"Hands-on C++ game animation programming involves practical exercises and examples to quickly master the fundamentals of creating animated game elements using C++."
Here’s a simple code snippet demonstrating a basic frame update for animation in C++:
#include <iostream>
#include <chrono>
#include <thread>
void animate() {
for (int frame = 0; frame < 10; ++frame) {
std::cout << "Animating frame: " << frame << std::endl;
std::this_thread::sleep_for(std::chrono::milliseconds(200)); // Simulate frame delay
}
}
int main() {
animate();
return 0;
}
Introduction to C++ Game Animation Programming
What is Game Animation?
Game animation refers to the process of creating movement and transitions for characters, objects, and scenes in video games. It is a crucial aspect of game design that brings a game to life by providing visual feedback and enhancing the player’s experience. Effective animation can communicate emotions, reactions, and the dynamic nature of game environments. In C++, understanding animation concepts allows developers to harness the power of the language for high-performance graphics programming.
Why Use C++ for Game Animation?
C++ stands out in the game development community primarily due to its performance capabilities. Its close relationship with system hardware offers optimizations that higher-level languages may not. Additionally, C++ provides extensive control over memory management, allowing developers to maximize rendering efficiency—crucial for achieving smooth animations in real-time environments. C++ is also an industry-standard programming language, widely used in major game engines like Unreal Engine and many AAA titles.
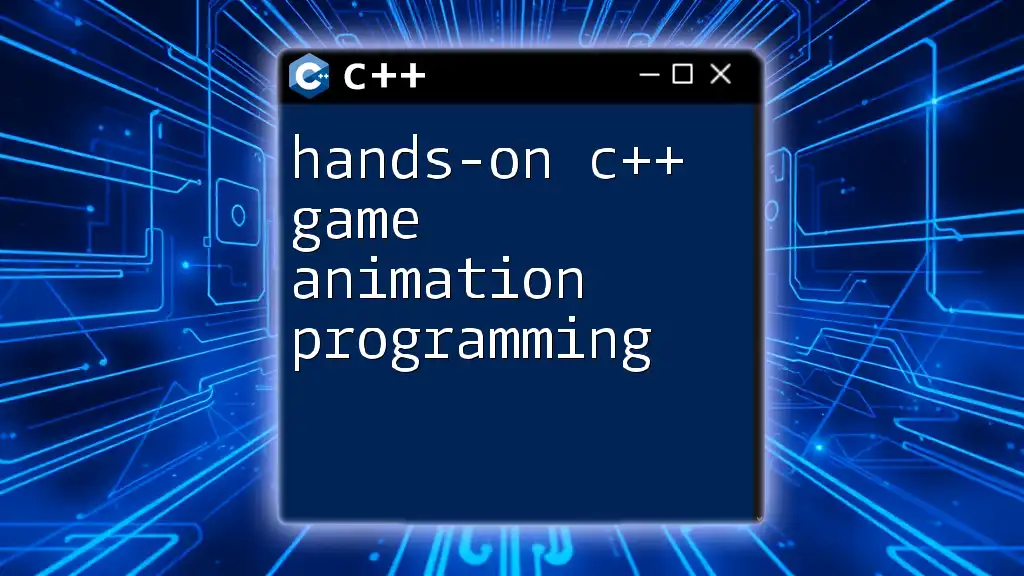
Getting Started with C++ Animation Basics
Setting Up Your Development Environment
Before diving into animation programming, it is essential to set up a proper development environment. Popular IDEs such as Visual Studio and Code::Blocks are highly recommended because they come equipped with tools that simplify debugging and project management. Moreover, incorporating graphics libraries like SFML or SDL can facilitate powerful 2D and 3D rendering capabilities needed for game animations.
Understanding Basic Animation Concepts
Frames and Frame Rates
Animation relies heavily on the concept of frames. Each frame represents a still image that, when sequenced together, creates the illusion of movement. The number of frames displayed within one second is referred to as frame rate (FPS). For instance, a common target for smooth animations is 60 FPS, where 60 frames are drawn every second.
When developing animations in C++, understanding how to manage frame rates efficiently is vital. Utilizing a game loop to manage the updates and render cycles will ultimately influence the fluidity of your animations.
Interpolation
Interpolation is a technique used to determine intermediate values between two keyframes. It helps create smoother transitions from one state to another rather than abrupt changes.
Linear interpolation is a straightforward method where the value between two points is calculated proportionally over time. In contrast, spline interpolation provides a more naturally curved movement often used in more complex animations.
