C++ through game programming offers a dynamic way to learn the language by creating interactive experiences, enhancing both coding skills and understanding of programming concepts.
#include <iostream>
int main() {
std::cout << "Welcome to the C++ Game!" << std::endl;
int playerScore = 0;
for (int level = 1; level <= 5; ++level) {
std::cout << "Level " << level << ": Score +10" << std::endl;
playerScore += 10;
}
std::cout << "Your final score is: " << playerScore << std::endl;
return 0;
}
What is C++?
C++ is a powerful, versatile programming language that has stood the test of time since its creation in the early 1980s by Bjarne Stroustrup. Known for its efficiency and performance, C++ has become a staple in various domains, particularly in systems and application software, as well as in game development.
C++ allows for low-level memory manipulation and has facilities for both procedural and object-oriented programming, making it an ideal choice for creating resource-intensive applications like video games.
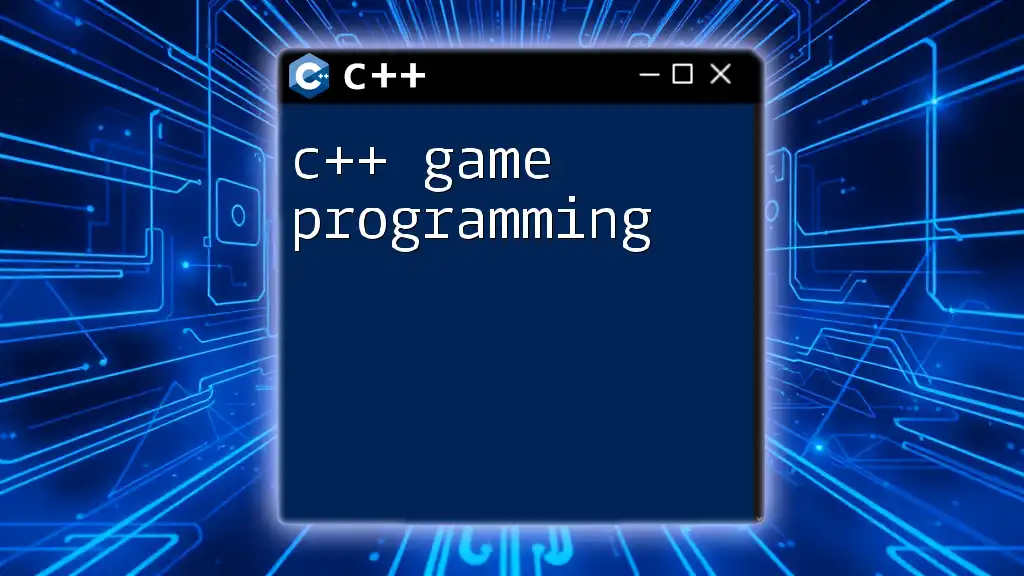
Why Use Game Programming to Learn C++?
Using game programming as a vehicle to learn C++ offers a host of benefits. Firstly, it transforms what can often be a dry subject into an engaging and hands-on experience. Diligently working through the steps of game development fosters a meaningful understanding of fundamental programming concepts. Not only will you acquire technical skills, but you will also learn problem-solving, creativity, and the ability to debug effectively—skills that are invaluable in any programmer's toolkit.
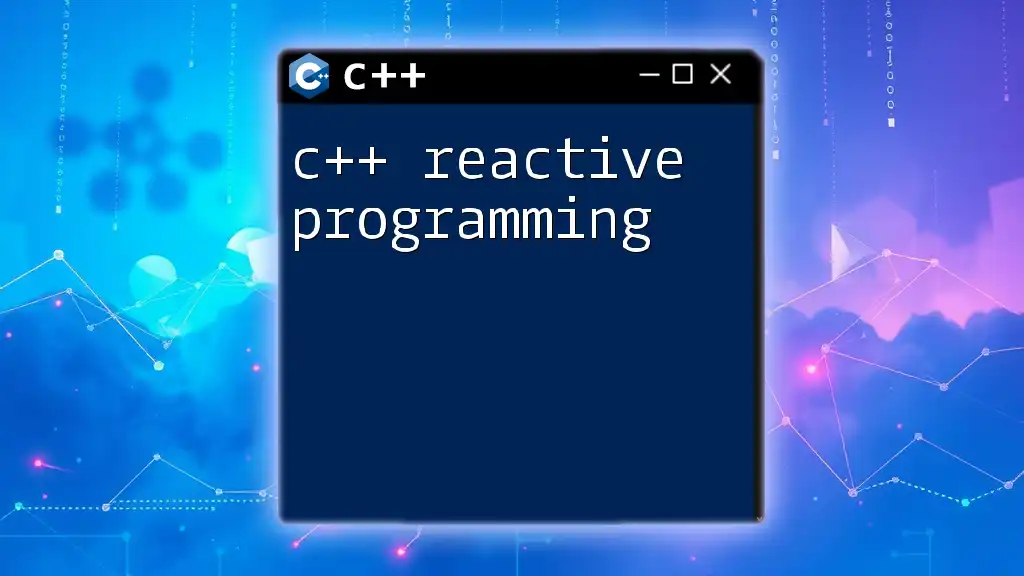
Setting Up Your Development Environment
Choosing the Right IDE
To get started, it's crucial to have the right Integrated Development Environment (IDE). Popular choices include:
- Visual Studio: Known for its comprehensive toolset tailored for Windows.
