In C++, a reference is an alias for another variable that allows you to access and manipulate that variable directly without using a pointer.
#include <iostream>
using namespace std;
void increment(int &ref) {
ref++;
}
int main() {
int num = 5;
increment(num);
cout << "Incremented value: " << num << endl; // Outputs: Incremented value: 6
return 0;
}
Understanding References in C++
A reference in C++ is an alias for an existing variable, allowing you to create a new name that refers to the same memory location. This powerful feature enhances the flexibility of your code, enabling you to manage and manipulate data without unnecessary duplication. Unlike pointers, which can point to different memory addresses, a reference must always be bound to a variable and cannot be re-assigned to refer to another variable.
Syntax of Reference Declaration
The syntax for declaring a reference is straightforward:
data_type& reference_name = variable_name;
For example, consider the following code snippet:
int x = 10;
int& ref = x; // ref is a reference to x
In this case, `ref` is an alias for `x`: any changes made to `ref` will directly affect `x`.
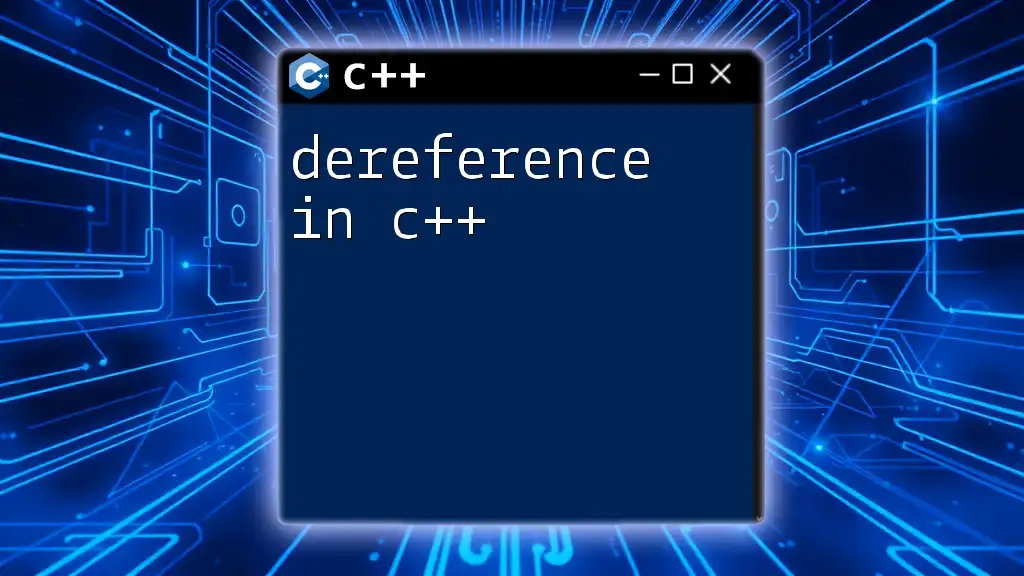
How References Work in C++
Memory Management with References
References simplify memory management in C++. When a reference is created, it points to the same memory location as the referenced variable. Thus, instead of copying data, you modify the existing value while saving compute resources.
Examples of Reference Usage
References can be used to modify original variables seamlessly. For instance:
int value = 20;
int& refValue = value; // refValue is a reference to value
refValue = 30; // value is now 30, as refValue modifies value directly
This example illustrates how a change to `refValue` directly influences `value`, demonstrating the power of references.
