Pointer syntax in C++ allows you to declare pointers that hold memory addresses of variables, enabling direct manipulation of variable content through dereferencing. Here's a simple example:
int main() {
int var = 42; // Declare an integer variable
int* ptr = &var; // Declare a pointer and initialize it with the address of 'var'
*ptr = 100; // Dereference the pointer to change 'var's value
return 0;
}
Understanding Pointers in C++
Pointers are an essential part of C++ that are often misunderstood but are crucial for efficient memory management and manipulation. A pointer is essentially a variable that holds the memory address of another variable. This allows developers to directly access and modify the contents of memory locations, which can lead to more efficient code and better performance.
Why Pointers Matter
Using pointers can significantly enhance performance due to their direct access capabilities. They allow for dynamic memory allocation and deallocation, enabling the creation of complex data structures like linked lists, trees, and graphs. Pointers also play a vital role in resource management, especially in applications where memory usage is critical.
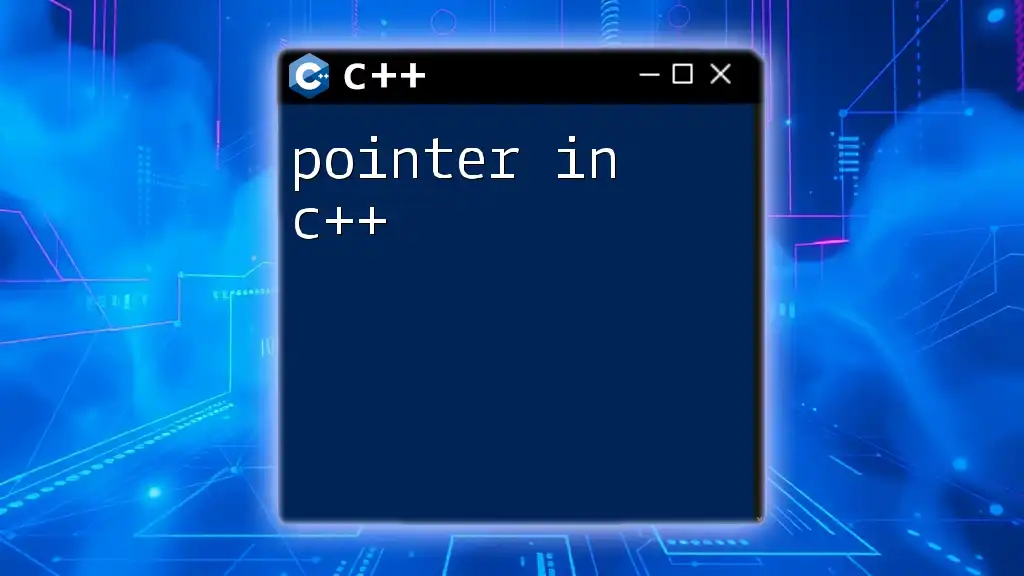
Basics of Pointer Syntax
What is Pointer Syntax?
The pointer syntax in C++ defines how pointers are declared, initialized, and dereferenced. A pointer is declared using the asterisk symbol (`*`), which indicates that the variable being declared is a pointer type.
Declaring Pointers
The syntax for declaring pointers is straightforward. You need to specify the type of data the pointer will point to, followed by the asterisk. For example:
int* p1; // Pointer to an integer
char* p2; // Pointer to a character
In these declarations, `p1` is defined as a pointer to an integer, while `p2` is a pointer to a character. Notice that the asterisk follows the type name, indicating that we're working with a pointer, not a normal variable.
Initializing Pointers
After declaration, pointers must be initialized to point to a valid memory address. This is accomplished using the address-of operator (`&`), which returns the address of a variable. For example:
int num = 5;
int* ptr = # // ptr now holds the address of num
In this case, `ptr` is initialized to hold the address of the variable `num`. It’s important to ensure that pointers are always initialized before they are used to avoid undefined behavior.
