Basic syntax in C++ involves the fundamental rules for structuring code, including headers, main functions, and statements for output, as demonstrated in the following example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Syntax
What is Syntax?
Syntax refers to the set of rules that defines the combinations of symbols and keywords that are considered to be correctly structured in a programming language. Understanding syntax is crucial for any programmer, as even a minor error can lead to bugs or prevent the program from compiling. C++ has its unique syntax that differs from languages like Python or Java.
Basic Structure of a C++ Program
The basic structure of a C++ program consists of several key components:
-
Include Directives: These lines at the beginning of the program tell the compiler to include certain libraries or dependencies. They generally enable the compiler to recognize standard functions.
-
Main Function: Every C++ program must contain a `main` function. This is where the execution starts. The structure is as follows:
int main() { // Code goes here return 0; }
-
Return Statement: The `return` statement exits the program and can also send a value back to the operating system. A return value of `0` usually indicates successful execution.
Hello World Example
One of the simplest programs you can write in C++ is the classic Hello World program. Here's how it looks:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
This simple program uses the `#include` directive to include the iostream library, which facilitates input and output operations. `std::cout` is used to print the message "Hello, World!" to the console, and the `std::endl` line adds a line break.
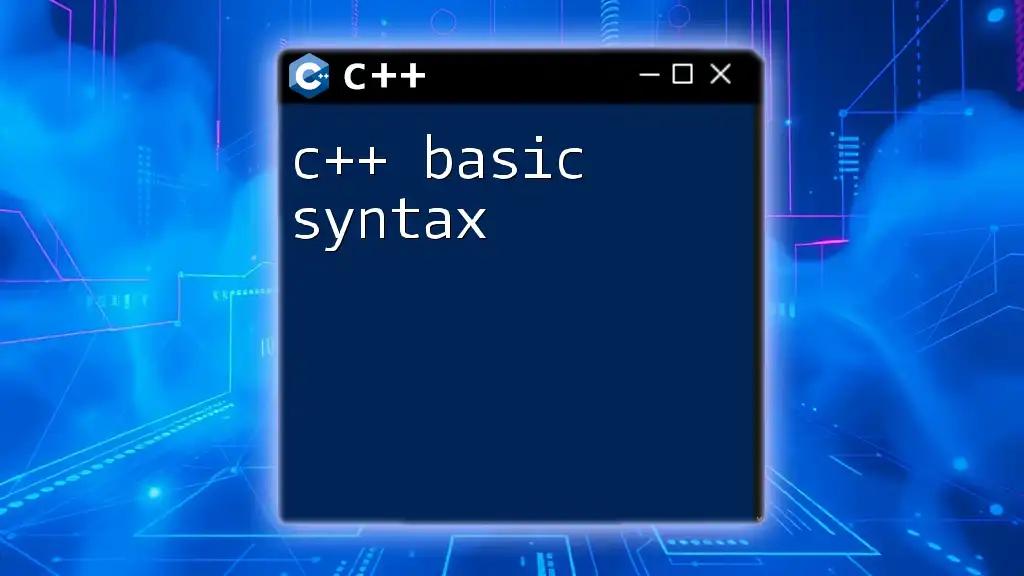
Variables and Data Types
What are Variables?
In programming, a variable is a named storage location in memory that can hold a value. Variables allow you to store data that can be used later in your program.
Data Types in C++
C++ offers various data types which define the type of data a variable can hold. Here are the fundamental data types:
- int: Used for integer numbers.
- float: Used for floating-point (decimal) numbers.
- double: Provides double precision for decimal numbers.
- char: Represents a single character.
- bool: Holds a value of either true or false.
Declaring and Initializing Variables
To declare a variable, you must specify its type followed by its name. Here’s how it looks:
int age = 25;
float salary = 50000.50;
char grade = 'A';
It's important to follow naming conventions for your variables. For example, variable names should start with a letter and can include letters, numbers, and underscores.

