C++ concurrency allows multiple threads to execute simultaneously, improving the efficiency of applications by sharing the workload.
Here’s a simple example demonstrating how to create and run threads in C++:
#include <iostream>
#include <thread>
void hello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(hello); // Create a new thread that runs the hello function
t.join(); // Wait for the thread to finish
return 0;
}
Understanding Concurrency
What is Concurrency?
Concurrency is the ability of a system to handle multiple tasks at once. It allows time-sharing among processes, enabling users to perform multiple operations simultaneously. A crucial distinction to make here is between concurrency and parallelism. While concurrency refers to the structuring of problems to be solved independently, parallelism involves executing multiple operations simultaneously, often on multiple cores. A simple analogy can be understanding concurrency as multitasking—performing several tasks at once—while parallelism can be likened to working with a team where tasks are genuinely tackled simultaneously.
Why Use Concurrency in C++?
In modern C++ applications, concurrency has become essential due to its myriad benefits. Some key advantages include:
- Performance: By running tasks concurrently, applications can significantly reduce execution time, particularly in CPU-bound programs.
- Responsiveness: In UI applications, using concurrency ensures that the interface remains responsive while performing lengthy operations in the background.
- Resource Utilization: Concurrency allows for better utilization of system resources, particularly on multicore CPUs, as multiple threads can operate on different cores.
In various scenarios such as web servers handling multiple client requests or desktop applications offering smooth user interactions, concurrency plays a pivotal role.
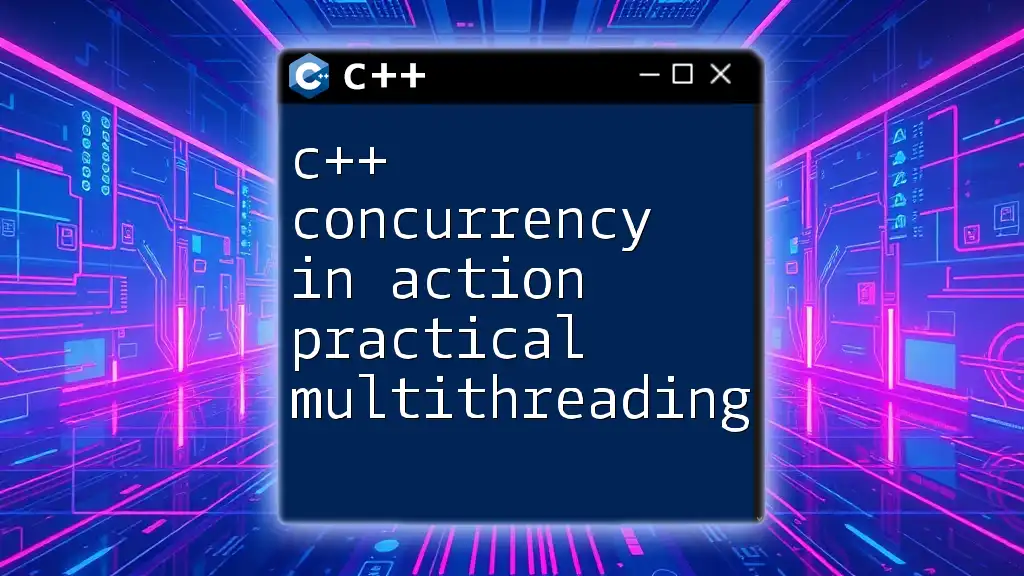
C++ Concurrency Basics
C++ Standard Library Overview
The C++ Standard Library has integrated robust concurrency support since C++11. Some of the critical headers to include when working with concurrency are:
- `<thread>`: Provides the thread functionalities.
- `<mutex>`: Contains classes for mutual exclusion.
- `<condition_variable>`: Allows synchronization between threads.
- `<future>`: Facilitates asynchronous operations and promises.
Threads: The Building Blocks of Concurrency
In C++, threads are foundational for implementing concurrency. You can create and manage threads using the `std::thread` class.
