In C++, dereferencing a pointer allows you to access or modify the value stored at the memory address the pointer points to, using the dereference operator (`*`).
Here's a code snippet demonstrating how to dereference a pointer in C++:
#include <iostream>
int main() {
int num = 42;
int* ptr = # // Pointer to num
std::cout << "Value before dereferencing: " << *ptr << std::endl; // Dereferencing the pointer
*ptr = 100; // Modifying the value via the pointer
std::cout << "Value after dereferencing: " << num << std::endl; // Checking the modified value
return 0;
}
Understanding Pointers in C++
What is a Pointer?
A pointer in C++ is a variable that stores the memory address of another variable. Pointers are fundamental in C++ because they enable direct memory management, which is crucial for efficient programming. They allow you to manipulate memory and facilitate advanced data structures like linked lists, trees, and more.
When you declare a pointer, the syntax typically looks like this:
int* ptr; // ptr is a pointer to an integer
Why We Use Pointers
Pointers are essential for several reasons:
-
Memory Management and Efficiency: By using pointers, you can manage memory allocation more effectively, especially for dynamic memory. This leads to reduced memory overhead.
-
Dynamic Memory Allocation: Pointers allow you to allocate memory on the heap during runtime. This is vital for creating variable-sized data structures.
-
Passing by Reference: Pointers enable functions to modify variables defined outside of their scope. This is essential for performance-sensitive applications where copying large data structures would be inefficient.
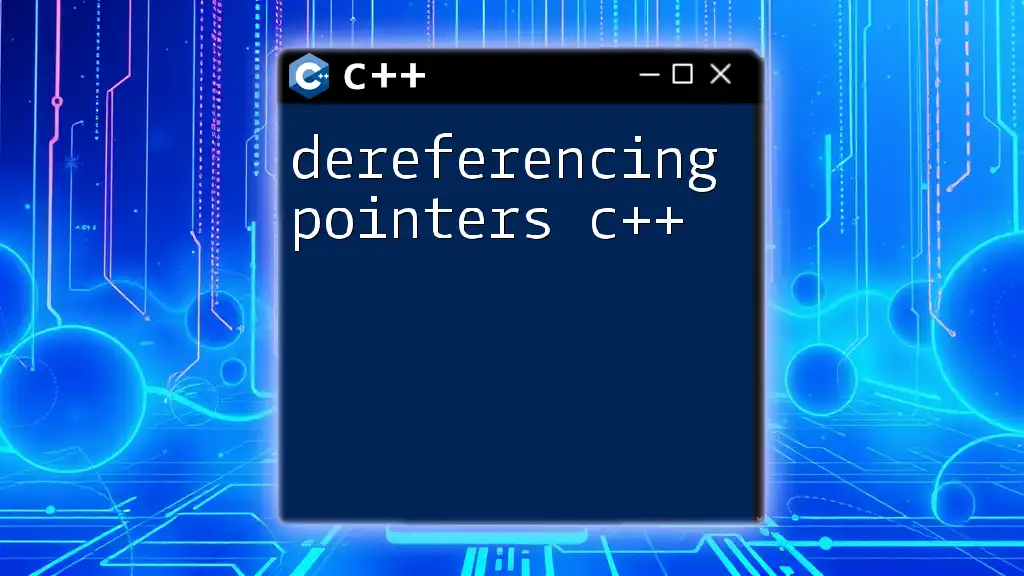
Introduction to Dereferencing
What Does Dereferencing Mean?
Dereferencing is the process of accessing the value that a pointer is pointing to. In simpler terms, when you dereference a pointer, you retrieve the actual data stored at the memory address that the pointer holds.
Understanding how dereferencing works is crucial for manipulating data in C++.
The Significance of Dereferencing in C++
Dereferencing is important because it allows you to:
- Access the actual value stored in memory, rather than just the address.
- Modify the data pointed to by the pointer, enabling dynamic and flexible code execution.
