In this post, we will explore essential C++ programming interview questions that evaluate your understanding of the language's syntax and features, providing succinct explanations and examples. Here's a simple code snippet that demonstrates basic C++ syntax for defining a class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Understanding C++ Programming Concepts
Key C++ Features
C++ is a powerful programming language that facilitates various programming styles through its features. Among the key concepts are:
- Object-Oriented Programming (OOP): This paradigm allows developers to create modular programs based on objects, making code more reusable and easier to manage.
- Memory Management: C++ provides developers with manual memory management capabilities, which can lead to efficient memory usage but also requires a solid understanding to avoid memory leaks.
- Templates and STL (Standard Template Library): Templates facilitate generic programming, while the STL provides essential data structures and algorithms for efficient data handling.
Importance of Fundamentals
A solid grasp of fundamental principles is essential not only for coding challenges but also for demonstrating your understanding during interviews. Familiarity with programming paradigms like procedural vs object-oriented programming can help distinguish you from other candidates. Understanding basic syntax, algorithm efficiency, and memory architecture will further reinforce your expertise and readiness for cpp programming interview questions.
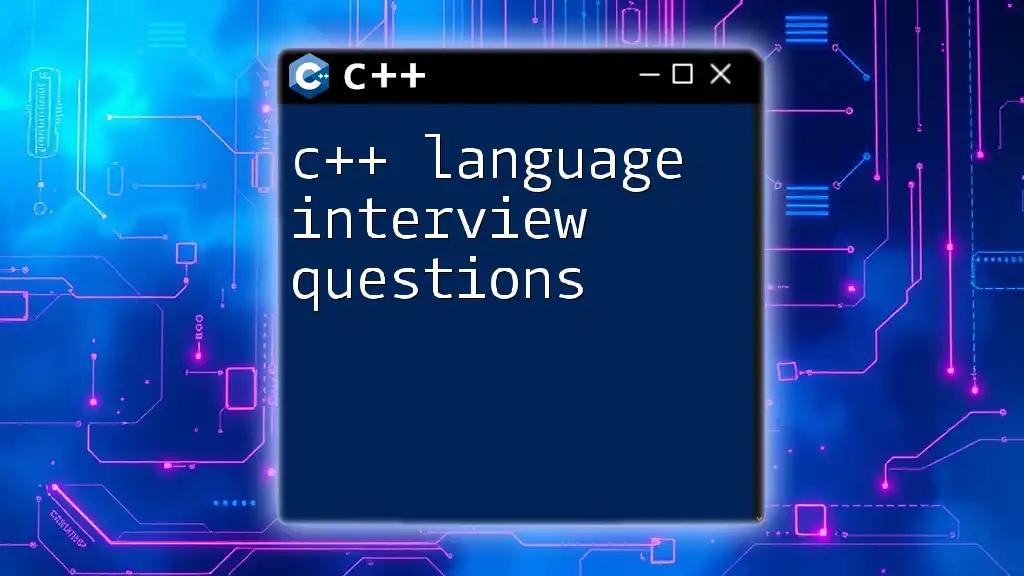
Common C++ Interview Questions
Basics of C++
What is C++?
C++ is an extension of the C programming language, first developed by Bjarne Stroustrup in 1979. It combines the efficiency of low-level programming with the features of high-level programming, such as:
- Encapsulation: Bundling of data and methods that operate on that data.
- Inheritance: Mechanism for creating new classes based on existing ones.
- Polymorphism: Ability for functions or methods to process objects differently based on their data type or class.
Understanding the key differences between C and C++ is vital, as C++ has richer features, especially in object-oriented programming.
What are the basic data types in C++?
C++ provides several built-in data types, crucial for variable declarations and memory allocation:
- int: Integer type, typically used for whole numbers.
- char: Character type for single characters.
- float: Single-precision floating-point type.
- double: Double-precision floating-point type.
- bool: Boolean type used for true or false values.
Here is an example of variable declarations using these data types:
int age = 30;
char grade = 'A';
float height = 5.9;
double salary = 50000.50;
bool isEmployed = true;
Object-Oriented Programming
What are Classes and Objects?
Classes in C++ serve as blueprints for creating objects, encapsulating data and functions. An object represents an instance of a class.
