C++ programming examples demonstrate the essential syntax and functionalities of the C++ language, allowing learners to quickly grasp concepts through practical code snippets.
Here’s a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++
What is C++?
C++ is a general-purpose programming language that was developed by Bjarne Stroustrup at Bell Labs in the early 1980s as an enhancement of the C programming language. It incorporates features such as object-oriented programming, strong type checking, and a rich standard library. C++ is widely used in software development, systems programming, and game development due to its performance and flexibility.
Setting Up Your C++ Environment
Before diving into C++ programming examples, it’s essential to have the right environment set up. Here are some recommended IDEs and compilers:
-
Integrated Development Environments (IDEs):
- Visual Studio (Windows)
- Code::Blocks (Cross-platform)
- Qt Creator (Cross-platform)
- Eclipse CDT (Cross-platform)
-
Compilers:
- g++ (GNU Compiler Collection)
- clang (LLVM)
Follow the installation steps specific to your operating system to get started.
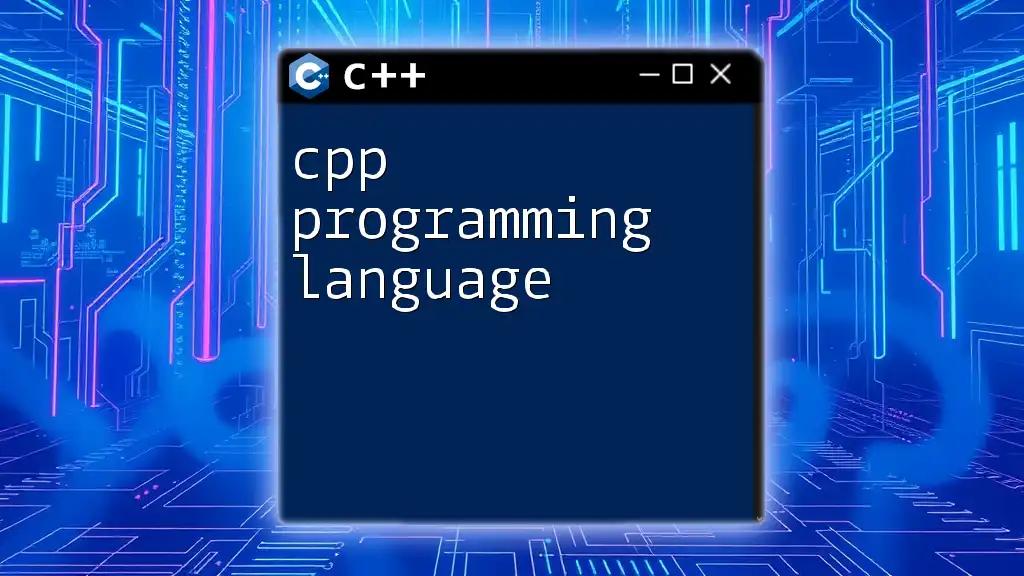
Basic C++ Syntax
Understanding the Structure of a C++ Program
A C++ program typically consists of several components. Here’s a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation:
- `#include <iostream>`: This statement includes the necessary header file for input and output operations.
- `int main()`: This denotes the main function, where program execution begins.
- `std::cout << "Hello, World!" << std::endl;`: This line outputs "Hello, World!" to the console.
- `return 0;`: This indicates that the program has finished successfully.
Data Types in C++
C++ supports a variety of fundamental data types, which are essential for defining variables.
Examples include:
- int for integers
- float for floating-point numbers
- char for characters
- bool for boolean values
Here’s how to declare and initialize variables:
int number = 10;
float pi = 3.14;
char letter = 'A';
Selecting appropriate data types is vital as it impacts memory consumption and performance.
