In C++, a class pointer is a variable that stores the memory address of an object created from a class, allowing for dynamic memory management and access to class members through the pointer.
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
MyClass* objPtr = new MyClass(); // Creating a class pointer
objPtr->display(); // Accessing member function through the pointer
delete objPtr; // Deleting the allocated memory
return 0;
}
Introduction to C++ Class Pointers
A class pointer in C++ is a powerful concept that allows developers to work with dynamically allocated class objects. Understanding how to effectively use class pointers is essential for mastering memory management and enabling advanced functionality in C++ applications.
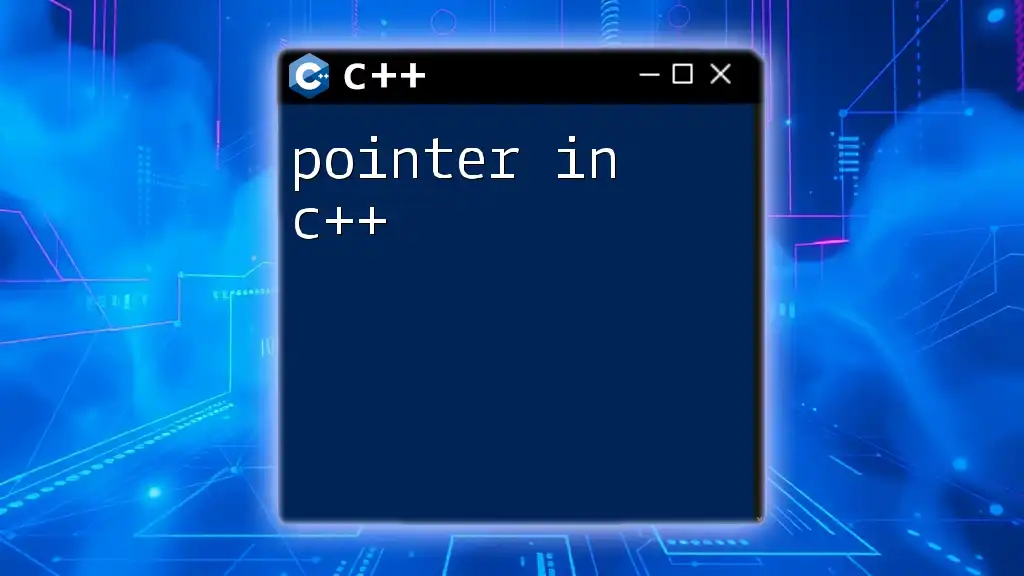
Understanding Pointers in C++
What are Pointers?
Pointers are variables that hold the memory address of another variable. They serve as tools for manipulating memory and accessing values more efficiently. The key distinction between pointers and references is that pointers can be reassigned, whereas references are fixed once assigned.
Declaring and Initializing Class Pointers
To declare a pointer to a class, use the following syntax:
MyClass *myPtr;
This statement defines `myPtr` as a pointer to an object of the `MyClass`. To initialize it, you can dynamically allocate memory for the class instance using the `new` operator like so:
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
MyClass *myPtr = new MyClass();
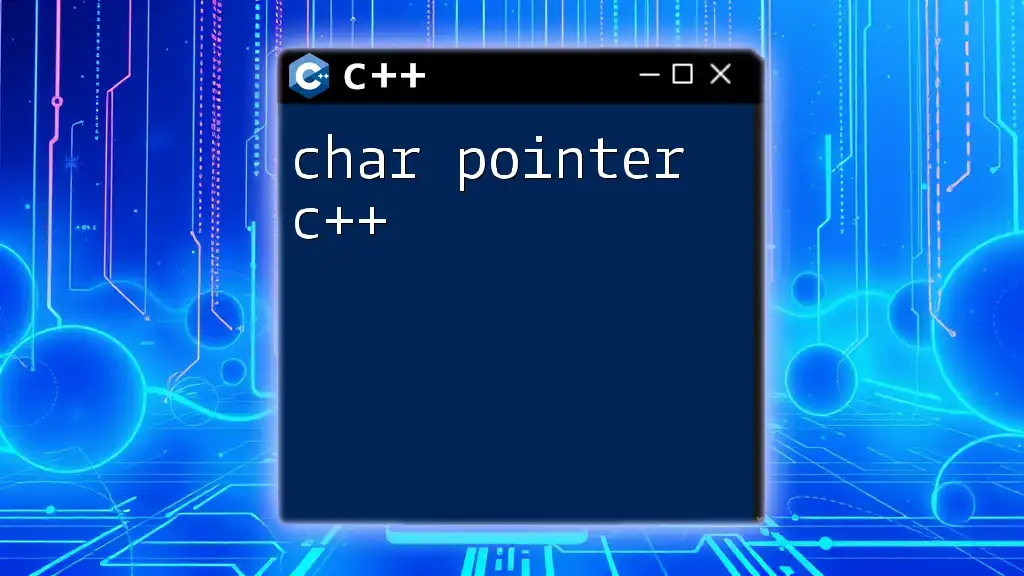
Creating and Using Class Pointers
Dynamically Allocating Objects
Dynamic memory allocation is crucial in C++. When you allocate memory using `new`, it allows for the creation of class instances at runtime, making your application more flexible. For instance:
MyClass *objPtr = new MyClass();
objPtr->display();
In this code, we allocate memory for `MyClass` and call the `display` method through the pointer.
