C++ programming challenges provide practical exercises that help learners enhance their coding skills and problem-solving abilities through hands-on experience.
Here's a simple example of a C++ program that calculates the factorial of a number:
#include <iostream>
using namespace std;
int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "Factorial of " << num << " is " << factorial(num) << endl;
return 0;
}
What Are C++ Programming Challenges?
C++ programming challenges are tasks or problems designed to test and enhance your programming skills in C++. These challenges vary in complexity and format, ranging from simple exercises for beginners to complex algorithmic problems typically found in competitive programming.
Why Engage in C++ Coding Challenges?
Engaging in C++ coding challenges is crucial for several reasons:
- Skill Enhancement: Regular practice with programming challenges sharpens your coding abilities and enhances your problem-solving skills.
- Confidence Building: Successfully solving challenges boosts your confidence and prepares you for real-world programming situations.
- Networking Opportunities: Participating in challenges often connects you with other programmers, helping you to build a network within the tech community, allowing for collaboration and knowledge exchange.
- Career Benefits: Completing challenges showcases your dedication and proficiency, making your resume more appealing to potential employers.
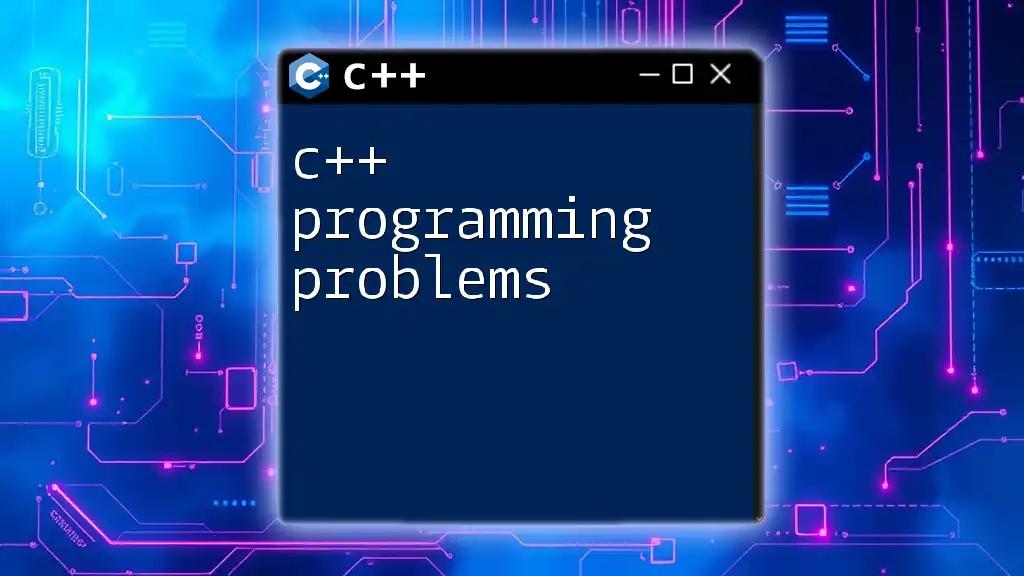
Types of C++ Programming Challenges
Beginner Challenges
Beginner challenges are designed to help new programmers grasp the basics of C++. These tasks often cover fundamental concepts such as control structures, loops, and basic functions.
Example: Write a program to calculate the factorial of a number.
#include <iostream>
using namespace std;
int factorial(int n) {
return (n == 1 || n == 0) ? 1 : factorial(n - 1) * n;
}
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
cout << "Factorial: " << factorial(num) << endl;
return 0;
}
Explanation: In this code snippet, the `factorial` function calculates the factorial of a given number `n` recursively. The base case returns 1 when `n` is 1 or 0. The `main` function prompts the user for input and displays the result.
Intermediate Challenges
Intermediate challenges require a grasp of data structures and algorithms, pushing you to apply your knowledge in practical scenarios.
Example: Create a program that sorts an array using the quicksort algorithm.