Example code snippet:
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2022;
myCar.displayInfo();
return 0;
}
This code defines a `Car` class and creates an instance of it. Demonstrating your ability to create and manipulate classes and objects is crucial during interviews.
Explain Inheritance in C++
Inheritance allows one class to inherit properties and methods from another, promoting code reusability. C++ supports various inheritance types, such as:
- Single Inheritance: One class inherits from another.
- Multiple Inheritance: A class can inherit from multiple classes.
- Multilevel Inheritance: Inheritance forms a chain of classes.
- Hierarchical Inheritance: Multiple classes inherit from a single base class.
Example Code:
class Vehicle {
public:
void honk() {
cout << "Honk!" << endl;
}
};
class Car : public Vehicle {
public:
void display() {
cout << "This is a car." << endl;
}
};
int main() {
Car myCar;
myCar.honk(); // Inherited method
myCar.display(); // Car-specific method
return 0;
}
Demonstrating your understanding of inheritance in C++ can significantly enhance your responses to cpp programming interview questions.
What is Polymorphism?
Polymorphism allows functions to act differently based on the type of input. It can be categorized into:
- Compile-time Polymorphism (Method Overloading): The same function name can operate on different data types or parameter counts.
class Overload {
public:
void print(int i) {
cout << "Integer: " << i << endl;
}
void print(double d) {
cout << "Double: " << d << endl;
}
};
- Runtime Polymorphism (Method Overriding): A base class reference is used to invoke derived class methods.
class Base {
public:
virtual void show() {
cout << "Base class show function called." << endl;
}
};
class Derived : public Base {
public:
void show() override {
cout << "Derived class show function called." << endl;
}
};
int main() {
Base* b; // Base class pointer
Derived d; // Derived class object
b = &d; // Base class pointer points to derived class
b->show(); // Calls the overridden function
return 0;
}
Understanding polymorphism can be a significant advantage in cpp programming interview questions.
Advanced C++ Concepts
What is a Smart Pointer?
Smart pointers manage the lifecycle of dynamically allocated objects. They automatically deallocate memory when no longer in use. Types of smart pointers include:
- unique_ptr: Ensures a single owner for a dynamically allocated object.
- shared_ptr: Allows multiple pointers to own the same object, keeping a reference count to manage memory.
- weak_ptr: Used in conjunction with shared_ptr, it prevents circular references.
Example of `shared_ptr`:
#include <iostream>
#include <memory>
using namespace std;
int main() {
shared_ptr<int> ptr1 = make_shared<int>(10);
{
shared_ptr<int> ptr2 = ptr1; // ptr2 shares ownership of ptr1
cout << *ptr2 << endl; // Outputs 10
} // ptr2 goes out of scope, but ptr1 remains valid
cout << *ptr1 << endl; // Outputs 10
return 0;
}
Understanding smart pointers is crucial for effective memory management and showcasing your C++ knowledge during interviews.
Templates in C++
Templates provide a way to create functions and classes that work with any data type. This feature helps in creating reusable code.
Example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << add<int>(3, 4) << endl; // Outputs 7
cout << add<double>(3.5, 2.5) << endl; // Outputs 6.0
return 0;
}
Utilizing templates effectively demonstrates your coding proficiency and versatility when responding to cpp programming interview questions.
Common Algorithms and Data Structures
Implementing a Linked List
Linked lists are data structures consisting of nodes, where each node comprises data and a pointer to the next node. They are crucial in understanding dynamic data allocation.
Example of a simple singly linked list implementation:
class Node {
public:
int data;
Node* next;
};
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
void insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
}
void display() {
Node* temp = head;
while (temp != nullptr) {
cout << temp->data << " -> ";
temp = temp->next;
}
cout << "null" << endl;
}
};
int main() {
LinkedList list;
list.insert(5);
list.insert(10);
list.display(); // Outputs: 10 -> 5 -> null
return 0;
}
Mastering such data structures is essential for understanding core concepts and for successfully navigating cpp programming interview questions.
Sorting Algorithms
Sorting algorithms are foundational in computer science. Understanding how these algorithms work and their time complexities is crucial.
