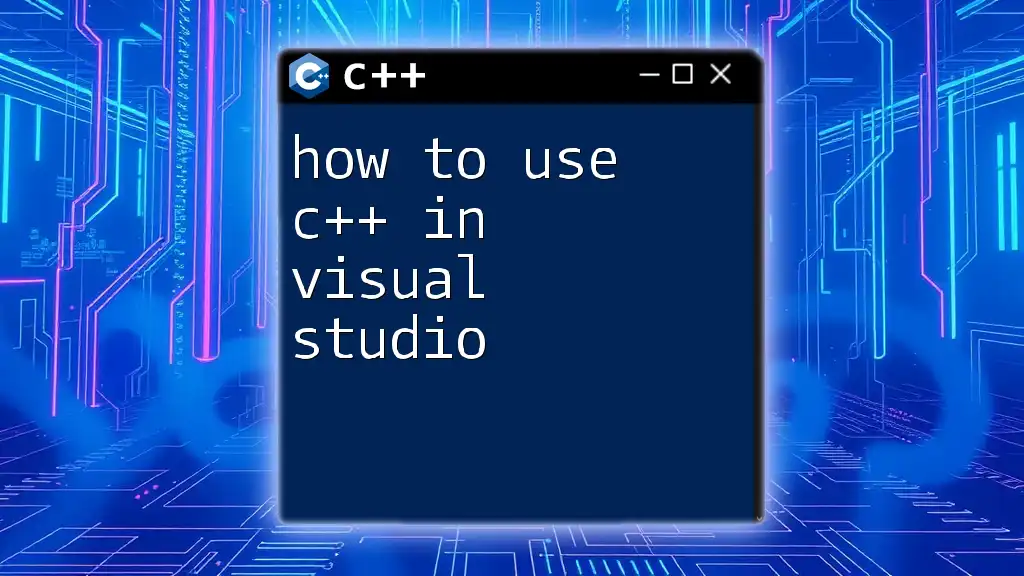
Troubleshooting Common Installation Issues
While installing Visual Studio, you may encounter some issues:
-
Installation Fails: If the installation fails, it could be due to various factors such as insufficient disk space, a slow internet connection, or conflicts with existing software. Make sure your system meets all requirements before retrying the installation.
-
Missing Components: Occasionally, certain components may not install as expected. If you find missing pieces, you can rerun the Visual Studio installer, select the "Modify" option, and ensure that the necessary workloads and components are checked.
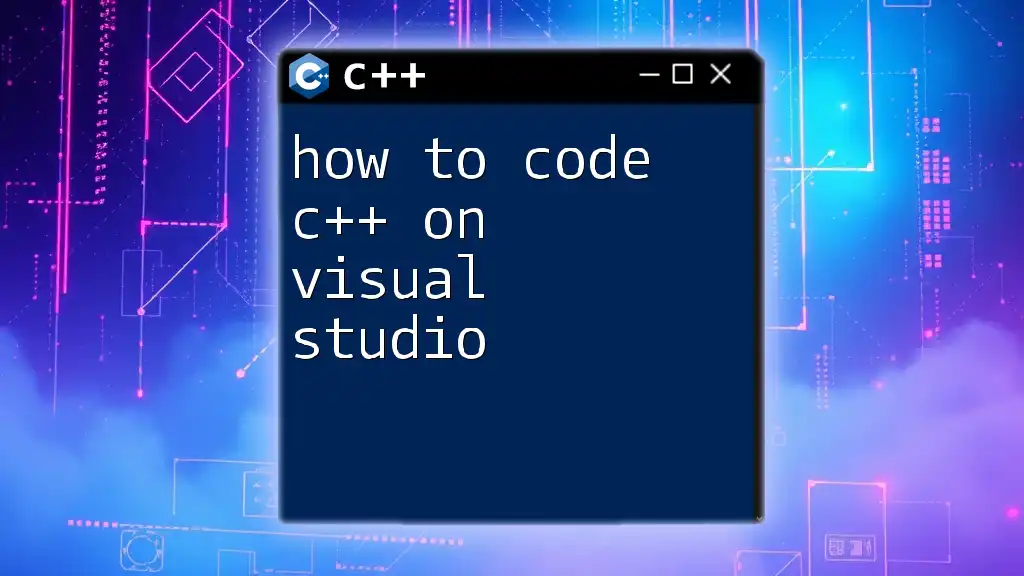
Useful Resources
To further support your journey in learning C++ and using Visual Studio, several resources are available:
-
Documentation: The official Microsoft Visual Studio documentation is an extensive source of information and is invaluable for troubleshooting and understanding features.