Here’s a code snippet demonstrating linear interpolation in C++:
float lerp(float a, float b, float t) {
return a + (b - a) * t; // t varies from 0 to 1
}
Using the `lerp` function, you can create smooth transitions by updating the position of an object between two points based on the time elapsed.
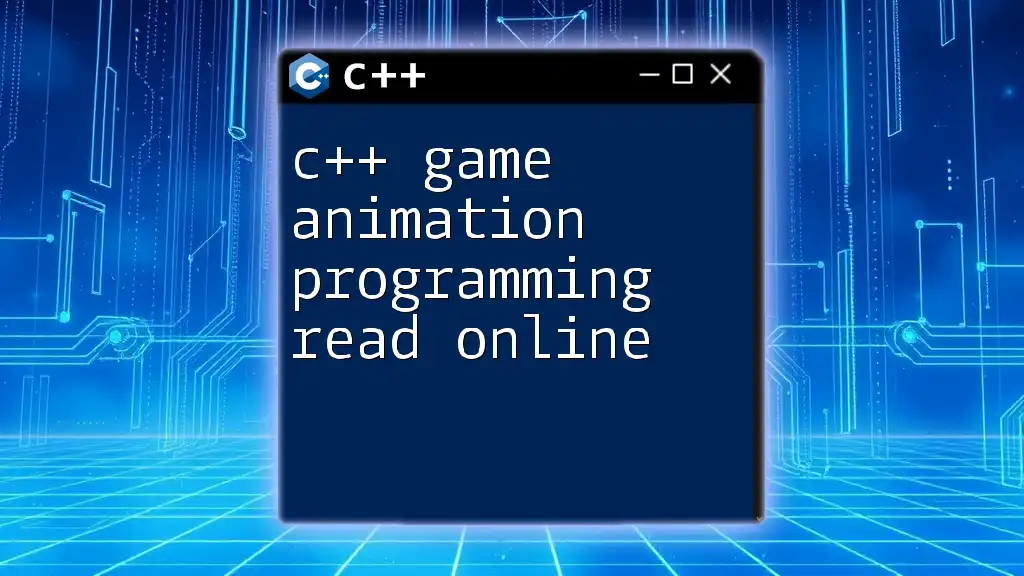
Creating Your First Animation in C++
Building a Simple Animation Framework
To begin animating with C++, you first need to establish a project framework. A basic setup might involve creating a main source file where your game loop resides.
Here’s how to initialize a simple animation framework:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "Simple Animation");
// Additional code to define textures and objects will be added here...
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed) {
window.close();
}
}
// Animation updates will be performed here...
window.clear();
// Drawing code will go here...
window.display();
}
return 0;
}
Animating a Sprite
To animate a sprite, you need to load an image into a texture and create a sprite object from it. Once you set up your sprite, you can utilize the game loop to create movement or animation effects.
Here’s an example of how to animate a sprite in C++:
sf::Texture texture;
if (!texture.loadFromFile("sprite.png")) {
// Handle loading error
}
sf::Sprite sprite(texture);
// Game loop
while (window.isOpen()) {
// Update sprite position
sprite.move(0.1f, 0); // Move right
window.clear();
window.draw(sprite);
window.display();
}
In this example, your sprite continuously moves to the right, showcasing basic animation.
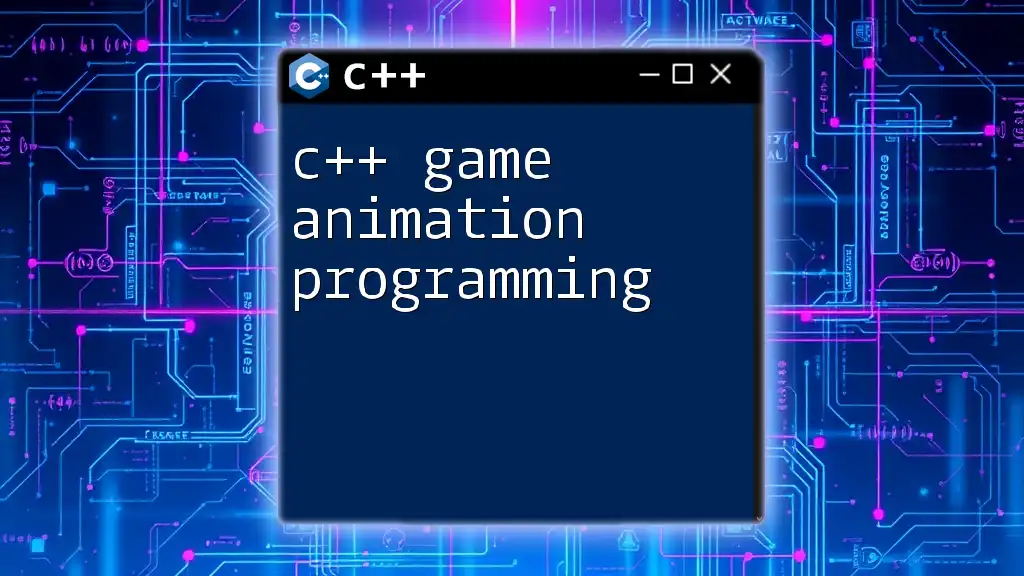
Advanced Animation Techniques
Character Animation
When creating character animations, understanding the differences between skeletal and frame-based animation is essential.