#include <iostream>
using namespace std;
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
void quicksort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quicksort(arr, low, pi - 1);
quicksort(arr, pi + 1, high);
}
}
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr) / sizeof(arr[0]);
quicksort(arr, 0, n - 1);
cout << "Sorted array: ";
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
cout << endl;
return 0;
}
Explanation: This code implements the quicksort algorithm. The `quicksort` function is called recursively to sort subarrays, relying on the `partition` function that rearranges the elements, ensuring smaller elements are positioned before the pivot. The `main` function initializes an array, calls `quicksort`, and then prints the sorted array.
Advanced Challenges
Advanced challenges tend to be the most intricate, often encountered in competitive programming contexts where efficiency and algorithmic strategy are paramount.
Example: Solve the N-Queens problem.
#include <iostream>
using namespace std;
bool isSafe(int board[][100], int row, int col, int N) {
for (int i = 0; i < col; i++)
if (board[row][i]) return false;
for (int i = row, j = col; i >= 0 && j >= 0; i--, j--)
if (board[i][j]) return false;
for (int i = row, j = col; j >= 0 && i < N; i++, j--)
if (board[i][j]) return false;
return true;
}
bool solveNQUtil(int board[][100], int col, int N) {
if (col >= N) return true;
for (int i = 0; i < N; i++) {
if (isSafe(board, i, col, N)) {
board[i][col] = 1;
if (solveNQUtil(board, col + 1, N)) return true;
board[i][col] = 0;
}
}
return false;
}
bool solveNQ(int N) {
int board[100][100] = {0};
if (solveNQUtil(board, 0, N) == false) {
cout << "Solution does not exist";
return false;
}
return true;
}
int main() {
int N = 4;
solveNQ(N);
return 0;
}
Explanation: This code attempts to place N queens on an NxN chessboard in such a way that no two queens threaten each other. The `isSafe` function checks whether a queen can be safely placed at a given position. The `solveNQUtil` function uses backtracking to find valid placements, while the `solveNQ` function initializes the board and starts the process.
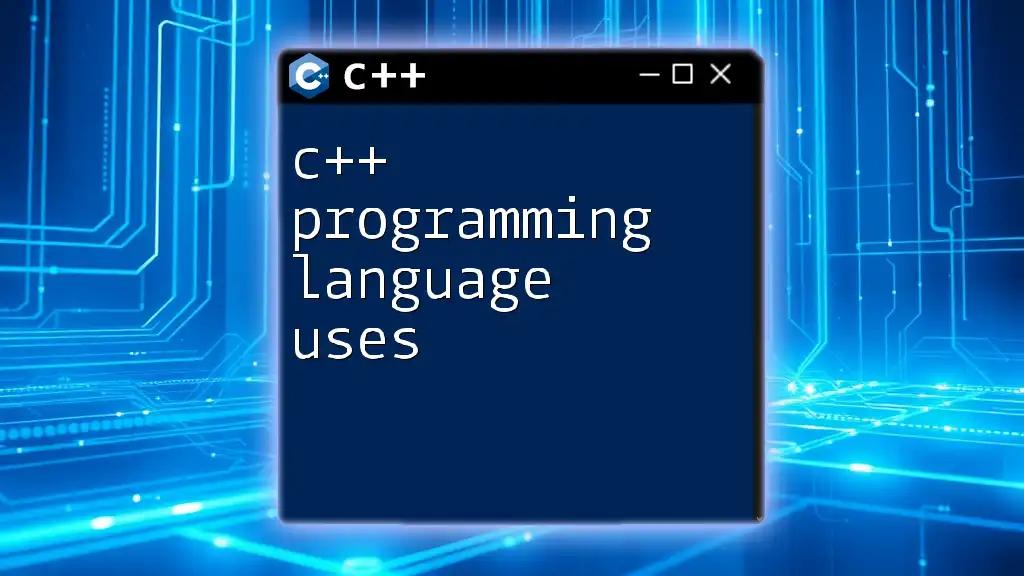
Where to Find C++ Coding Challenges
Online Platforms
A wealth of resources is available on various online platforms specifically structured for C++ programming challenges. Some of the most popular ones include:
- LeetCode: Offers a multitude of coding problems categorized by difficulty, along with discussions and solutions.
