C++ basic syntax consists of the fundamental elements and rules that govern how C++ programs are written, including variable declarations, control structures, and functions.
Here’s a simple example demonstrating C++ basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Syntax
What is Syntax?
In programming, syntax refers to the set of rules that defines the combinations of symbols that are considered to be correctly structured programs in a language. In simple terms, it determines how you should write your C++ code so that the compiler can understand it without errors. Mastering syntax is critical in ensuring that your programs run effectively.
Structure of a C++ Program
A typical C++ program is composed of various elements that define its structure:
- Headers: These are libraries included at the beginning of the program using the `#include` directive. Headers allow you to utilize pre-built functions and data types.
- Main Function: Every C++ program must have a `main` function. This serves as the entry point of any C++ application.
- Statements: Each line of code within the program is generally a statement and is executed sequentially.
Basic Program Structure
Starting with #include Directive
The `#include` directive is essential for including libraries that contain useful functions or objects. For instance, the `<iostream>` library is often used for input and output operations.
#include <iostream>
Defining the main function
The `main` function is the place where execution starts. Its typical definition returns an integer and looks like this:
int main() {
// Code here
return 0;
}
Here, `return 0;` signifies that the program has exited successfully.
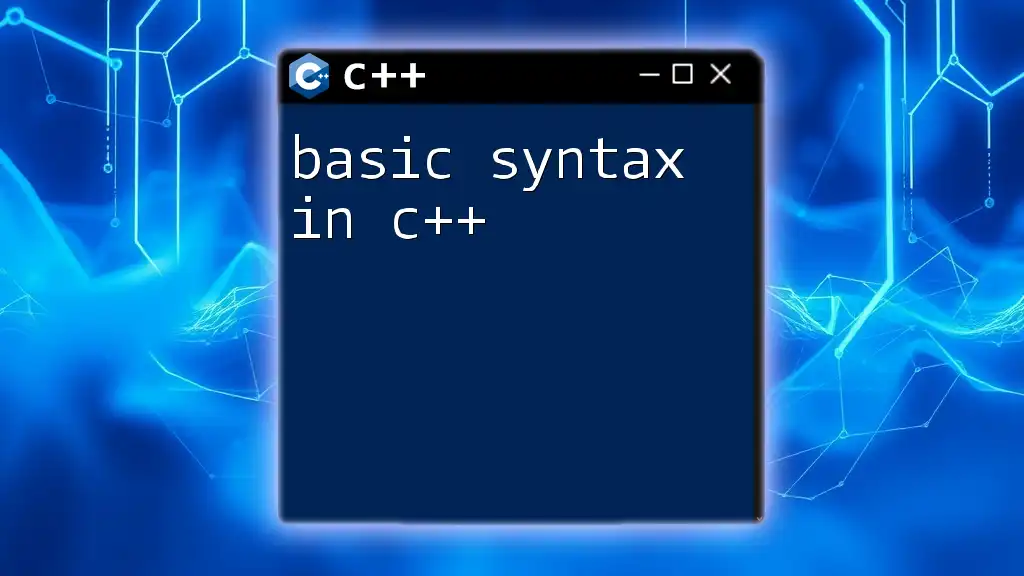
Key Components of C++ Syntax
Variables and Data Types
In C++, variables are used to store data values. Variables have types that define the kind of data they will hold. Common data types include:
- int: for integers
- float: for floating-point numbers
- char: for characters
- string: for sequences of characters
Example:
int age = 25;
float salary = 50000.50;
char grade = 'A';
Operators
Operators in C++ perform operations on variables and values. Common types of operators include:
- Arithmetic Operators: Used for mathematical operations. For example, `+`, `-`, `*`, and `/`.
- Relational Operators: Used to compare two values, such as `==`, `!=`, `>`, `<`, `>=`, and `<=`.
- Logical Operators: Used to combine two or more relational operations, like `&&` (AND), `||` (OR), and `!` (NOT).
Here’s an example of arithmetic operations:
int a = 5, b = 10;
int sum = a + b; // sum will be 15
Control Structures
Conditional Statements
Conditional statements execute different sections of code based on conditions. The basic structure involves the `if`, `else if`, and `else` statements.
Example:
if (age >= 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
This code checks if the variable `age` is 18 or above and outputs "Adult"; otherwise, it outputs "Minor".
Loops
Loops allow you to execute a block of code repeatedly under certain conditions. The three primary types of loops in C++ are:
- For Loop: Iterates a set number of times.
- While Loop: Continues to execute as long as a condition is true.
- Do-While Loop: Similar to the while loop but guarantees at least one execution.
