C++ program execution involves compiling the source code into machine code and then running this compiled code to perform the desired operations.
Here's a simple code snippet that illustrates a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Program Execution
What is Program Execution?
Program execution refers to the process through which a program is run by the computer. This entails converting code written in C++ into an executable format that the machine can comprehend and process. Understanding this process is crucial for programmers, as it directly affects how efficiently a program operates and how errors are managed during its runtime.
Compilation vs. Interpretation
C++ is a compiled language, which means that its source code must first be translated into machine code through a compiler before it can be executed. This contrasts with interpreted languages, where code is executed line-by-line.
Compilation: Definition and Process
Compilation involves several distinct stages, each of which transforms the high-level C++ code into machine-level instructions. This includes preprocessing, compilation, and linking, as we’ll explore in greater detail.
Interpretation: Definition and Process
While C++ relies on compilation, some other languages (like Python) interpret code. Interpreted programs can be executed more flexibly; however, they often face performance issues compared to compiled programs due to this real-time processing.
The Compilation Process
Preprocessing
Before the actual compilation begins, the C++ compiler performs preprocessing. This step involves handling directives that begin with a `#`. Common directives include:
- `#include` – Used to include header files.
- `#define` – Creates macros.
An example of a simple preprocessor directive is:
#include <iostream>
This line tells the compiler to include the standard input-output library, allowing the use of `std::cout` for displaying output.
Compilation
After preprocessing, the compiler translates the preprocessed code into an intermediate object code. Key components of this stage are:
- Syntax Analysis: The compiler checks the syntax of the code to ensure it adheres to C++ standards.
- Semantic Analysis: This involves evaluating the meaning of the statements and validating that the operations performed are correct.
For example, compiling a simple C++ code snippet:
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The compiler analyzes this code for syntactic correctness and prepares it for the linking stage.
Linking
Linking merges the compiled object files into a single executable. There are two types of linking:
- Static Linking: All necessary library code is included in the executable. As a result, the executable is larger but can run independently.
- Dynamic Linking: The executable only contains references to the libraries, which are loaded during runtime. This approach reduces the executable size but relies on the presence of the required libraries.
To demonstrate linking, let's say we use a static library for mathematical functions. The linking process involves merging these functions with our main program.
Example: From Source Code to Executable
To illustrate the process of creating an executable from source code, consider the following steps:
- You write a program called `hello.cpp`.
- You compile it with a simple command:
g++ hello.cpp -o hello
-
This command generates an executable file called `hello`.
-
Running the executable can be done by:
./hello
The output will display:
Hello, World!
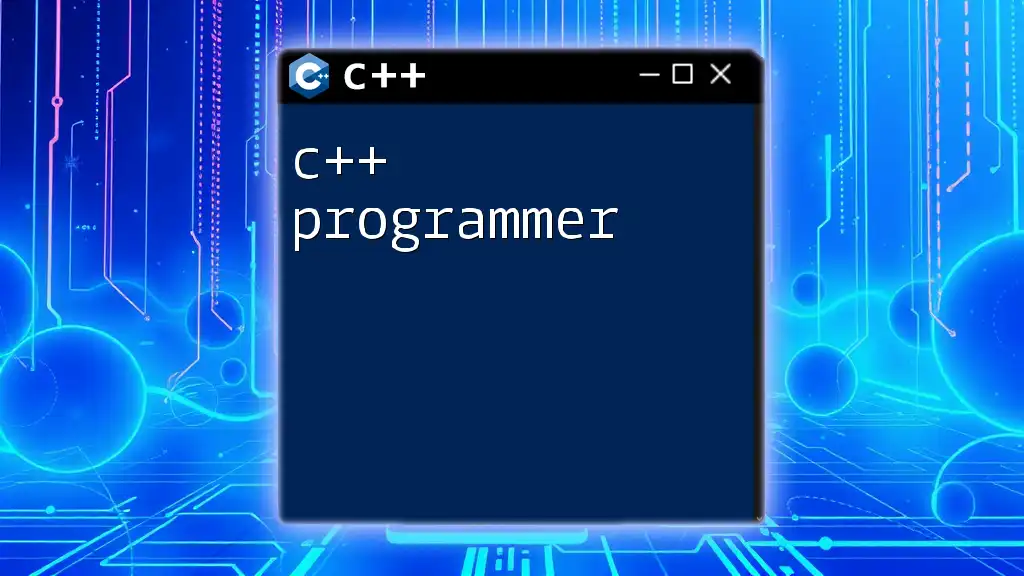
Execution of C++ Programs
Running the Executable
An executable file is the output of the compilation process and contains machine-code instructions that the computer can execute directly. To run your compiled C++ program, open your command line interface and use:
./hello
This command initiates the program, and the C++ runtime environment takes over.
