To compile a C++ program in Visual Studio, simply open your project, ensure you have a C++ file selected, and use the hotkey Ctrl + F5 or select "Start Without Debugging" from the Debug menu.
Here's a basic code snippet you might compile:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is Visual Studio?
Visual Studio is a powerful Integrated Development Environment (IDE) developed by Microsoft. It provides everything you need to build applications for Windows, the web, and beyond. With extensive support for C++, Visual Studio allows developers to write, debug, and compile their code efficiently. The IDE is renowned for its user-friendly interface, making it an excellent choice for both beginners and seasoned developers.

Why Learn to Compile C++?
Understanding how to compile C++ code is essential for every programmer. Compiling transforms your human-readable code into machine code that a computer can execute. This process allows you to test and verify your programs, ensuring they work as expected before deployment. Moreover, compiling frequently helps you discover errors early in your coding process, making debugging significantly easier.

Getting Started with Visual Studio
Installing Visual Studio
To begin, you'll need to install Visual Studio on your system. Here’s how to do it:
-
Download Visual Studio: Navigate to the official Visual Studio website and choose the version that suits you best (Community, Professional, or Enterprise).
-
Select C++ Workloads: During installation, make sure to select “Desktop development with C++”. This will install the necessary components and libraries required for C++ development.
Creating a New C++ Project
Once you have Visual Studio installed, follow these steps to create a new C++ project:
- Open Visual Studio.
- On the startup screen, select “Create a new project”.
- In the project template search bar, type “C++”. Choose “Console App” from the available templates.
- Give your project a name and specify the location where it should be saved.

Writing Your First C++ Program
The Basic Structure of a C++ Program
Every C++ program starts with the `main` function, which is where execution begins. A typical C++ program includes the following elements:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet:
- `#include <iostream>` is a preprocessor directive that allows you to use input and output features.
- `std::cout` is used to output text to the console.
- The `main` function must return an integer, which signifies successful termination of the program.
Building Your Understanding
After running your first program, experiment by modifying it. For example, try changing the output message or adding new variables to work with. This hands-on practice solidifies your understanding of C++ syntax and structure.

How to Compile C++ in Visual Studio
Compiling C++ with Visual Studio: Step-by-Step
Accessing the Build Menu
In Visual Studio, the Build menu is your primary tool for compiling code. It is located at the top of the window. This menu features options such as Build Solution and Rebuild Solution.
Using the Compile Command
To compile your code, select Build Solution from the menu or use the keyboard shortcut `Ctrl + Shift + B`. This command compiles all files in your project and links them together.
- Build: Compiles only the files that have changed since the last build.
- Rebuild: Cleans and compiles all files, ensuring you have the latest version of all components.
Exploring Keyboard Shortcuts
Mastering keyboard shortcuts can significantly speed up your workflow. Here are a few essential shortcuts for compiling C++ code:
- `Ctrl + Shift + B`: Build the current solution.
- `F5`: Start debugging your application.
- `Ctrl + F5`: Start without debugging.
Understanding Build Configuration
Visual Studio provides several build configurations. The two most crucial are Debug and Release:
- Debug: This configuration is used during the development phase. It includes debugging information and is not optimized for performance.
- Release: This configuration is optimized for execution speed. Use it when you’re ready to deploy your application.
You can switch between these configurations via the dropdown menu in the toolbar.

Handling Compilation Errors
Common Compilation Errors
As you compile your code, you may encounter errors. Some common compilation errors include:
- Syntax Errors: Mistakes in the code structure, such as missing semicolons.
- Undeclared Identifiers: Using variables or functions that have not been declared.
Debugging Compiling Issues
When you encounter a compilation error, the Output Window located at the bottom of the Visual Studio interface provides detailed error messages. Analyzing these messages helps you understand what went wrong.
Look for error codes and descriptions; these will guide you in troubleshooting your code. Learning to interpret these messages is a valuable skill that will improve your coding efficiency.