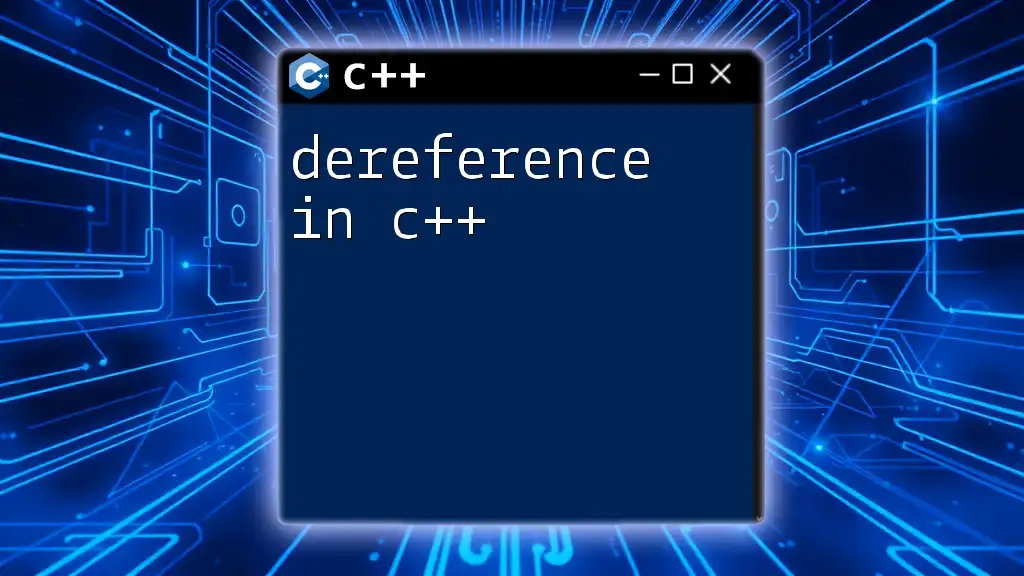
How to Dereference a Pointer C++
Basic Syntax of Dereferencing
The primary operator used for dereferencing is the dereference operator `*`.
Here's a simple example demonstrating how to dereference a pointer:
int num = 10; // An integer variable
int* ptr = # // ptr is pointing to num
std::cout << *ptr; // Outputs 10
In this example, `*ptr` accesses the value `10`, which is stored at the memory address contained in `ptr`.
Dereferencing a Pointer C++
Accessing and Modifying Values
Using the dereference operator, you can not only read values but also modify them:
*ptr = 20; // Modifying the value of num through the pointer
std::cout << num; // Outputs 20
It's crucial to be cautious when dereferencing, as attempting to dereference a null or uninitialized pointer can lead to undefined behavior and crashes.
Pointer to Pointer
What is a Pointer to a Pointer?
A pointer to a pointer is a more advanced concept where a pointer points to another pointer. This allows for multi-level data management, which can be useful in various scenarios.
Here’s an example:
int** ptr_to_ptr = &ptr; // ptr_to_ptr points to ptr
std::cout << **ptr_to_ptr; // Outputs 20
In this case, `**ptr_to_ptr` first dereferences to `ptr`, and then to the actual value (`20`).