Runtime Environment
The runtime environment is crucial during the execution of any C++ program. It manages resources and organizes how the program interacts with the operating system and hardware. Key components to consider include:
- Memory Management: Allocating memory correctly is vital to avoid memory leaks or overflow.
- Stack vs. Heap: The stack is used for static memory allocation, while the heap is used for dynamic memory allocation. Understanding when to use each can optimize your program's performance.
Whenever variables are created, the runtime environment allocates memory for them, and these variables span the life of the program's execution.
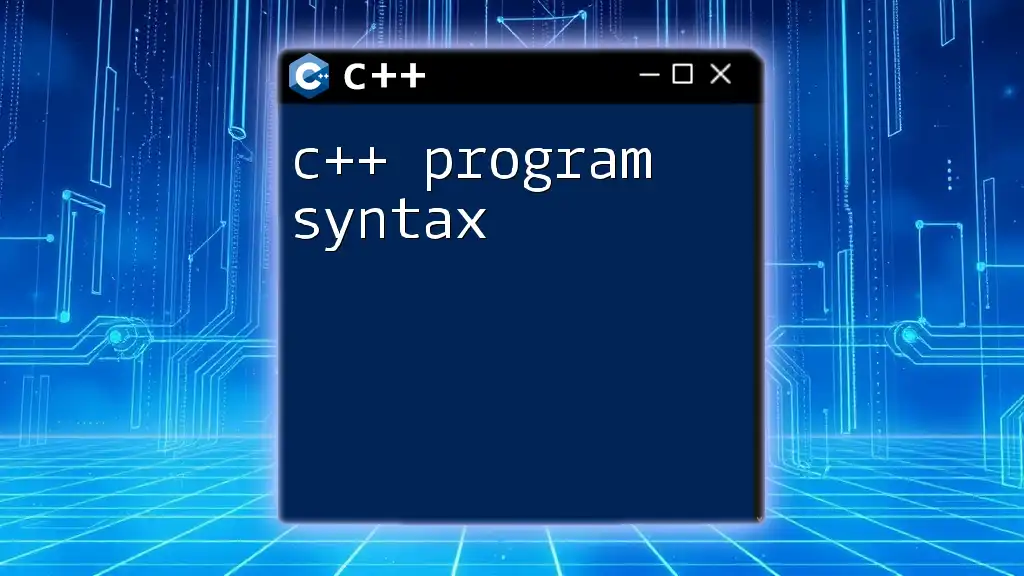
Debugging and Error Handling
Common Execution Errors
During the execution of a C++ program, you may encounter various errors. It's essential to be aware of the two main types:
-
Compile-time Errors: These are syntax errors caught by the compiler. For instance, forgetting a semicolon can lead to a compile-time error.
-
Runtime Errors: These errors only show up when the program runs. An example could be trying to access an out-of-bounds element in an array.
Handling these errors effectively is crucial for developing robust applications.
Tools for Debugging
Debugging is an integral part of C++ programming. Tools like `gdb` (GNU Debugger) and `valgrind` can help identify errors or performance issues during execution. Using `gdb`, you can run your program in debug mode:
gdb ./hello
This allows you to set breakpoints, inspect variables, and step through code to find errors efficiently.