Advanced Compilation Techniques
Using Command-Line Tools
For those who prefer using the command line, Visual Studio offers a Developer Command Prompt. Here’s how to compile C++ code using it:
- Open the Developer Command Prompt for Visual Studio from the Start Menu.
- Navigate to the directory where your C++ file is located.
- Use the following command to compile:
cl /EHsc my_program.cpp
This command invokes the C++ compiler, `cl`, and `/EHsc` enables exception handling.
Additional Tools within Visual Studio
Using NuGet Packages
Sometimes your C++ projects will require external libraries. NuGet Package Manager in Visual Studio allows you to add these libraries effortlessly. You can search for packages directly from the Manage NuGet Packages option in your project.
Custom Build Configurations
You can create custom build configurations within your project settings. This feature lets you set specific compiler flags or include paths to cater to the needs of your project, making it a versatile tool for advanced compilation tasks.
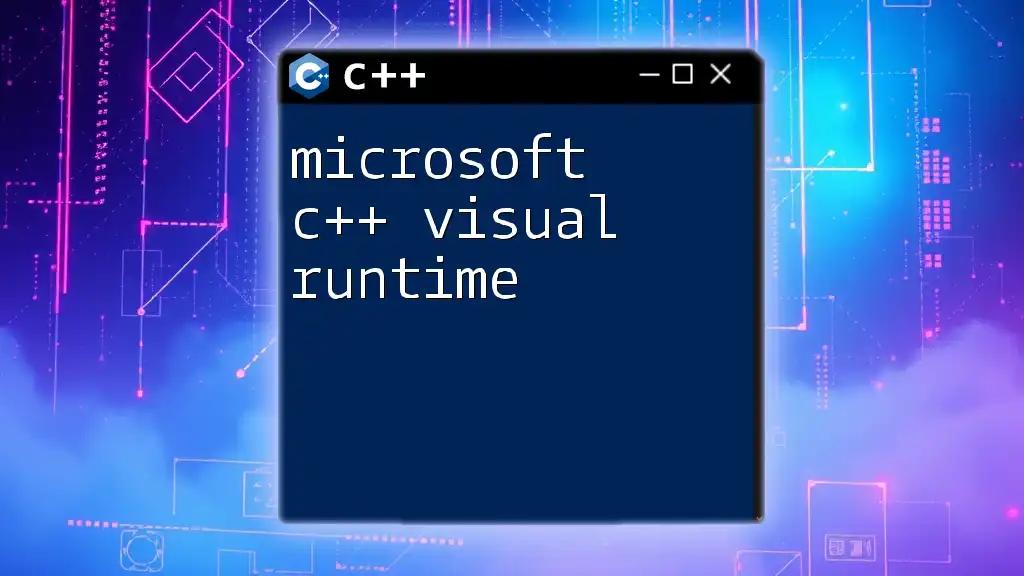
Conclusion
Recap of Key Points
In this guide, we've explored how to compile C++ in Visual Studio, from installation to creating and compiling your first project. We discussed the importance of compiling for testing and debugging, as well as how to handle common compilation errors.
Next Steps for Aspiring C++ Developers
Continuously practicing compiling your C++ code in Visual Studio will significantly enhance your programming skills. Explore additional resources, engage with the developer community, and dive deeper into advanced C++ programming to further your knowledge.
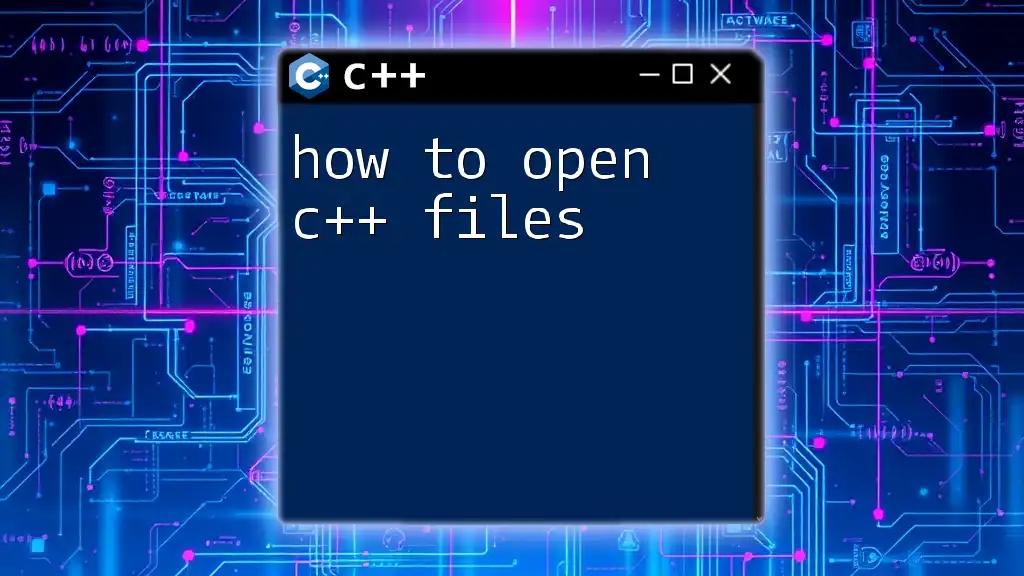
Resources
Additional Learning Materials
Consider exploring online courses, forums, and documentation dedicated to C++ and Visual Studio. Books specifically designed for beginners can also provide a robust foundation for your C++ programming journey.
Engage with the Community
Don’t forget to connect with other C++ developers on platforms like Stack Overflow or specialized C++ forums. Engaging with such communities not only provides support but also opportunities to share knowledge and experiences.