C++ game animation programming involves creating smooth and efficient movements of game characters or objects through the use of interpolation techniques and frame updates.
Here's a simple example of a C++ code snippet that demonstrates basic animation using a game character position update:
#include <iostream>
#include <chrono>
#include <thread>
struct Character {
float x, y;
void updatePosition(float deltaX, float deltaY) {
x += deltaX;
y += deltaY;
}
void displayPosition() {
std::cout << "Character Position: (" << x << ", " << y << ")\n";
}
};
int main() {
Character character = {0.0f, 0.0f};
while (true) {
character.updatePosition(1.0f, 0.0f); // Move right
character.displayPosition();
std::this_thread::sleep_for(std::chrono::milliseconds(100)); // Pause for 100ms
}
return 0;
}
Understanding the Basics of Game Animation
Animation Principles
Key Frames
In the realm of animation, keyframes serve as the foundational points that define specific positions or states of an object at certain times. For example, in C++ game animation programming, if you're animating a character waving, the keyframes would represent the critical points of the arm's movement—like the lowest and highest points of the wave. By defining these keyframes, you can interpolate the frames in between, resulting in smooth motion.
Tweening
Tweening, short for in-betweening, involves creating intermediate frames between keyframes to achieve smooth transitions. In C++, libraries like SFML or SDL can aid in implementing tweening effectively. This technique reduces the workload of hand-drawing every frame, allowing games to appear more fluid and dynamic.
Common Animation Techniques
Sprite Animation
2D sprite animation is the process of displaying a sequence of images to create the illusion of movement. Each image represents a single frame of the character's motion. For instance, a running character sprite might have multiple frames for each leg movement.
To manage sprite animation in C++, you can define a `Sprite` class that handles the loading of sprite sheets and updates the displayed frame based on the game's timing logic.
Skeletal Animation
Skeletal animation is a method used in 3D models, where a 'skeleton' made of bones controls the movement of a mesh. Instead of animating the object as a whole, skeletal animation allows for more natural and complex movements, making it ideal for character animation in modern games. This technique is highly efficient as it only requires a few bones to manipulate complex character models.
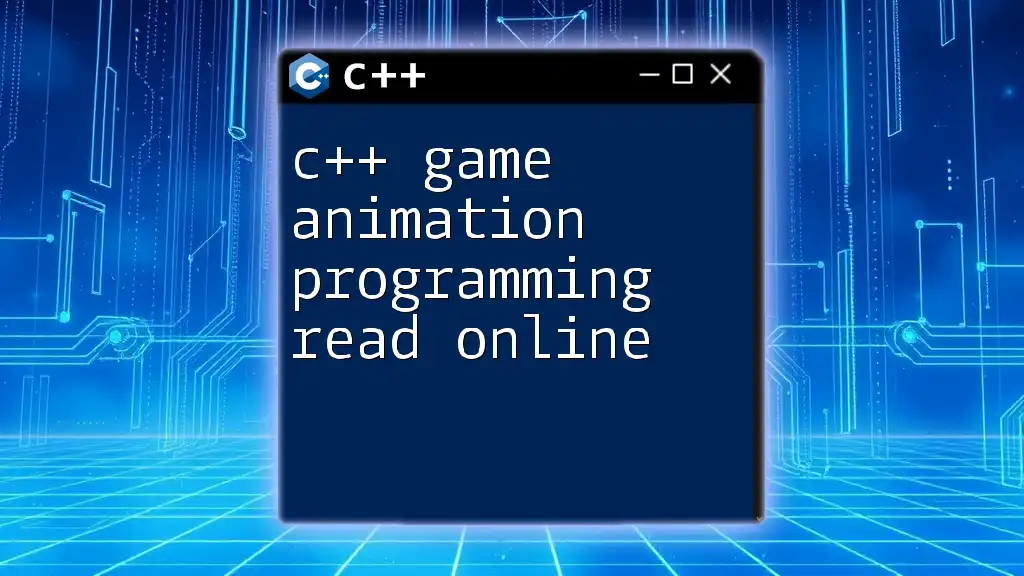
Setting Up Your C++ Game Development Environment
Required Tools and Libraries
Game Engine Overview
In C++ game animation programming, choosing a game engine with robust animation support is crucial. Unreal Engine offers a powerful built-in animation system, while Cocos2d is another popular choice for 2D games. Both engines provide libraries and tools specifically aimed at simplifying the animation process.
