C++ program syntax consists of structured commands and statements that define the logic and flow of a program, enabling developers to perform various tasks effectively.
Here’s a simple example of C++ syntax that includes a basic program to print "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of C++ Syntax
What is Syntax?
In programming, syntax refers to the set of rules that define how code is written and structured. Just as natural languages have grammar rules, programming languages like C++ have specific syntax requirements that must be followed for the code to be understood by the compiler.
Structure of a C++ Program
A typical C++ program consists of several key components:
- Preprocessor Directives: These lines tell the compiler to include certain files or libraries before actual code compilation.
- Main Function: It serves as the entry point for any C++ program. Every C++ program must have a `main` function.
- Statements and Expressions: These carry out the actions and computations in your program.
- Return Statements: This tells the program what value to send back to the operating system when the program finishes executing.
Here's a simple example to illustrate these components:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this example, `#include <iostream>` is a preprocessor directive, `int main()` defines the main function, and `std::cout << "Hello, World!" << std::endl;` is a statement that outputs text to the console.
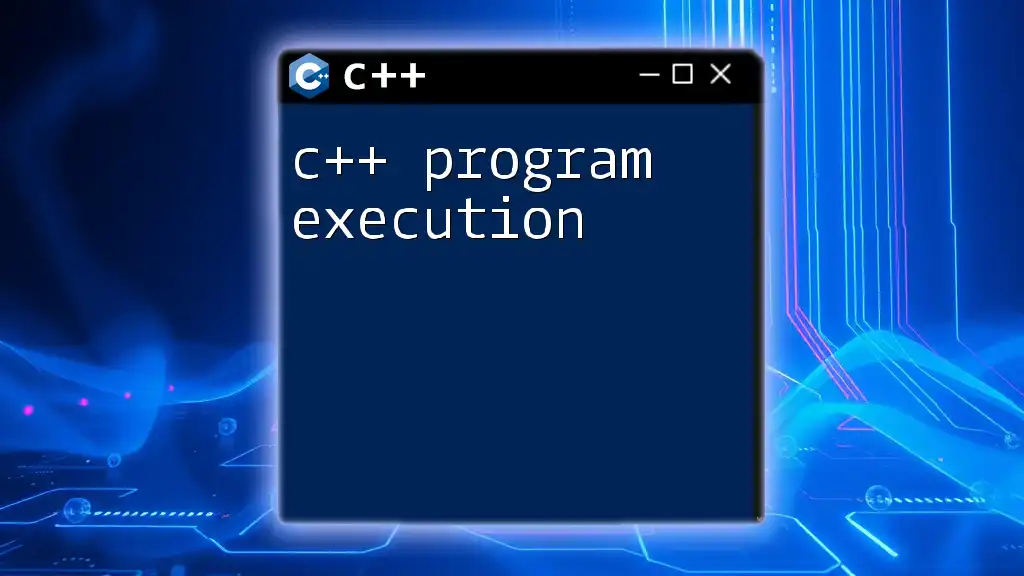
Key Components of C++ Syntax
Data Types
C++ has several basic data types which dictate what kind of data can be stored in a variable. Some of the primary data types include:
- Integers (`int`): Represents whole numbers.
- Floats (`float`): Represents decimal numbers.
- Characters (`char`): Represents single characters.
- Booleans (`bool`): Represents true/false values.
To declare basic variables, you might write:
int age = 25;
float salary = 50000.50;
char grade = 'A';
bool isEmployed = true;
Variables and Constants
Variables in C++ are used to store information that can change during program execution. Constants, however, are fixed values. You can declare constants using the `const` keyword:
const float PI = 3.14;
This value of `PI` will not change throughout the execution of the program, ensuring its integrity.
Operators
C++ provides a variety of operators that allow you to perform different operations on variables. These include:
- Arithmetic Operators: Used for mathematical calculations (+, -, *, /)
- Relational Operators: Used to compare two values (==, !=, >, <)
- Logical Operators: Used to combine conditional statements (&&, ||, !)