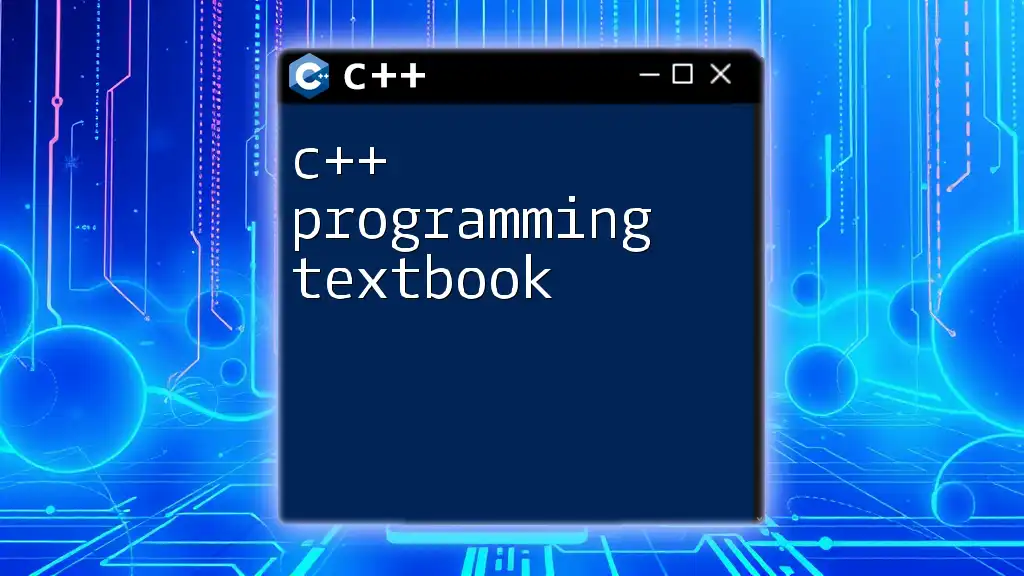
Optimizing C++ Program Execution
Improving Compilation Time
Long compilation times can slow down development. Here are some tips to mitigate this:
- Use precompiled headers (PCH): This technique compiles frequently used headers only once, speeding up the process for subsequent compilations.
Runtime Optimization Techniques
The efficiency of a C++ program can also be optimized during execution. Consider these techniques:
- Loop Optimization: Eliminate unnecessary calculations within a loop.
- Memory Management: Use the right data structures and avoid memory leaks by deallocating unused memory.
Here’s a simplified example of optimized code:
#include <vector>
#include <iostream>
void process(std::vector<int>& nums) {
for(auto& num : nums) {
num *= 2; // Doubling the number
}
}
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
process(numbers);
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
This code effectively doubles each element of the vector, showcasing better memory use compared to older methods like manual indexing.
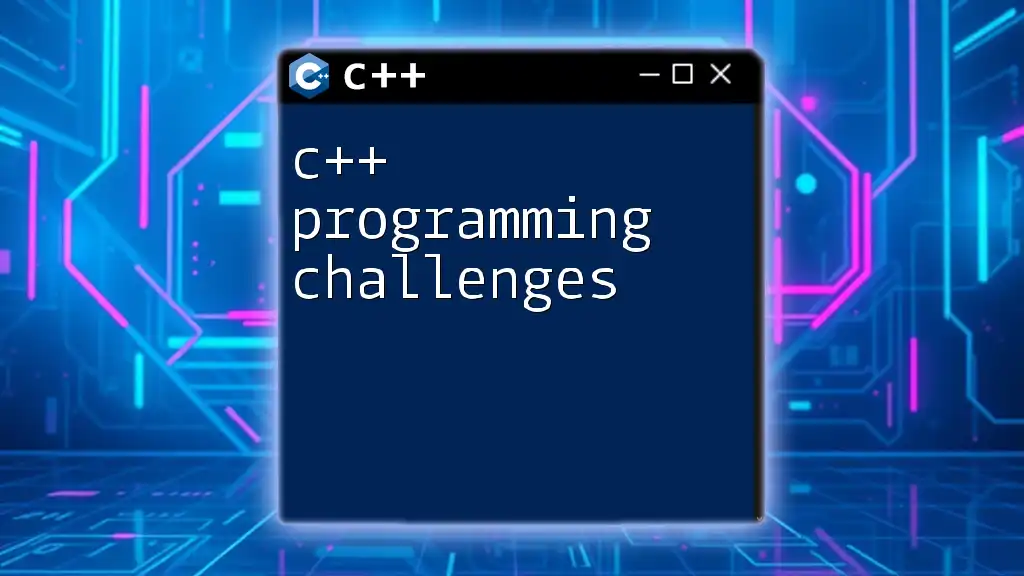
Case Studies
Simple C++ Program Execution
To provide context, let’s look at a simple program that accelerates the understanding of the execution flow. We can create a program that calculates the factorial of a number. The following snippet illustrates a straightforward implementation:
#include <iostream>
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
int main() {
int num = 5;
std::cout << "Factorial of " << num << " is " << factorial(num) << std::endl;
return 0;
}
When executed, this code computes and returns the factorial of `5`, illustrating flow control and recursion in action.
