Discover the best free IDEs for C++ that empower you to write, debug, and optimize your code efficiently, such as Visual Studio Code or Code::Blocks.
Here's a simple C++ code snippet to demonstrate a "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is an IDE?
An Integrated Development Environment (IDE) is a comprehensive software application that provides developers with the tools they need to write, compile, debug, and manage their code efficiently. Key features that an IDE typically includes are:
- Code Editing: IDEs feature advanced text editors with syntax highlighting, auto-completion, and error detection, which help programmers write code more efficiently.
- Debugging: Effective debugging tools that allow developers to trace errors in their applications are essential for maintaining code quality.
- Compilation and Build Management: IDEs streamline the building process by integrating compilers and build systems, making it easier to turn source code into executable applications.
- Project Management: Organizing code files into projects helps keep related files together, enhancing collaboration and efficiency.
- Version Control Integration: Many IDEs offer built-in support for version control tools like Git, enabling smoother collaboration among developers.
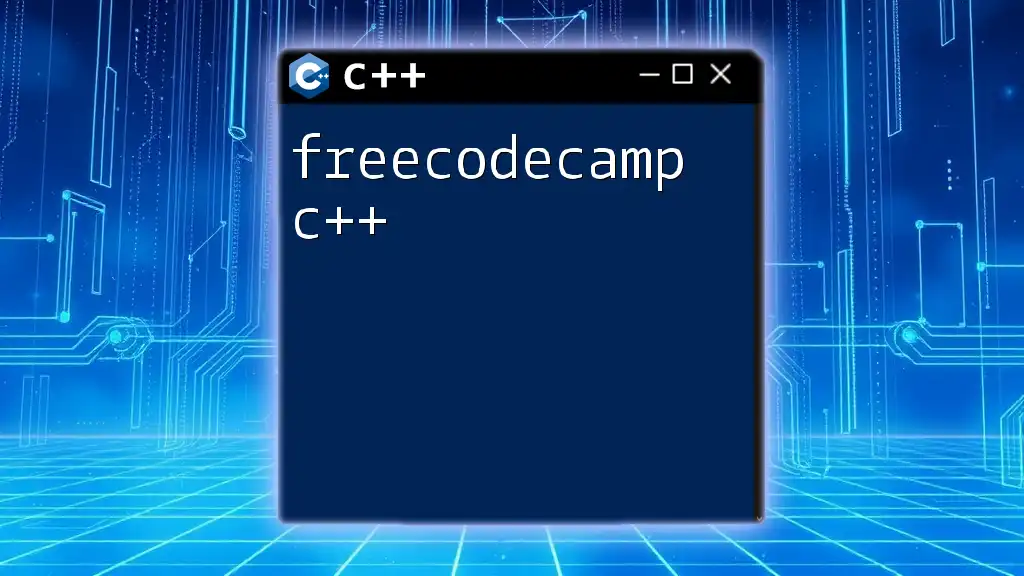
Why Choose Free IDEs for C++?
Opting for free IDEs for C++ programming offers several advantages:
- Cost-Effectiveness: For beginners or those learning out of interest, free IDEs eliminate financial barriers, enabling access to necessary tools without upfront investment.
- Community-Driven Ecosystem: Free IDEs often benefit from large user communities, providing a wealth of tutorials, forums, and plugins to enhance the tool's functionalities.
- Flexibility and Customization: Many free IDEs are highly customizable, allowing developers to tailor the environment to their specific needs and preferences.
Moreover, while proprietary IDEs may offer advanced features, many free IDEs can compete effectively, especially for newcomers.