-
Community Forums: Engaging with platforms like Stack Overflow and the Visual Studio Community can provide helpful insights and solutions from fellow developers facing similar issues.
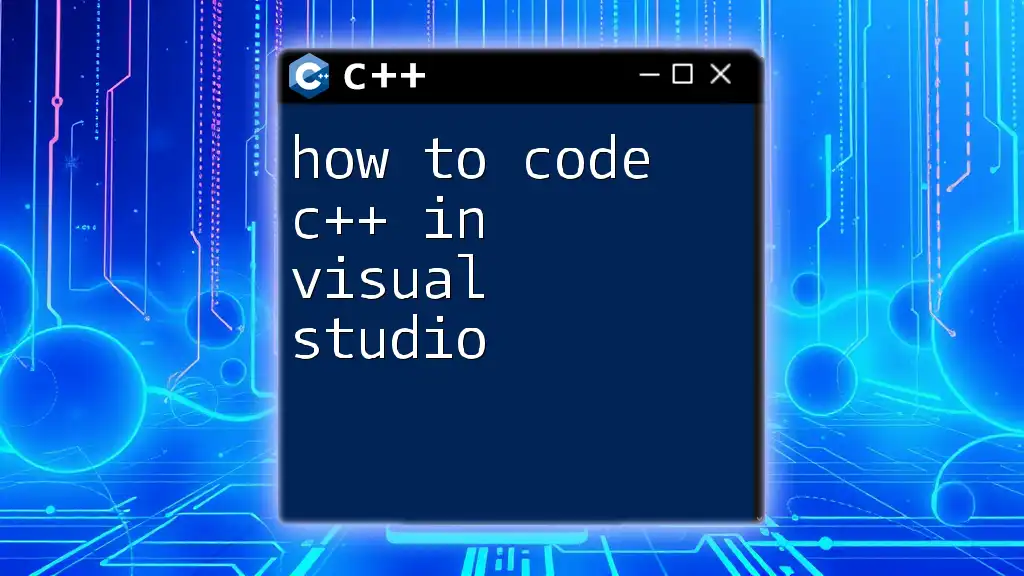
Conclusion
Installing C++ on Visual Studio opens doors to a world of programming possibilities. With its intuitive interface and powerful features, Visual Studio is a wise choice for both beginners and advanced developers.
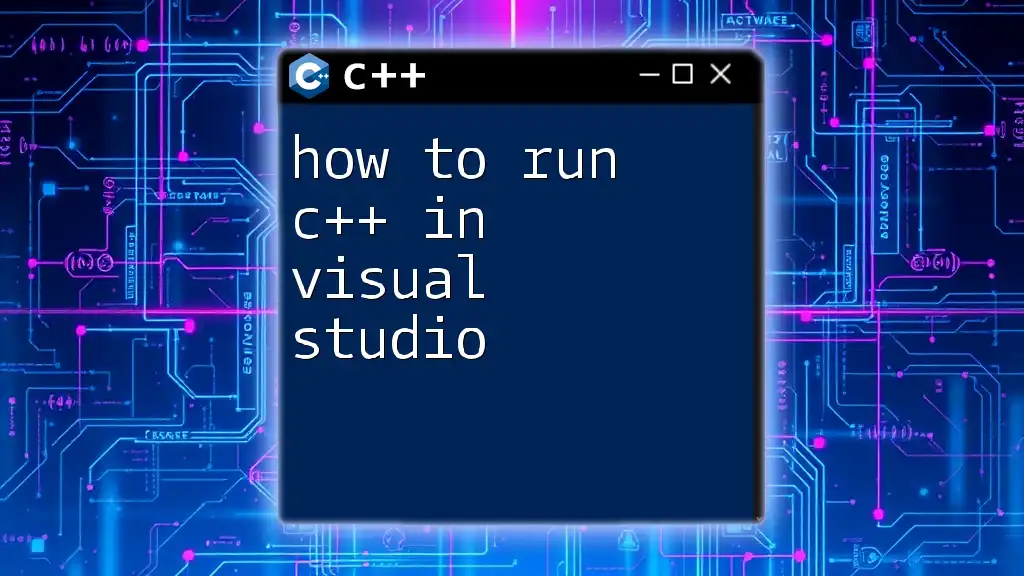
Call to Action
We encourage you to start coding and experimenting with your newfound skills in C++. For more tutorials and guides on C++ programming and Visual Studio, check out additional resources on our website. Happy coding!