Here's a simple example of thread creation:
#include <iostream>
#include <thread>
void printMessage() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread myThread(printMessage);
myThread.join(); // Wait for the thread to finish
return 0;
}
In this code snippet, we first define a function `printMessage`, which is executed by the thread. The call to `join()` ensures that the main program waits for `myThread` to finish executing before continuing.
It's essential to understand how threads operate and their lifecycle. After creating a thread, you can either join it to ensure synchronization or detach it to let it run independently.
Shared Data and Synchronization
The Need for Synchronization
When working with shared data, multiple threads may attempt to read or modify this data simultaneously, leading to data races. Data races can cause unpredictable behavior or crashes in your programs. Therefore, ensuring thread safety through proper synchronization mechanisms is crucial.
Mutexes and Locks
To prevent concurrent access to shared resources, C++ provides several synchronization tools, such as `std::mutex`. A mutex acts like a lock that ensures only one thread can access the shared resource at any given time. Here is how to use a mutex:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Create a mutex
int sharedResource = 0;
void incrementResource() {
std::lock_guard<std::mutex> lock(mtx); // Lock the mutex
++sharedResource;
}
int main() {
std::thread t1(incrementResource);
std::thread t2(incrementResource);
t1.join();
t2.join();
std::cout << "Shared Resource: " << sharedResource << std::endl;
return 0;
}
Using `std::lock_guard`, we can automatically manage mutex locking and unlocking, which prevents common pitfalls like forgetting to unlock a mutex.
Condition Variables
For more complex scenarios, such as when threads need to wait on certain conditions, condition variables come into play. A condition variable allows a thread to wait until another thread notifies it that it can proceed. Here’s a simplified producer-consumer example:
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <queue>
std::queue<int> dataQueue;
std::mutex mtx;
std::condition_variable condVar;
void producer() {
for (int i = 0; i < 10; ++i) {
std::lock_guard<std::mutex> lock(mtx);
dataQueue.push(i);
condVar.notify_one(); // Notify a waiting thread
}
}
void consumer() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
condVar.wait(lock, [] { return !dataQueue.empty(); }); // Wait for data
int value = dataQueue.front();
dataQueue.pop();
std::cout << "Consumed: " << value << std::endl;
// Exit condition for demonstration (if needed)
if (value == 9) break;
}
}
int main() {
std::thread prod(producer);
std::thread cons(consumer);
prod.join();
cons.join();
return 0;
}
In this example, the producer adds items to a queue, notifying the consumer when data is available. The consumer waits on the condition variable until it can process an item from the queue.
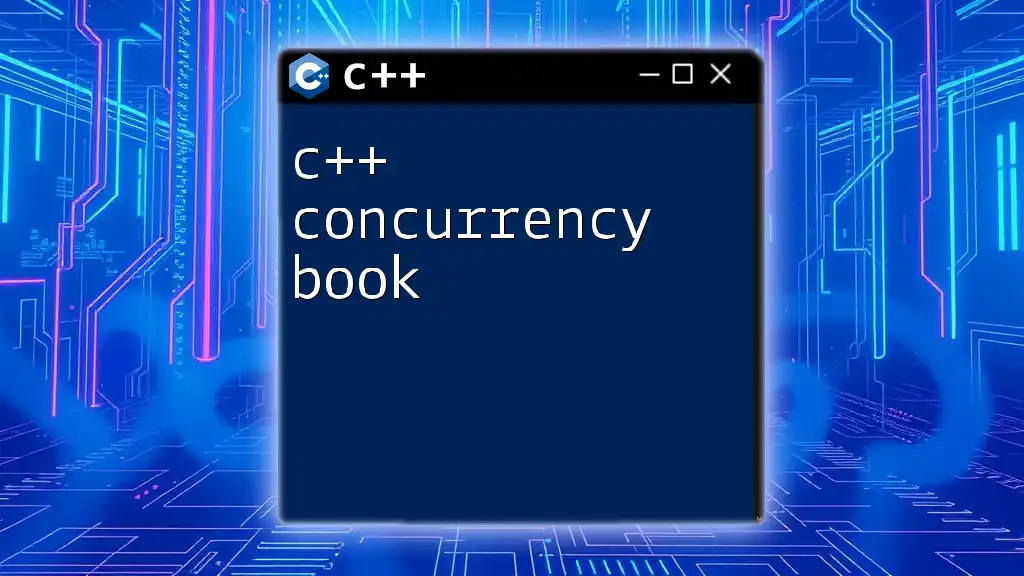
Advanced Concurrency Features
Futures and Promises
C++ provides a powerful mechanism for asynchronous programming through futures and promises. With `std::promise`, a thread can set a value that another thread retrieves via `std::future`. This is particularly useful when you want to perform tasks in the background and fetch their results later.