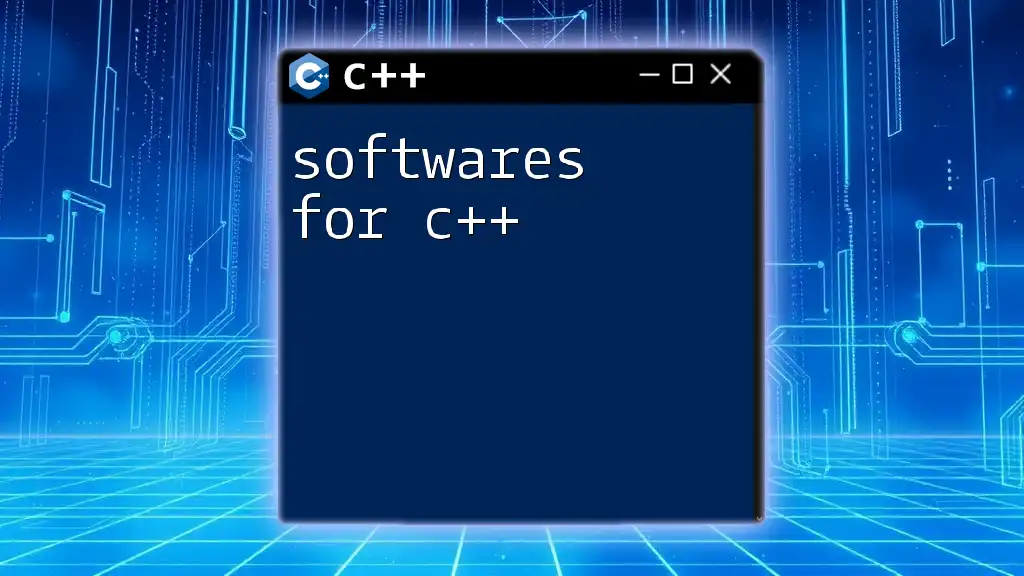
Popular Free C++ IDEs
Code::Blocks
Code::Blocks is a free, open-source IDE designed specifically for C++ programming. It is known for its versatility and flexibility.
Key Features:
- Cross-Platform Support: Available on Windows, Linux, and macOS, making it suitable for a wide range of developers.
- Modular Plugin System: Users can enhance the IDE's functionality through various plugins.
- Easy to Set Up: The installation process is straightforward, requiring minimal configuration.
Installation Guide: Installing Code::Blocks on Windows is simple. Visit the [official website](https://www.codeblocks.org/downloads/) and download the binary release. Once downloaded, run the installer and select the components you wish to install, including the MinGW compiler.
Example Usage: To write a simple "Hello World" program in Code::Blocks, follow these steps:
- Open Code::Blocks and create a new project.
- Select "Console Application", then choose C++.
- Write the following code in the main file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
- Press F9 to compile and run the program.
Pros and Cons: While Code::Blocks is powerful and versatile, it may not be as beginner-friendly as other IDEs, and its user interface could benefit from modernization.
Eclipse CDT
Eclipse CDT (C/C++ Development Tooling) offers a robust and powerful IDE experience.
Key Features:
- Powerful Code Analysis Tools: Helps maintain code quality and catch potential issues early in development.
- Comprehensive Debugging Capabilities: Equipped with various debugging tools for breakpoints, watchpoints, and variables inspection.
Installation Guide: To set up Eclipse CDT on Windows, download the Eclipse IDE from the [Eclipse downloads page](https://www.eclipse.org/downloads/). During installation, choose the C/C++ package. Once installed, confirm that you have a C++ compiler set up in your system.
Example Usage: Creating a basic C++ project in Eclipse involves the following steps:
- Open Eclipse and select "File" > "New" > "C++ Project".
- Choose "Hello World C++ Project" and select the appropriate toolchain.
- Write your code in the generated main file.
- Compile and run with the provided toolbar buttons.
Pros and Cons: Eclipse offers extensive features but can be overwhelming for beginners due to its complexity and large memory usage.
Dev-C++
Dev-C++ is a lightweight and user-friendly IDE primarily for Windows users.
Key Features:
- Lightweight and Easy to Use: Quick installation and minimal resource usage.