- Codewars: Provides a unique approach to coding challenges, allowing users to create and solve challenges at different skill levels.
- HackerRank: Features a variety of challenges that can ensure your coding skills are sharp and relevant for interviews.
Participating in these coding platforms not only enhances your C++ skills but also encourages interaction with a programming community.
Books and Resources
Additionally, several books provide excellent resources for practicing C++ challenges:
- “Programming Pearls” by Jon Bentley: This book contains numerous problems with insightful explanations that enhance problem-solving skills.
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: A perfect resource for those preparing for technical interviews, featuring a variety of common C++ problems and their solutions.
Moreover, consider joining online forums or communities where programmers share problems, solutions, and experiences.
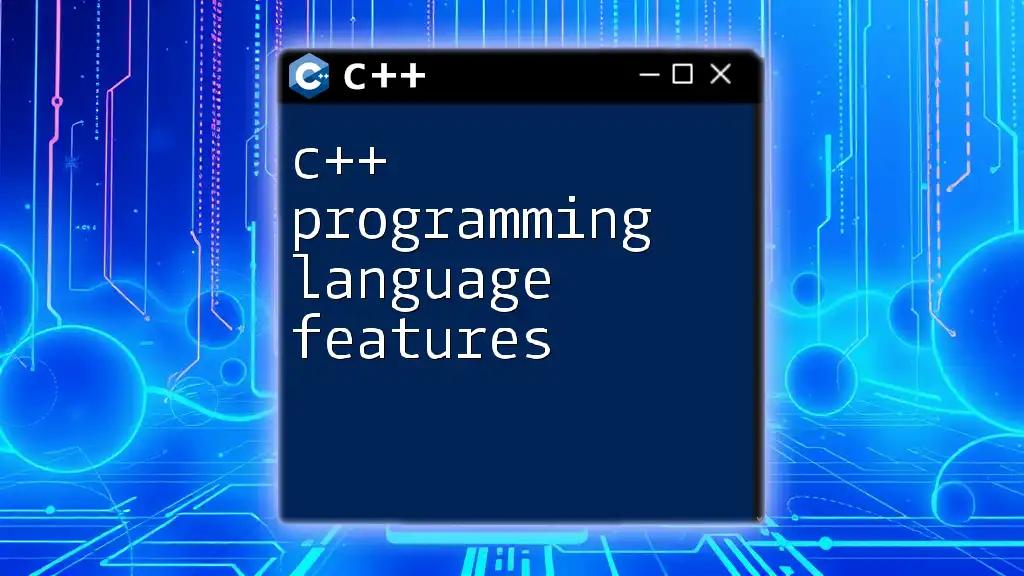
Tips for Success in C++ Programming Challenges
Structured Approach
To tackle challenges effectively, adopting a structured approach is essential. Always break down complex problems into smaller, manageable components. Before diving into coding, writing pseudocode can help clarify your thoughts and outline the solution effectively.
Practice and Persistence
Consistency is key. Make coding challenges a regular part of your learning routine. Don’t be discouraged by initial failures; each mistake is an opportunity to learn. The more diverse the challenges you tackle, the broader your understanding of C++ will become.
Revision of Concepts
Regular revision of C++ concepts is crucial for success. Instead of memorizing, focus on understanding the logic behind specific algorithms or data structures. This foundational knowledge will pay off when faced with real-world programming problems.