Here’s an example:
#include <iostream>
#include <thread>
#include <future>
int calculateSquare(int x) {
return x * x;
}
int main() {
std::promise<int> promiseObj;
std::future<int> futureObj = promiseObj.get_future();
std::thread t([&promiseObj] {
promiseObj.set_value(calculateSquare(10)); // Set the future value
});
std::cout << "Square: " << futureObj.get() << std::endl; // Retrieve the value
t.join();
return 0;
}
In this example, `calculateSquare` computes the square of a number, and the `promiseObj` sets the computed value in the future, which can be retrieved later with `futureObj.get()`.
Thread Pools
What is a Thread Pool?
A thread pool is a collection of pre-instantiated threads that can handle multiple tasks. Instead of creating and destroying threads for each task, a thread pool allows for reusing threads, improving performance and resource management.
Implementing a Simple Thread Pool
Here's a fundamental implementation of a thread pool:
#include <iostream>
#include <vector>
#include <thread>
#include <queue>
#include <functional>
#include <condition_variable>
class ThreadPool {
public:
ThreadPool(size_t threads);
~ThreadPool();
void enqueue(std::function<void()> task);
private:
std::vector<std::thread> workers;
std::queue<std::function<void()>> tasks;
std::mutex mtx;
std::condition_variable cv;
bool stop;
};
ThreadPool::ThreadPool(size_t threads) : stop(false) {
for (size_t i = 0; i < threads; ++i) {
workers.emplace_back([this] {
for (;;) {
std::function<void()> task;
{
std::unique_lock<std::mutex> lock(this->mtx);
this->cv.wait(lock, [this] { return this->stop || !this->tasks.empty(); });
if (this->stop && this->tasks.empty())
return;
task = std::move(this->tasks.front());
this->tasks.pop();
}
task();
}
});
}
}
ThreadPool::~ThreadPool() {
{
std::unique_lock<std::mutex> lock(mtx);
stop = true;
}
cv.notify_all();
for (std::thread &worker : workers)
worker.join();
}
void ThreadPool::enqueue(std::function<void()> task) {
{
std::unique_lock<std::mutex> lock(mtx);
tasks.emplace(std::move(task));
}
cv.notify_one();
}
int main() {
ThreadPool pool(4);
for (int i = 0; i < 8; ++i) {
pool.enqueue([i] {
std::cout << "Task " << i << " is being processed." << std::endl;
});
}
return 0;
}
In this example, `ThreadPool` manages a pool of workers that can process tasks. Workers wait for tasks to be enqueued and execute them, managing concurrency efficiently.
Lock-Free Programming
Lock-free programming enables concurrent access to shared data without the necessity of locks. This design helps avoid deadlocks and improves performance in certain scenarios.
Using `std::atomic`, you can perform lock-free operations. Here’s a simple demonstration with atomic integer increments:
#include <iostream>
#include <thread>
#include <atomic>
#include <vector>
std::atomic<int> atomicCount(0);
void increment() {
for (int i = 0; i < 1000; ++i) {
atomicCount++;
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final Count: " << atomicCount.load() << std::endl;
return 0;
}
In this code, multiple threads increment a shared atomic integer. The atomic operations ensure that the counter remains accurate without using locks.
