FreeCodeCamp offers a variety of resources for learning C++, enabling users to master essential commands and syntax through hands-on practice.
Here's a basic example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is FreeCodeCamp?
FreeCodeCamp is a non-profit organization dedicated to making learning web development and programming accessible to everyone. Their mission is to help people learn to code for free, promoting the idea that anyone can gain valuable skills, regardless of their background or financial situation.
This platform promotes a community-driven ethos, allowing learners from around the world to interact, support each other, and share knowledge. The website offers a wealth of resources, including interactive tutorials, articles, and hands-on projects, making it an excellent place for aspiring programmers.
Available Learning Resources
FreeCodeCamp's resources are diverse and designed to cater to various learning styles:
- Interactive Coding Challenges: Users can practice coding by solving challenges directly in their browsers.
- Comprehensive Tutorials: In-depth articles and tutorials explain different programming concepts clearly and effectively.
- Projects: Practical, real-world projects allow learners to apply their skills, from building a simple calculator to more complex applications.
By leveraging these resources, learners can deepen their understanding of programming languages like C++.

Getting Started with C++ on FreeCodeCamp
Setting Up Your Development Environment
Before diving into C++, it's essential to set up a development environment. Here are some popular Integrated Development Environments (IDEs) you might consider:
- Code::Blocks: A user-friendly IDE that supports multiple compilers and is ideal for beginners.
- Visual Studio: A powerful IDE perfect for both novice and experienced programmers. It offers extensive features for C++ development.
- Eclipse: Known for its versatility, Eclipse has a C++ plugin that can help you develop C++ applications efficiently.
Follow the installation steps on their respective websites, where you can find detailed instructions and screenshots to guide you through. Alternatively, online compilers like repl.it or Ideone are great for quick coding experiments without any downloads.
First Steps in C++
Understanding Basic Syntax
The syntax of C++ is influenced by its predecessor, C, and follows a structured format. Here’s a simple example demonstrating the basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Key components include:
- `#include <iostream>`: This preprocessor directive includes the Input/Output stream library, necessary for using `std::cout`.
- `int main()`: This function is the entry point of every C++ program.
- `std::cout << "Hello, World!"`: Here, `std::cout` prints the output to the console.
Grasping the structure of a simple program like this lays a solid foundation for further learning in C++.
Variables and Data Types
Understanding variables and data types is critical for any programming language. In C++, you can declare variables to hold different types of data, such as integers, floats, characters, and booleans. Here's an example:
int age = 30;
float salary = 85000.50;
char grade = 'A';
In this snippet:
- int: Represents integer values.
- float: Represents decimal numbers, which require more precision.
- char: Indicates a single character.
Variables have scope (where they can be accessed in the code) and lifetime (how long they exist in memory), which is essential to managing data effectively.
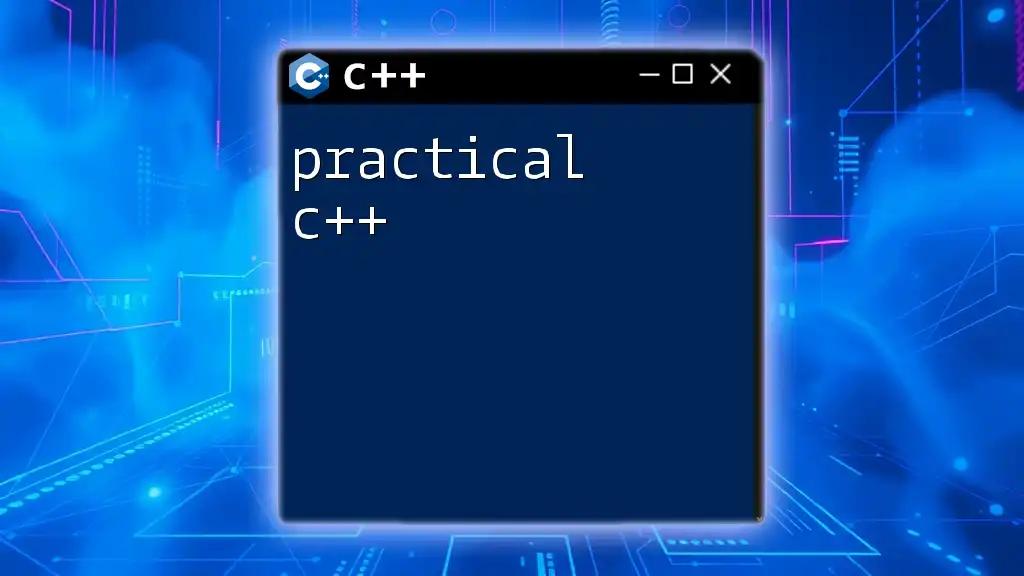
C++ Fundamental Concepts
Control Structures
Control structures allow you to dictate the flow of your program. Two significant types include conditional statements and loops.