- Code::Blocks: A lightweight, cross-platform alternative that is easy to use.
- CLion: A commercial product from JetBrains, ideal for programmers who appreciate robust features.
Once you have chosen your IDE, follow the installation instructions available on their official sites.
Setting Up a C++ Compiler
A C++ compiler is essential for turning your high-level code into executable programs. Commonly used compilers include:
- GCC (GNU Compiler Collection): Available on multiple platforms.
- MSVC (Microsoft Visual C++): Tightly integrated with Visual Studio.
To install GCC, you can use a package manager like `apt` for Ubuntu or download the installer from the official website for Windows systems.
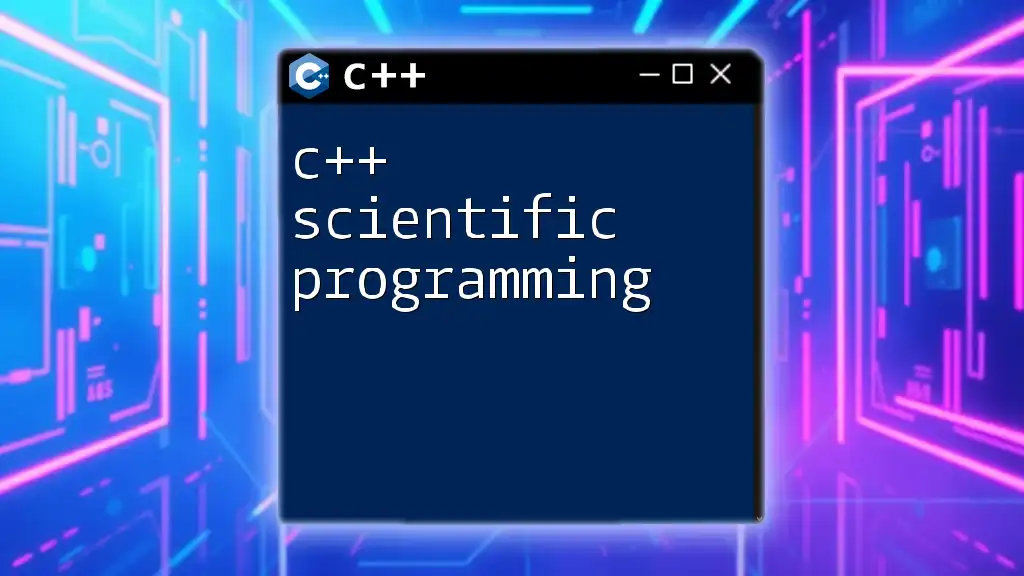
Understanding Game Development Basics
Key Concepts in Game Development
To navigate the world of game programming, you'll need to understand some key concepts:
Game Loop: The core of every game, the game loop continuously updates the game state and renders the graphics. Without a game loop, your game would not be able to respond to user input or update visuals in real time.
Game States: These represent different phases of your game (e.g., main menu, gameplay, pause). Managing these states effectively is vital for a smooth gaming experience.
Game Programming Paradigms
Object-Oriented Programming (OOP) in Games: C++ heavily leverages OOP principles, allowing you to create complex game structures. This includes:
- Classes: Define object types with attributes and methods.
- Inheritance: Create new classes that derive from existing ones.
- Polymorphism: Allow methods to do different things based on the object calling them.
Component-Based Architecture: This design pattern is gaining popularity in modern game engines, where gameplay elements are managed as independent components. This allows for greater flexibility, as different entities can share the same components, promoting code reuse.