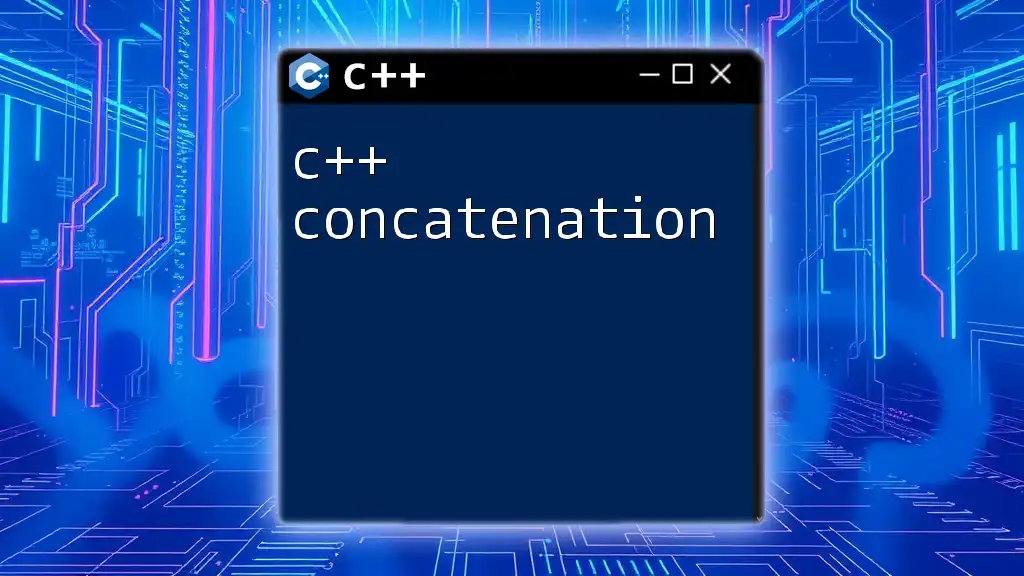
Practical Applications of C++ Concurrency
Concurrent Data Processing
Concurrency is particularly advantageous in applications that need to process large datasets. For instance, when performing data analysis, dividing the workload among multiple threads can speed up the computation significantly.
Consider a simplified scenario where we need to sum elements of a large vector concurrently:
#include <iostream>
#include <vector>
#include <thread>
#include <numeric>
void partialSum(const std::vector<int>& data, int& result, size_t start, size_t end) {
result = std::accumulate(data.begin() + start, data.begin() + end, 0);
}
int main() {
std::vector<int> data(1'000'000, 1); // Initialize a vector with 1 million elements
int result1 = 0, result2 = 0;
std::thread t1(partialSum, std::cref(data), std::ref(result1), 0, data.size() / 2);
std::thread t2(partialSum, std::cref(data), std::ref(result2), data.size() / 2, data.size());
t1.join();
t2.join();
int total = result1 + result2;
std::cout << "Total sum: " << total << std::endl;
return 0;
}
This example showcases how you can split a vector's data and process two halves concurrently, significantly improving performance for computations.
Building Responsive Applications
In interactive applications, responsiveness is paramount. C++ concurrency allows developers to offload heavy computations and maintain a responsive user interface. For instance, in GUI applications, computational tasks should run on separate threads to avoid freezing the interface.
When using libraries like Qt, you can utilize concurrent features seamlessly. For example, invoking a long-running calculation in a separate thread ensures that GUI events can continue to be processed.
