In C++, a reference is an alias for an existing variable that cannot be changed to refer to another variable, while a pointer is a variable that holds the memory address of another variable and can be reassigned to point to different variables.
#include <iostream>
int main() {
int mainValue = 10; // mainValue is an integer variable
int& mainRef = mainValue; // mainRef is a reference to mainValue
int* mainPtr = &mainValue; // mainPtr is a pointer to mainValue's address
std::cout << "Value: " << mainValue << ", Reference: " << mainRef
<< ", Pointer: " << *mainPtr << std::endl;
mainValue = 20; // Changing mainValue to 20
std::cout << "Updated Value: " << mainValue << ", Reference: " << mainRef
<< ", Pointer: " << *mainPtr << std::endl;
return 0;
}
Understanding References in C++
What is a Reference?
In C++, a reference is essentially an alias for another variable. Declaring a reference means that you are creating a new name for an existing variable, allowing you to use the reference as if it were the original variable.
The syntax for declaring a reference is:
int value = 10;
int &ref = value; // ref is a reference to value
Here, `ref` is now a reference to `value`. Any changes made to `ref` will directly affect `value`.
Characteristics of References
References come with a few defining characteristics that distinguish them from pointers:
- Must be Initialized: A reference must be initialized when declared; it cannot exist without being tied to a variable.
- Cannot be Null: Unlike pointers, references cannot point to nothing; they must always refer to a valid variable.
- Immutability: Once a reference is initialized to a variable, it cannot be changed to reference another variable. This characteristic gives references a stable nature.
- Alias Behavior: You can think of references as different ways to access the same data, providing a clearer and more concise syntax.
Use Cases for References
Function Parameters: One of the most common use cases for references is in function parameters. Using references allows you to modify the actual variable without creating a copy of it, saving both time and memory.
void increment(int &num) {
num++; // This modifies the original variable
}
When you pass a variable to this function, any increment made affects the original variable directly, simplifying your code.
Return Types: Returning references can be beneficial, especially in operator overloading or when you need to provide access to an internal variable of an object.
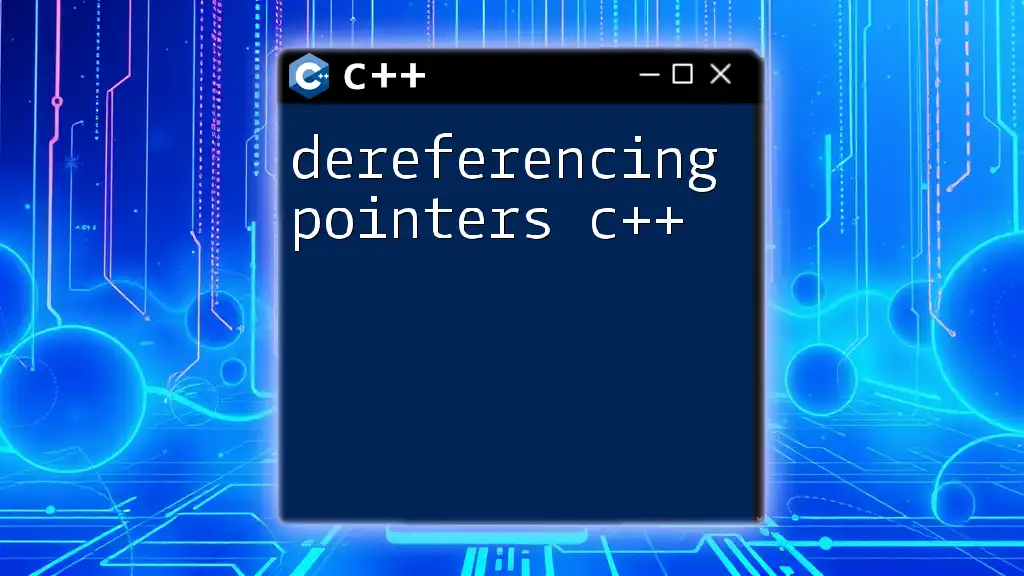
Understanding Pointers in C++
What is a Pointer?