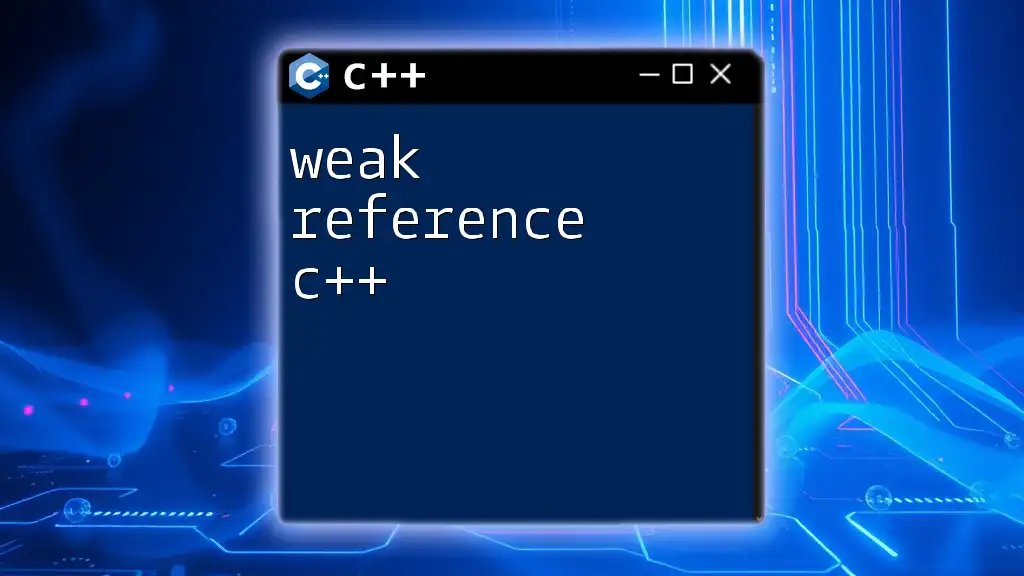
Types of References
Lvalue and Rvalue References
In C++, references can be categorized into two types: lvalue references and rvalue references.
-
Lvalue Reference: Typically used for variables that occupy a specific address in memory. The syntax is simply:
int x = 100; int& lref = x; // lvalue reference
-
Rvalue Reference: Introduced in C++11, these can refer to temporary objects or values that are about to go out of scope. The syntax looks like this:
int&& rref = 200; // rvalue reference
Use Cases for Each Type
-
Lvalue references are pertinent when you need to manipulate existing variables’ values or pass them to functions. They provide direct access to variables that have a permanent address in memory.
-
Rvalue references allow for efficient resource management in situations where temporary objects are created, enhancing the performance and memory efficiency of your applications.
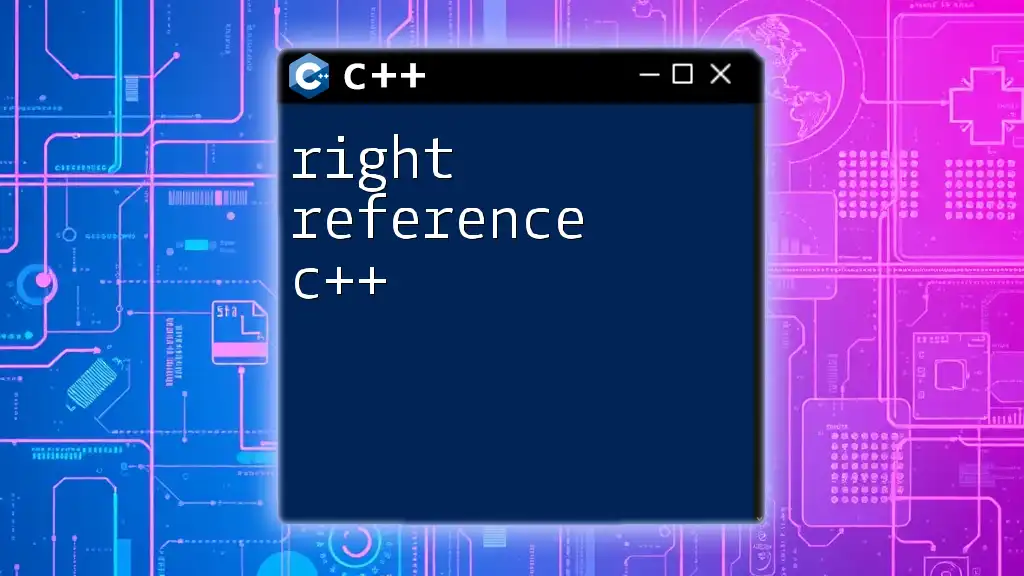
Advantages of Using References
Enhanced Performance
One of the primary benefits of using references is improved performance. When you pass parameters by reference rather than by value, you avoid the overhead associated with creating copies. For instance:
void increment(int& number) {
number++;
}
Here, the `increment` function modifies `number` directly without copying, which is particularly advantageous with large data structures.