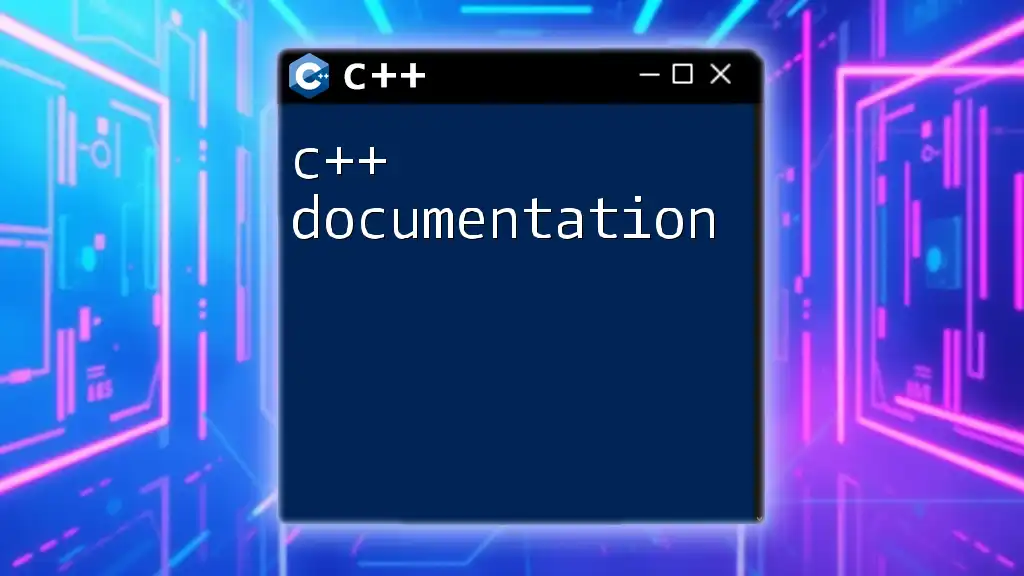
Debugging Concurrent Applications
Common Pitfalls
Concurrency introduces complexities that can lead to several issues, including:
- Deadlocks: When two or more threads wait indefinitely for each other to release locks.
- Race Conditions: Occurrences where the program's outcome depends on the sequence or timing of uncontrollable events.
Recognizing and avoiding these pitfalls through careful design and understanding of synchronization primitives is essential.
Tools for Debugging
Debugging concurrent applications can be challenging. Luckily, several tools can assist in identifying and resolving concurrency-related issues:
- Thread Sanitizer: A powerful tool for detecting data races and deadlocks.
- Valgrind: Useful for detecting memory leaks and threading errors.
Using these tools, you can analyze and troubleshoot concurrency problems effectively, leading to more robust applications.
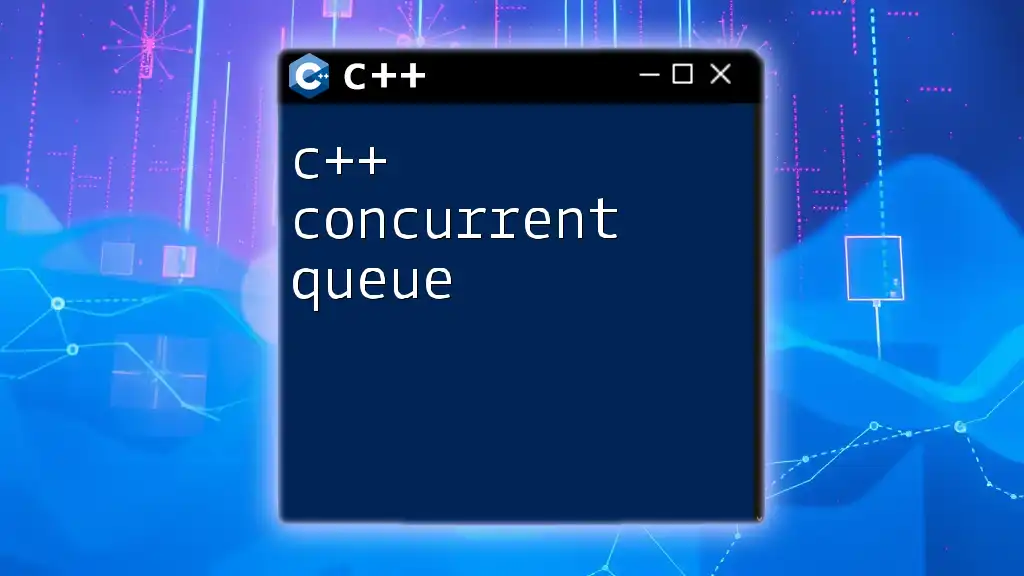
Conclusion
C++ concurrency in action opens up a world of possibilities for enhancing the performance and responsiveness of applications. With proper understanding and utilization of the features provided by the C++ Standard Library, developers can significantly improve their applications' efficiency. As you delve deeper into this topic, consider engaging with different frameworks and libraries to explore more advanced concurrency concepts and best practices. Happy coding!