Development Tools
Setting up an Integrated Development Environment (IDE) like Visual Studio can streamline your coding experience. Additionally, using libraries such as SFML or SDL can greatly assist in the management of animations, providing functions for sprite handling and frame updates.
Project Structure
Organizing Your Files
Maintaining a well-organized project structure is essential for effective C++ game animation programming. Ideally, you should structure your files into folders for assets (like images and models), scripts for animation logic, and a separate folder for shaders if you are using custom graphics. This organization not only keeps things tidy but also facilitates easier debugging and collaboration.
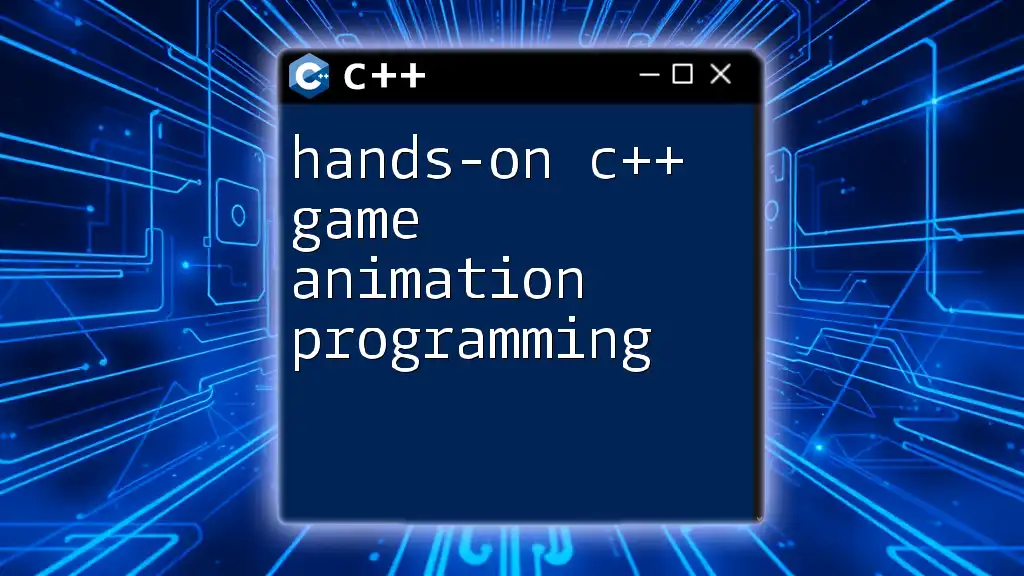
C++ Basics for Game Animation
Essential C++ Concepts
Classes and Objects
Understanding object-oriented programming (OOP) principles is vital for effective animation in C++. Classes can represent animated entities, allowing properties and methods to be encapsulated. For instance, you might create a `Character` class that contains all properties related to movement and animation.
Inheritance and Polymorphism
Using inheritance and polymorphism allows for creating a hierarchy of animated objects. For example, if you have a base `Character` class, you could derive a `Hero` and an `Enemy` class, each with its specialized animation methods, while still adhering to shared properties and behaviors defined in the parent class.
Setting Up a Basic Game Loop
Introduction to the Game Loop
The game loop is the heart of any game. It continuously processes player input, updates the game state, and renders the output to the screen. In C++ game animation programming, a typical game loop might look like this:
while (running) {
processInput();
update();
render();
}
This structure allows for consistent and real-time updates to animations based on game events and player actions.