For instance, the QuickSort algorithm uses divide-and-conquer principles. Here's a basic implementation:
void quickSort(vector<int>& arr, int left, int right) {
int i = left, j = right;
int pivot = arr[(left + right) / 2];
while (i <= j) {
while (arr[i] < pivot) i++;
while (arr[j] > pivot) j--;
if (i <= j) {
swap(arr[i], arr[j]);
i++;
j--;
}
}
if (left < j) quickSort(arr, left, j);
if (i < right) quickSort(arr, i, right);
}
int main() {
vector<int> arr = {3, 6, 8, 10, 1, 2, 1};
quickSort(arr, 0, arr.size() - 1);
for(int num : arr) {
cout << num << " ";
}
return 0;
}
Being able to explain and implement sorting algorithms will showcase your technical capabilities in cpp programming interviews.
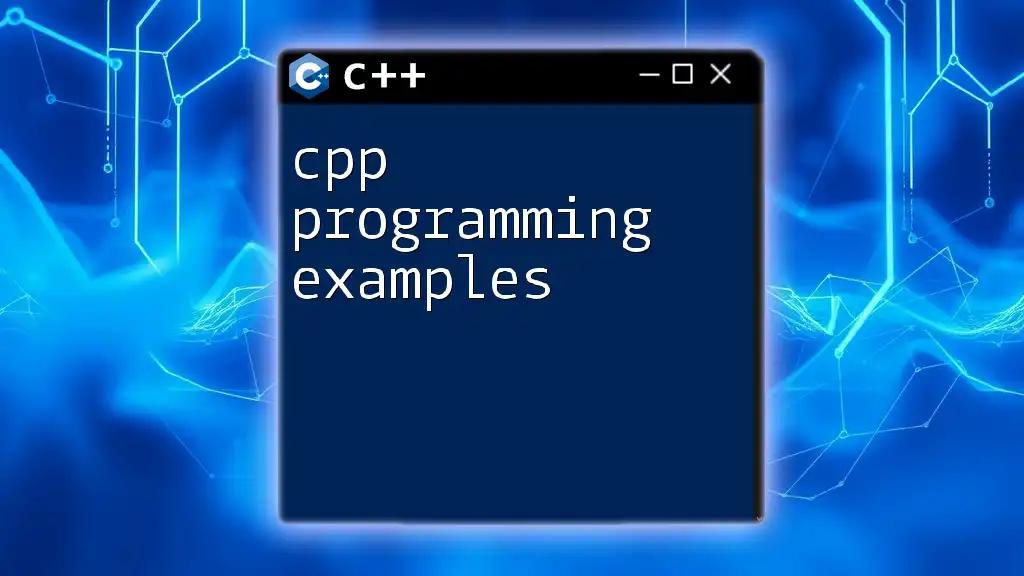
Behavioral Questions in C++ Interviews
Problem-Solving Approaches
During interviews, problem-solving is key. Begin by clarifying the problem. Break it down into smaller, manageable components. Always communicate your thought process clearly to interviewers, showing how you arrive at solutions.
Discussing Past Projects
When discussing your projects, focus on specific challenges you faced and how you overcame them. Highlight your contributions and the technologies used. Tailoring your examples to include C and C++ elements can emphasize your programming journey.

Tips for C++ Programming Interviews
Communication is Key
Effective communication can set you apart from fellow candidates. Practice articulating your thought process, as interviewers often gauge your problem-solving skills through your explanation.
Practice Makes Perfect
Empower your preparation by consistently practicing coding challenges. Leverage platforms like LeetCode and HackerRank. Simulating timed coding sessions will enhance your ability to work under pressure during real interviews.