Real-World Example: Execution in a C++ Project
In a more complex C++ project like a game engine, program execution becomes multifaceted. Emphasis on real-time performance, rendering, and user input management is vital. The execution cycle involves game loop management, where the game constantly updates the game state and renders graphics in response to player actions. Understanding C++ program execution in such contexts helps programmers create responsive and efficient applications.
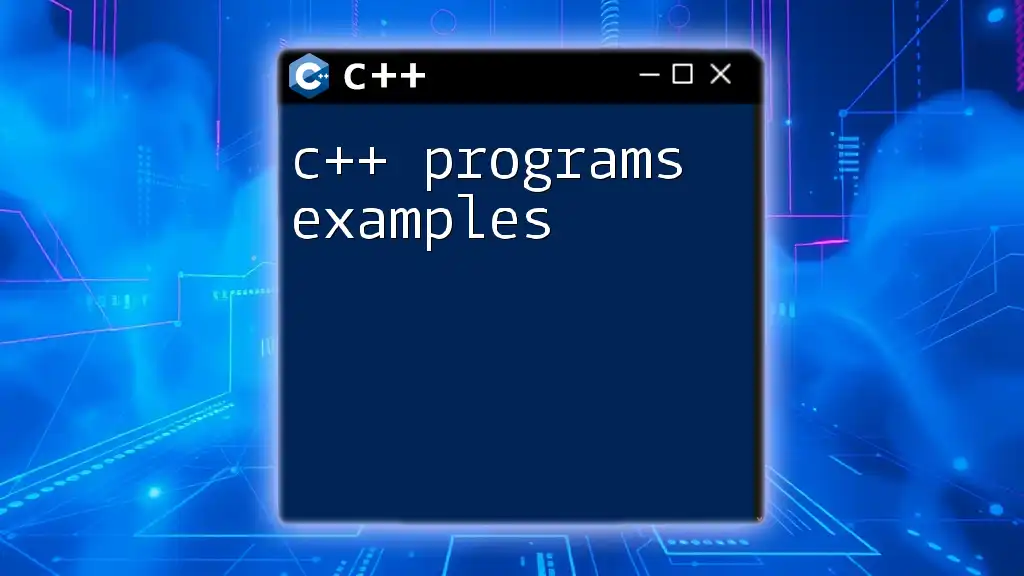
Conclusion
A thorough understanding of C++ program execution is essential for producing efficient and robust software. By mastering the compilation process, optimizing runtime performance, and employing effective debugging techniques, developers can enhance their coding skills significantly. Embrace the opportunity to implement these insights in your projects, ensuring that each execution contributes to your programming journey.