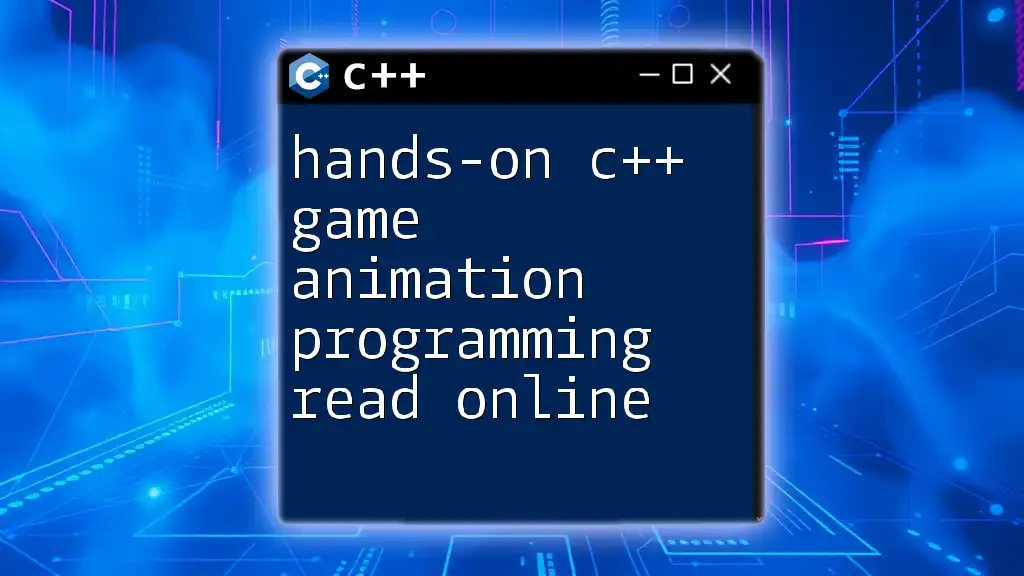
Implementing Animation
Creating Animated Sprites
Loading Sprite Sheets
Loading sprite sheets efficiently is key to managing animated sprites. Use `SFML` or another library to help load and manage the images. A simple loading function can look like this:
Texture texture;
if (!texture.loadFromFile("spritesheet.png")) {
// Handle error
}
Animating Sprites
To animate sprites, you will typically update the displayed texture based on the current animation frame. A function to handle this could look like:
void animateSprite(Sprite& sprite, int frameCount, float deltaTime) {
sprite.setTextureRect(IntRect(currentFrame * frameWidth, 0, frameWidth, frameHeight));
currentFrame = (currentFrame + 1) % frameCount; // Loop through frames
}
Working with 3D Models
Importing 3D Models
If working with 3D models, you'll require libraries like Assimp to load model files. Once imported, properly structuring your classes to handle vertex data and animation skeletons is imperative.
Animating Using Bone Systems
Skeletal animation requires you to bind a mesh to a skeleton. Each bone moves the vertices associated with it during animation. Quality libraries like Assimp can help import bone animations directly.
Implementing Transition Effects
Fading and Tweening
Transition effects like fading or tweening animations can significantly enhance the user experience in games. You might implement a simple fade function as follows:
void fadeOut(Sprite& sprite, float duration) {
for (float alpha = 255; alpha > 0; alpha -= 255 * (deltaTime / duration)) {
sprite.setColor(Color(255, 255, 255, (int)alpha));
}
}
Sample Code Snippet
Using tweening can create smooth transitions. A typical implementation might look like this:
void tweenPosition(Object& obj, Vector3 targetPosition, float duration) {
Vector3 start = obj.getPosition();
for (float t = 0; t < duration; t += deltaTime) {
float alpha = t / duration;
obj.setPosition(lerp(start, targetPosition, alpha));
}
}
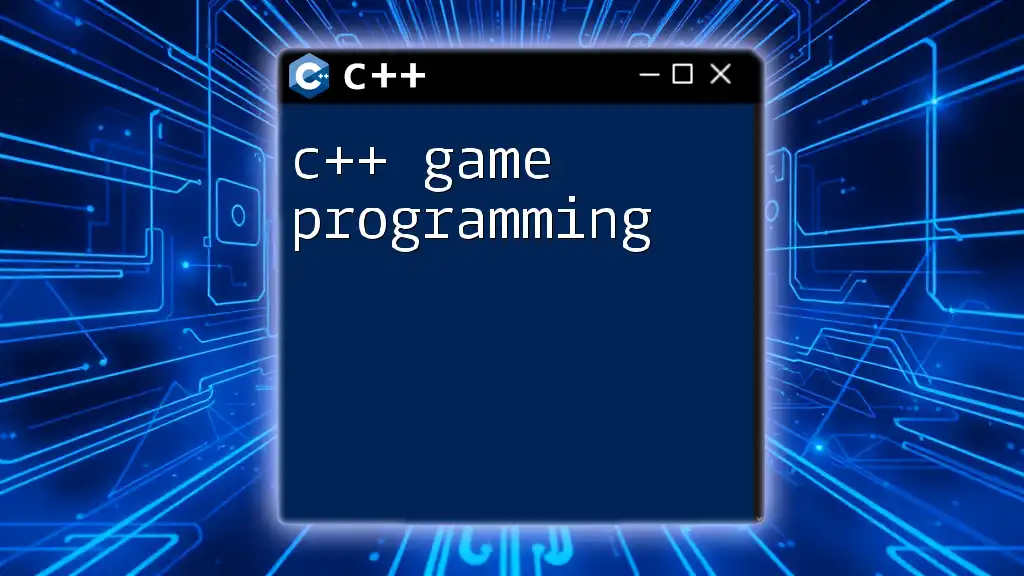
Advanced Animation Techniques
Blend Trees
What Are Blend Trees?