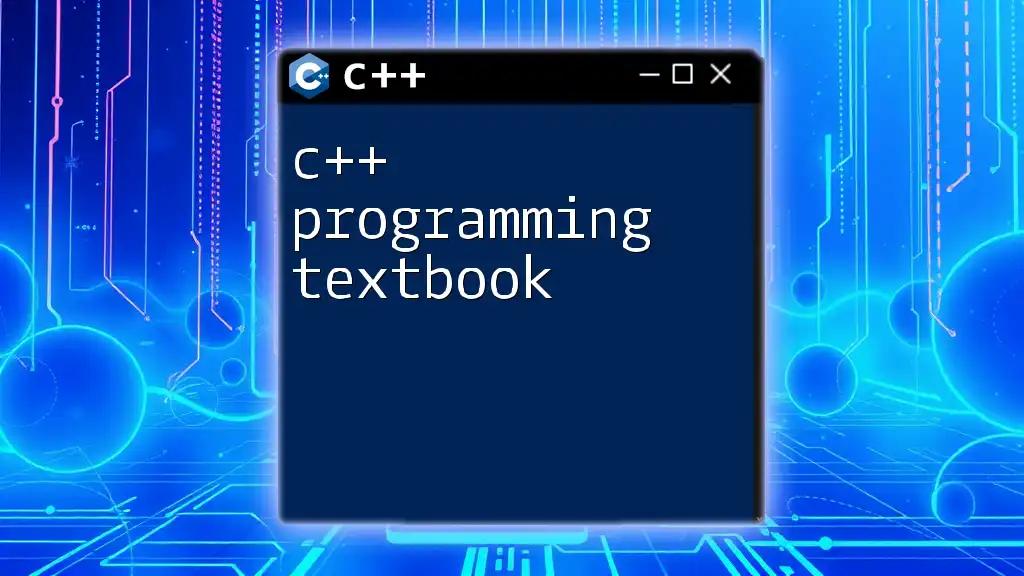
Conclusion
The world of C++ programming interview questions can seem daunting, but a strong foundation in core concepts, problem-solving strategies, and practical coding skills will prepare you effectively. Continuous practice and learning will bolster your confidence and competence in tackling C++ challenges.
Additional Resources
To extend your C++ knowledge, explore recommended books and online courses. Engage with programming forums and local communities to gain insights and further your skills.
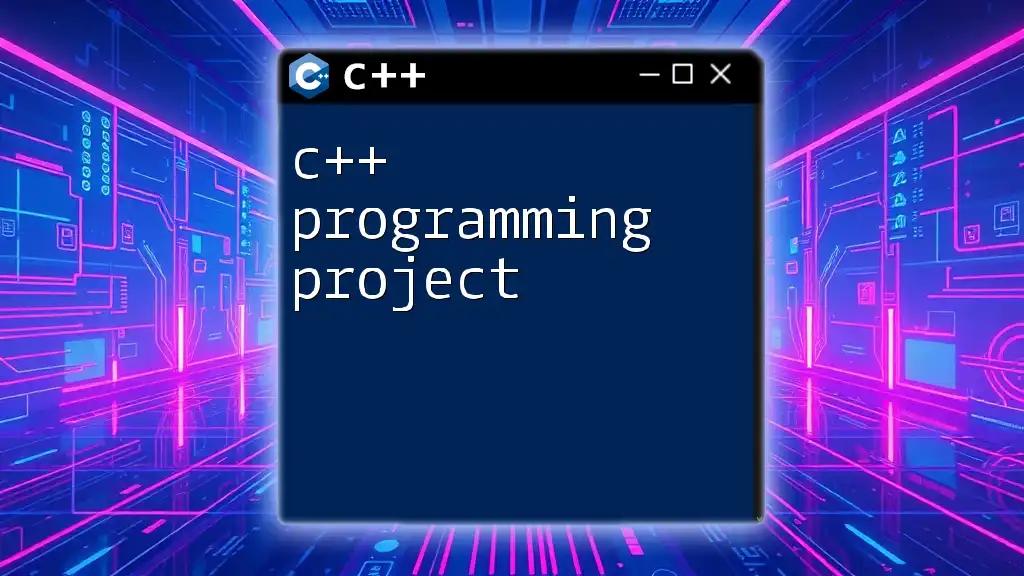
FAQs about C++ Programming Interviews
Frequently Asked Questions
-
What should I focus on during my preparation? Focus on core concepts, data structures, algorithms, and object-oriented programming principles.
-
How can I improve my coding speed? Regular practice and timed exercises can significantly improve your coding speed and thought processes.
-
What common mistakes should I avoid during interviews? Avoid jumping to conclusions without understanding the problem, and ensure your code is clean and well-documented.