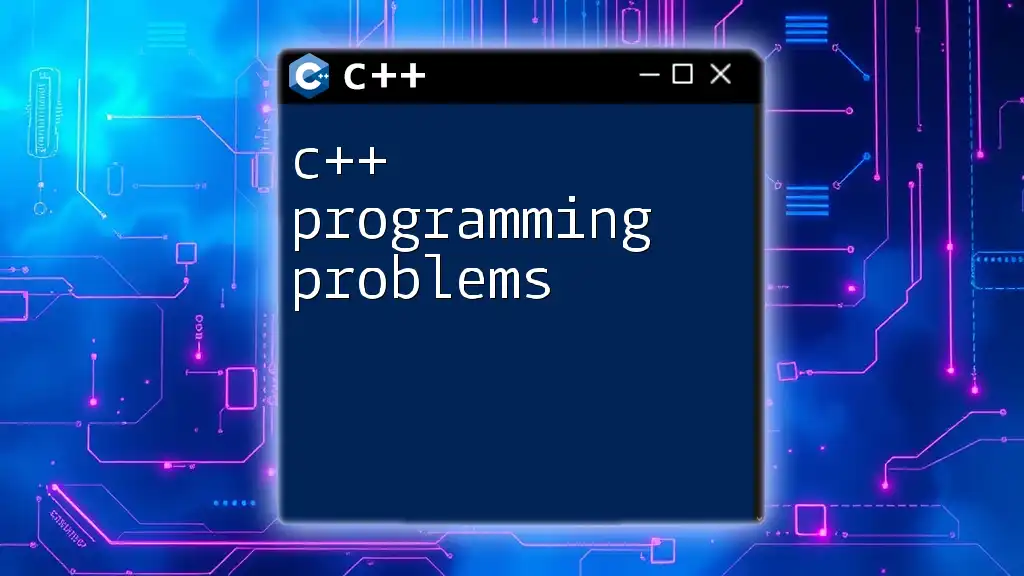
Additional Resources
To further your knowledge, consider exploring online C++ documentation, reputable programming books, and engaging courses. Participating in community forums and coding sites can also provide additional assistance and insights as you continue your journey with C++.
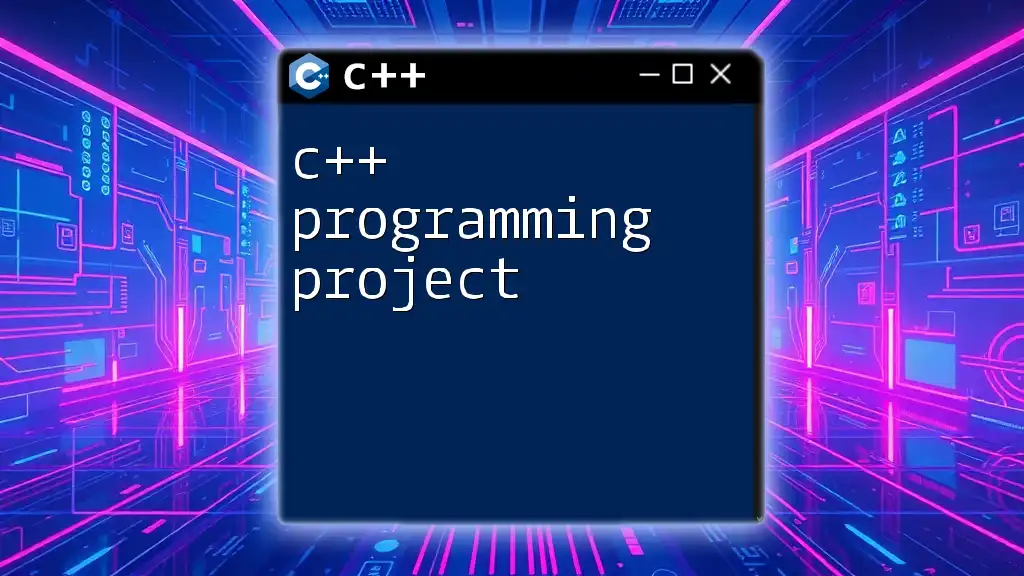
Call to Action
Now that you have a solid foundation on C++ program execution, put your skills to the test! Try implementing your own C++ projects, experiment with different features, and share your experiences or questions in the comments.