Skeletal Animation involves defining a rig with bones and joints, making it possible to animate complex movements easily. This method is efficient in terms of resource usage since it allows for the reuse of animations with different character models.
Frame-based Animation, on the other hand, involves playing sequences of pre-rendered frames. Each frame serves as a distinct pose, and transitions are explicit in the graphics.
Using Animation Libraries and Tools
Many libraries like SFML offer built-in support for various animations. Additionally, external tools like Spine and DragonBones allow developers to design animations and export them directly to their C++ projects.
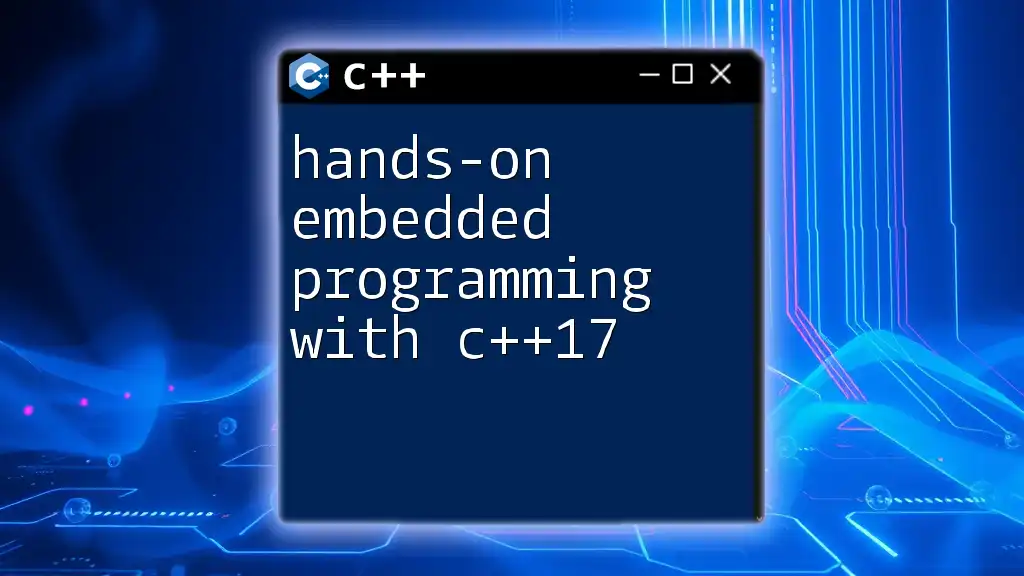
Managing Animation States
Creating Animation States
Effective animation management often includes the development of states for your characters or objects, such as idle, running, or jumping. Defining these states allows you to control when specific animations should trigger based on interactions or environmental conditions.
Switching Between Animations
To enhance gameplay experience, you’ll frequently switch between different animations based on player input or game events. Here's an example of how to manage different animation states in C++:
if (isJumping) {
playJumpAnimation();
} else if (isRunning) {
playRunAnimation();
} else {
playIdleAnimation();
}
This approach provides a clear and dynamic way to respond to player actions and create a more immersive experience.