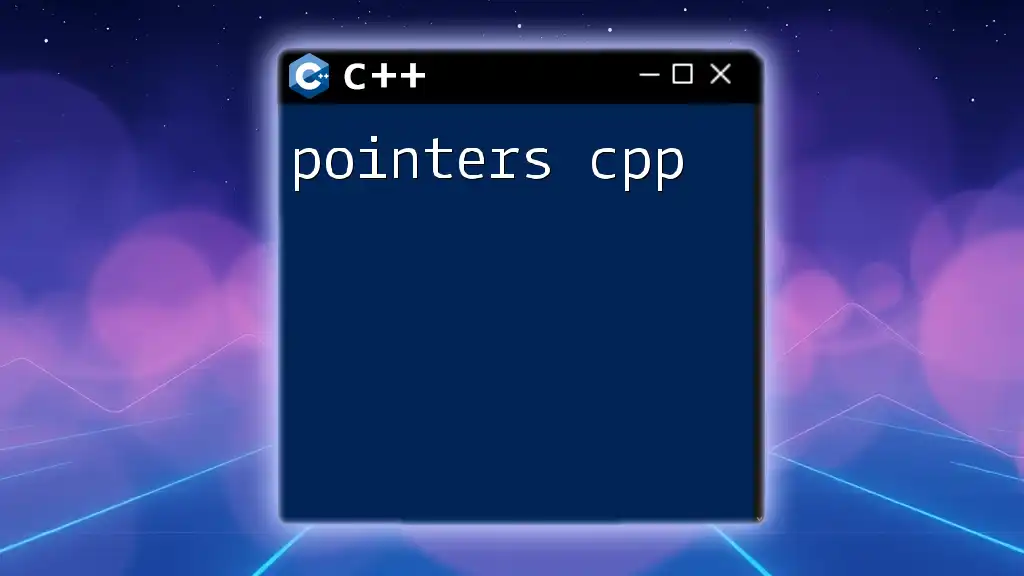
Dereferencing Pointers
Understanding Dereferencing
Dereferencing is the process of accessing the value at the memory address held by a pointer, using the asterisk operator once more. When you dereference a pointer, you gain access to the data it points to.
How to Dereference Pointers
To dereference a pointer, simply use the asterisk before the pointer variable. Here's an example:
int value = 20;
int* ptr = &value;
cout << *ptr; // Outputs: 20
In this example, the statement `*ptr` retrieves the value stored at the memory address contained in `ptr`, which is `20`.
Using Dereferencing in Assignments
Dereferencing also allows you to change the value of the variable that the pointer points to. Here's how it works:
*ptr = 30; // The value of value is now 30
Now, the variable `value` holds the number `30`. This highlights the power of pointers in managing and accessing memory.
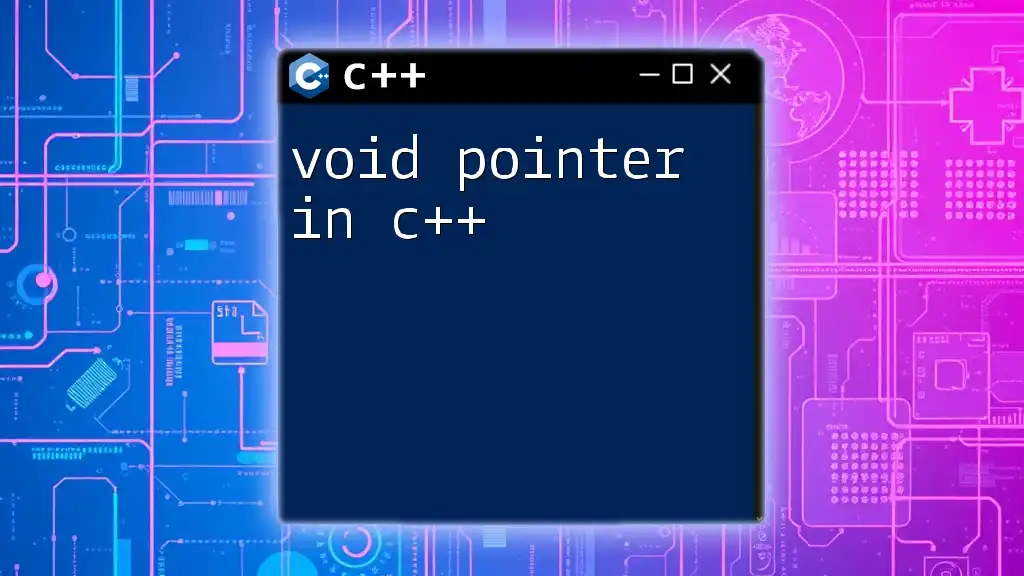
Pointer Arithmetic
Overview of Pointer Arithmetic
Pointer arithmetic is a powerful feature of C++ that allows you to perform arithmetic operations on pointers. This is particularly useful when dealing with arrays.