- Built-in Compiler: Comes with the MinGW compiler, allowing for immediate code execution.
Installation Guide: To install Dev-C++, download it from [Bloodshed Software](http://www.bloodshed.net/devcpp.html). Execute the downloaded file and follow the installation prompts.
Example Usage: To compile and run a C++ file in Dev-C++, do the following:
- Open Dev-C++ and create a new source file.
- Insert the C++ code for a simple application.
- Save the file and click on "Execute" > "Compile & Run".
Pros and Cons: Dev-C++ is suitable for beginners looking for simplicity but may lack advanced features available in other IDEs.
Visual Studio Community
Visual Studio Community Edition is a powerful IDE from Microsoft, offering a range of features at no cost to individual developers.
Key Features:
- Rich IntelliSense Support: Auto-completion and smart suggestions greatly enhance coding efficiency.
- Advanced Debugging Tools: Offers an intuitive debugger that simplifies the debugging process.
Installation Guide: To set up Visual Studio Community on Windows, download it from the [Visual Studio downloads page](https://visualstudio.microsoft.com/). During installation, select the "Desktop development with C++" workload.
Example Usage: Building a console application in Visual Studio involves the following:
- Open Visual Studio and create a new project.
- Select "Console App".
- Write your code in the generated main file.
- Click the "Start" button for compilation and execution.
Pros and Cons: While Visual Studio provides robust features, it can be resource-intensive and may not run as smoothly on lower-end machines.
CLion (30-Day Free Trial)
CLion, developed by JetBrains, is a potent and modern IDE, though it requires a subscription after the trial period.
Key Features:
- Smart C++ Coding Assistance: Auto-completion, code generation, and refactorings save time.
- Integrated Debugger: Efficiently pinpoint issues and analyze program behavior.
Installation Guide: To install CLion, download it from the [JetBrains site](https://www.jetbrains.com/clion/). Follow the prompts for installation and activate the 30-day trial.
Example Usage: Utilizing refactoring tools can make code maintenance easier. To refactor a function name, right-click on the function name in the code and choose "Refactor" > "Rename", then input the new name.
Pros and Cons: While CLion is feature-rich, it is primarily suited for those who are familiar with JetBrains products and prefer a modern interface.
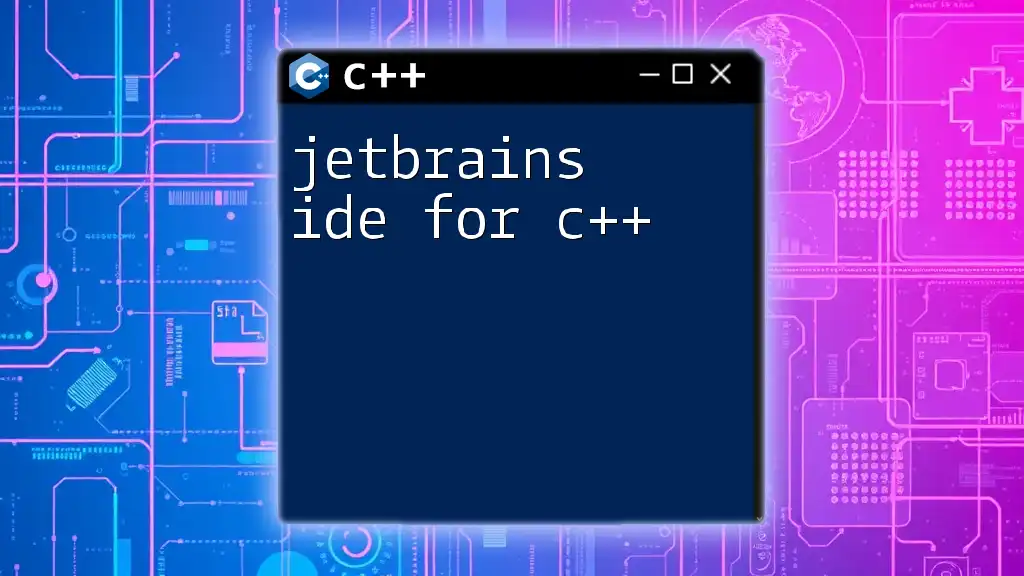
Choosing the Right C++ IDE for You
When deciding on the most suitable C++ IDE, consider factors such as:
- Platform Compatibility: Ensure the IDE is compatible with your operating system.