Operators in C++
Arithmetic Operators
C++ supports several arithmetic operators for performing mathematical calculations. These include:
- `+` (addition)
- `-` (subtraction)
- `*` (multiplication)
- `/` (division)
- `%` (modulus)
Here’s a quick example using these operators:
int a = 10;
int b = 20;
int sum = a + b; // sum is 30
Relational Operators
Relational operators allow you to compare two values and return a boolean result. The common relational operators include:
- `==` (equal to)
- `!=` (not equal to)
- `>` (greater than)
- `<` (less than)
- `>=` (greater than or equal to)
- `<=` (less than or equal to)
You can use them in conditions, like so:
if (a < b) {
std::cout << "a is less than b" << std::endl;
}
Logical Operators
Logical operators combine multiple conditions. These include:
- `&&` (logical AND)
- `||` (logical OR)
- `!` (logical NOT)
They are particularly useful in conditional statements where you want multiple criteria:
if (age >= 18 && grade == 'A') {
std::cout << "You are an adult with an excellent grade." << std::endl;
}
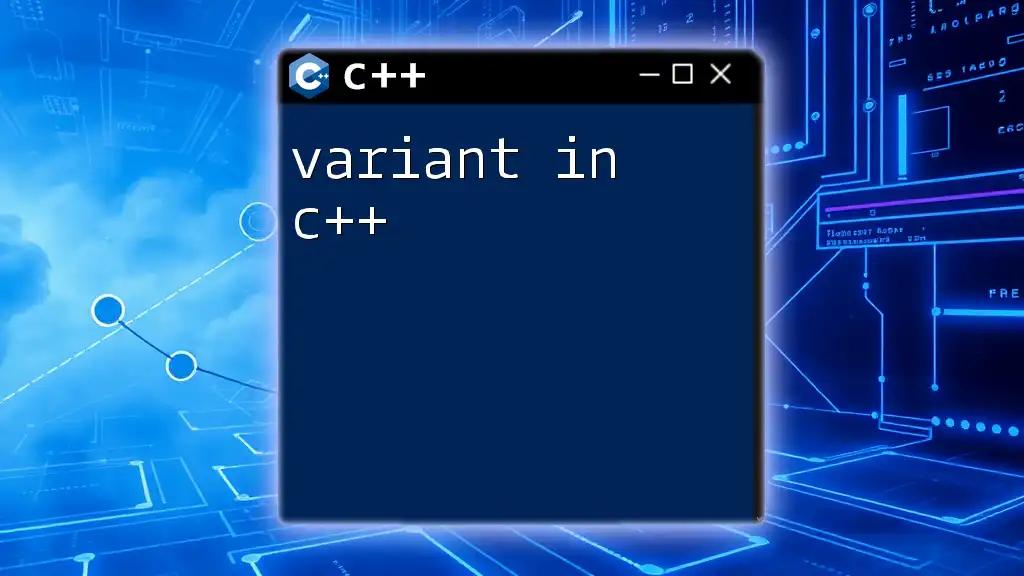
Control Structures
Conditional Statements
Conditional statements direct the flow of your program based on certain conditions. The most basic form is the `if` statement:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
This checks if the age is 18 or more and prints the corresponding message.
Switch Statement
A `switch` statement provides a clean way to dispatch to different code based on the value of a given expression. It’s often more readable than multiple `if-else` statements:
switch (grade) {
case 'A':
std::cout << "Excellent!" << std::endl;
break;
case 'B':
std::cout << "Good!" << std::endl;
break;
default:
std::cout << "Invalid grade!" << std::endl;
}
Loops
C++ supports several types of loops for repeating operations. The most common ones are:
- For Loop: Executes a block of code a specific number of times.
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
- While Loop: Continues executing as long as a condition remains true.
int count = 0;
while (count < 5) {
std::cout << count << std::endl;
count++;
}
- Do-While Loop: Similar to the while loop, but guarantees at least one execution.
int num = 0;
do {
std::cout << num << std::endl;
num++;
} while (num < 5);

Functions
Defining a Function
Functions allow you to encapsulate code for reuse. Here’s how you define a simple function:
int sum(int a, int b) {
return a + b;
}
This function takes two integers, adds them together, and returns the result. Functions improve readability and reduce code duplication.
Function Overloading
C++ supports function overloading, which allows you to define multiple functions with the same name but different parameter types or counts. For example:
int sum(int a, int b) {
return a + b;
}
double sum(double a, double b) {
return a + b;
}

Input and Output in C++
Using `cin` and `cout`
C++ uses `cin` to read input from the user and `cout` for displaying output. The standard input/output library must be included for these to function. Here’s a simple example:
int number;
std::cout << "Enter a number: ";
std::cin >> number;
std::cout << "You entered: " << number << std::endl;
Format Output
C++ offers format manipulators to control the output format. For instance, you can set the width of output or the number of decimal places for floating-point numbers using `std::setw` and `std::setprecision`.