A pointer is a variable that stores the memory address of another variable. This allows for more complex memory management as pointers can be reassigned to point to different variables or memory locations.
The syntax for declaring a pointer is:
int value = 10;
int *ptr = &value; // ptr holds the address of value
In this example, `ptr` is pointing to the memory address of `value`.
Characteristics of Pointers
Pointers come with their own unique characteristics:
- Reassignment: Pointers can be reassigned to point to different variables at any time, making them more flexible.
- Nullability: Pointers can be initialized to null, which means they do not point to any valid memory location until explicitly assigned.
int *ptr = nullptr; // pointer initialized to null
- Dereferencing: To access the value that a pointer points to, you must dereference it with the `*` operator.
cout << *ptr; // Outputs the value pointed by ptr
Use Cases for Pointers
Dynamic Memory Allocation: One of the primary uses of pointers is for dynamic memory management using `new` and `delete`.
int *arr = new int[5]; // allocates array of 5 integers in dynamic memory
delete[] arr; // free allocated memory to avoid leaks
Arrays and Pointers: In C++, arrays and pointers are closely related. The name of an array acts as a pointer to the first element of the array.
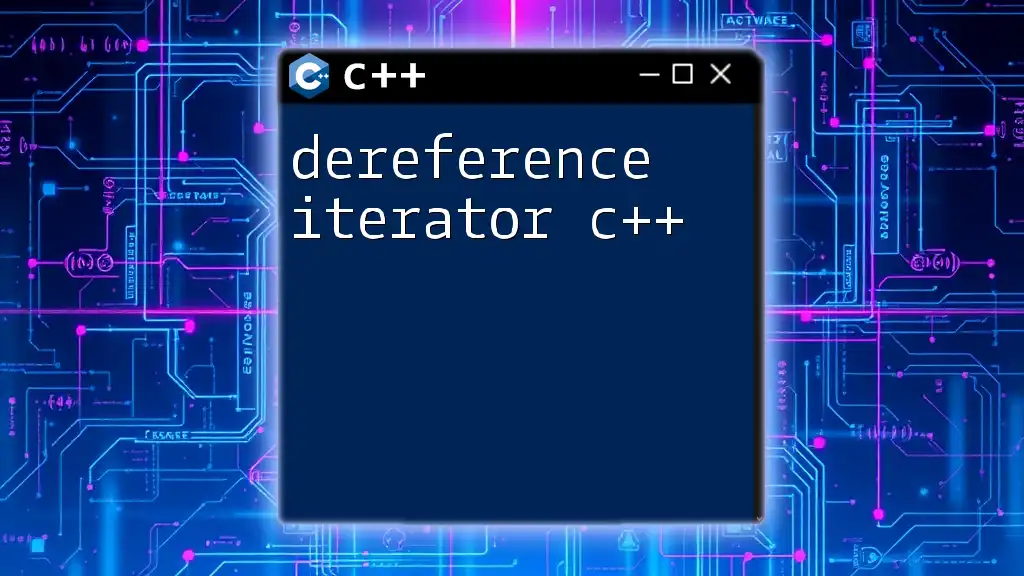
Comparing Reference and Pointer in C++
Syntax Differences
The syntax for references and pointers is notably different. A reference uses `&` when declared, while a pointer uses `*`.
int &ref = value; // Reference
int *ptr = &value; // Pointer
Memory Management
References generally provide safer and easier management of memory compared to pointers. Since references cannot be null and must always point to a valid variable, they reduce the risk of unintentional bugs.
Pointers, however, require close attention to avoid memory leaks and dangling pointers. It’s crucial to free any dynamically allocated memory using `delete` to prevent leaks.