Accessing Class Members Using Pointers
To access members of a class using a pointer, you use the arrow operator (`->`). This operator provides a shorthand to access members without dereferencing the pointer explicitly. Consider the following example:
class Rectangle {
public:
int width, height;
int area() { return width * height; }
};
Rectangle *rectPtr = new Rectangle();
rectPtr->width = 5;
rectPtr->height = 10;
std::cout << "Area: " << rectPtr->area() << std::endl;
In this snippet, we define a `Rectangle` class and manipulate its properties using a class pointer.
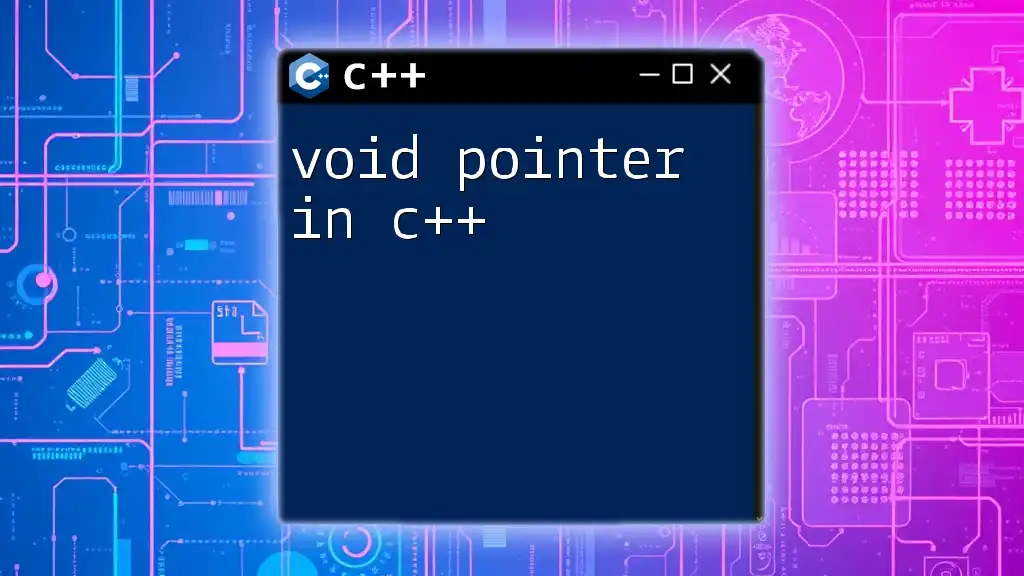
Class Pointer vs. Object Instance
Differences Between Class Pointer and Object Instance
When you instantiate a class object directly, memory for the object is allocated on the stack. Conversely, when you create a class pointer and allocate it with `new`, memory goes on the heap. This distinction determines object lifetime and lifetime management.
A simple example demonstrating both methods looks like this:
MyClass obj; // Stack allocation
MyClass *ptr = new MyClass(); // Heap allocation
When to Use Class Pointers
Class pointers come in handy for various scenarios, particularly when dealing with polymorphism or when you need to allocate arrays of objects. For example, you may want to process different shapes using a common interface:
void processShape(Shape *shape) {
shape->draw();
}
This function can accept any derived class of `Shape` passed through a pointer, showcasing the versatility of class pointers.