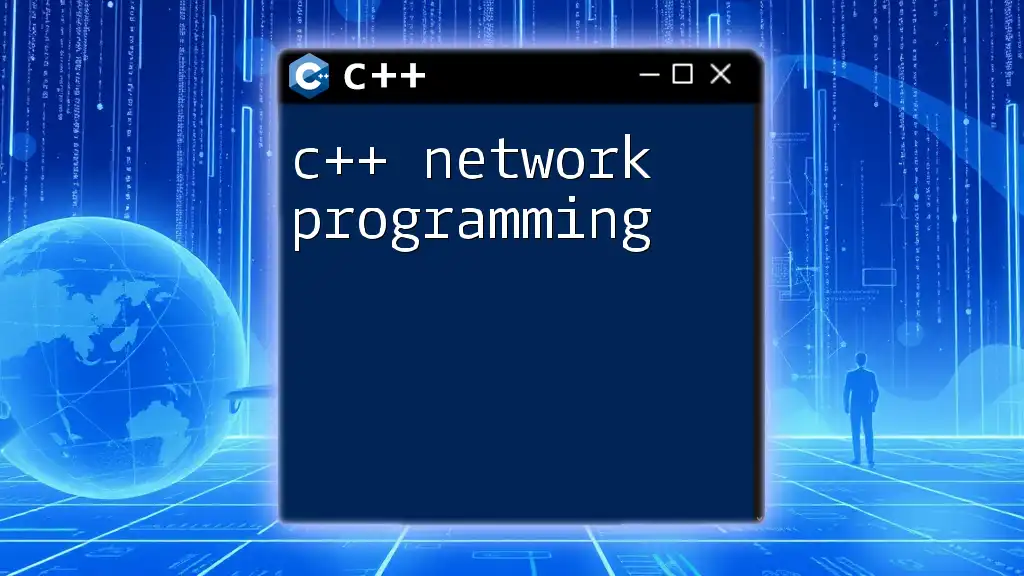
Your First Game: Building a Simple Console Game
Project Overview: A Text-Based Adventure Game
To begin putting your skills to the test, let’s outline a simple text-based adventure game. This game will require players to navigate through various scenarios and make choices that affect the story's outcome.
Setting Up the Project
Create a new directory for your project and structure it effectively. Consider having folders such as `/src` for source code and `/assets` for any resources like images or sounds you may use in future projects.
Code Snippet: Basic Game Loop
A fundamental aspect of your game will be the game loop:
while (gameRunning) {
// Process Input
// Update Game Logic
// Render Graphics
}
Example: Player Interaction
To handle user interactions, you’ll want to capture input effectively. Here’s a simple way to do this:
std::string playerInput;
std::getline(std::cin, playerInput);
This allows the player to enter their decisions seamlessly.
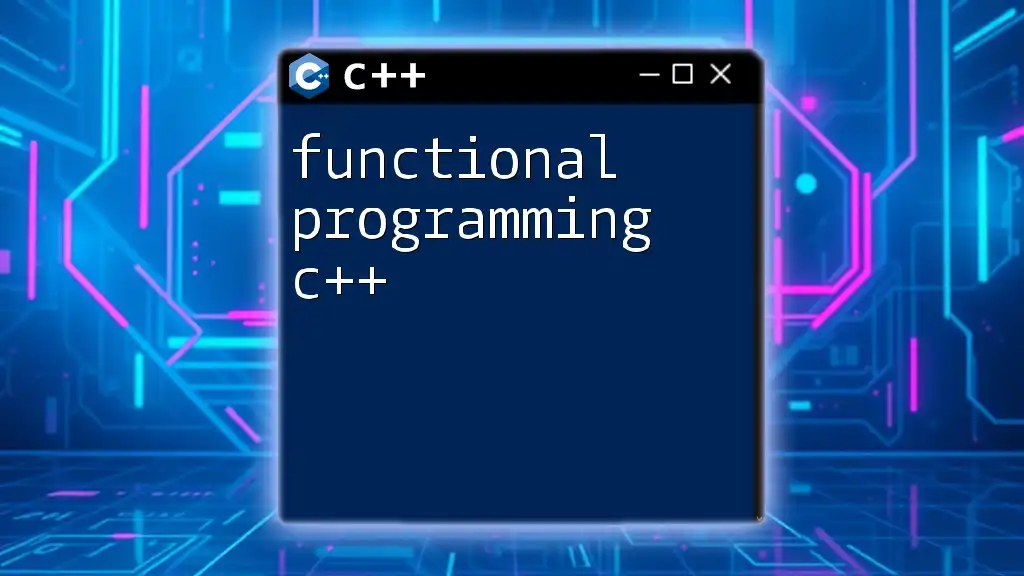
Moving to 2D Game Development
Choosing a 2D Game Library
To take the next step, you’ll want to work with a library that facilitates 2D game development. Popular choices include SDL (Simple DirectMedia Layer), SFML (Simple and Fast Multimedia Library), and Allegro.