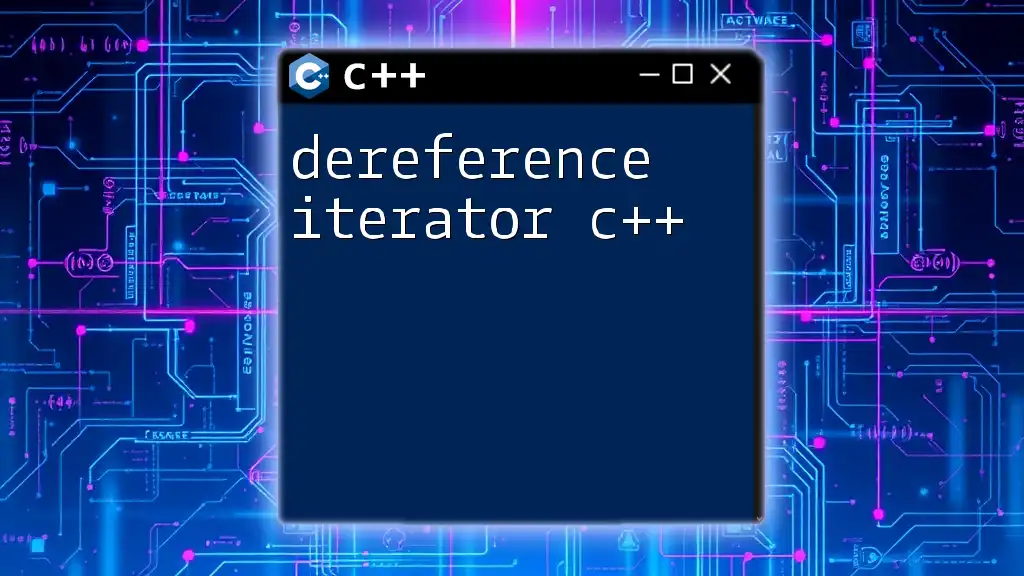
Dereferencing in C++ with Complex Data Types
Dereferencing Pointers to Objects
When working with classes, you also use dereferencing to access the member variables. Here’s an example:
class MyClass {
public:
int data;
MyClass(int val) : data(val) {} // Constructor
};
MyClass obj(10); // Object instance
MyClass* objPtr = &obj; // Pointer to the object
std::cout << objPtr->data; // Outputs 10 using dereferencing
In this example, `objPtr->data` effectively dereferences the pointer and accesses the `data` member of the object.
Dereferencing Arrays and Dynamic Arrays
Array Pointers
When working with arrays, pointers lend powerful functionality through pointer arithmetic. The name of the array represents the starting address of the array's memory.
Here’s an example of dereferencing an element in an array:
int arr[] = {1, 2, 3}; // An array of integers
int* arrPtr = arr; // Pointer to the first element
std::cout << *(arrPtr + 1); // Outputs 2
This code snippet illustrates that `*(arrPtr + 1)` accesses the second element of the array.
Dynamic Memory and Dereferencing
Pointers are also fundamental for dynamically allocated arrays. Here’s an example:
int* dynamicArray = new int[3]{1, 2, 3}; // Dynamically allocated array
std::cout << dynamicArray[0]; // Outputs 1
delete[] dynamicArray; // Important to avoid memory leaks
Common Mistakes and Best Practices in Dereferencing
Dereferencing Null or Uninitialized Pointers
One of the most critical aspects of working with pointers is ensuring they are initialized before dereferencing. Dereferencing a null or uninitialized pointer can cause runtime errors:
int* nullPtr = nullptr;
// std::cout << *nullPtr; // This will lead to undefined behavior
Best Practices for Safe Dereferencing
To operate safely in C++, always consider the following best practices:
-
Always Initialize Pointers: Uninitialized pointers can lead to unpredictable behavior.