Code Clarity and Maintenance
References help improve code clarity as they eliminate the confusion that can arise from pointers. Instead of managing explicit memory addresses, you work with familiar variable names. For example, using references would result in simpler, clearer code compared to handling pointers:
void updateValue(int& val) {
val = 42; // clearer than manipulating pointers
}
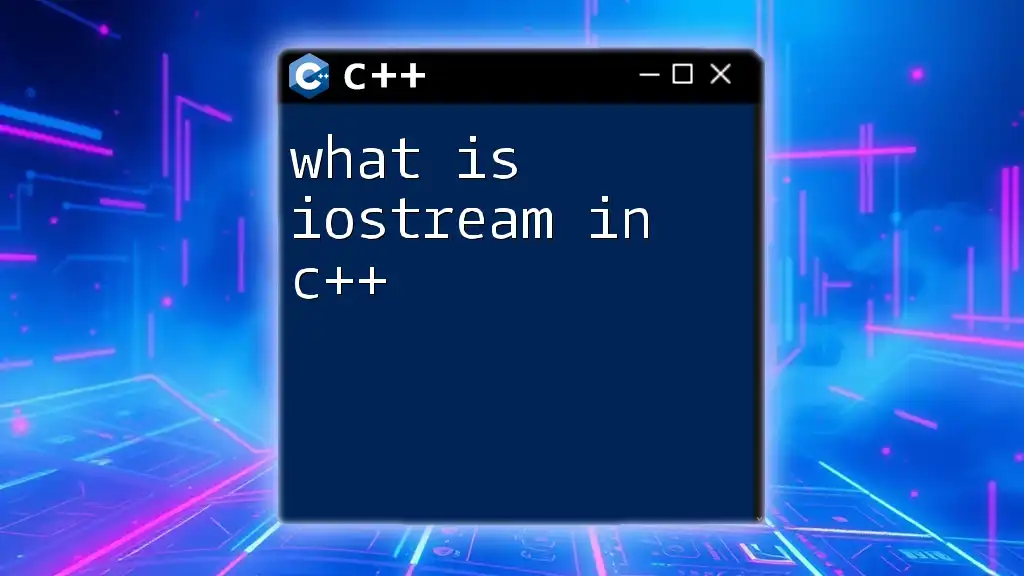
Potential Pitfalls with References
Dangling References
One must be cautious to avoid dangling references, which occur when a reference refers to a variable that has gone out of scope. For instance, consider:
int* ptr = new int(5);
int& refDangling = *ptr; // refDangling points to allocated memory
delete ptr; // refDangling now refers to deallocated memory
Accessing `refDangling` after `ptr` has been deleted can lead to undefined behavior.
Const References
Using const references provides a safety net by preventing modification of the referenced data. This feature is invaluable, especially when passing arguments to functions:
void display(const int& number) {
cout << number << endl; // Cannot modify number
}
By using const references, you ensure that data integrity is maintained.