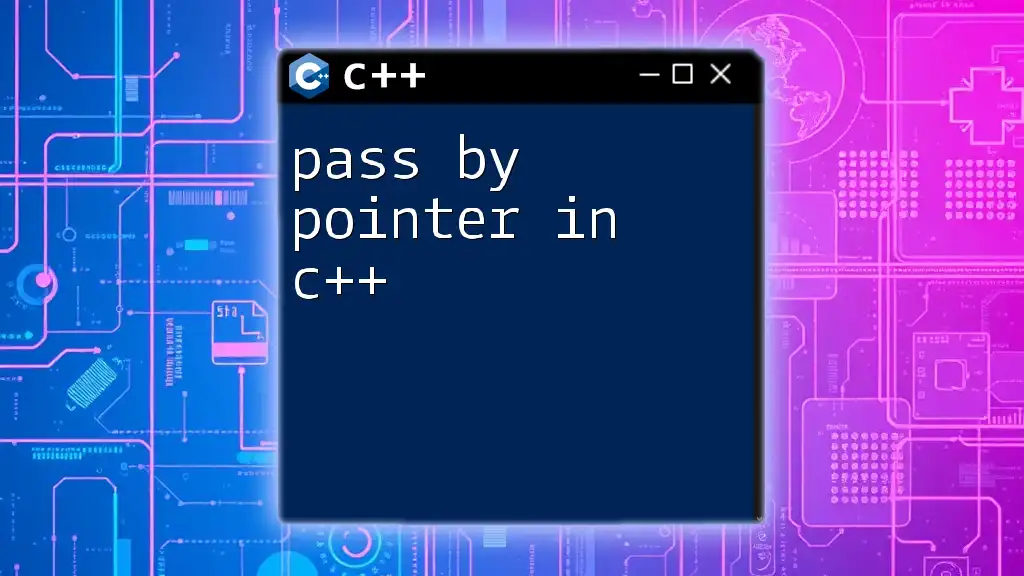
Memory Management with Class Pointers
Importance of Memory Deallocation
When using class pointers, memory management becomes crucial to prevent memory leaks, which occur when dynamic memory is not properly released. Anytime you allocate memory using `new`, you must free it with `delete`:
delete objPtr; // Deallocating memory
For arrays of objects, don’t forget to use `delete[]` to avoid undefined behavior.
Best Practices for Memory Management
Managing memory effectively is paramount. Here are some guidelines:
- Always pair `new` with `delete` to prevent leaks.
- Consider using smart pointers, a feature of modern C++, which handle memory automatically.
For example, using `std::unique_ptr` can greatly simplify memory management:
#include <memory>
std::unique_ptr<MyClass> ptr = std::make_unique<MyClass>();
ptr->display();
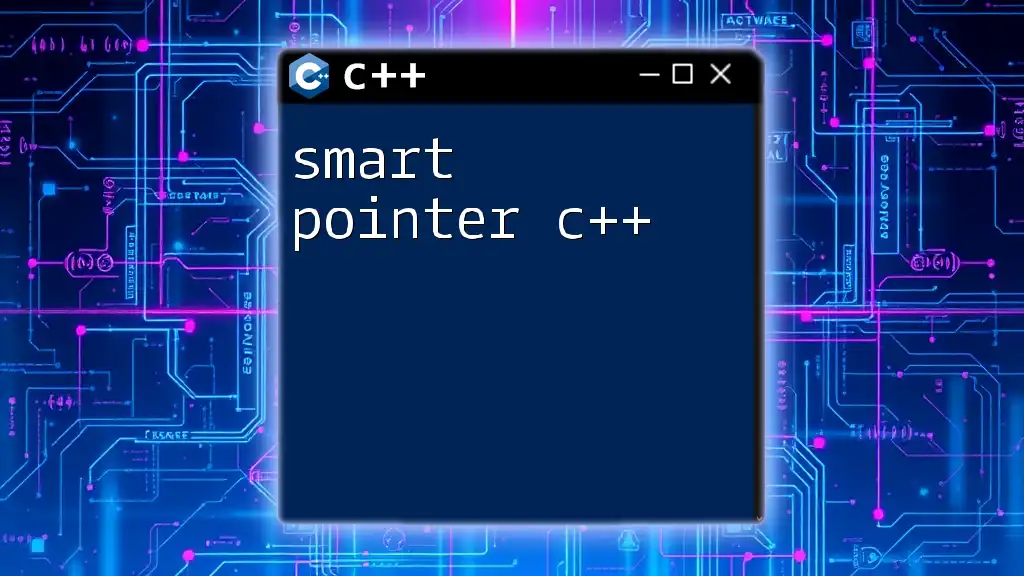
Advanced Topics in Class Pointers
Pointer to Pointer (Multi-level Pointers)
The pointer-to-pointer concept enables you to create a pointer that holds the address of another pointer. While this can be useful in special situations, it is generally recommended to use it sparingly to avoid complexity. An example would look like this:
MyClass **pptr = &myPtr;
Pointers and Inheritance in C++
Class pointers are especially beneficial when working with inheritance. You can use a base class pointer to refer to a derived class object, allowing dynamic polymorphism.
