In C++, `free` is used to deallocate memory that was allocated with `malloc`, while `delete` is used to deallocate memory allocated with `new`, and they should not be mixed to avoid undefined behavior.
#include <iostream>
int main() {
int* arr = new int[10]; // Allocates memory with new
delete[] arr; // Use delete to free the memory
// int* p = (int*)malloc(10 * sizeof(int)); // Allocates memory with malloc
// free(p); // Use free to deallocate the memory
return 0;
}
The Basics of Dynamic Memory Allocation
What is Dynamic Memory?
Dynamic memory refers to the memory that is allocated during the runtime of a program. This type of memory management is crucial in C++, allowing for flexible memory usage based on the program's needs. Unlike static memory allocation, which is fixed and determined at compile time, dynamic memory enables developers to allocate memory as required, making efficient use of resources.
Allocating Memory in C++
In C++, memory can be allocated dynamically using two primary techniques: `new` and `malloc()`.
Using `new`: The `new` operator allocates memory for a single object or an array and returns a pointer to the allocated memory.
int* array = new int[10]; // Allocates an array of 10 integers
Using `malloc()`: The `malloc()` function, part of the C standard library, also allocates memory but does not call constructors for objects.
int* array = (int*)malloc(10 * sizeof(int)); // Allocates an array of 10 integers
Both `new` and `malloc()` provide ways to allocate memory, but they differ in their approach to initialization and object-oriented features.
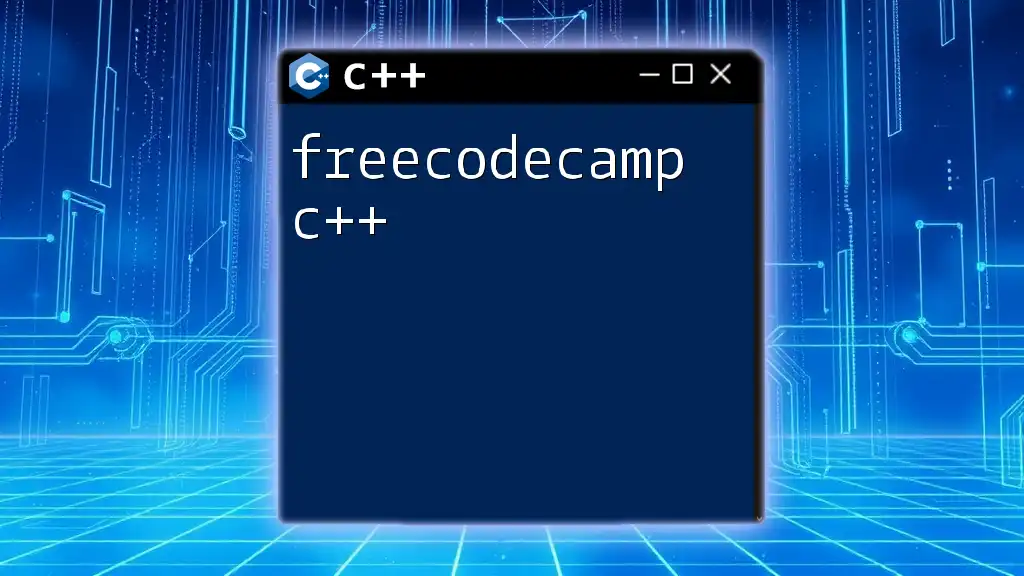
Understanding Free in C++
What is `free()`?
The `free()` function is used to deallocate memory that was previously allocated with `malloc()`, `calloc()`, or `realloc()`. The function takes a pointer to the memory block that you wish to free, effectively returning that memory to the system.
Using `malloc()` and `free()` Together
Here is an example of the complementary use of `malloc()` and `free()`:
int* array = (int*)malloc(10 * sizeof(int)); // Dynamically allocate an array
if (array != nullptr) {
// Use the array...