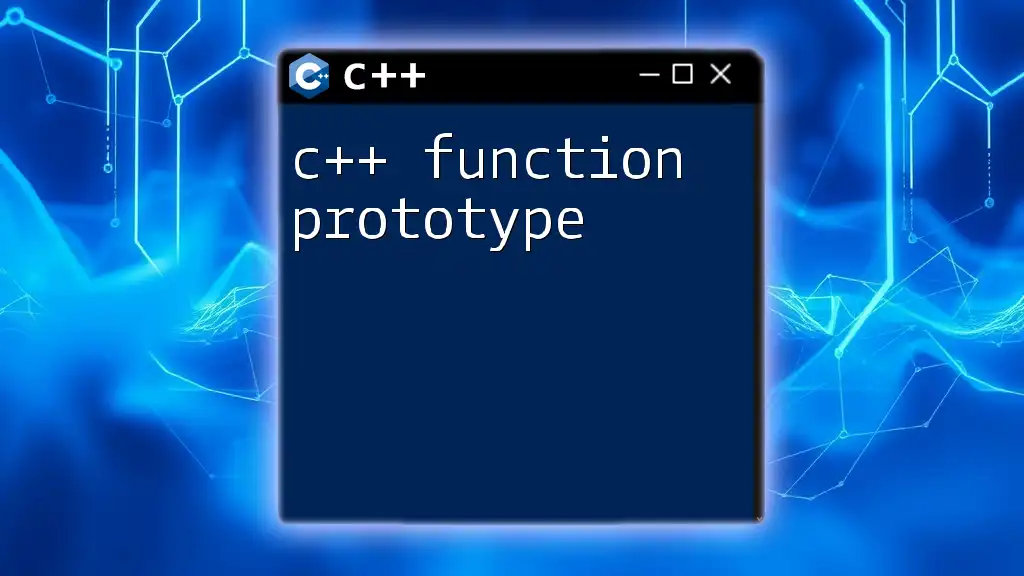
Optimizing Your C++ Animations
Improving Performance
Animation can be resource-intensive, so optimizing performance becomes crucial. One effective method is to reduce the computational load by limiting frame updates—only updating animations when necessary, such as when the player is active.
Efficient memory management is another critical factor. Monitor the memory used by textures and other visual resources to prevent leaks. Implement object pooling techniques to reuse objects instead of constantly creating and destroying them.
Profiling Your Animation
Employ profiling tools to analyze the performance of your animations. Tools like Valgrind or built-in profilers in IDEs can help identify bottlenecks in rendering and logic that may slow down performance.
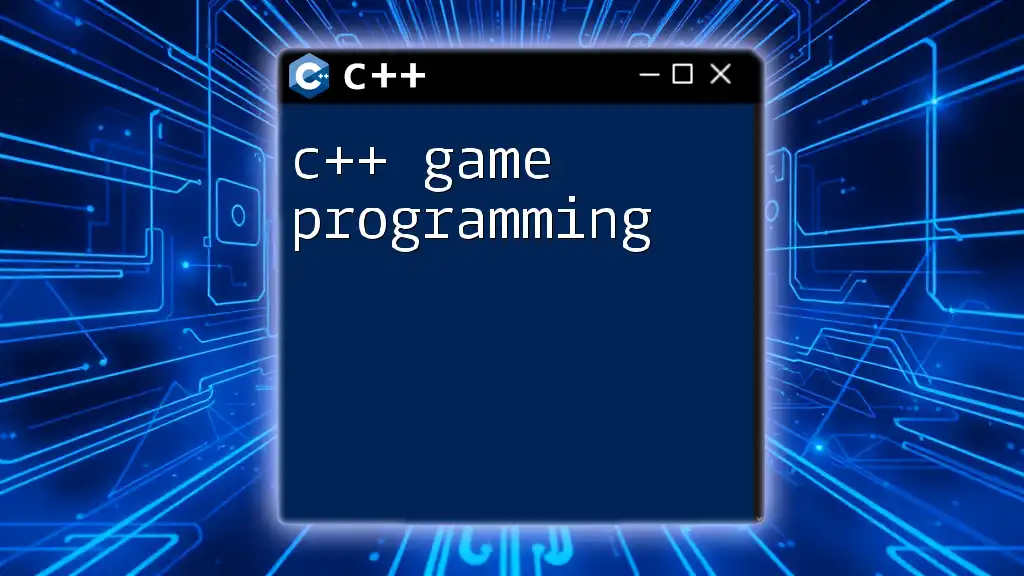
Real-World Applications and Projects
Case Studies of Successful C++ Game Projects
There are numerous successful games that exemplify the power of C++ in animation. Titles like Call of Duty and Assassin’s Creed utilize complex animations that showcase real-time interactions, leveraging C++’s performance advantages.
Project Ideas for Practice
Aspiring developers can enhance their skills by practicing with projects such as:
- A 2D platformer featuring character jumps and enemy movements.
- An interactive art gallery where objects animate based on user proximity.
- A simple physics demo where animated objects react to forces and collisions.