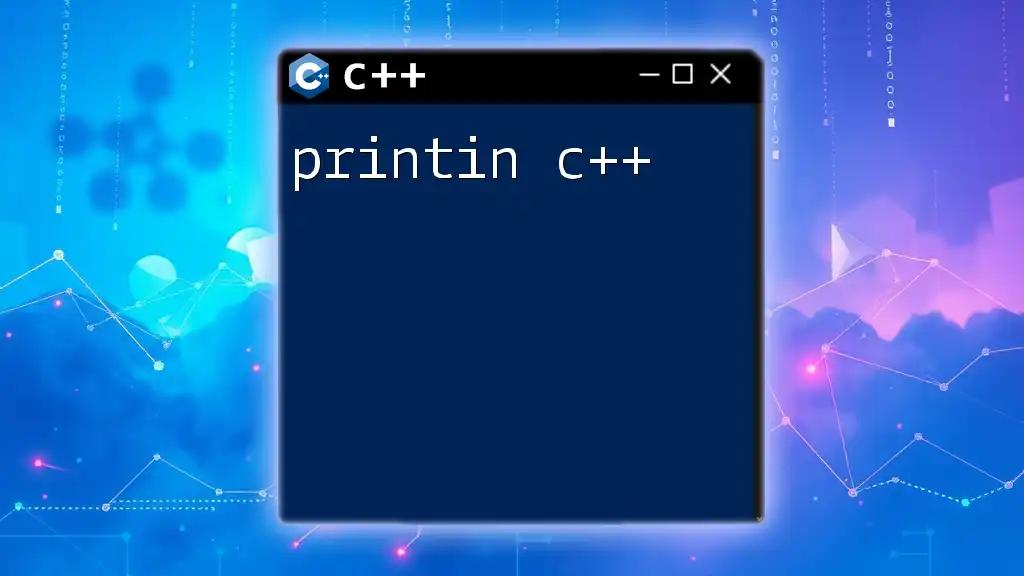
Error Handling in C++
Syntax Errors vs Runtime Errors
Syntax errors occur during compilation and prevent your code from running. Examples include missing semicolons or mismatched parentheses. In contrast, runtime errors occur while the code executes, such as dividing by zero.
Using `try`, `catch`, and `throw`
C++ also has a robust error handling mechanism using `try`, `catch`, and `throw`, making it easier to manage errors gracefully. Here’s a simple example:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << e.what() << std::endl;
}
This code attempts to throw a runtime error and catches it, allowing for a clean response to the error scenario.
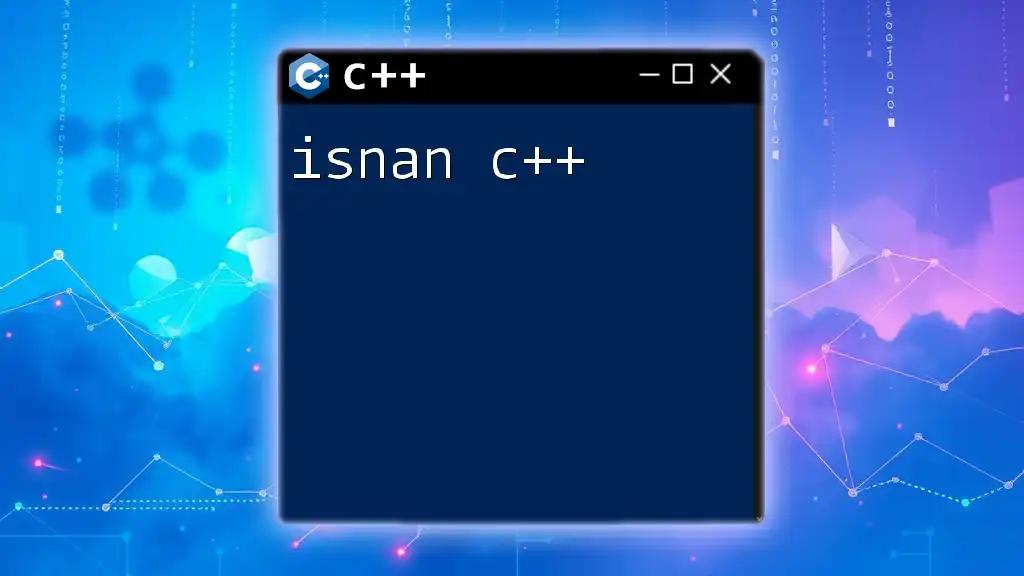
Conclusion
Understanding basic syntax in C++ is fundamental for composing well-structured programs. From the building blocks of a C++ program to more complex concepts like functions and error handling, mastering these elements will enhance your programming proficiency. To solidify your knowledge, practice writing more code and build on these basic concepts. The world of C++ is rich and full of opportunities for growth.