- Assignment Operators: Used to assign values to variables (=, +=, -=)
An example using these operators could look like this:
int a = 10;
int b = 20;
int sum = a + b; // Arithmetic
bool isEqual = (a == b); // Relational
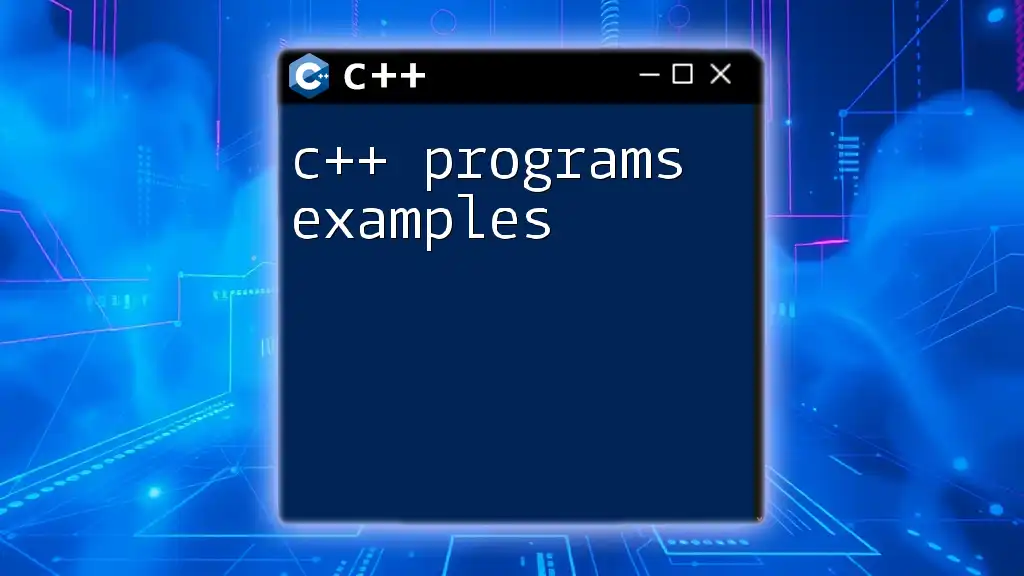
Control Flow Statements
Conditional Statements
Conditional statements allow the program to make decisions based on specific conditions. In C++, `if`, `else if`, and `else` statements are commonly used for this purpose.
Example of using if statements:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
In this example, the program checks if the `age` is greater than 18 and outputs either "Adult" or "Minor" based on the condition.
Loops
Loops in C++ are crucial for executing a block of code repeatedly until a specified condition is met. The most common loop types include `for`, `while`, and `do-while` loops.
Here’s an example of a for loop:
for(int i = 0; i < 5; i++) {
std::cout << "Iteration " << i << std::endl;
}
This loop will print the current iteration number from 0 to 4.
Switch Statements
The switch statement can be an elegant alternative to multiple if-else conditions when working with variables that can take on different values.