For instance, consider this `for` loop to print numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i;
}
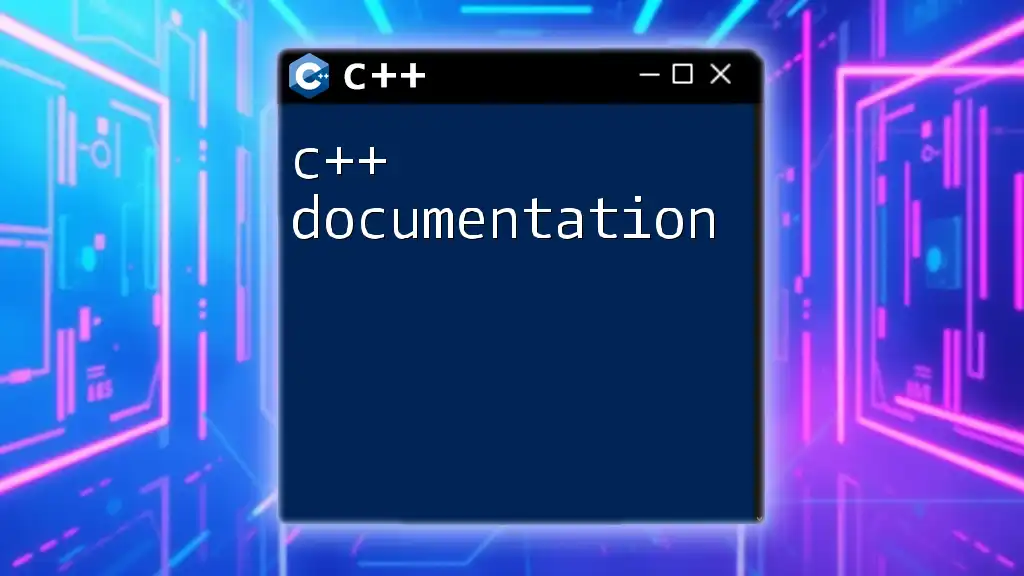
Functions
Defining Functions
Functions encapsulate code to be reused multiple times. They help in creating modular and manageable programs. Here’s how you can define a basic function:
void greet() {
std::cout << "Hello, World!";
}
Function Arguments and Return Types
You can pass data to functions through parameters and receive outputs via return values. The syntax for a function with arguments and a return type looks like this:
int add(int x, int y) {
return x + y;
}
In this example, the function `add` takes two integers as arguments and returns their sum.
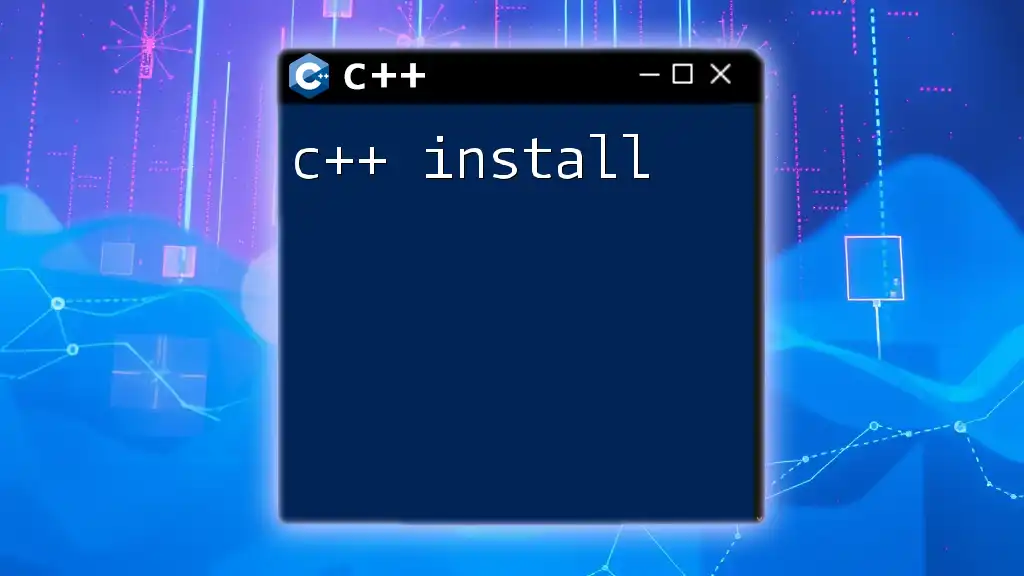
Comments in C++
Single-Line Comments
Comments are crucial for documenting your code and explaining complex sections. You can create single-line comments using `//`.
Example:
// This is a single-line comment
Multi-Line Comments
For comments that span multiple lines, use the `/* ... */` syntax.