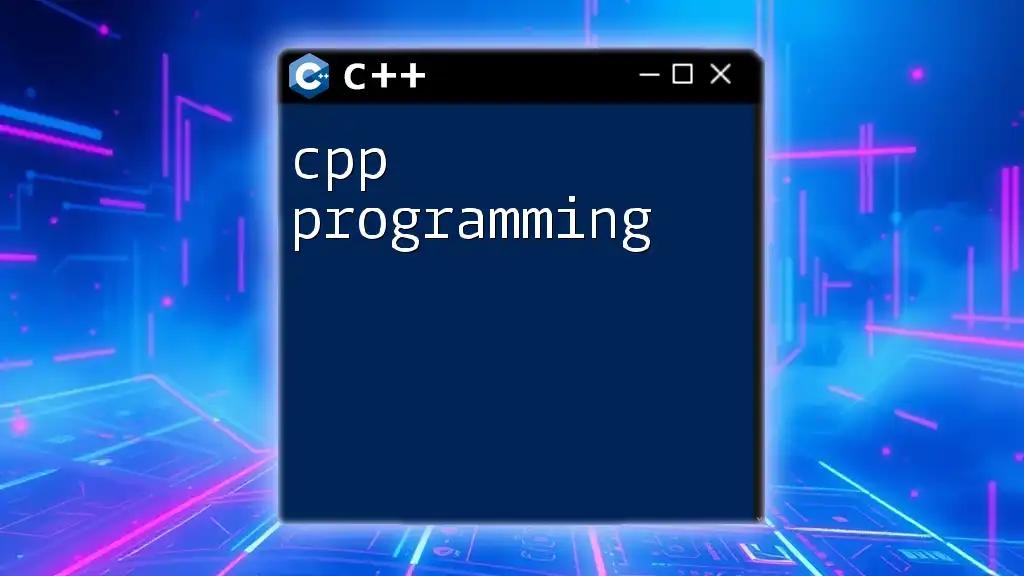
Control Structures
Conditional Statements
Conditional statements allow your program to make decisions. Here’s a breakdown using `if`, `else if`, and `else`:
int age = 20;
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
Explanation:
- The program checks the condition `age >= 18`. If true, it outputs "Adult"; if false, it outputs "Minor".
Loops
For Loop
The `for` loop is a control structure that allows for repeated execution based on a condition:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Explanation:
- Initializes `i` to `0`, checks that it's less than `5`, and increments `i` by `1` in each iteration. The output will be numbers 0 through 4.
While Loop
The `while` loop continues execution as long as a condition remains true:
int i = 0;
while (i < 5) {
std::cout << i << std::endl;
i++;
}
Explanation:
- This loop will output 0 to 4 as long as `i` is less than `5`.
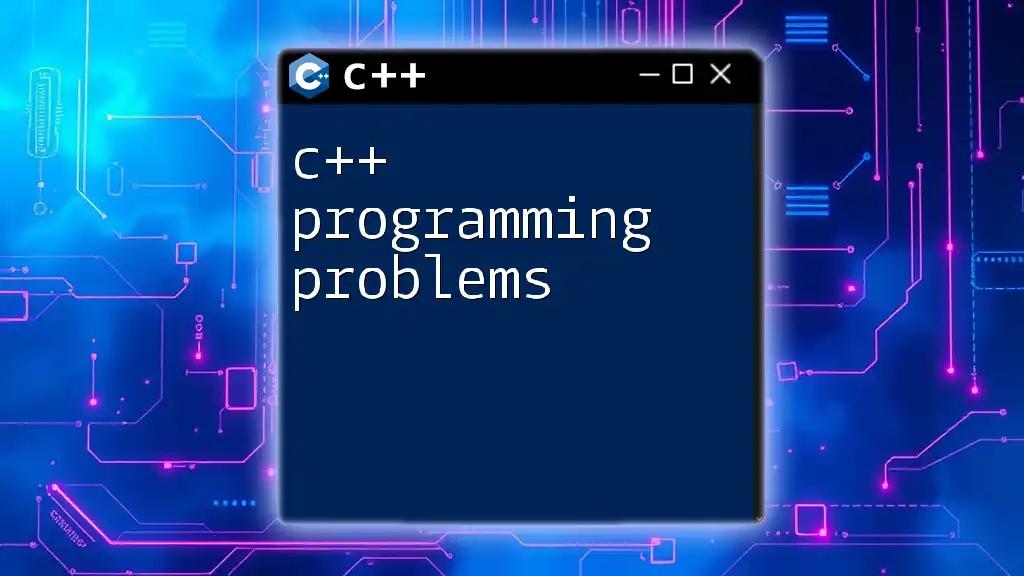
Functions in C++
Defining and Calling Functions
Functions in C++ encapsulate code for modularization and reuse. Here’s a simple function:
void greet() {
std::cout << "Hello!" << std::endl;
}
int main() {
greet();
return 0;
}
Explanation:
- The `greet()` function is declared and can be called in the `main()` function. This allows you to maintain organized code.