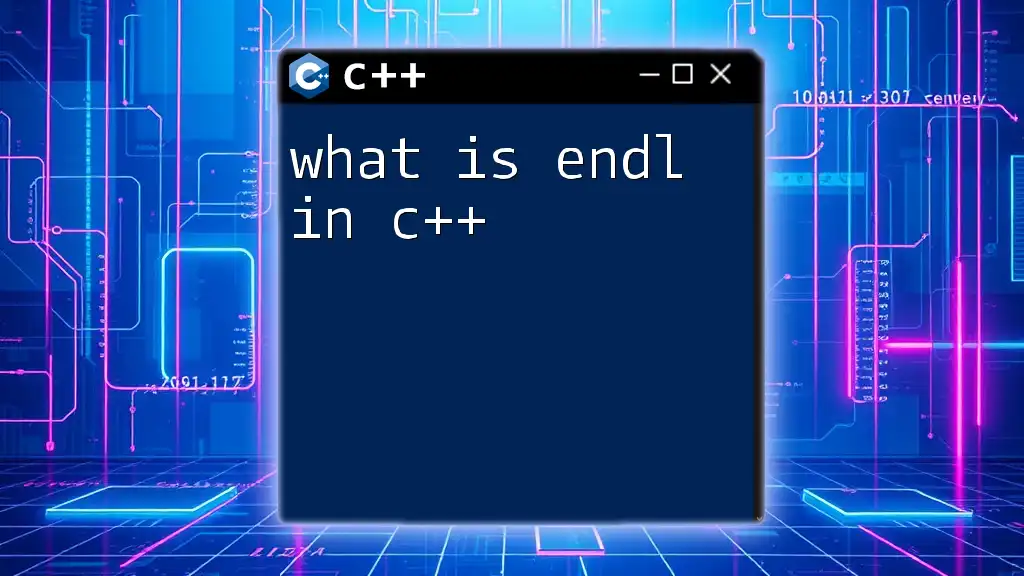
Practical Applications of References in C++
Function Parameters
References are particularly useful for function parameters. They allow you to modify the original argument directly and facilitate efficient data manipulation:
void increment(int& value) {
value++;
}
Here, passing by reference allows the function to modify `value` directly without needing a return statement.
Operator Overloading
References are also essential in operator overloading, allowing you to create more intuitive and efficient operations. For example:
class Number {
public:
int value;
Number& operator=(const Number& other) {
value = other.value; // Using reference for assignment
return *this;
}
};
This example illustrates how overloaded operators utilize references to manage data efficiently, ensuring that the operations remain straightforward and efficient.
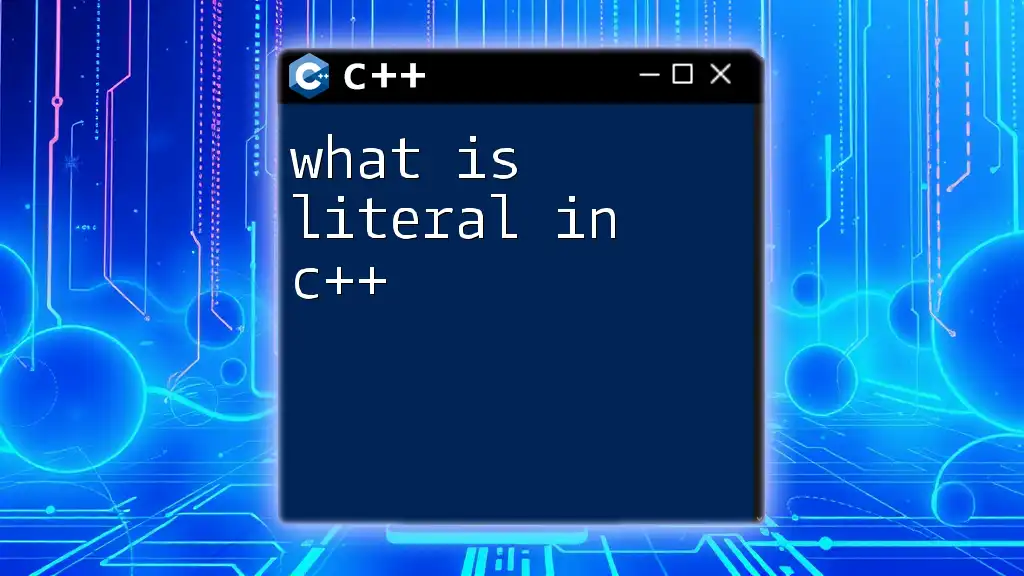
Conclusion
In conclusion, understanding what a reference is in C++ is vital for writing efficient, maintainable code. References provide a means to work with variables without unnecessary copies, enhance performance, and save time, all while keeping your code clean and understandable. By carefully applying references in your programming practices, you can significantly improve the quality and efficiency of your C++ applications.