Example of a switch-case structure:
switch (grade) {
case 'A':
std::cout << "Excellent!" << std::endl;
break;
case 'B':
std::cout << "Good!" << std::endl;
break;
default:
std::cout << "Invalid grade." << std::endl;
}
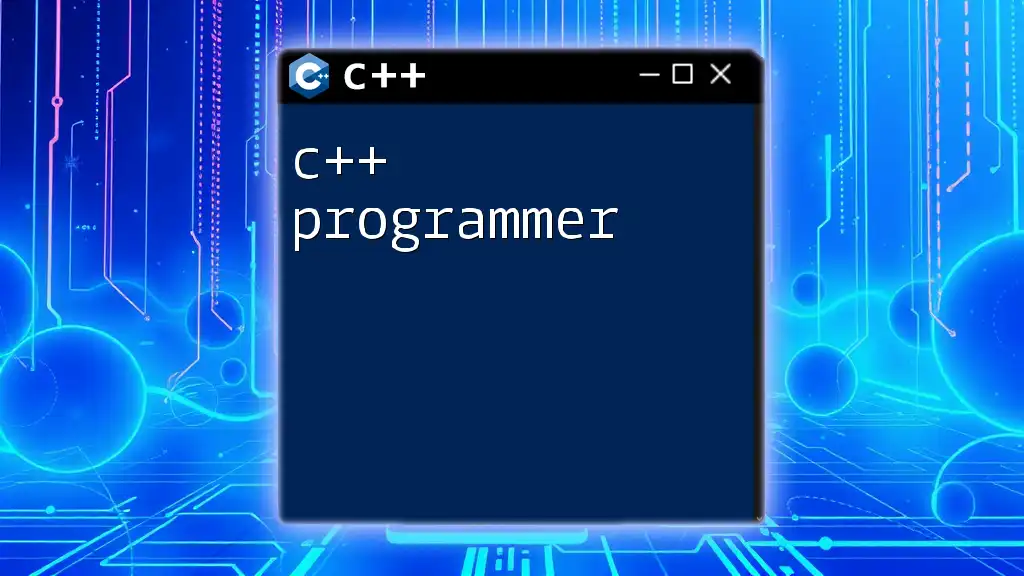
Functions and Scope
Defining Functions
In C++, functions allow you to encapsulate code for specific tasks. They can return values or be void (return nothing). Here's how to define and call a function:
void greet() {
std::cout << "Hello!" << std::endl;
}
int add(int x, int y) {
return x + y;
}
You would call the `greet` function simply by invoking `greet();`, and for the `add` function, you'd say `int result = add(5, 10);`.
Scope of Variables
The scope of a variable determines where in your program the variable can be accessed.
- Local Variables: Defined within a function and can only be used inside that function.
- Global Variables: Defined outside of all functions and can be accessed anywhere in the program.
Here's an example demonstrating variable scope:
int globalVar = 5;
void testScope() {
int localVar = 10;
std::cout << globalVar << " " << localVar << std::endl; // Outputs: 5 10
}
This code shows how `globalVar` can be accessed within the function `testScope`, while `localVar` cannot be accessed outside that function.
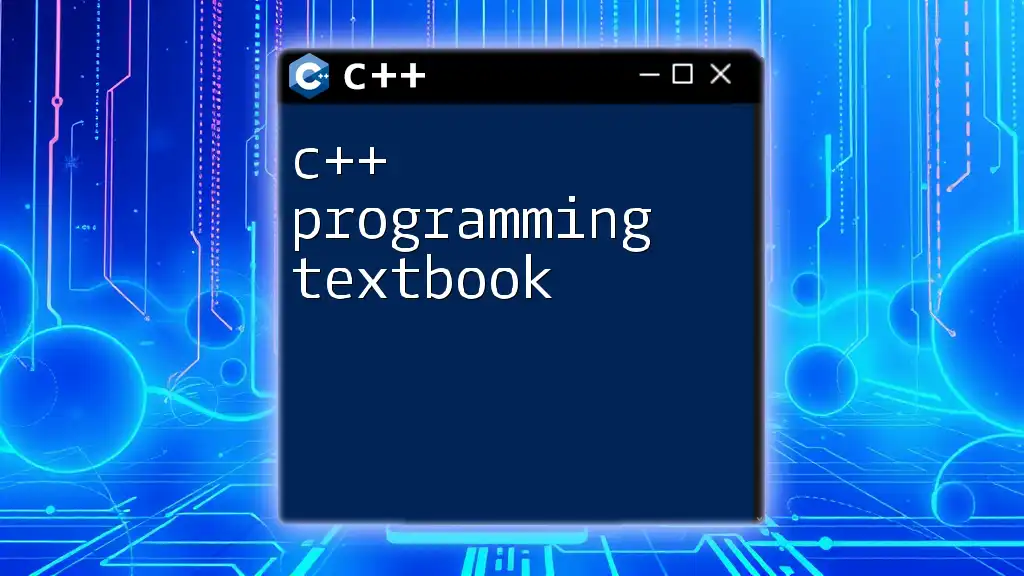
Object-Oriented Syntax
Classes and Objects
In C++, object-oriented programming is a fundamental feature that allows you to define classes and create objects. A class serves as a blueprint for creating objects with properties and methods.