Function Parameters and Return Types
Functions can take arguments and return values. Here’s an example of a function that adds two integers:
int add(int a, int b) {
return a + b;
}
int main() {
int sum = add(5, 3);
std::cout << "Sum: " << sum << std::endl;
return 0;
}
Explanation:
- The `add` function takes two parameters, calculates their sum, and returns the result. The use of parameters allows for a more flexible and reusable function.
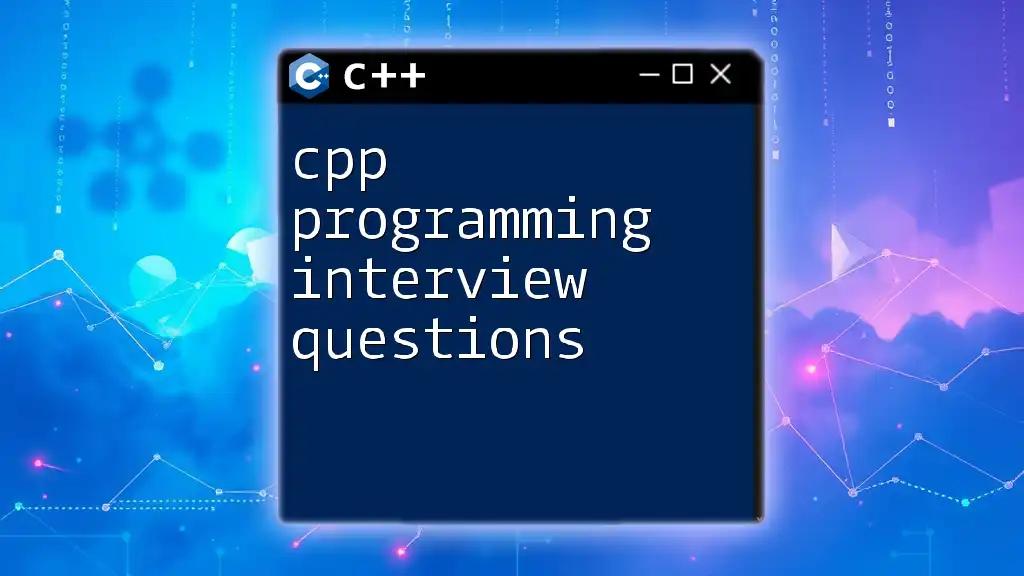
Object-Oriented Programming in C++
Classes and Objects
C++ enables the use of classes and objects to promote encapsulation and modular code:
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
int main() {
Animal dog;
dog.speak();
return 0;
}
Explanation:
- The `Animal` class has a public method `speak()`. In the `main()` function, an object of type `Animal` is created and the method is called.
Inheritance
Inheritance allows one class to inherit attributes and methods from another, promoting code reuse:
class Dog : public Animal {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog dog;
dog.speak(); // Inherited from Animal
dog.bark(); // Dog specific method
return 0;
}
Explanation:
- The `Dog` class inherits from the `Animal` class, allowing it to access the `speak()` method while also defining its own `bark()` method.