Conditional Statements
Using conditional statements such as `if`, `else`, and `switch` is vital for determining how your program executes under certain conditions. Here’s an example of an `if` statement:
int number = 10;
if (number > 0) {
std::cout << "Positive Number" << std::endl;
} else {
std::cout << "Negative Number" << std::endl;
}
This code evaluates whether the `number` variable is greater than zero and prints a corresponding message. Understanding when and how to use these constructs is crucial for effective problem-solving in programming.
Loops
Loops facilitate the repetition of code based on specific conditions. C++ supports several types of loops:
- for loops: Useful when the number of iterations is known.
- while loops: Best when the number of iterations isn't predetermined.
- do-while loops: Similar to `while`, but guarantees at least one iteration.
Here’s an example of a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
In this example, the loop will print the numbers from 0 to 4, demonstrating how loops can simplify tasks that require repetition.
Functions in C++
Functions are fundamental in breaking down programs into manageable chunks. They encapsulate reusable pieces of code. Here’s a simple function example illustrating how to define and use functions in C++:
int add(int a, int b) {
return a + b;
}
int main() {
int sum = add(5, 10);
std::cout << "Sum: " << sum << std::endl;
return 0;
}
In this code, the `add` function receives two integers, performs an addition, and returns the result, which is then printed in the `main` function.

Object-Oriented Programming in C++
Classes and Objects
C++ is well-known for its object-oriented programming (OOP) capabilities. OOP allows programmers to model real-world entities as objects. Here’s an example of defining a class and creating an object:
class Car {
public:
std::string model;
Car(std::string m) {
model = m;
}
};
In this example, the `Car` class has a public attribute called `model` and a constructor to initialize it. Creating objects from classes provides both encapsulation and organization to your code.
Inheritance and Polymorphism
Inheritance enables a new class (child) to inherit properties from another class (parent). This practical feature enhances code reusability. Here’s a brief look at polymorphism:
class Animal {
public:
virtual void sound() {
std::cout << "Animal sound" << std::endl;
}
};
The `virtual` keyword allows derived classes to override the `sound` function, providing flexibility and enabling dynamic method resolution.
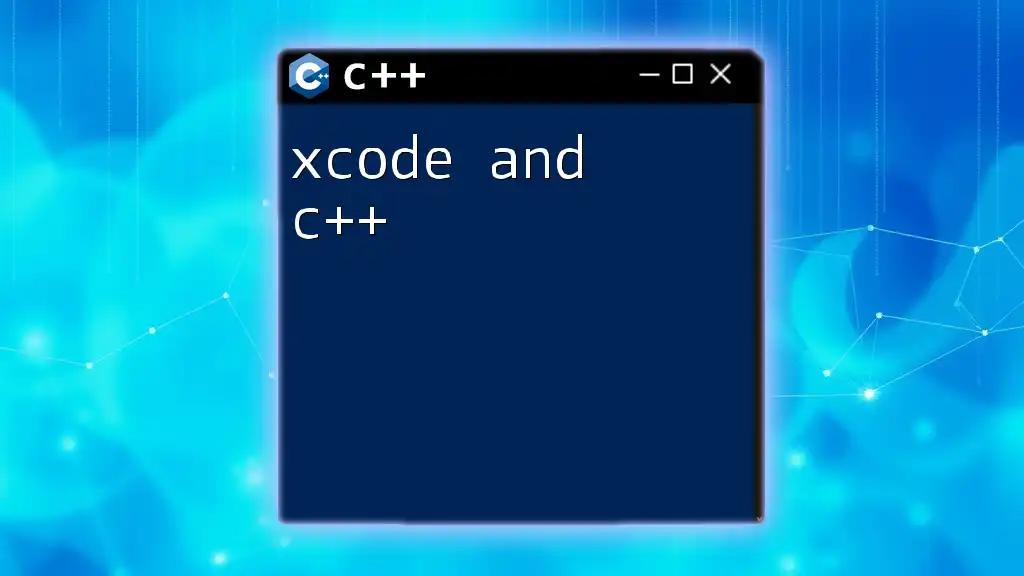
Projects and Challenges on FreeCodeCamp
Project Ideas
FreeCodeCamp offers various projects that cater to different skill levels. Here are some project ideas to help you apply your C++ knowledge:
- Calculator: Build a simple command-line calculator that performs basic arithmetic operations.
