A double in C++ is a data type that represents a double-precision floating-point number, allowing for the storage of decimal values with greater accuracy than the float type.
double pi = 3.14159;
Understanding Data Types in C++
Understanding Data Types
In programming, data types are classifications that specify which type of value a variable can hold. Each type is defined by its storage size, the range of values it can represent, and the operations that can be performed on those values. Choosing the appropriate data type is crucial for both performance and memory management in your application.
Overview of Floating-Point Data Types
Among various data types, floating-point types are used for representing real numbers, which can include fractions. In C++, there are three primary floating-point data types:
- `float`: Typically used for single-precision, requiring 4 bytes of memory.
- `double`: Used for double-precision, generally occupying 8 bytes.
- `long double`: A higher precision type, with varying memory size depending on the architecture, but often larger than 8 bytes.

What is a Double in C++?
Definition of Double
A double in C++ is a data type that represents double-precision floating-point numbers. It is capable of storing large ranges of values with considerable precision, making it suitable for scientific calculations, high-precision computations, and applications requiring large numeric ranges.
Characteristics of Double
Memory Size and Precision
A `double` typically consumes 8 bytes (64 bits) of memory. This larger size allows it to store numbers in a significantly broader range and with increased accuracy than a `float`. A `double` can represent values ranging from approximately 1.7E-308 to 1.7E+308, which is useful when working with extreme values in mathematical computations.
Double vs Other Floating-Point Types
When comparing double with other floating-point types, we can summarize their use cases as follows:
-
Double vs Float: While `float` is often sufficient for simple mathematical operations and graphics, `double` is preferred when higher precision is required. For example, engineers and scientists selecting values from particular formulas might opt for `double` to minimize rounding errors.
-
Double vs Long Double: In situations where extreme precision is necessary, such as in high-level scientific computations, `long double` may be used. However, this can vary by compiler and platform, making `double` the more reliable choice for general applications.
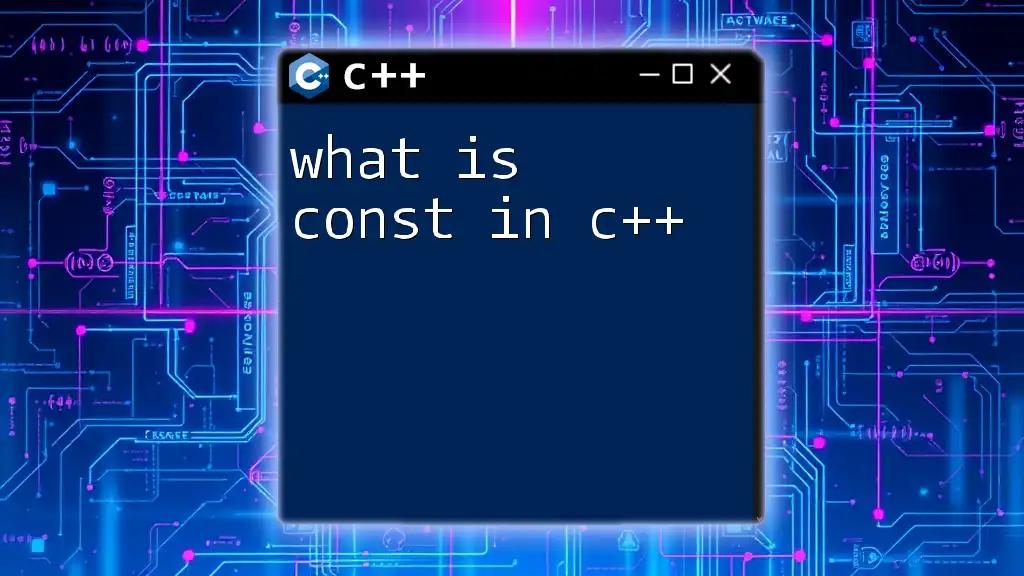
How to Declare a Double in C++
Syntax for Declaration
To declare a double variable in C++, you use the following syntax:
double variableName;
Examples of Double Declarations
You can declare a `double` variable in several ways. For instance:
-
Single Declaration:
double pi = 3.14159;
Here, `pi` effectively represents the well-known mathematical constant.
-
Multiple Declarations in One Line:
double length = 10.5, width = 5.0, area;
This allows you to declare multiple variables at once for conciseness in your code.