- Feature Set vs. Simplicity: If you're a beginner, prioritize ease-of-use over advanced features.
- Community and Support: A strong community can provide valuable resources and support.
For beginners, lightweight IDEs like Dev-C++ may offer the best introduction. Intermediate programmers might prefer Code::Blocks or Eclipse CDT for more extensive functionalities. Advanced users might find Visual Studio Community or CLion more fitting due to their robust feature sets.
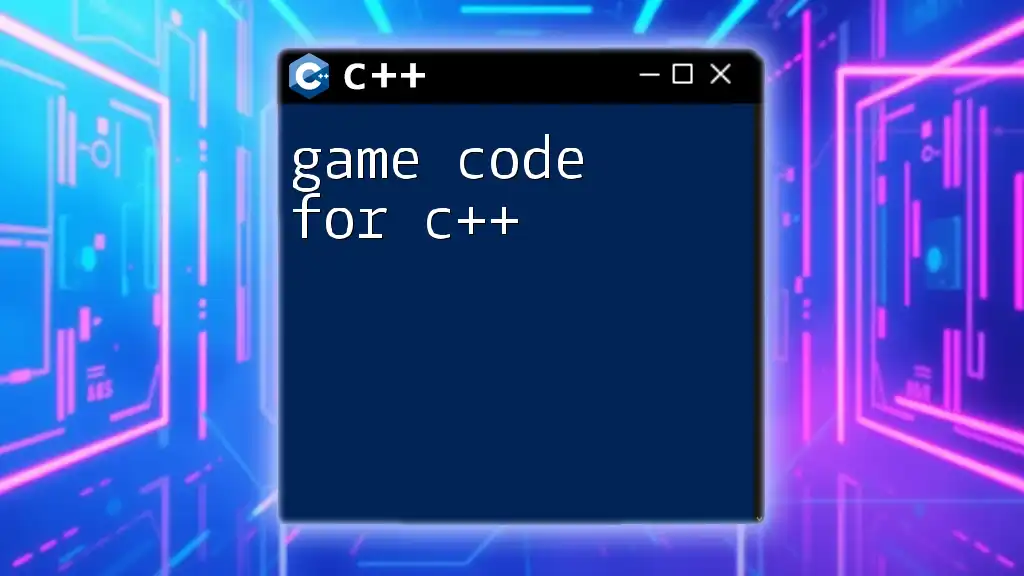
Additional Resources
To further explore free IDE options for C++, check the following:
- Official websites for each IDE provided in the previous sections.
- Community forums like Stack Overflow and Reddit for user experiences and tips.
- Online tutorials and courses that detail using these IDEs effectively.
