In C++, `float` and `double` are both data types used to represent floating-point numbers, with `float` being a single-precision (32-bit) type and `double` being a double-precision (64-bit) type, allowing for more precision in numerical calculations.
#include <iostream>
int main() {
float f = 3.14f; // Single precision
double d = 3.141592; // Double precision
std::cout << "Float: " << f << "\nDouble: " << d << std::endl;
return 0;
}
What are Float and Double?
Definition of Float
A float in C++ is a single-precision floating-point data type, occupying 4 bytes in memory. This type is used to store real numbers, helping represent any value that can have a fractional component. Common use cases for `float` include graphics applications, where a smaller memory footprint is greater than extreme precision.
Definition of Double
On the other hand, a double is a double-precision floating-point type, which takes up 8 bytes in memory. It provides more precision compared to `float`, making it suitable for computations that require greater accuracy. Scenarios such as scientific calculations and high-precision measurements typically favor the use of `double`.
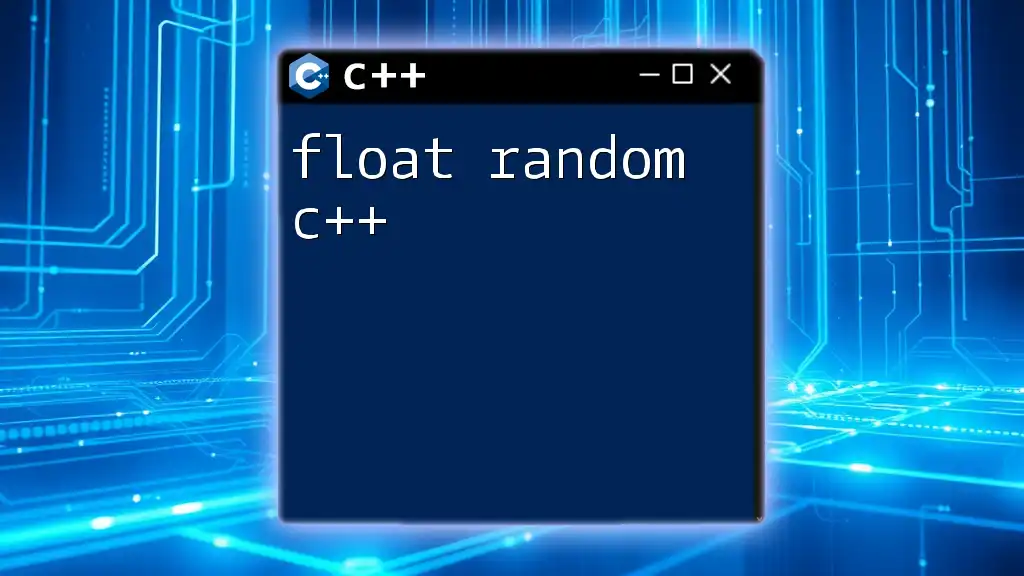
The Difference Between Float and Double in C++
Memory Usage
Understanding the size in memory between `float` and `double` is crucial when deciding which data type to use.
- A `float` consumes 4 bytes of memory.
- A `double` consumes 8 bytes of memory.
This difference can significantly affect performance, particularly in systems where memory usage is a priority. In such cases, opt for `float` when precision can be sacrificed for speed and space.
Precision and Range
The precision of a floating-point type denotes how many significant figures it can represent accurately. Here’s a breakdown:
- Float: Can typically maintain approximately 7 decimal digits of precision.
- Double: Can maintain around 15 decimal digits of precision.
The range of these types also differs:
- Float: From 1.2E-38 to 3.4E+38.
- Double: From 2.3E-308 to 1.7E+308.
The trade-off between the two lies in both precision and range. For instance, using `float` in a financial application could lead to rounding errors in monetary calculations due to its limited decimal places. On the contrary, `double` offers more significant digits, suitable for critical calculations. An example of precision differences can be demonstrated in this code snippet:
float f1 = 1.1234567f; // Only 7 digits
double d1 = 1.123456789012345; // More precision with double
Performance Considerations
While it might be tempting to think that `float` is always faster due to its smaller memory footprint, this isn't universally true. The impact on performance can vary significantly based on the processor architecture. Some architectures handle `double` types more efficiently than `float`. Therefore, it is essential to perform benchmarks specific to your environment, especially when working within performance-critical applications.