-
Use Smart Pointers: In modern C++, smart pointers (like `std::unique_ptr` and `std::shared_ptr`) help manage memory automatically, reducing the risk of memory leaks. Here’s a quick example of a smart pointer:
#include <memory>
std::unique_ptr<int> smartPtr = std::make_unique<int>(10);
std::cout << *smartPtr; // Outputs 10
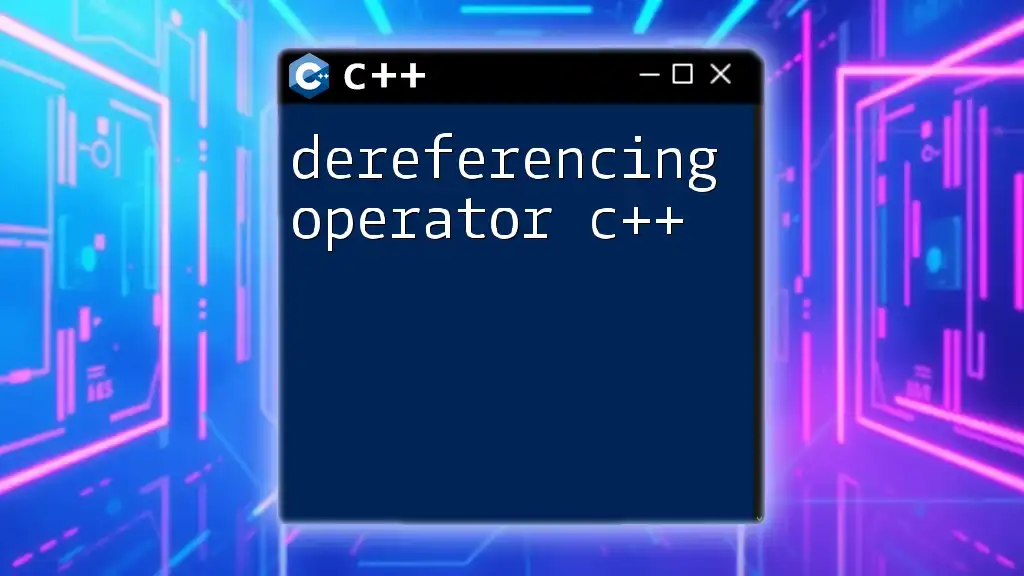
Conclusion
Recap of Key Points
Understanding how to dereference a pointer in C++ is vital for effective memory management and data manipulation. Remember the syntax and significance of the `*` operator when working with pointers. This knowledge helps you efficiently handle dynamic data and complicated structures.
Final Thoughts
I encourage you to practice dereferencing with various data types, including primitive types, classes, and arrays. Gaining proficiency in these concepts is essential for becoming an adept C++ programmer.
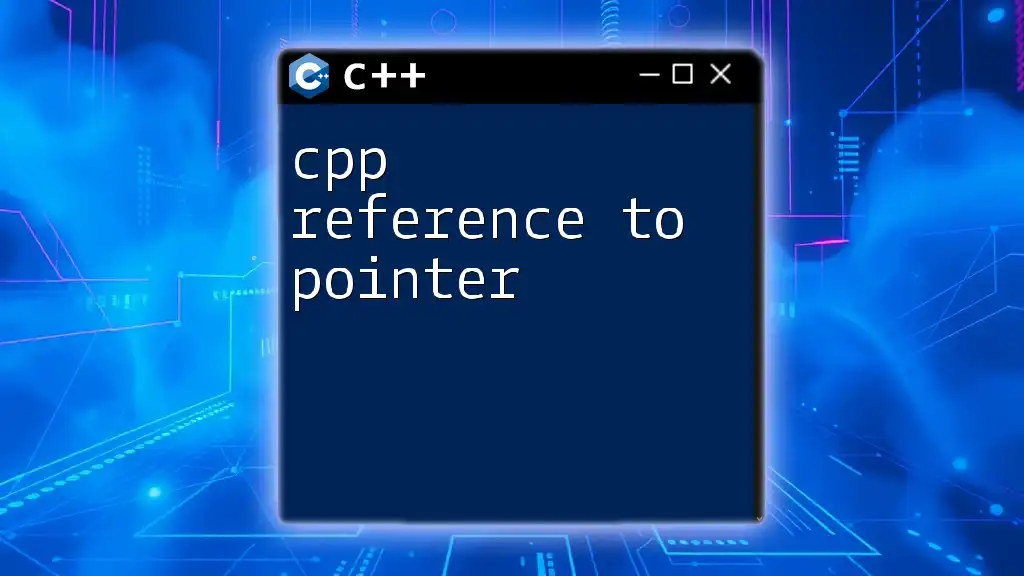
Call to Action
Join Us for More Lessons
For those eager to learn more and master C++ commands, consider signing up for our tutorials. We provide in-depth training and hands-on workshops to deepen your understanding of C++. Stay tuned for our upcoming sessions!