Installing and Setting Up SDL
Installing SDL can often be done through a package manager or by downloading the binaries from the SDL website. Once you have it set up, link it to your project in your IDE.
Example Project: Create a Simple 2D Game
For this project, let’s create a basic platformer. You’ll need to handle graphics, sounds, and player input.
Code Example: Rendering a Sprite
Rendering graphics is a pivotal part of any game. Here’s a basic snippet to render a sprite:
SDL_RenderCopy(renderer, spriteTexture, NULL, &destRect);
This command tells the renderer to display the texture at the specified destination rectangle.
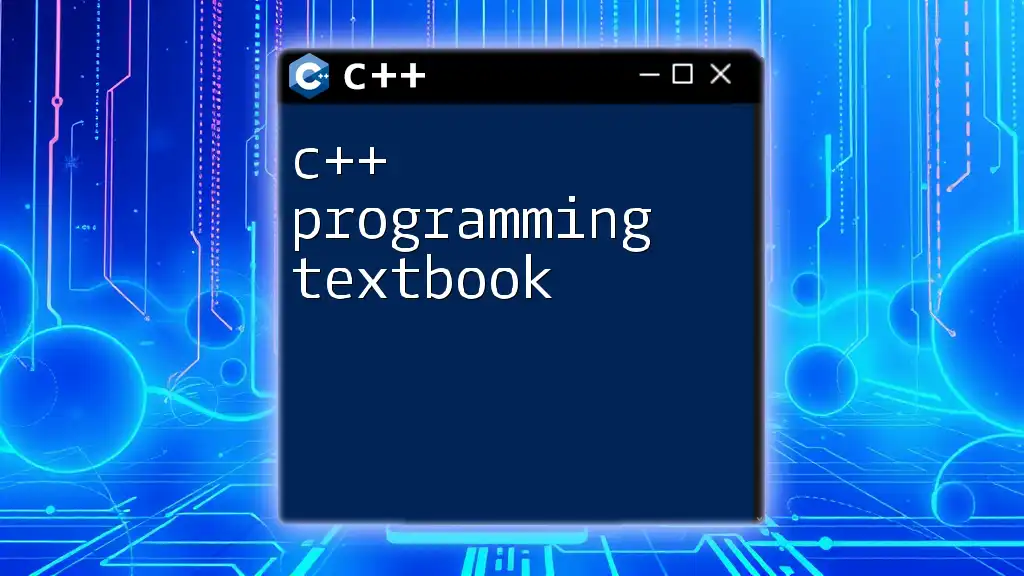
Expanding Your Skills: Introduction to 3D Game Programming
Overview of 3D Engines Using C++
If you want to delve into 3D game development, you might consider using engines like Unreal Engine or Unity (where you can use C++ alongside C#). Both engines allow for extensive customization and have large support communities.
Implementing Basic 3D Graphics
To create a basic 3D structure, understanding vector math is essential. Begin with mastering the concepts of points, vectors, and matrices, as they are fundamental in placing and rotating objects in 3D space.
Code Snippet: 3D Object Creation
An example of creating a 3D object, in pseudo-code, would look like this:
// Pseudo code for creating a 3D object
createMesh(vertices, indices);
This represents the step of defining an object through its geometric properties.