Blend trees are advanced structures that allow for the blending of multiple animations based on character states or user inputs. For instance, blending between running and jumping animations creates a more natural movement flow.
Implementation Example
To implement a blend tree in C++, you need to manage multiple animation states and their transitions, updating the current animation based on the character's state.
Physics-Based Animation
Integrating Physics with Animation
Incorporating physics into animations can yield incredibly realistic results. By tying a character's movement to physics simulations, such as gravity, you can achieve organic motion. For example, applying forces to a character model during a jump makes it appear more lifelike.
Sample Code
An example showing how physics affect animation can be implemented using a physics engine:
void updateCharacterPhysics(Character& character) {
if (character.isInAir()) {
applyGravity(character);
// Update character's position based on physics
}
}
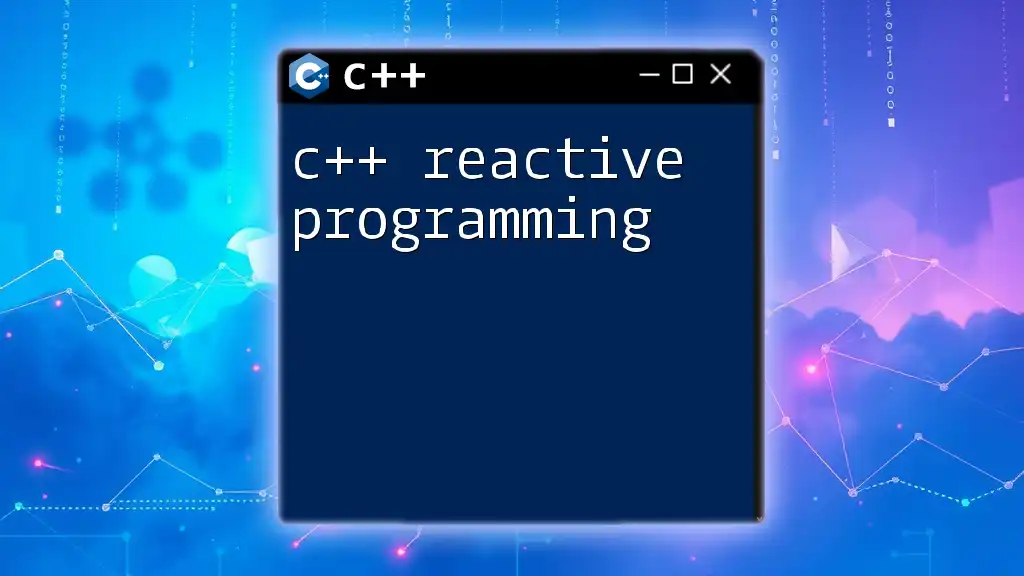
Performance Optimization for Animation
Managing Resources Efficiently
Reducing Memory Usage
To ensure efficient performance in C++, focusing on memory management is crucial. Consider loading assets as needed and unloading them when no longer required. Using techniques such as texture atlases can also minimize memory overhead by combining multiple textures into a single image.
Efficient Rendering Techniques
Batch rendering, where multiple sprites are drawn in a single call, can drastically improve performance. This technique reduces the number of draw calls the GPU must handle.
Profiling and Debugging Animations
Using Profiling Tools
Tools like Visual Studio Profiler can help identify performance bottlenecks in animation logic. Regular profiling will allow you to optimize frame rates and ensure smooth animations.
Common Animation Issues
It’s common to face animation glitches, such as jittering or desynchronization when dealing with complex animations. Ensure that your animation updates are consistent and maintain a stable frame rate by synchronizing animation timing with the game loop.