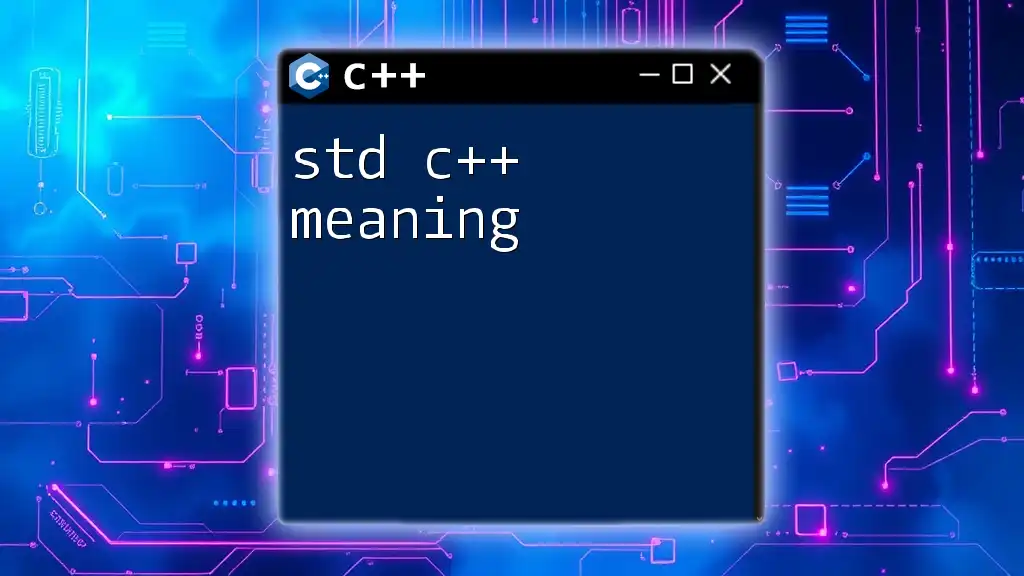
Conclusion
In conclusion, the journey into hands-on C++ game animation programming begins with understanding fundamental concepts and developing a solid foundation. With practice, optimization, and the effective use of tools, you can create immersive experiences that captivate players. Continue to explore the many resources available and challenge yourself to build and innovate. Your animation programming skills in C++ can open up exciting opportunities in the game development industry, making your creative visions a reality.
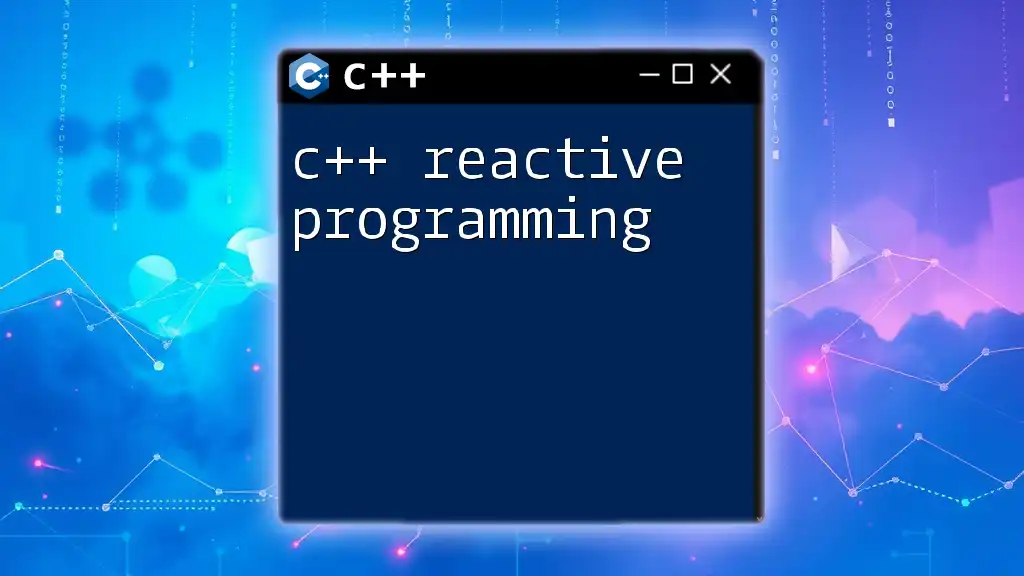
Additional Resources
Books and Online Courses
Some excellent resources to consider for further deepening your knowledge include:
- "C++ Primer" for foundational programming concepts.
- Online courses focused on game development and animation techniques.
Online Communities and Forums
Joining communities such as GameDev.net or participating in forums like Stack Overflow can provide invaluable support and networking opportunities with fellow developers.