}
free(array); // Deallocate memory
It is essential to ensure that `free()` is called only with pointers allocated via `malloc()`; otherwise, it may lead to undefined behavior.
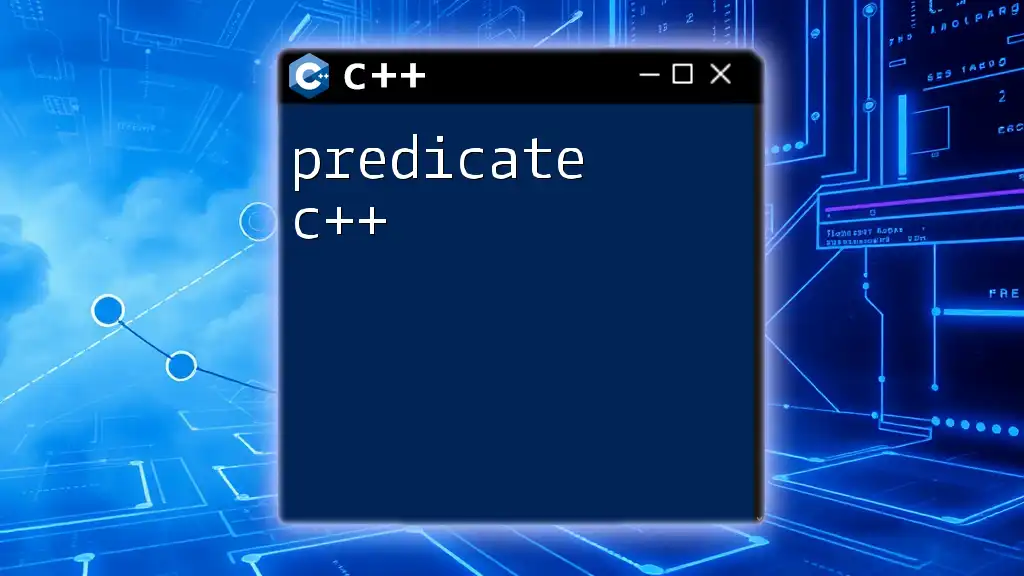
Understanding Delete in C++
What is `delete`?
The `delete` operator is used to deallocate memory that was allocated with `new`. Unlike `free()`, `delete` also calls the destructor of the class when dealing with objects, ensuring proper resource management.
Using `new` and `delete` Together
Example of using `new` and `delete`:
int* array = new int[10]; // Dynamically allocate an array
// Use the array...
delete[] array; // Deallocate memory
In this case, `delete[]` is essential as it frees memory allocated with `new[]`, ensuring that each element's destructor is called appropriately.
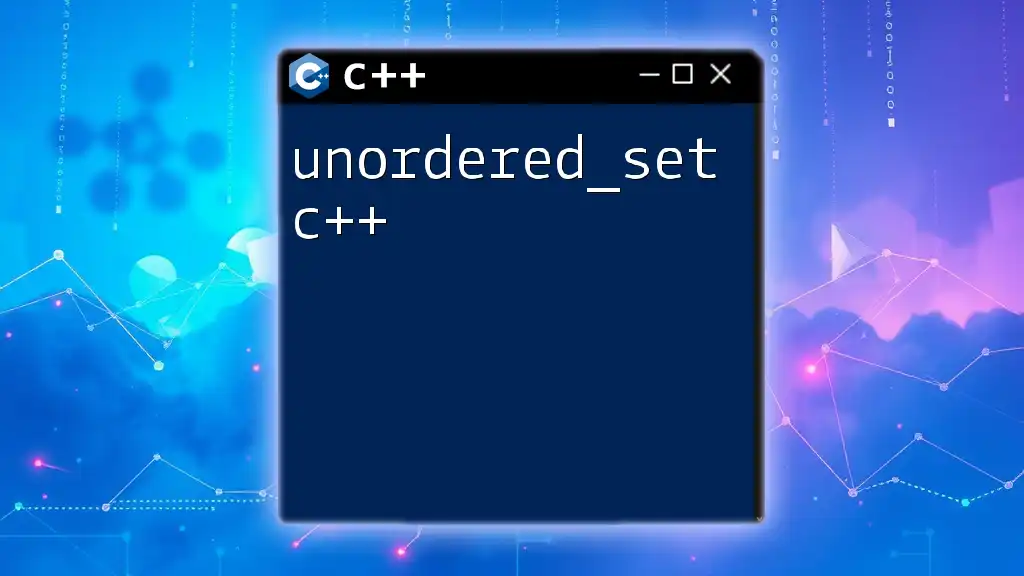
Key Differences Between Free and Delete
Memory Management Functions
- Syntax and Usage:
- `free()` is a function, while `delete` is an operator. This fundamental difference implies that `delete` also manages destructors.