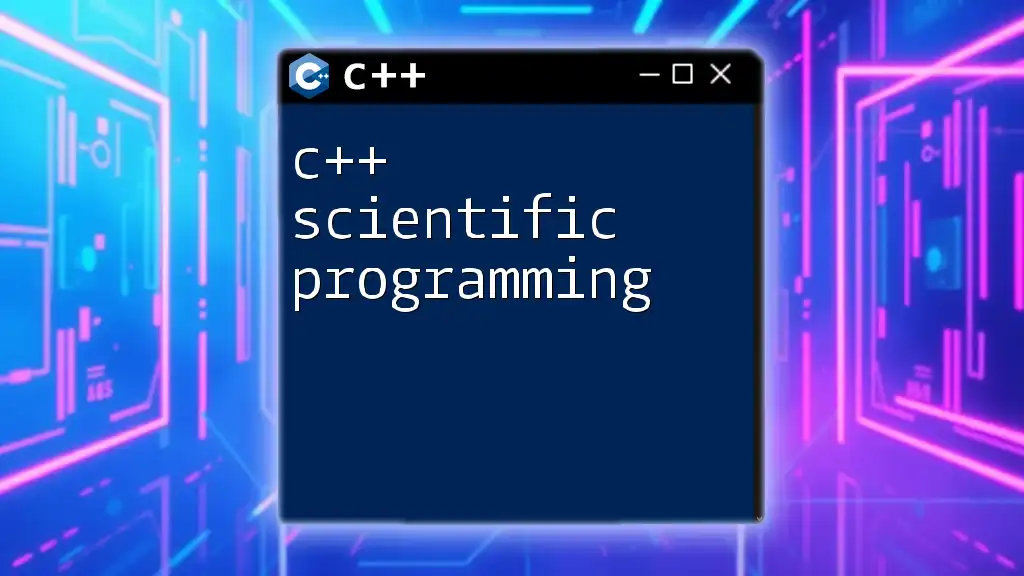
Conclusion
In C++ game animation programming, mastering the concept of animation is crucial for enhancing the overall gaming experience. From understanding keyframes and tweening to implementing advanced techniques like blend trees and physics-based animation, the journey through game animation is rich with possibilities. As you delve deeper, remember that experimentation and practice will lead to mastery.
Take advantage of the myriad of resources available, and don't hesitate to delve into community forums for support and inspiration as you bring your animated characters to life!
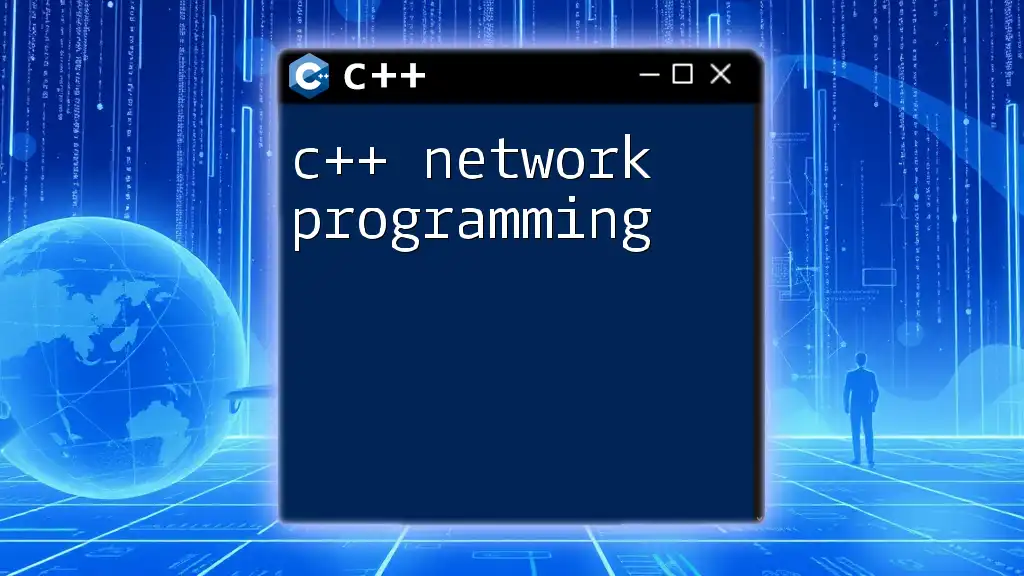
Additional Resources
Recommended Reading and Tools
Explore books focused on C++ game development and animation techniques. Online communities and forums like Reddit's r/gamedev and Stack Overflow are excellent for connecting with fellow developers, sharing your challenges, and getting feedback on your projects.