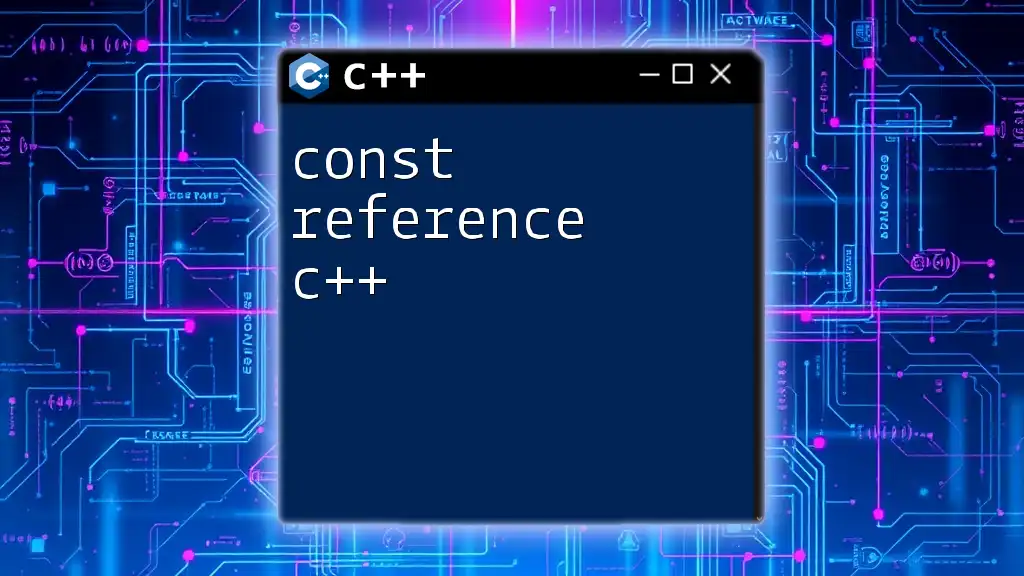
Further Reading and Resources
For more in-depth information, consider exploring the official C++ documentation, engaging with recommended books on C++, and enrolling in courses dedicated to mastering C++ programming.
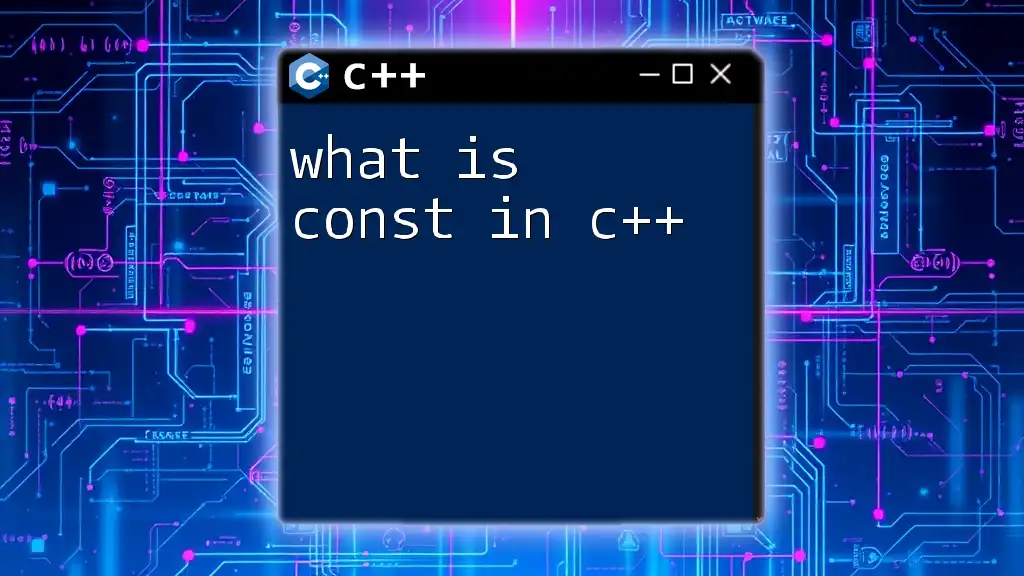
Call to Action
Embrace the power of references in your coding endeavors — practice applying these concepts to become more proficient in C++. Don’t forget to share this knowledge with your peers and join a community of learners to further enhance your C++ skills!