Memory Types
- Memory Allocated with `malloc()` vs `new`:
- `malloc()` and `free()` work with raw memory allocation, while `new` and `delete` are designed for objects, invoking constructors and destructors as needed.
Destructor Calls
- Destructor Behavior:
- Using `delete` ensures that destructors for class instances are executed, which is not the case with `free()`. This is critical for classes that manage resources, such as file handles, pointers, etc.
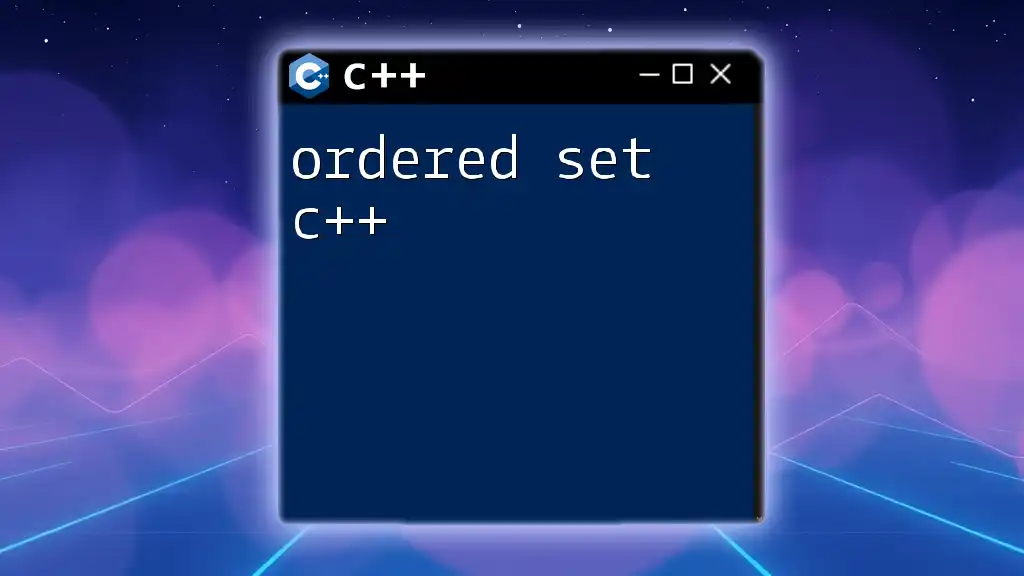
When to Use Free vs Delete
Choosing the Right Tool for the Job
- Use `free()` when working with memory allocated by `malloc()`, `calloc()`, or `realloc()`.
- Use `delete` when handling objects created with `new`.
Performance Considerations
In terms of performance, while `free()` is generally faster because it doesn’t check destructor calls, using `delete` offers safety in resource management, particularly in complex applications.