Nullability
One of the most significant differences is the concept of nullability. A reference, by definition, can never be null, while pointers can safely be set to null.
Here's how you can check a pointer’s value:
if (ptr) {
// Pointer is pointing to a valid memory location
} else {
// Pointer is null
}
Reassignment
Pointers can be reassigned to point to different variables, enabling potentially complex point-to-point relationships. In contrast, a reference remains fixed to the original variable it was initialized with.
Usage in Function Calls
When passed to functions, pointers can be null, while references cannot. This feature means that if a reference is received by a function, the function must have a valid variable to operate on, ensuring the function cannot encounter null pointer dereferences.
Performance Considerations
In terms of performance, references can yield slight advantages since no pointer dereferencing is necessary. However, the differences are often negligible and the context of use will determine the choice between pointers or references.
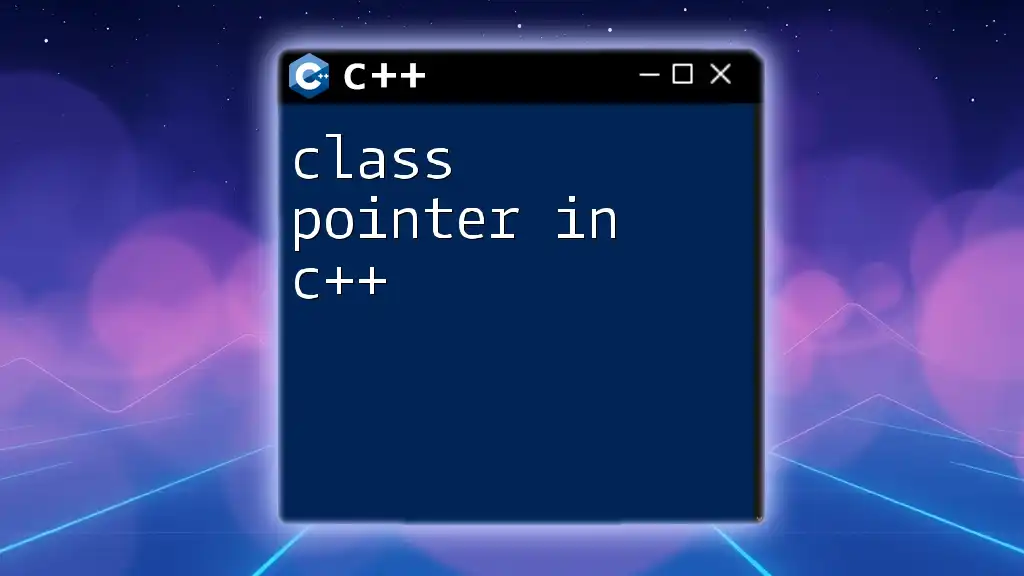
Practical Examples
Example of Using References
void display(int &num) {
cout << "Number is: " << num;
}
When you call `display(value);`, the function accesses the original variable directly without creating a copy.
Example of Using Pointers
void display(int *ptr) {
if(ptr != nullptr) {
cout << "Number is: " << *ptr;
}
}
Here, you can call `display(&value);`, but you must ensure that `ptr` is checked for null to prevent dereferencing a null pointer.