Incrementing and Decrementing Pointers
You can increment or decrement pointers, which will move them forward or backward in memory based on the type of data they point to. For example:
int arr[] = {1, 2, 3};
int* p = arr;
cout << *(p + 1); // Outputs: 2
In this example, `p` points to the first element of `arr`. By adding `1` to `p`, you move the pointer to the next element in the array, and dereferencing it yields `2`.
Limitations and Considerations
While pointer arithmetic is useful, it comes with restrictions. Pointer operations should only be performed on pointers of the same type, and care must be taken to avoid accessing memory outside of valid bounds to prevent undefined behavior.
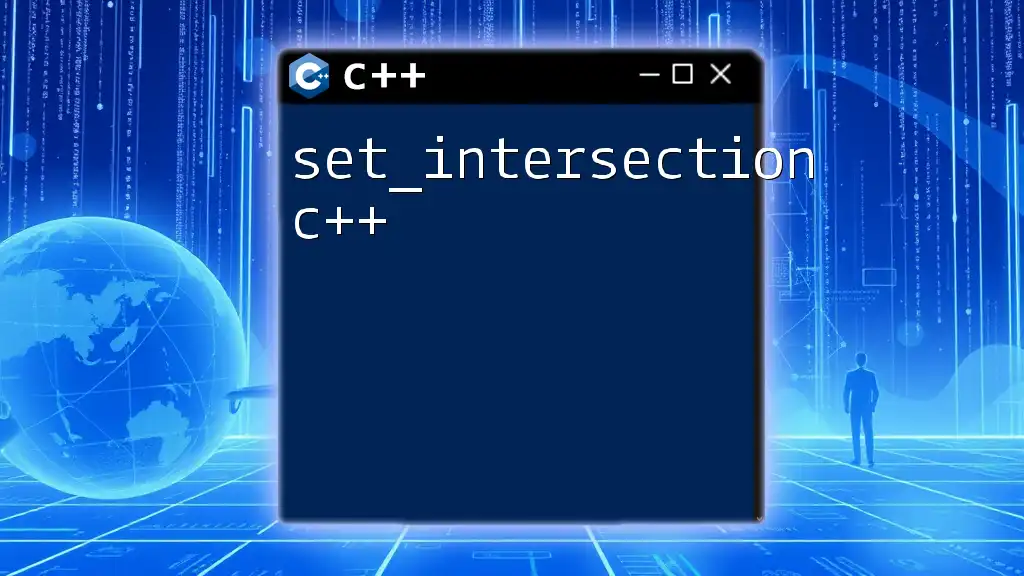
Pointers to Pointers
What are Pointers to Pointers?
A pointer to a pointer, or double pointer, is essentially a pointer that holds the address of another pointer. This can be useful in various scenarios, such as when working with dynamic data structures.