Here’s how you might define a simple class:
class Car {
public:
void start() {
std::cout << "Car started." << std::endl;
}
};
Car myCar;
myCar.start();
This code defines a `Car` class with a method `start` and creates an object `myCar`, calling the `start` method.
Constructors and Destructors
Constructors and destructors are special member functions that are called when an object is created or destroyed, respectively.
Example of defining constructors:
class Book {
public:
Book() {
std::cout << "Book object created!" << std::endl;
}
~Book() {
std::cout << "Book object destroyed!" << std::endl;
}
};
When a `Book` object is instantiated, "Book object created!" will print. When it goes out of scope, "Book object destroyed!" shows up.
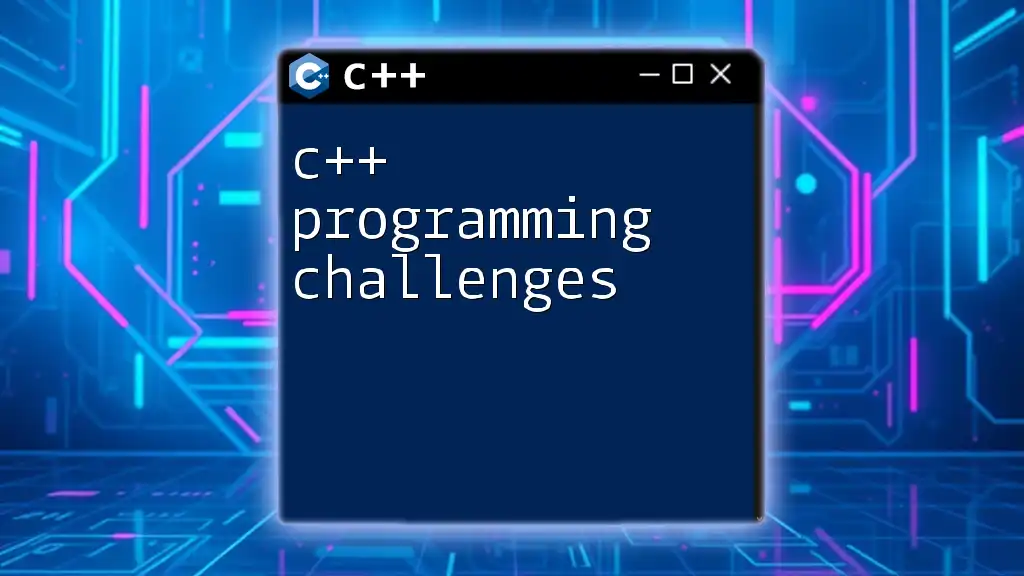
Best Practices for Writing C++ Code
Consistency and Readability
Maintaining consistency in your code enhances readability, making it easier for yourself and others to understand your work. Stick to naming conventions, use camelCase for variables, and PascalCase for classes.
Commenting
Comments are vital because they help anyone reading the code to understand what each section does without needing to interpret every line. Use comments judiciously and write them in a manner that explains the why behind complex logic.
Example of effective commenting:
// This function adds two numbers
int add(int a, int b) {
return a + b; // Return the sum
}
Error Handling
In C++, handling errors gracefully is crucial for building robust applications. Exception handling allows the program to deal with unexpected situations without crashing.
Here’s a simple example using try-catch:
try {
// Code that may throw an exception
} catch (std::exception& e) {
std::cout << "An error occurred: " << e.what() << std::endl;
}
This structure ensures that if an error occurs within the try block, the program can catch the exception and handle it appropriately in the catch block.
