C++ concurrency allows you to perform multiple tasks simultaneously, enhancing performance and responsiveness, as demonstrated in the following code snippet that showcases basic multithreading using the C++ Standard Library.
#include <iostream>
#include <thread>
void printMessage(int id) {
std::cout << "Thread " << id << " is running.\n";
}
int main() {
std::thread t1(printMessage, 1);
std::thread t2(printMessage, 2);
t1.join(); // Wait for thread t1 to finish
t2.join(); // Wait for thread t2 to finish
return 0;
}
What is Concurrency in C++?
Concurrency is the ability to handle multiple tasks simultaneously within a program, often through multithreading. In C++, concurrency is essential for writing efficient applications that can leverage the full capabilities of modern multi-core processors. By understanding C++ concurrency in action, you can create applications that are responsive and perform better under load.

Understanding Multithreading
Defining Threads
A thread is the smallest unit of processing that can be scheduled by an operating system. Leverage threads to execute tasks concurrently, allowing multiple operations to run in parallel. In contrast, a process is a heavier-weight construct, typically with its own memory space.
Benefits of Multithreading
Utilizing multithreading can lead to:
- Improved Performance: Multithreaded applications perform better by dividing tasks across multiple CPU cores.
- Better Resource Utilization: Threads allow better use of system resources by optimizing wait time and improving throughput.
- Enhanced Responsiveness: In user interfaces, background threads keep the application responsive while performing intensive operations.

Basics of C++ Multithreading
The C++11 Thread Library
Starting with C++11, the C++ Standard Library introduced robust threading support. To utilize multithreading, include the necessary headers, such as `<thread>`, `<mutex>`, and `<condition_variable>`, which provide the tools to create and manage threads, synchronize access to shared resources, and communicate between threads.
Creating Threads in C++
Spawning Threads
Creating threads in C++ is straightforward. You can spawn threads with the `std::thread` class, passing a function (or lambda) that the thread will execute. Here is a simple example:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(threadFunction);
t.join(); // Wait for the thread to finish
return 0;
}
In this example, the `threadFunction` executes on a separate thread. The `join()` method blocks the main thread until the newly created thread has finished executing.
Thread Management
Joining and Detaching Threads
Understanding how to manage threads is crucial for effective multithreading. You can either join or detach a thread:
- Join: Blocks the calling thread until the specified thread completes. It is essential to call join on threads that you want to wait for before proceeding.
- Detach: Allows a thread to execute independently, effectively running in the background. It is important to handle resources carefully when detaching.
Here's a practical illustration:
std::thread t1(threadFunction);
t1.join(); // blocks until t1 finishes
std::thread t2(threadFunction);
t2.detach(); // t2 runs independently
Using `join()` ensures that `t1` completes before the program exits, while `t2` continues to run even if the main thread reaches its end.
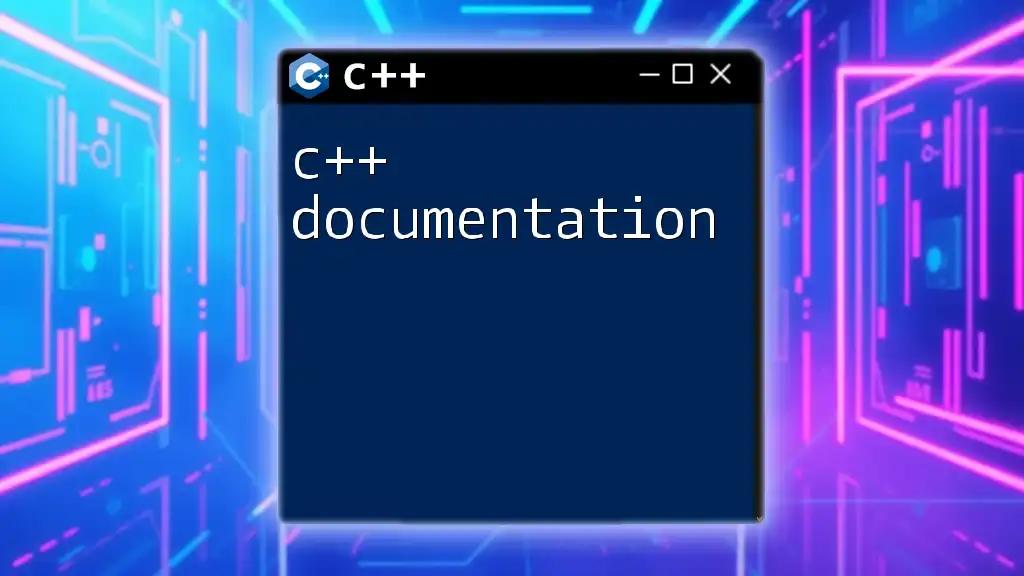
Synchronization Mechanisms
The Need for Synchronization
When multiple threads access shared resources, synchronization is essential to prevent data races and inconsistencies. Race conditions can occur when threads modify shared data at the same time, leading to unpredictable results. To avoid these pitfalls, synchronization mechanisms such as mutexes and condition variables are employed.
Mutex (Mutual Exclusion)
Mutexes are used to ensure mutual exclusion, allowing only one thread to access a resource at a time. By wrapping critical sections of code within mutex locks, you can safely manage shared data. Here’s an example:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void threadFunction(int id) {
mtx.lock();
std::cout << "Thread " << id << " is working." << std::endl;
mtx.unlock();
}
int main() {
std::thread t1(threadFunction, 1);
std::thread t2(threadFunction, 2);
t1.join();
t2.join();
return 0;
}
In this example, the mutex `mtx` restricts access during the critical section, ensuring that only one thread can print its message at a time.
Condition Variables
Condition variables allow threads to signal each other about changes in state and are often used in conjunction with mutexes. They enable a thread to wait for a particular condition to be true before proceeding. Here’s how to implement condition variables:
#include <iostream>
#include <thread>
#include <condition_variable>
#include <mutex>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void waitForSignal() {
std::unique_lock<std::mutex> lck(mtx);
cv.wait(lck, [] { return ready; });
std::cout << "Thread is running after signal." << std::endl;
}
void sendSignal() {
std::this_thread::sleep_for(std::chrono::seconds(1));
std::lock_guard<std::mutex> lck(mtx);
ready = true;
cv.notify_one();
}
int main() {
std::thread waiter(waitForSignal);
std::thread notifier(sendSignal);
waiter.join();
notifier.join();
return 0;
}
In the above code, the `waitForSignal` thread waits for `ready` to become `true` before proceeding. The `sendSignal` thread updates this variable, signaling the waiting thread to continue.