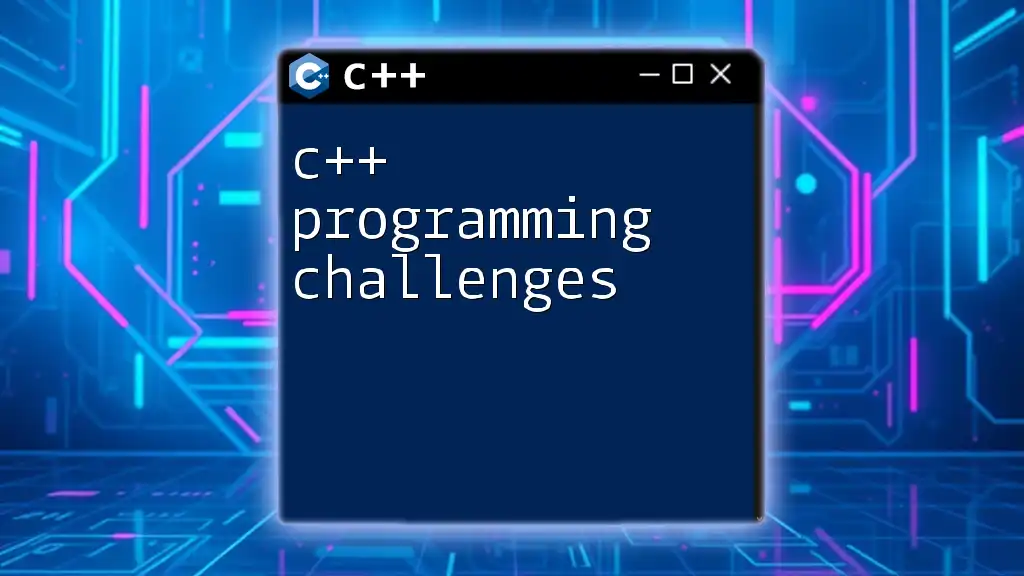
Debugging and Optimization Techniques
Common Bugs in Game Programming
As with any programming, bugs can be common, especially in complex games. Understanding typical issues—like memory leaks, infinite loops, or incorrect game states—will be valuable as you debug your code.
Debugging Tools and Techniques
Utilizing the built-in debugging features of your IDE can significantly streamline the process of identifying and fixing bugs. Breakpoints, watches, and call stacks are your allies in understanding your code's behavior.
Performance Optimization Tips
Game performance can suffer if not managed properly. Implement these practices:
- Memory management: Always allocate and deallocate memory wisely.
- Resource utilization: Load textures and sounds only when necessary, and dispose of resources when they are no longer needed.
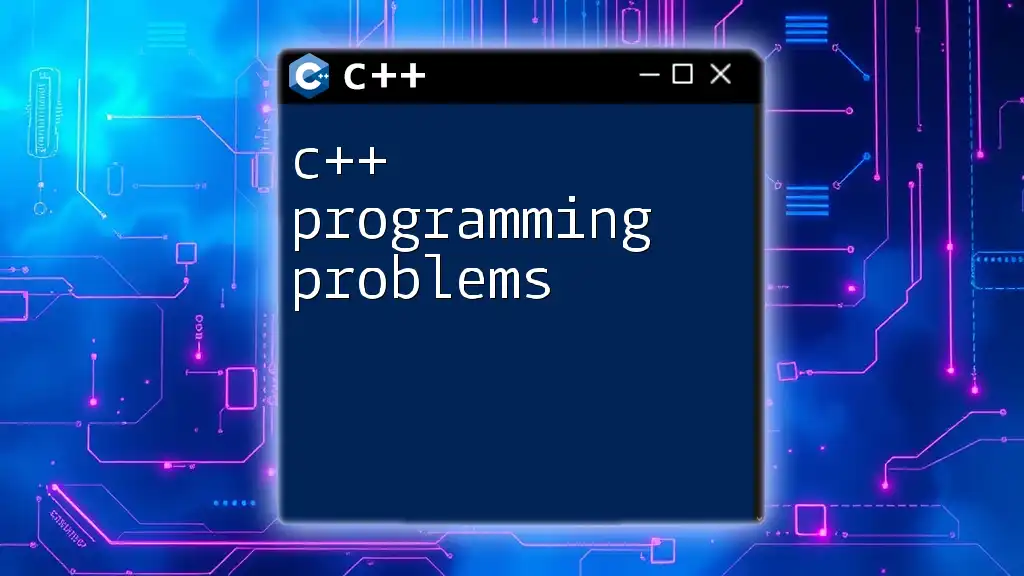
Best Practices for C++ Game Development
Writing Clean and Maintainable Code
Writing neat and maintainable code is essential. Use clear variable names, comment on complex logic, and maintain a consistent style throughout your project.
Version Control Systems for Game Projects
Using version control, like Git, is crucial in game development. It allows you to track changes, collaborate with others, and roll back to previous versions if something goes wrong.