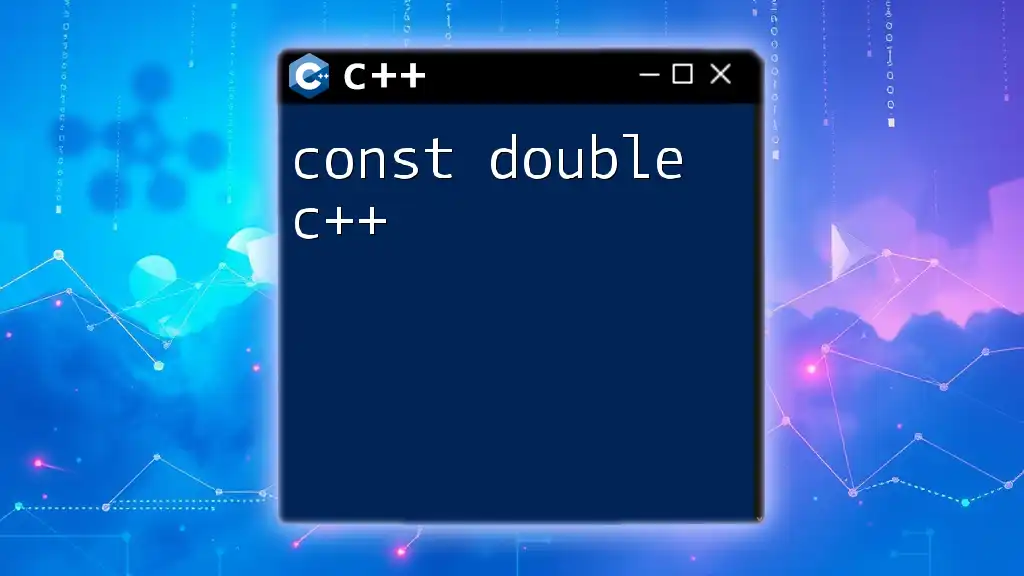
Practical Examples
Example of Float Usage
Here’s a quick demonstration on how to use `float` in code:
float a = 5.75f;
float b = 2.25f;
float result = a + b; // result is 8.0
In this code:
- `a` and `b` are defined as `float` variables, with a suffix `f` added to ensure they are treated as floats.
- The addition of `a` and `b` results in `result`, which retains the expected float value, maintaining simplicity in operations.
Example of Double Usage
For a clearer illustration of the precision advantages of `double`, consider the following:
double x = 1.23456789012345;
double y = 0.00000000000100;
double sum = x + y; // sum shows higher precision
In this code snippet:
- `x` and `y` are defined as `double` values.
- The addition of `x` and `y` yields a sum that reflects the higher precision of `double`, showcasing an essential factor for applications that need meticulous calculations.
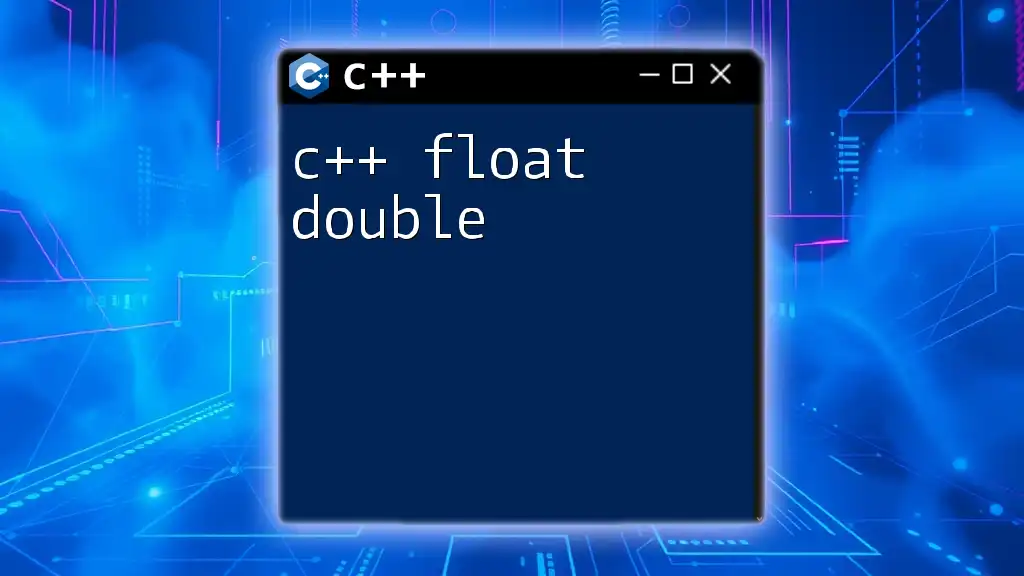
When to Use Float or Double
Scenarios Favoring Float
Using `float` is ideal when:
- The application requires lower memory consumption.