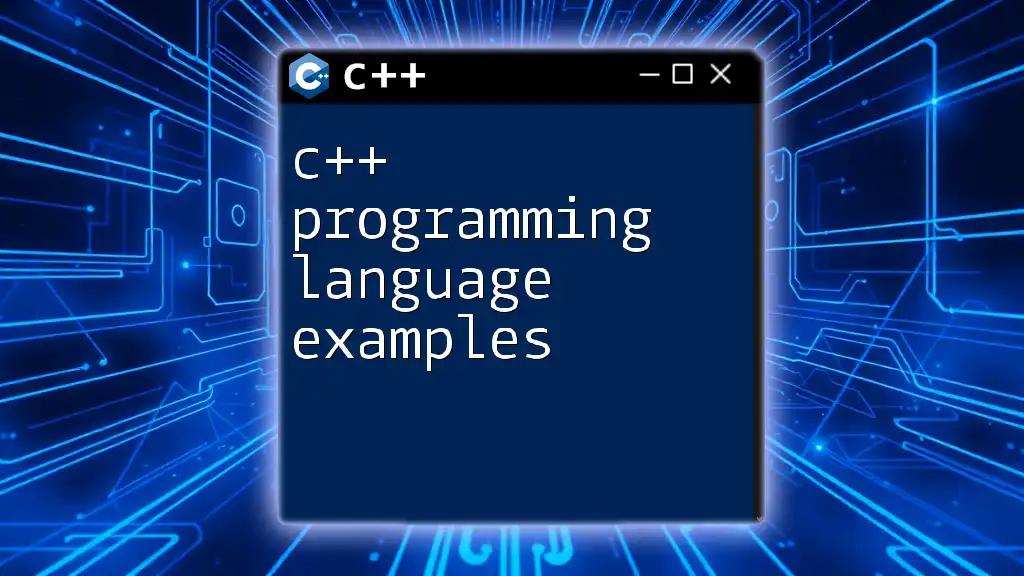
Conclusion
C++ programming challenges play a pivotal role in developing your coding abilities. By actively engaging with these challenges, you cultivate critical skills that will benefit you in both academic and professional settings. Start practicing, embrace the challenges, and watch your confidence and competence in C++ grow tremendously.
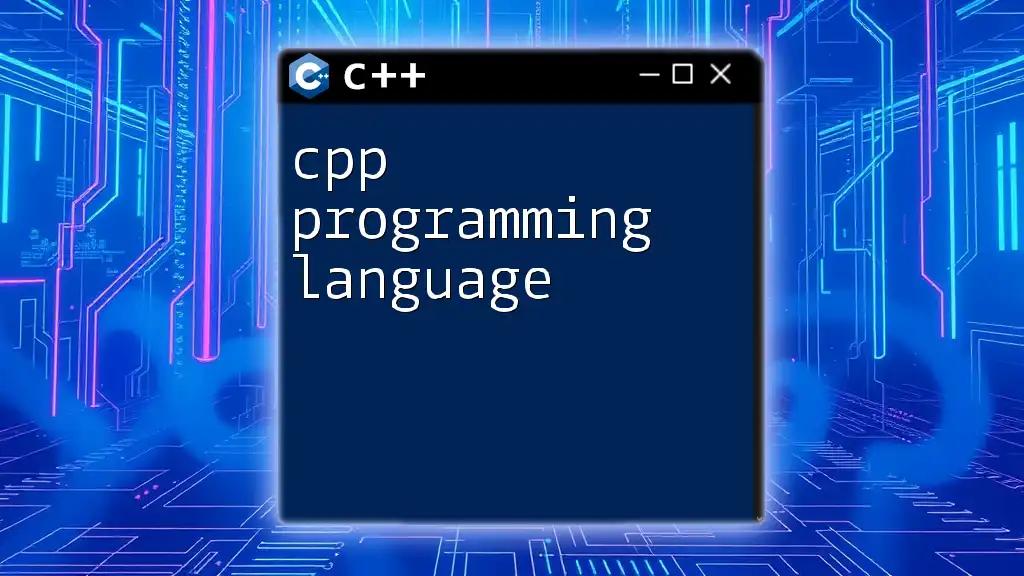
Call to Action
Sign up for our C++ challenge series today and subscribe for more tips and resources on mastering C++ programming. Participate actively, and you will soon be on your way to becoming a proficient C++ coder!