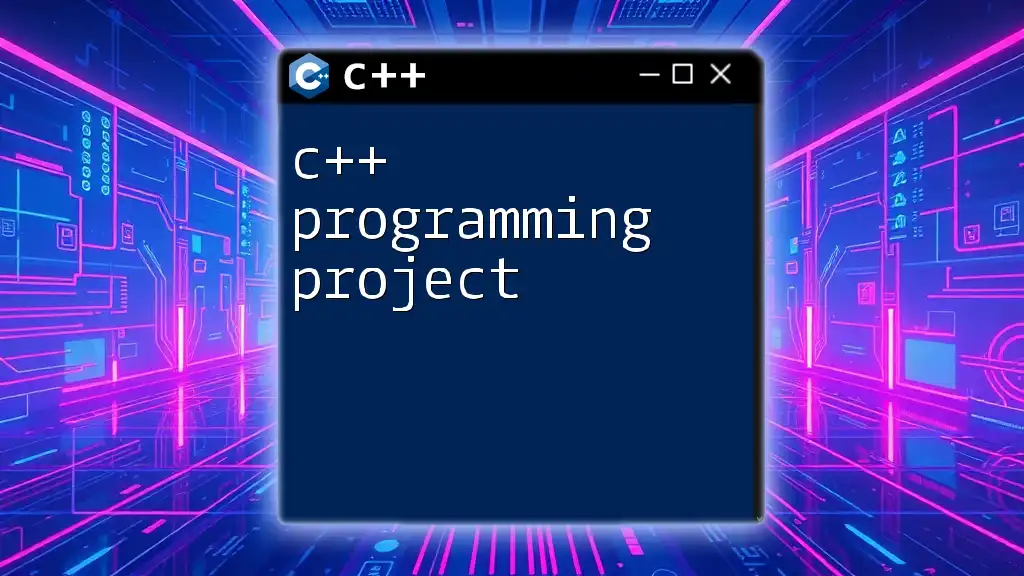
Conclusion: Continuing Your C++ Journey through Game Programming
Resources for Further Learning
To expand your knowledge, consider these resources:
- Books: Titles focusing on both C++ and game development can provide a deeper understanding.
- Online Courses: Platforms like Udemy or Coursera often have specialized C++ game programming courses.
- Tutorials and Forums: Engaging with the community through discussions or following tutorials can accelerate your learning.
Encouraging Your Next Steps
After mastering the basics, consider challenging yourself with more complex projects. Experiment with different genres, incorporate AI, or even develop a multiplayer game. Remember, the key to mastering C++ through game programming lies in practice and persistence.
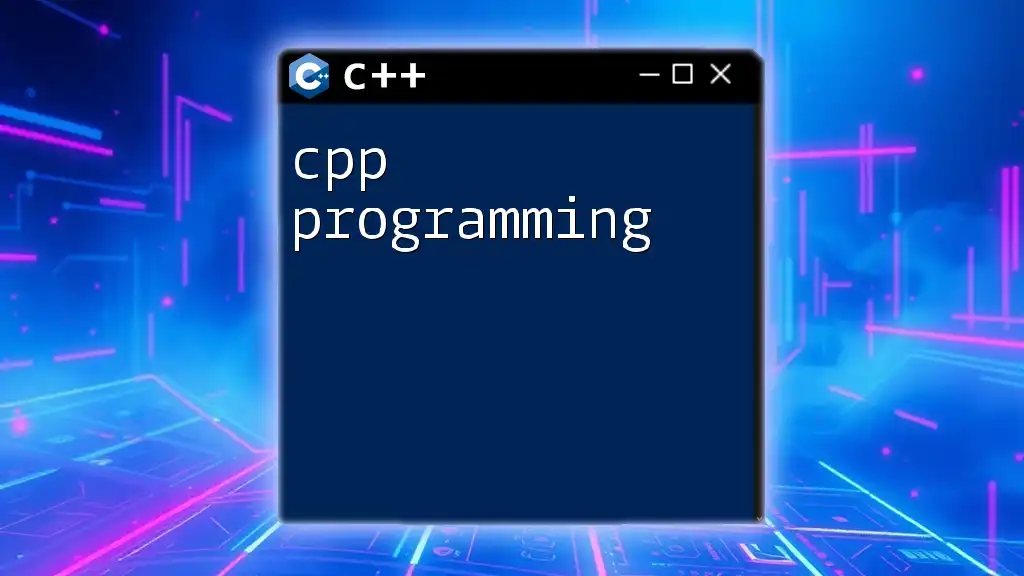
Call to Action
Join us and explore further learning opportunities as you continue your journey in mastering C++ through game programming. Stay tuned for more resources and insights to help you succeed in this exciting adventure!