Using Double in C++: Basic Operations
Arithmetic Operations
Doubles can be utilized in standard arithmetic operations. Here are some examples:
-
Addition:
double result = 2.5 + 3.8; // result = 6.3
-
Subtraction:
double diff = 5.0 - 2.5; // diff = 2.5
-
Multiplication:
double product = 4.0 * 2.5; // product = 10.0
-
Division:
double quotient = 10.0 / 3.0; // quotient ≈ 3.3333...
These operations illustrate how doubles can handle decimal values effectively, thus giving you the power to perform precise calculations.
Utilizing Double in Functions
You can also utilize doubles in functions. Here’s a simple example of a function that calculates the area of a rectangle:
double calculateArea(double length, double width) {
return length * width;
}
In this function, both parameters are of type `double`, ensuring that any decimal values passed to the function maintain their precision during calculations.

Features and Limitations of Doubles
Advantages of Using Doubles
One of the primary advantages of using doubles is higher precision. Doubles can manage larger ranges of floats and reduce rounding errors that can occur with `float`. This makes them a standard choice in scientific computing, where precision is paramount.
Limitations of Doubles
However, there are limitations associated with doubles. One significant issue is precision loss. While doubles offer increased precision over floats, they can still introduce rounding errors in calculations that require extreme accuracy. Additionally, while using doubles may provide precise results, they may introduce performance overhead compared to simpler data types.

Best Practices for Using Doubles in C++
When to Use Double
Using `double` is ideal when you are working with large numbers or require computations that involve non-integer values. It is particularly recommended in contexts like physics simulations, financial calculations, or any scientific applications that require precision.
Common Pitfalls to Avoid
-
Implicit Type Conversions: Mixing data types (like adding an `int` to a `double`) can lead to implicit conversions that alter values, introducing subtle bugs.
-
Arithmetic with Mixed Types: If you mix types during calculations, ensure you understand how C++ handles arithmetic operations between `float`, `double`, and `int` to avoid unexpected behavior in your results.

Conclusion
In summary, understanding what is a double in C++ is critical for any programmer interested in performing accurate numerical computations. Doubles represent double-precision floating-point numbers with a larger range and greater precision than floats, making them ideal for various applications. However, it is essential to be mindful of potential limitations, such as precision loss and performance concerns, while ensuring you apply best practices in your coding endeavors.
Engaging with double data types will empower you to handle a vast array of programming situations, so go ahead and practice using doubles in your projects to harness their full potential!
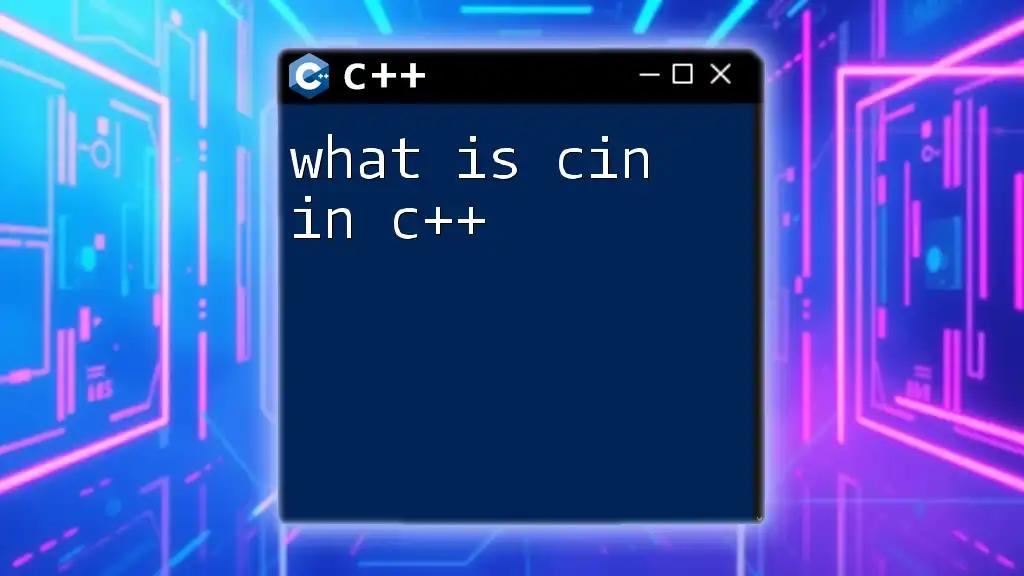
Additional Resources
Consider exploring various books and online courses to deepen your understanding of C++ and its data types. Joining community forums is also a great way to seek help and participate in discussions with fellow programmers.

FAQs
What is the maximum value a double can hold in C++?
The maximum value a `double` can store is approximately 1.7E+308. This range makes it suitable for extensive scientific calculations, although this can vary depending on the specific implementation and architecture.
Can a double store integer values?
Yes, a `double` can store integer values. However, when storing integers in a double, you may introduce unnecessary precision that can lead to potential rounding issues if not managed correctly. Always consider the context of your calculations to determine the best data type to use.