Example of a potential performance variable:
MyClass* obj = new MyClass(); // Allocates and constructs
delete obj; // Deallocates and destructs
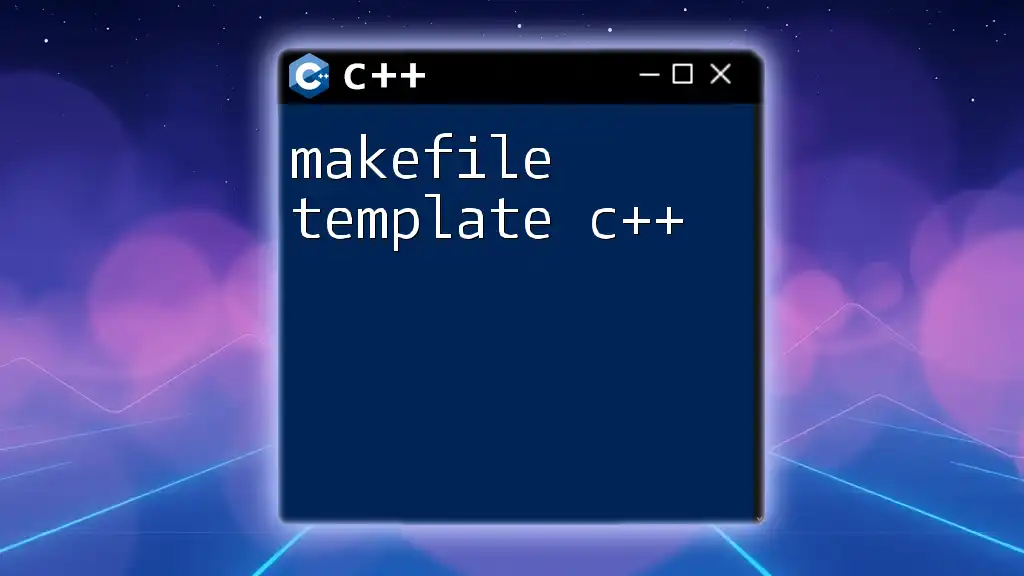
Common Pitfalls and Best Practices
Avoiding Memory Leaks
Memory leaks occur when dynamically allocated memory is not properly deallocated. It's essential to track all allocations and ensure that corresponding `free()` or `delete` calls are made.
Not Mixing `new`/`delete` with `malloc()`/`free()`
Mixing these can cause undefined behavior. For example:
int* ptr = new int;
free(ptr); // Undefined behavior
Best Practices for Resource Management
Utilizing smart pointers such as `std::unique_ptr` or `std::shared_ptr` can mitigate many common memory management issues. These provide automatic memory management by freeing memory when they go out of scope or are no longer referenced.
Example of using `std::unique_ptr`:
#include <memory>
std::unique_ptr<int> ptr(new int); // Automatically deallocates when going out of scope
![Effortless Memory Management: Delete[] in C++ Explained](/images/posts/d/delete-cpp.webp)
Conclusion
Understanding the differences and appropriate usage of `free()` and `delete` is critical for efficient memory management in C++. Mismanagement can lead to serious issues such as memory leaks and undefined behavior. By following the above guidelines and utilizing advanced features like smart pointers, developers can ensure better resource management in their applications.