- Tasks like graphics processing or gaming, where visual effects emphasize performance rather than accuracy.
Scenarios Favoring Double
Conversely, scenarios that benefit from `double` include:
- Scientific computations where accuracy is critical.
- Financial applications that require exact representations of currency without rounding errors.
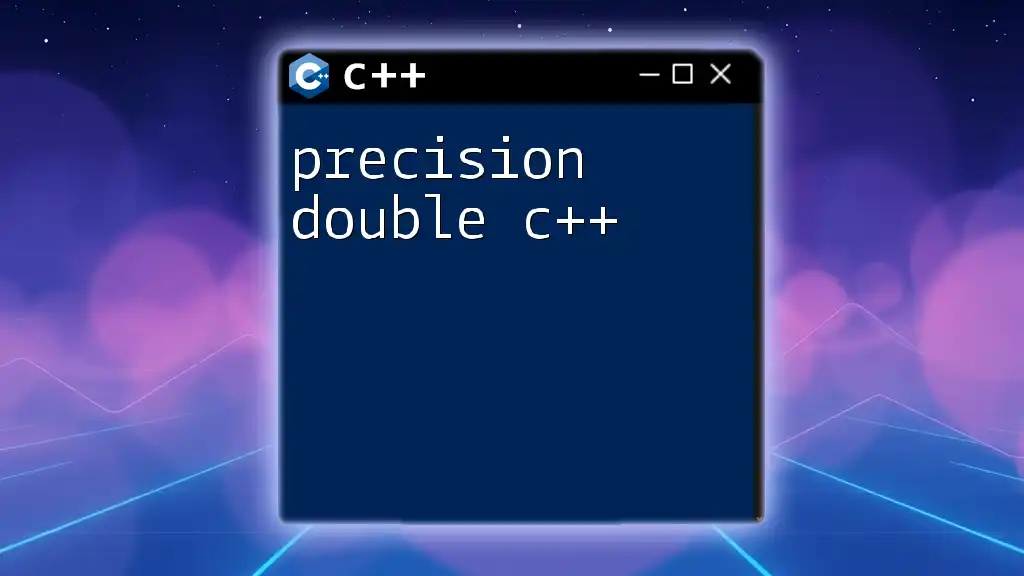
Common Misconceptions
Misconception About Speed
A prevalent misconception is that `float` consistently offers better performance than `double`. This is not always the case, as some modern processors are optimized for `double` operations. In applications where execution speed and accuracy are important, consider profiling performance rather than defaulting to `float`.
Misconception About Range
Many assume that the range difference diminishes due to being "just numbers." However, understanding the profound implications of their ranges—especially in high-stakes calculations—remains essential.