- To-Do List: Create a program that allows users to add, remove, and display tasks.
Engaging in these projects reinforces learning and provides a practical platform to apply your skills.
Utilizing Challenges to Enhance Skills
FreeCodeCamp is filled with algorithm challenges that can sharpen your problem-solving abilities. Approaching competitive programming as a challenge can help you develop creative solutions and improve your proficiency in C++.

Community and Support
Engaging with FreeCodeCamp Community
The FreeCodeCamp community is a powerful resource for networking and support. Engage in forums, join chat groups, or participate in local meetups to share experiences and foster friendships with fellow learners. These interactions provide not only knowledge but also motivation.
Seeking Help and Resources
Don’t hesitate to leverage documentation, seek help from more experienced programmers, and utilize online forums. Study groups can be invaluable, and connecting with a mentor can accelerate your learning journey.
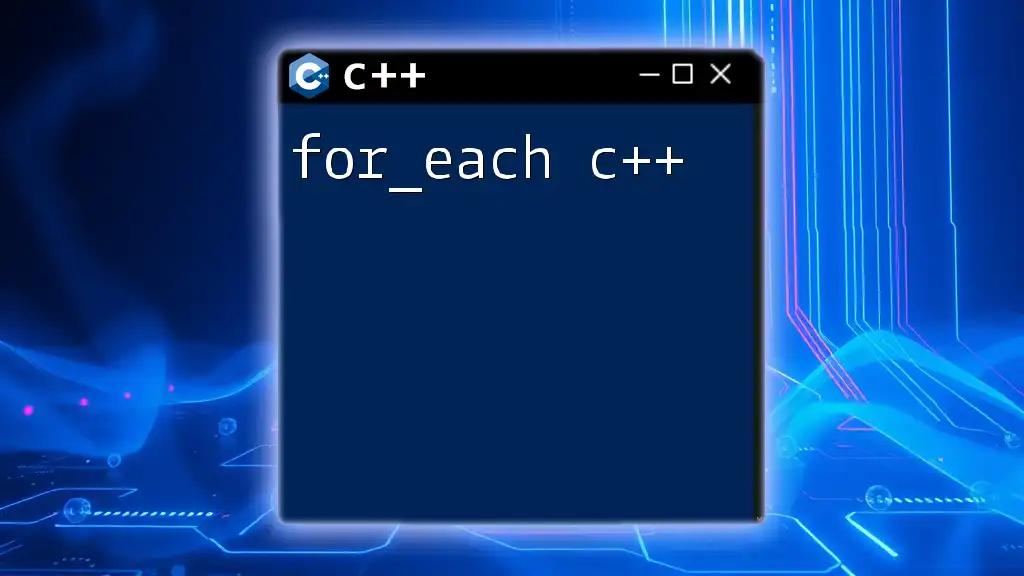
Conclusion
FreeCodeCamp offers an incredible opportunity for anyone interested in mastering C++. By making use of the platform's extensive resources, engaging in hands-on projects, and connecting with the community, learners can accelerate their skills in this powerful programming language.
Pursuing knowledge in C++ through FreeCodeCamp can open doors to various career paths in software development, game design, and more. Remember, the journey of learning to code is a marathon, not a sprint. Embrace the process, and keep coding!

Frequently Asked Questions
As you navigate your learning journey, you may encounter common questions regarding FreeCodeCamp and the C++ learning pathway. Here are a few insights to help you along the way:
-
What is the best way to learn C++ efficiently? Regular practice through challenges and projects, along with consistent engagement in the FreeCodeCamp community, can accelerate your learning.
-
How can I overcome challenges while learning C++? Don’t hesitate to ask for help and utilize community resources. Breaking problems into smaller parts can make them more manageable.
-
What are the career prospects with C++ skills? C++ knowledge can lead to opportunities in various fields, including software development, game development, and systems programming.
By maintaining a curious mindset and actively seeking knowledge, you can unlock the full potential of C++ and tap into numerous opportunities in the tech industry. Happy coding!