Declaration and Initialization
To declare a pointer to a pointer, you simply add another asterisk to the pointer type. For instance:
int num = 10;
int* ptr = #
int** ptr_to_ptr = &ptr; // Pointer to pointer
Here, `ptr_to_ptr` is a pointer that holds the address of `ptr`. This allows indirect access to the original variable.
Accessing Values via Pointers to Pointers
Dereferencing a double pointer is similar to dereferencing a single pointer but requires an additional dereference operator. For example:
cout << **ptr_to_ptr; // Outputs: 10
This retrieves the value held by `num`, demonstrating how to navigate through multiple layers of pointers.
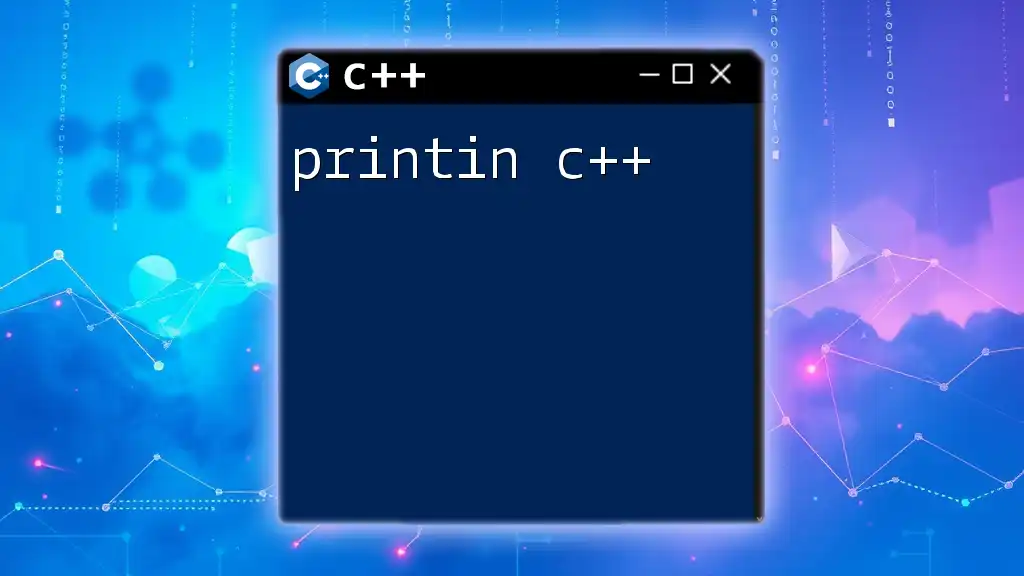
Common Pitfalls and Best Practices
Avoiding Dangling Pointers
Dangling pointers occur when a pointer retains the reference to a memory location that has already been freed or removed. This can lead to undefined behavior. To avoid dangling pointers, always ensure that pointers are set to `nullptr` after they have been deleted:
int* ptr = new int(5);
delete ptr;
ptr = nullptr; // Safeguard against dangling pointer
Memory Leaks and Efficient Memory Management
Memory leaks happen when dynamically allocated memory is not properly deallocated. Always ensure that allocated memory is released. The use of `delete` for single objects and `delete[]` for arrays is crucial for efficient memory management:
int* dynamicArray = new int[5];
// ... use array ...
delete[] dynamicArray; // Avoids memory leak
Implementing these best practices will help ensure that your applications run efficiently and remain free from memory-related issues.