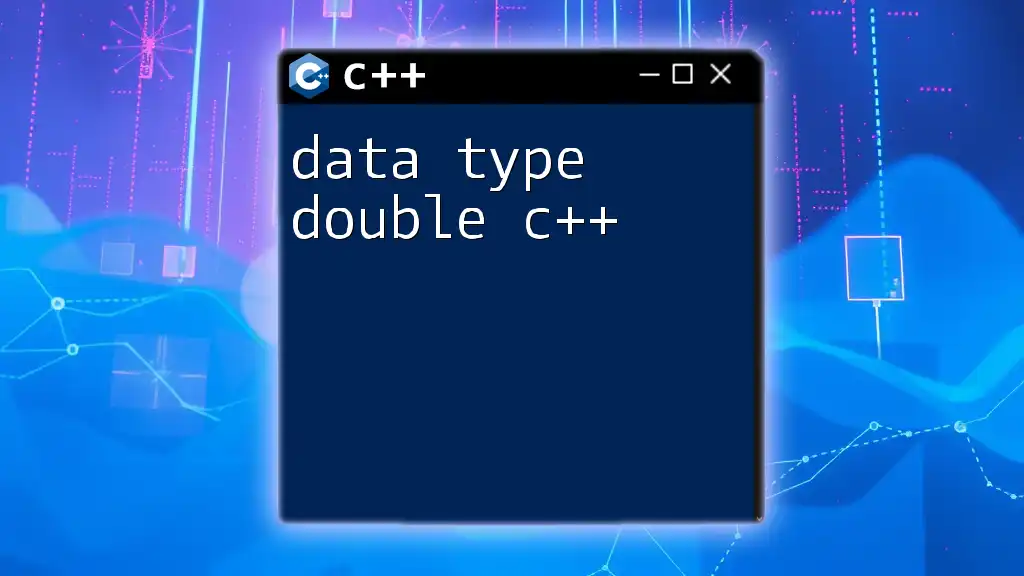
Conclusion
In conclusion, understanding the differences between `float vs double` in C++ is vital for any developer. The choice between these two types should be informed by the specifics of the application—memory usage, precision requirements, and performance considerations play significant roles. Always keep in mind the requirements of your project when deciding which to implement, and practice using these types in your coding challenges.
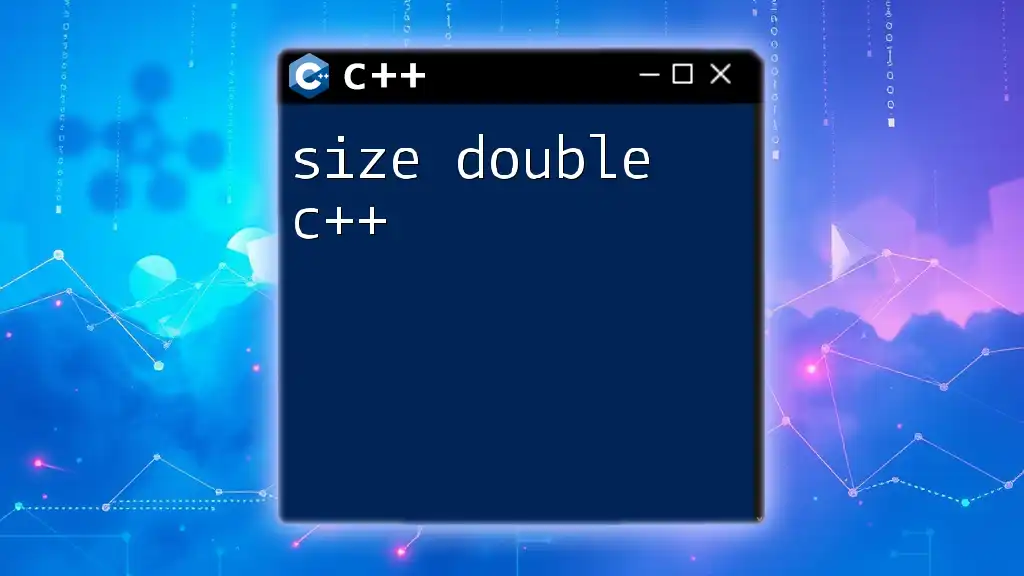
Additional Resources
For further reading on floating-point arithmetic, consider delving into the references provided in the C++ documentation. Gaining a deeper understanding of these concepts can enhance your programming skills and help you make better decisions in your coding practices.
Call to Action
We encourage you to share your experiences with selecting between `float` and `double` in your C++ projects. What challenges did you face? What solutions did you find? Join the conversation and expand your understanding of floating-point types!