Example:
/*
This is a multi-line comment
*/
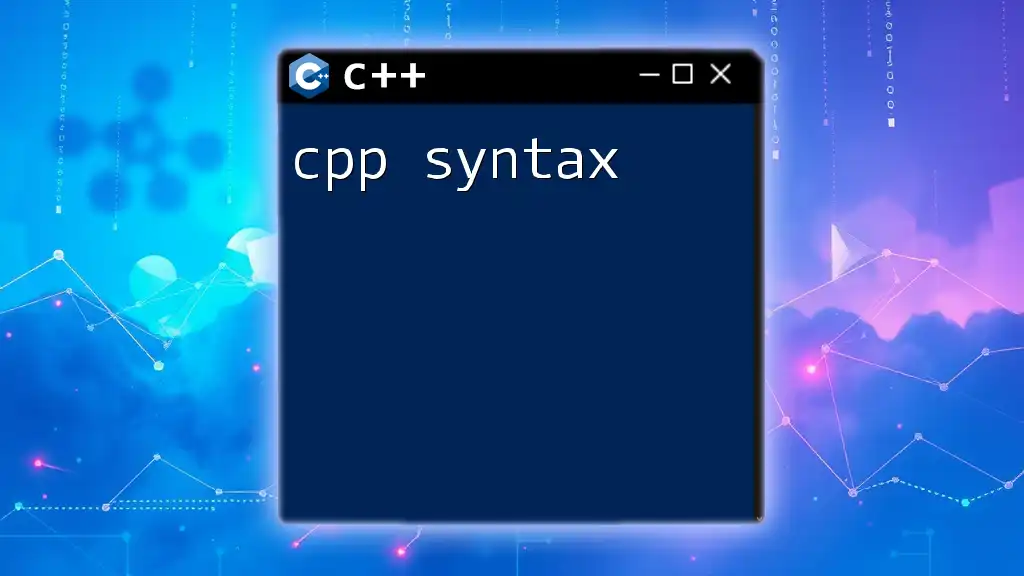
Conclusion
In summary, understanding C++ basic syntax is the foundation of programming in C++. It allows you to write clear and effective code, which ultimately leads to better programming practices. By grasping the structure, key components, control structures, functions, and comments, you’ll significantly enhance your coding skills.
As you continue your journey in learning C++, the next logical steps would involve delving into more advanced topics such as object-oriented programming, data structures, and algorithms to build robust and efficient applications.
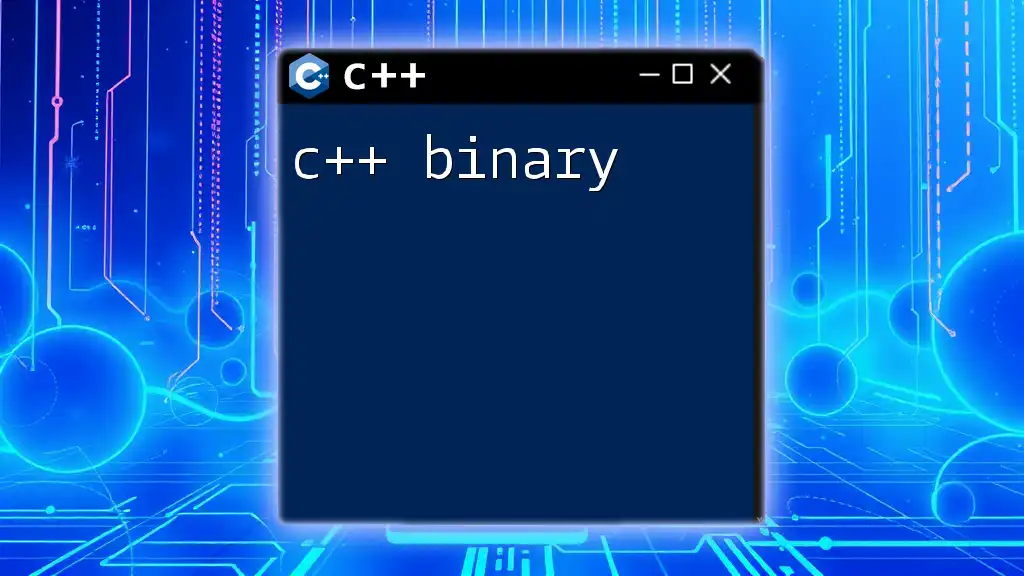
Additional Resources
To further your understanding of C++ and its syntax, consider exploring:
- Books on C++ programming - There are numerous well-regarded books that cover everything from basics to advanced topics.
- Online Courses and Tutorials - Many platforms offer comprehensive courses tailored to beginners.
- Helpful Programming Communities - Engaging with communities such as forums or Discord servers can provide support and additional learning opportunities.