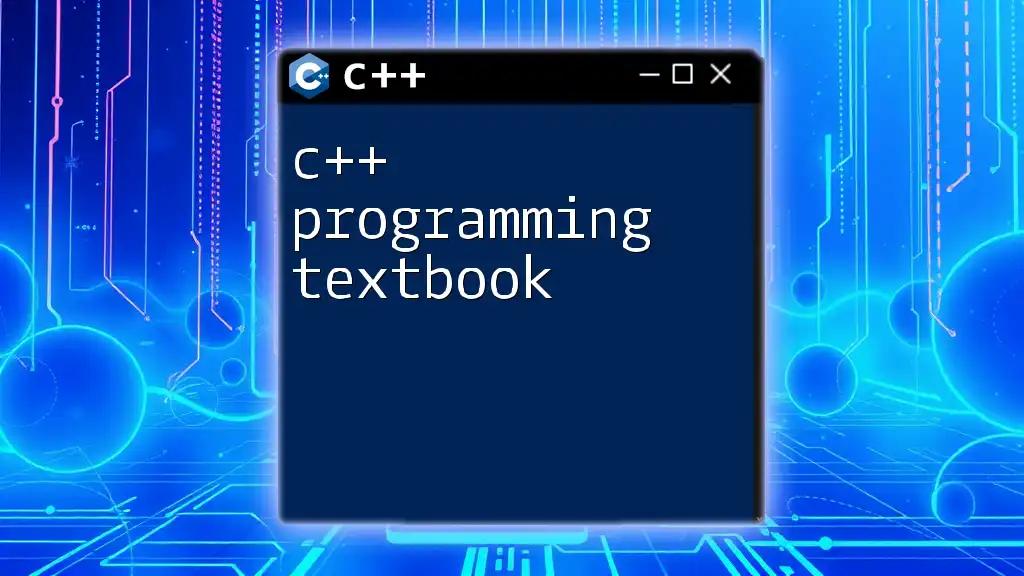
Advanced C++ Concepts
Templates
Templates enhance code reusability and type safety. Here’s a function template example:
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
std::cout << add<int>(5, 3) << std::endl; // returns 8
return 0;
}
Explanation:
- The `add` function is defined using a template so it can operate on different data types, showcasing how templates provide flexibility in defining functions.
Exception Handling
Proper error management is crucial in programming. C++ uses try-catch blocks for exception handling:
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cout << e.what() << std::endl;
}
Explanation:
- The `try` block contains code that may throw an exception. In this case, it throws a runtime error, which is caught in the `catch` block, allowing the program to handle errors gracefully.
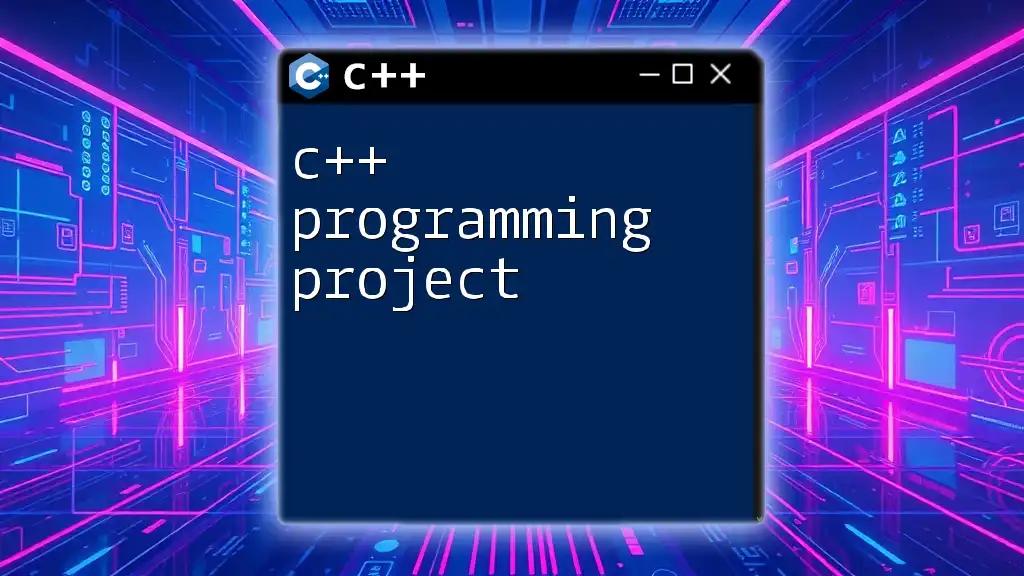
Conclusion
By exploring these C++ programming examples, you have discovered the essential components and structures that form the backbone of C++ programming. Applying these concepts in practical scenarios will enhance your coding skills, providing a solid foundation in C++. Embrace practice and experiment with writing your own code to reinforce your understanding.