Advanced Multithreading Concepts
Thread Pools
Thread pools manage a pool of threads to execute tasks concurrently. This is an efficient approach as it avoids the overhead of thread creation and destruction for every task. Thread pools reuse idle threads to perform new tasks, optimizing resource usage.
#include <iostream>
#include <vector>
#include <thread>
#include <future>
#include <functional>
class ThreadPool {
// Implementation details are omitted for brevity
};
int main() {
ThreadPool pool(4); // Initialize a pool with 4 threads
auto result = pool.enqueue([]{ return 7; });
std::cout << "Result: " << result.get() << std::endl;
return 0;
}
By using thread pools, you can efficiently manage execution without the overhead of constantly starting and stopping threads.
Future and Promises
Futures and promises provide a powerful mechanism for managing asynchronous computations. A promise is used to set a value or an exception that can be later retrieved by a future.
#include <iostream>
#include <thread>
#include <future>
std::promise<int> p;
std::future<int> f = p.get_future();
std::thread t([&p](){
std::this_thread::sleep_for(std::chrono::seconds(1));
p.set_value(10); // Set the value of the promise
});
std::cout << "Value from future: " << f.get() << std::endl; // Retrieves the value
t.join();
Here, the promise is fulfilled, allowing the main thread to retrieve the value after a brief delay. This mechanism is useful in coordinating tasks that depend on asynchronous results.

Common Pitfalls and Best Practices
Avoiding Deadlocks
Deadlocks occur when two or more threads are waiting on each other to release resources, causing the program to hang. Strategies to avoid deadlocks include:
- Lock ordering: Always acquire locks in a predetermined order.
- Timeouts: Implement timeouts when acquiring locks to allow threads to back off.
Profiling and Debugging Multithreaded Applications
Effective profiling and debugging can be challenging in multithreaded applications. Use tools like Valgrind, gdb, or thread sanitizers to manage and debug threads. Logging, too, plays a crucial role in understanding thread behavior and identifying bottlenecks.

Conclusion
C++ concurrency in action, particularly in practical multithreading scenarios, enables developers to build robust and efficient applications. By mastering key concepts such as threads, mutexes, condition variables, thread pools, and futures, you position yourself to leverage the full power of modern multi-core processors. With careful implementation, thorough testing, and attention to best practices, you can develop applications that not only perform well but are also resilient in the face of complex multithreading challenges. Continue exploring and practicing these concepts to enhance your skill set as a C++ developer.