Here’s an example:
class Base {
public:
virtual void show() { std::cout << "Base Class" << std::endl; }
};
class Derived : public Base {
public:
void show() override { std::cout << "Derived Class" << std::endl; }
};
Base *basePtr = new Derived();
basePtr->show(); // Will call Derived's show()
This ability to reference derived classes through base class pointers is a powerful feature of C++ that leverages class pointers effectively.
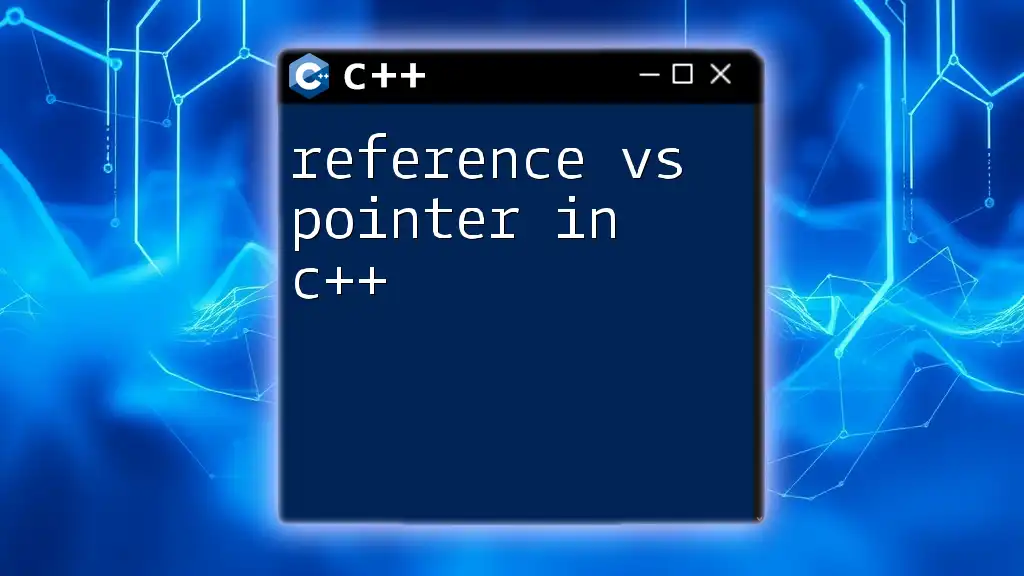
Conclusion
Class pointers in C++ are indispensable for dynamic memory allocation, object manipulation, and achieving polymorphic behavior. Mastery of class pointers not only enhances your C++ programming skills but also allows for cleaner and more efficient code. Practice the examples provided and explore various scenarios to deepen your understanding of class pointers in C++.
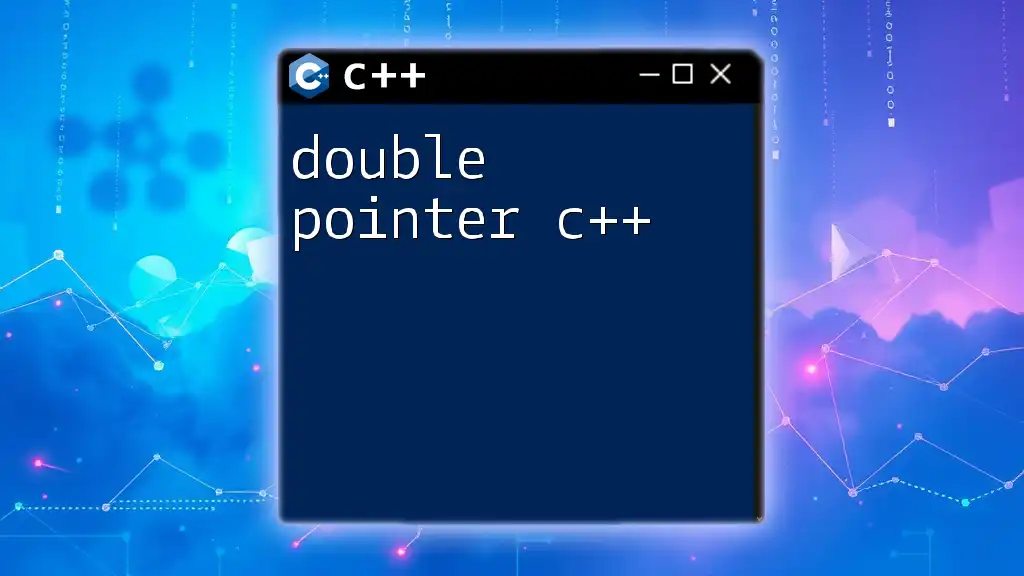
Additional Resources
For further learning, consider exploring additional tutorials on C++ pointers, memory management, and advanced C++ features. Engaging with these resources will broaden your understanding and empower you to write better C++ code.