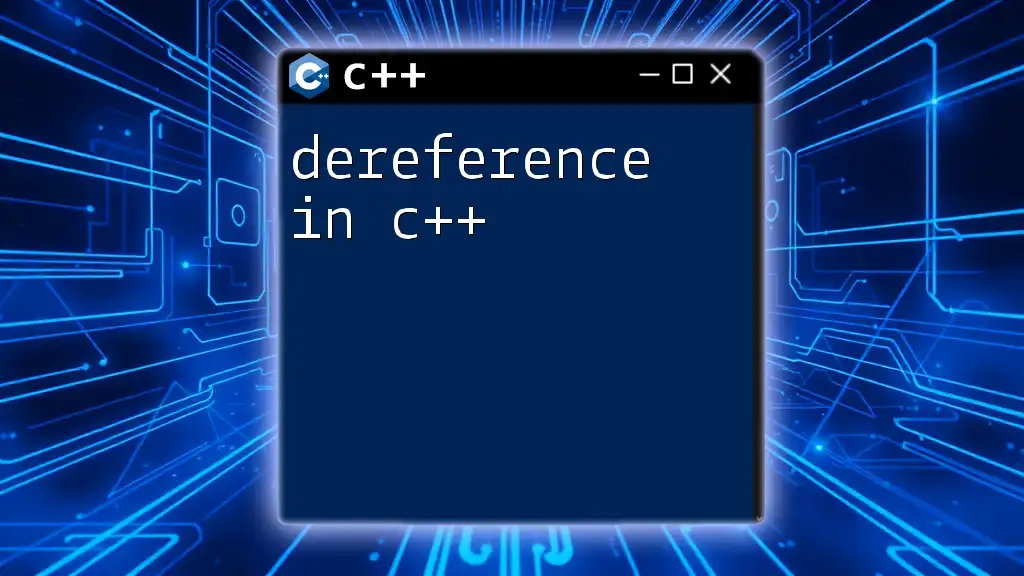
Common Misconceptions
References are Pointers
A common misconception is that references are pointers. While they often behave similarly, they are fundamentally different mechanisms in C++. References are more like constant pointers that cannot be reassigned to another variable after creation.
References are Safer
Although references provide a layer of safety, developers must understand the implications of both references and pointers. Safety in references means less complexity, but it may limit flexibility. Pointers are more powerful and expressive but require proper handling to avoid errors.
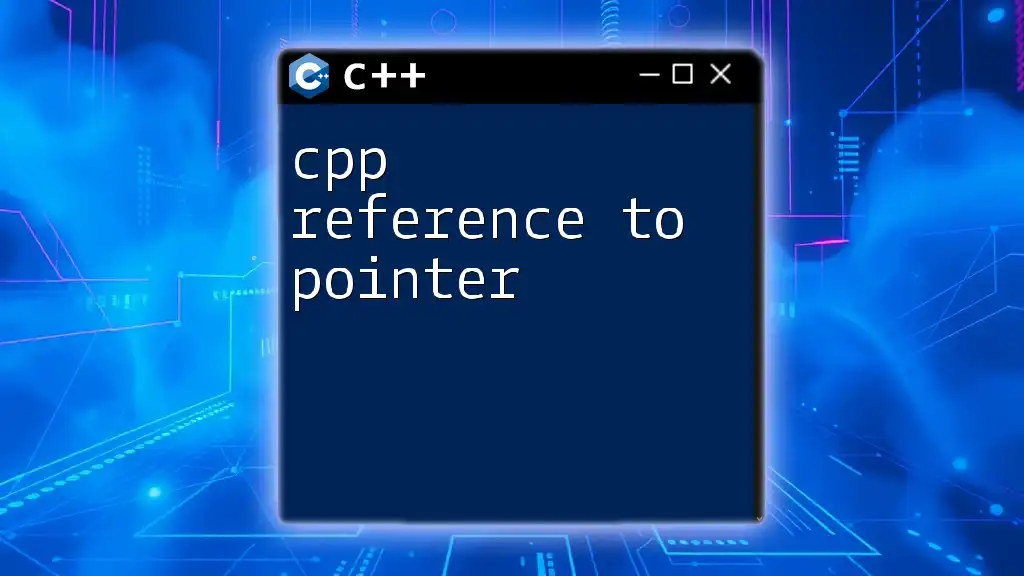
Conclusion
When considering the reference vs pointer in C++, understanding the distinctions is crucial for efficient programming. References provide safer, more concise syntax while pointers grant flexibility and control over memory. The choice between the two should be guided by the specific needs of the code you're writing, as each serves unique purposes that can greatly enhance your programming capabilities.