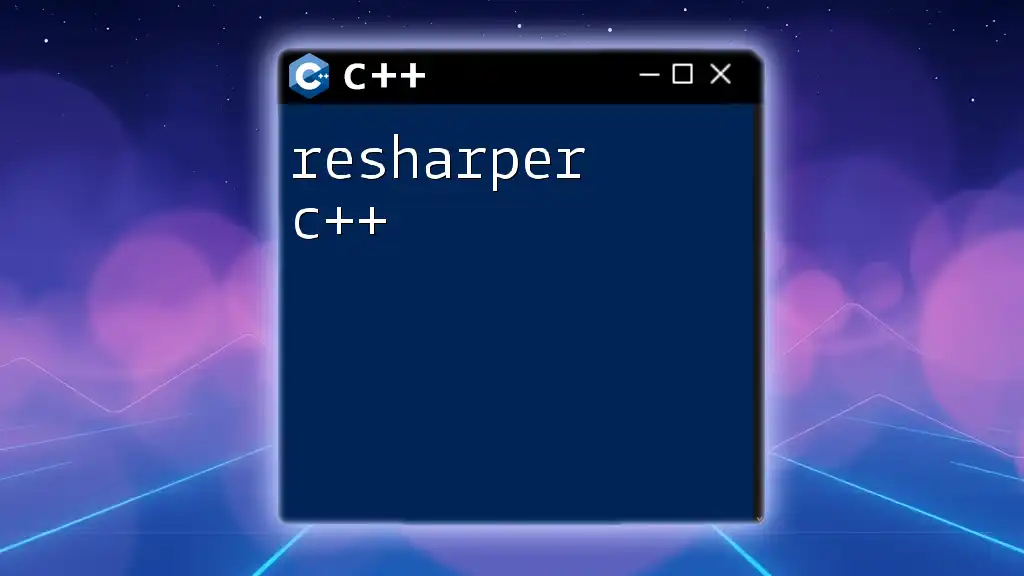
Code Snippets
Here are some simplified, clear code snippets demonstrating key concepts:
Using malloc and free:
int* arr = (int*)malloc(5 * sizeof(int)); // Allocate
for (int i = 0; i < 5; ++i) arr[i] = i; // Initialize
free(arr); // Deallocate
Using new and delete:
int* arr = new int[5]; // Allocate
for (int i = 0; i < 5; ++i) arr[i] = i; // Initialize
delete[] arr; // Deallocate
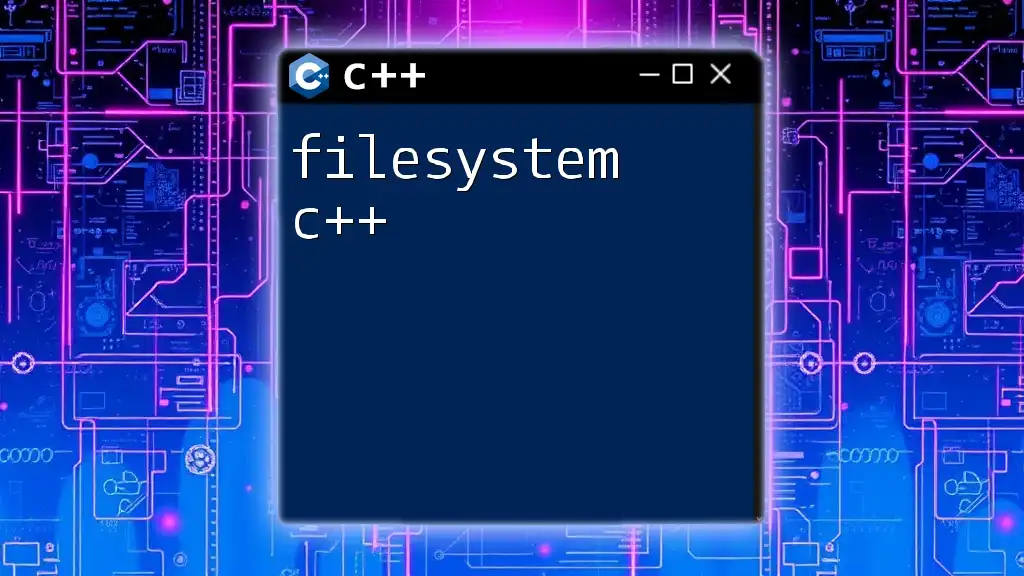
FAQs
Common Questions on Free vs Delete
-
Can I use `free()` on a pointer allocated with `new`?
- No. This will lead to undefined behavior.
-
What happens if I forget to call `delete` on an allocated object?
- This results in a memory leak, as the allocated memory will not be returned to the system.
By grasping these concepts, you’re well on your way to mastering memory management in C++, ensuring your applications run efficiently and reliably.