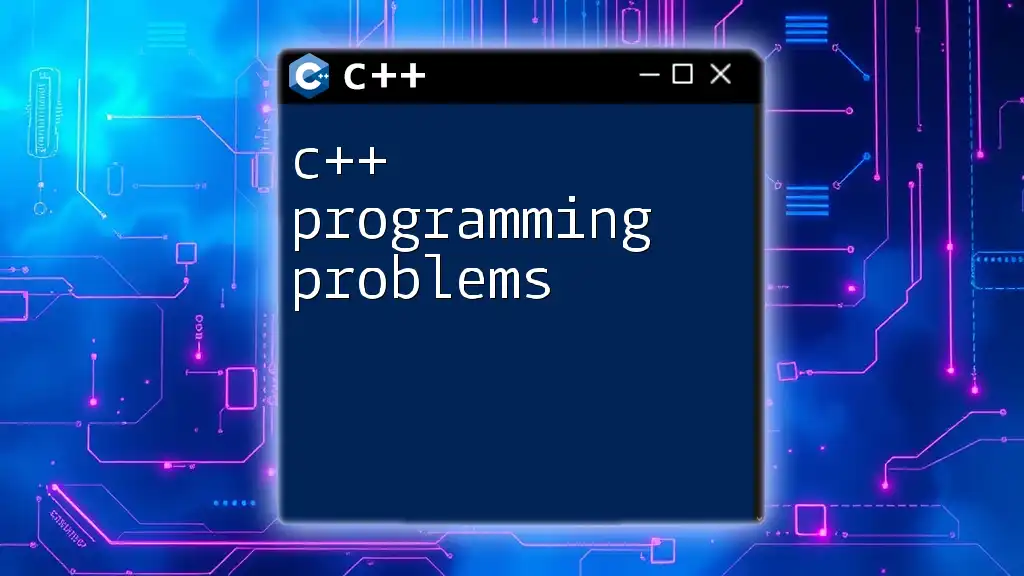
Conclusion
Mastering C++ program syntax is essential for effective programming. Grasping the foundational elements, control flows, and object-oriented features allows you to write efficient and maintainable code. By adhering to best practices in coding, such as clear commenting and error handling, you enhance your abilities as a developer. As you explore further, consistent practice and engagement with the C++ community will deepen your understanding and skills. With the knowledge gained from this guide, you are well-equipped to embark on your C++ programming journey!
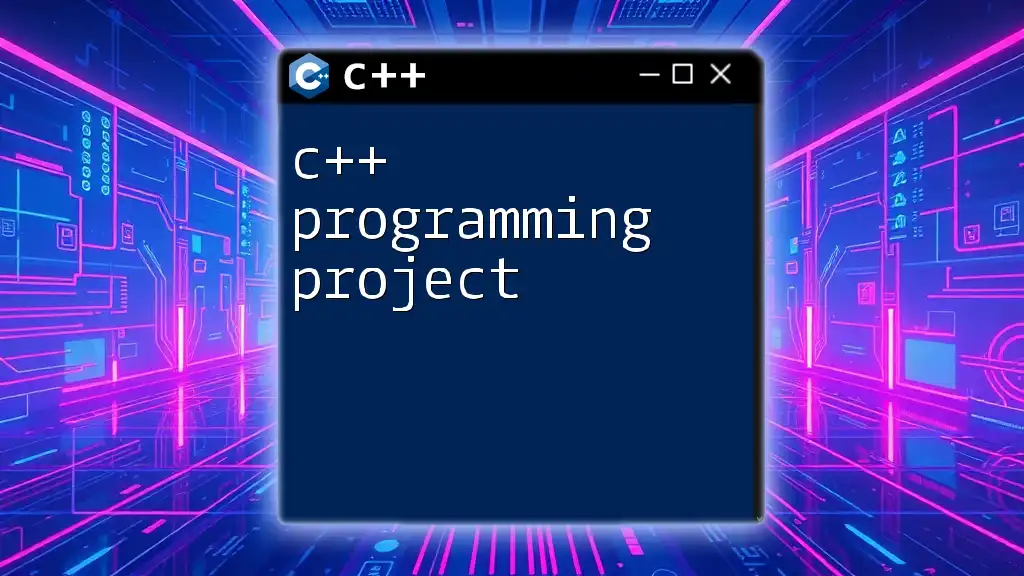
Additional Resources
For further learning, consider checking out books like "The C++ Programming Language" by Bjarne Stroustrup and online platforms like Coursera or Udacity that offer structured C++ courses. Engaging with communities on forums such as Stack Overflow or GitHub can also provide invaluable support and insights as you refine your skills.