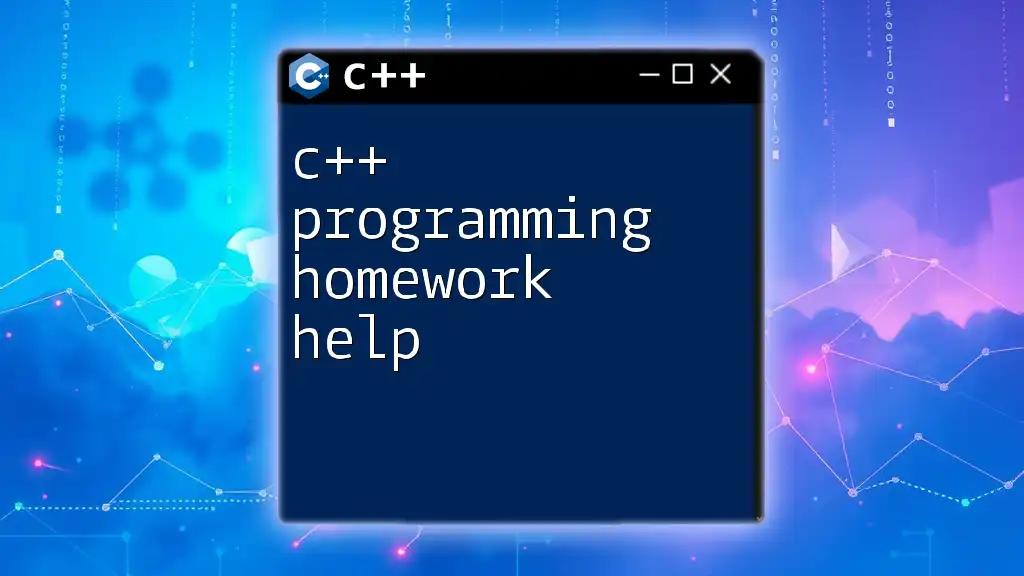
Additional Resources
Recommended C++ Learning Resources
To deepen your knowledge, consider exploring:
- Books: "C++ Primer" and "Effective C++"
- Online Courses: Sites like Coursera and Udemy
- Tutorials: Check out resources such as Codecademy and freeCodeCamp
Common C++ Libraries
Familiarize yourself with popular libraries:
- STL (Standard Template Library)
- Boost
- Qt for GUI application development
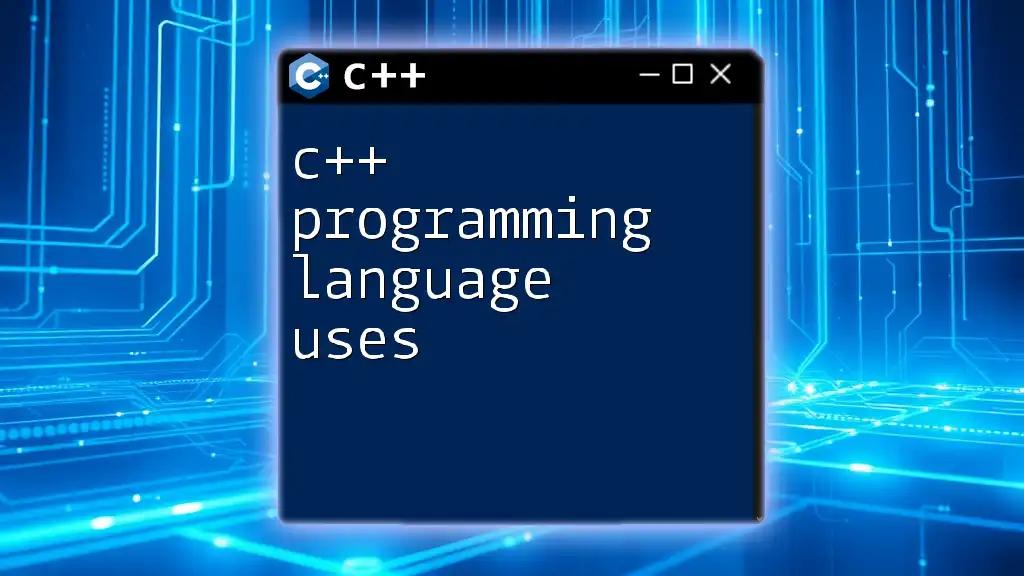
Call to Action
Do you have your own C++ projects or examples? Share your experience with the community! Subscribe for more updates and join our growing network of C++ enthusiasts to enhance your programming journey.