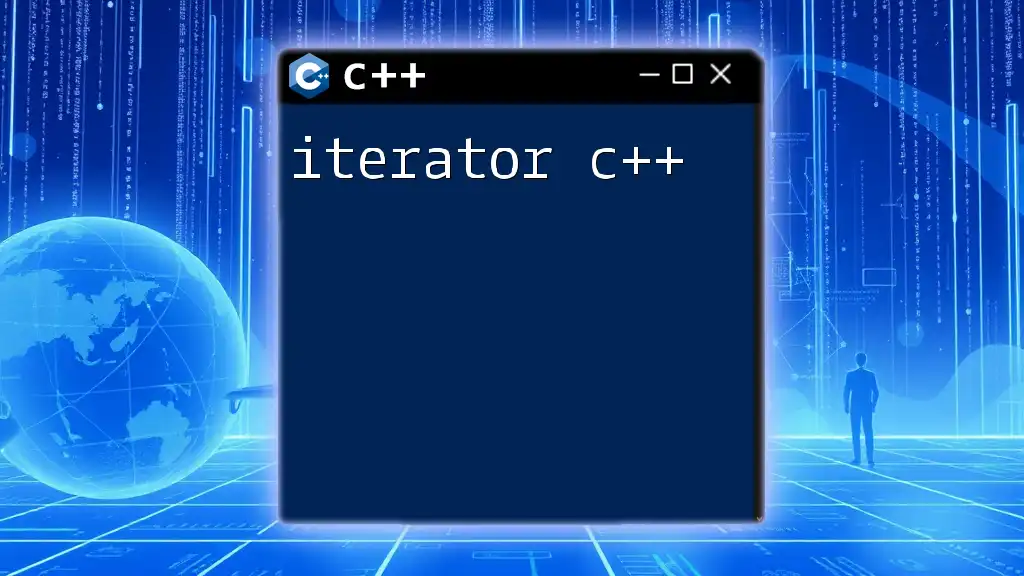
Conclusion
In summary, understanding pointer syntax in C++ is foundational for programming in this language. Pointers offer powerful capabilities for directly manipulating memory, improving program efficiency, and managing data. By mastering the concepts of pointer declaration, initialization, dereferencing, pointer arithmetic, and even pointers to pointers, you will be well-equipped to leverage these techniques in your own projects. As always, continuous practice and learning are essential for deepening your understanding of pointers and their applications in C++.
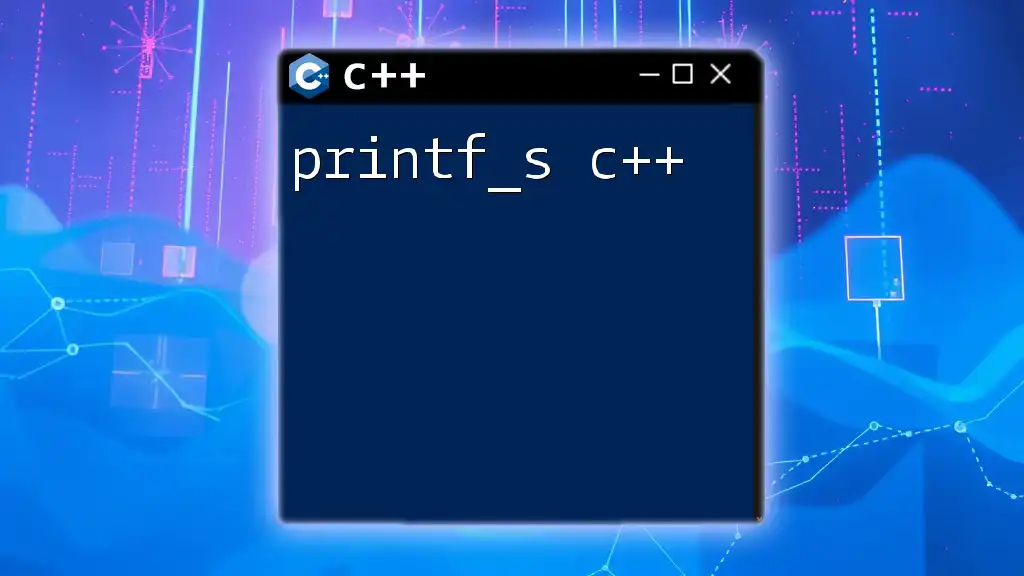
Call to Action
Join our community for more insights into C++ programming, and don't hesitate to leave your thoughts or questions in the comments. Your engagement helps foster a dynamic learning environment!