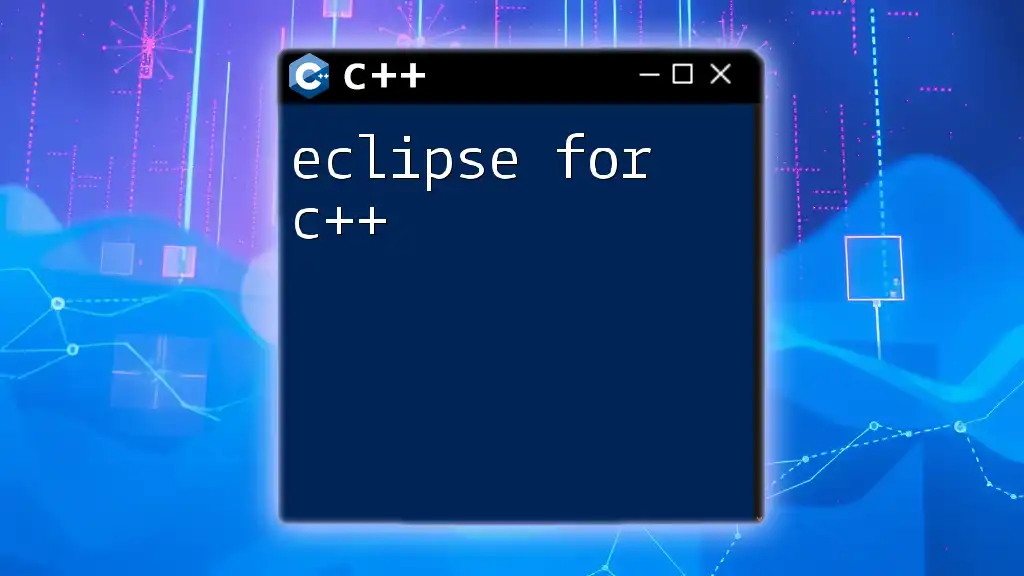
Conclusion
Choosing the right IDE is a vital step in becoming a proficient C++ programmer. Each IDE offers unique features and capabilities, making it essential to explore different options. Trying out various IDEs will help you find the one that aligns with your workflow and preferences. Engage with the community, share your experiences, and dive deeper into C++ programming!
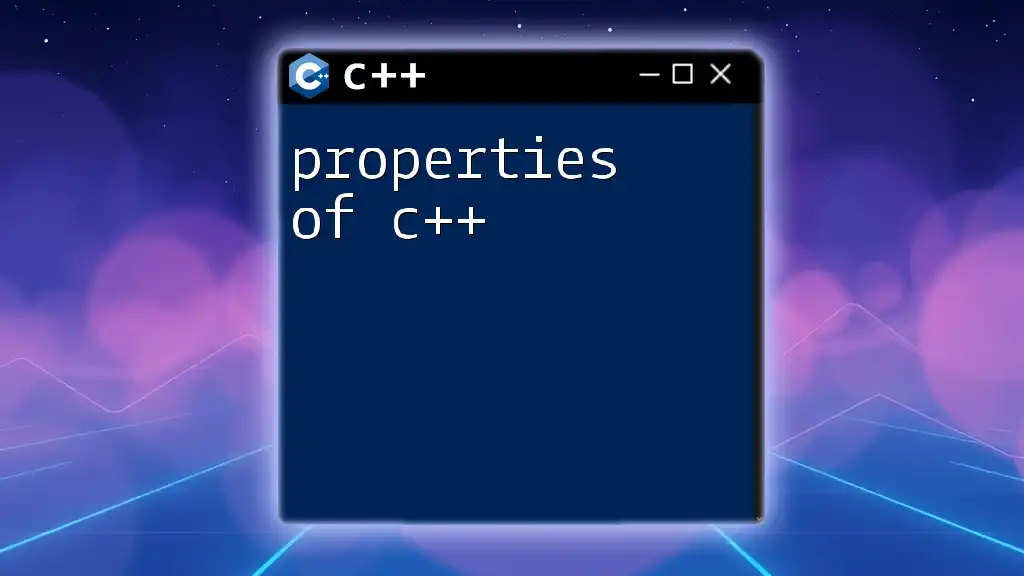
FAQs
What is the best free IDE for beginners?
Dev-C++ is often recommended for its simplicity, making it an excellent choice for newcomers to C++.
Are free IDEs as good as paid ones?
Many free IDEs offer comparable features to paid alternatives, especially for basic and intermediate programming tasks.
How do I install a C++ IDE on Linux?
Most free IDEs have Linux installations available on their official sites. Check the documentation for specific instructions based on your distribution.